实验要求
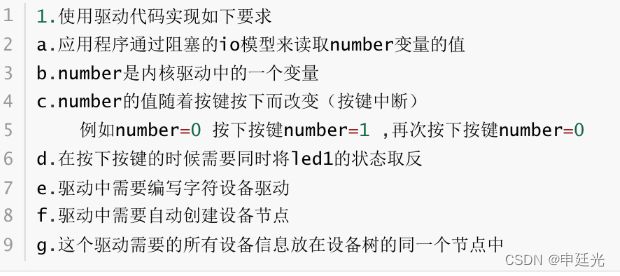
驱动代码
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
int i;
struct resource *res;
struct device_node *dnode;
unsigned int irqno[3];
unsigned int irqflag;
struct gpio_desc *gpiono[3];
unsigned char number;
struct cdev *cdev;
char kbuf[128] = {0};
unsigned int major = 0;
unsigned int minor = 0;
dev_t devno;
module_param(major, uint, 0664); // 方便在命令行传递major的值
struct class *cls;
struct device *dev;
unsigned int condition = 0;
// 定义一个等待队列头
wait_queue_head_t wq_head;
// 定义中断处理函数
irqreturn_t key_handler(int irq, void *dev)
{
int which = (int)dev;
if(number==0)
{
number=1;
kbuf[0]='1';
}else
{
number=0;
kbuf[0]='0';
}
switch (which)
{
case 0:
gpiod_set_value(gpiono[0], number);
break;
case 1:
gpiod_set_value(gpiono[1], number);
break;
case 2:
gpiod_set_value(gpiono[2], number);
break;
}
condition = 1; // 表示硬件数据就绪
wake_up_interruptible(&wq_head);
return IRQ_HANDLED;
}
// 封装probe函数
int pdrv_probe(struct platform_device *pdev)
{
res = platform_get_resource(pdev, IORESOURCE_MEM, 0);
if (res == NULL)
{
printk("获取men资源失败\n");
return -ENXIO;
}
printk("mem资源%x\n", res->start);
for (i = 0; i < 3; i++)
{
irqno[0] = platform_get_irq(pdev, 0);
}
if (irqno[0] < 0)
{
printk("获取中断类型资源失败\n");
return -ENXIO;
}
printk("irq资源%d\n", irqno[0]);
printk("%s:%s:%d\n", __FILE__, __func__, __LINE__);
// 设备树匹配成功后,设备树节点指针可以通过pdev->dev.of_node获取
// 基于设备树节点信息获取gpio_desc对象指针
gpiono[0] = gpiod_get_from_of_node(pdev->dev.of_node, "led1-gpio", 0, GPIOD_OUT_LOW, NULL);
if (IS_ERR(gpiono))
{
printk("解析GPIO管脚信息失败\n");
return -ENXIO;
}
gpiono[1] = gpiod_get_from_of_node(pdev->dev.of_node, "led2-gpio", 0, GPIOD_OUT_LOW, NULL);
if (IS_ERR(gpiono))
{
printk("解析GPIO管脚信息失败\n");
return -ENXIO;
}
gpiono[2] = gpiod_get_from_of_node(pdev->dev.of_node, "led3-gpio", 0, GPIOD_OUT_LOW, NULL);
if (IS_ERR(gpiono))
{
printk("解析GPIO管脚信息失败\n");
return -ENXIO;
}
return 0;
}
// 封装操作方法
int mycdev_open(struct inode *inode, struct file *file)
{
printk("%s:%s:%d\n", __FILE__, __func__, __LINE__);
return 0;
}
int mycdev_close(struct inode *inode, struct file *file)
{
printk("%s:%s:%d\n", __FILE__, __func__, __LINE__);
return 0;
}
ssize_t mycdev_read(struct file *file, char *ubuf, size_t size, loff_t *lof)
{
int ret;
// 判断IO方式
if (file->f_flags & O_NONBLOCK) // 非阻塞
{
}
else // 阻塞
{
wait_event_interruptible(wq_head, condition); // 先检查condition再将进程休眠
}
ret = copy_to_user(ubuf, kbuf, size);
if (ret)
{
printk("copy_to_user err\n");
return -EIO;
}
condition = 0; // 下一次硬件数据没有就绪
return 0;
}
// 定义操作方法结构体变量并赋值
struct file_operations fops = {
.open = mycdev_open,
.release = mycdev_close,
.read = mycdev_read,
};
// 封装remvoe函数
int pdrv_remove(struct platform_device *pdev)
{
// 释放GPIO信息
for (i = 0; i < 3; i++)
{
gpiod_put(gpiono[i]);
}
int i;
for (i = 0; i < 3; i++)
{
free_irq(irqno[i], (void *)i);
}
// 销毁设备文件
// 注销驱动
printk("%s:%s:%d\n", __FILE__, __func__, __LINE__);
return 0;
}
// 构建设备树匹配表
struct of_device_id oftable[] = {
{.compatible = "hqyj,myplatform"},
{},
};
// 定义驱动信息对象并初始化
struct platform_driver pdrv = {
.probe = pdrv_probe,
.remove = pdrv_remove,
.driver = {
.name = "ccccc",
.of_match_table = oftable, // 用来设备树匹配
},
};
static int __init mycdev_init(void)
{
// 注册
platform_driver_register(&pdrv);
// 解析按键的设备树节点
dnode = of_find_compatible_node(NULL, NULL, "hqyj,myirq");
if (dnode == NULL)
{
printk("解析设备树节点失败\n");
return -ENXIO;
}
printk("解析设备树节点成功\n");
// 解析按键的软中断号
int i;
for (i = 0; i < 3; i++)
{
irqno[i] = irq_of_parse_and_map(dnode, i);
if (!irqno[i])
{
printk("解析按键1软中断号失败\n");
return -ENXIO;
}
printk("解析按键软中断号成功%d\n", irqno[i]);
// 注册 按键中断
int ret = request_irq(irqno[i], key_handler, IRQF_TRIGGER_FALLING, "key_int", (void *)i);
if (ret < 0)
{
printk("注册按键中断失败\n");
return ret;
}
}
printk("注册按键中断成功\n");
init_waitqueue_head(&wq_head);
int ret;
// 为字符设备驱动对象申请空间
cdev = cdev_alloc();
if (cdev == NULL)
{
printk("字符设备驱动对象申请空间失败\n");
ret = -EFAULT;
goto out1;
}
printk("申请对象空间成功\n");
// 初始化字符设备驱动对象
cdev_init(cdev, &fops);
// 申请设备号
if (major > 0) // 静态指定设备号
{
ret = register_chrdev_region(MKDEV(major, minor), 3, "myled");
if (ret)
{
printk("静态申请设备号失败\n");
goto out2;
}
}
else if (major == 0) // 动态申请设备号
{
ret = alloc_chrdev_region(&devno, minor, 3, "myled");
if (ret)
{
printk("动态申请设备号失败\n");
goto out2;
}
major = MAJOR(devno); // 获取主设备号
minor = MINOR(devno); // 获取次设备号
}
printk("申请设备号成功\n");
// 注册字符设备驱动对象
ret = cdev_add(cdev, MKDEV(major, minor), 3);
if (ret)
{
printk("注册字符设备驱动对象失败\n");
goto out3;
}
printk("注册字符设备驱动对象成功\n");
// 向上提交目录信息
cls = class_create(THIS_MODULE, "myled");
if (IS_ERR(cls))
{
printk("向上提交目录失败\n");
ret = -PTR_ERR(cls);
goto out4;
}
printk("向上提交目录成功\n");
// 向上提交设备节点信息
for (i = 0; i < 3; i++)
{
dev = device_create(cls, NULL, MKDEV(major, i), NULL, "myled%d", i);
if (IS_ERR(dev))
{
printk("向上提交设备节点信息失败\n");
ret = -PTR_ERR(dev);
goto out5;
}
}
printk("向上提交设备信息成功\n");
return 0;
out5:
// 释放前一次提交成功的设备信息
for (--i; i >= 0; i--)
{
device_destroy(cls, MKDEV(major, i));
}
class_destroy(cls); // 释放目录
out4:
cdev_del(cdev);
out3:
unregister_chrdev_region(MKDEV(major, minor), 3);
out2:
kfree(cdev);
out1:
return ret;
return 0;
}
static void __exit mycdev_exit(void)
{
// 注册
platform_driver_unregister(&pdrv);
}
module_init(mycdev_init);
module_exit(mycdev_exit);
MODULE_LICENSE("GPL");
应用程序代码
#include
#include
#include
#include
#include
#include
#include
#include
int main(int argc, char const *argv[])
{
char buf[128] = {0};
int fd = open("/dev/myled0", O_RDWR);
if (fd < 0)
{
printf("打开设备文件失败\n");
exit(-1);
}
while (1)
{
read(fd,buf,sizeof(buf));
printf("%s\n",buf);
}
return 0;
}
实验现象
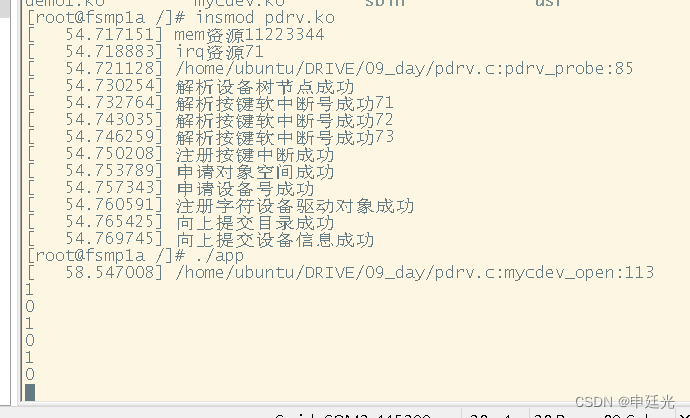