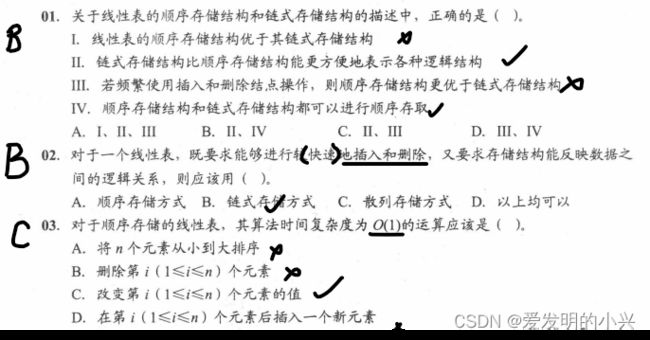
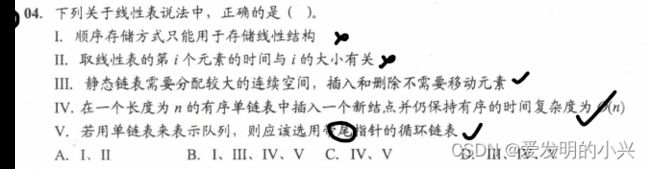
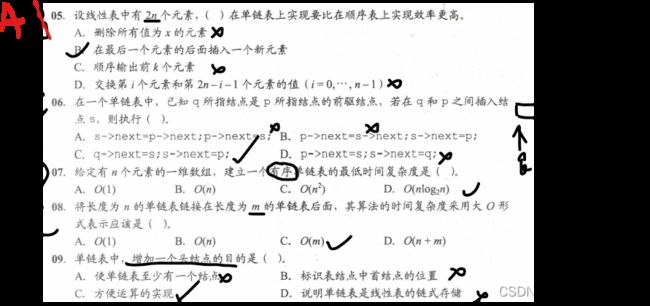
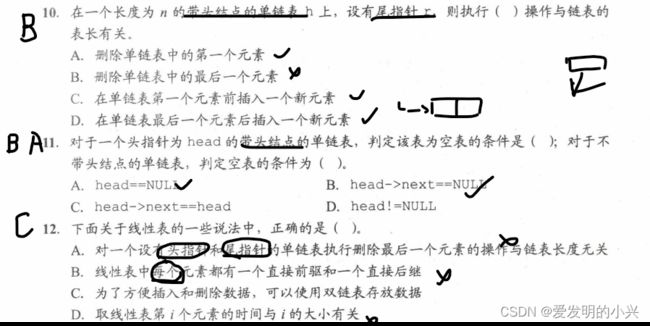
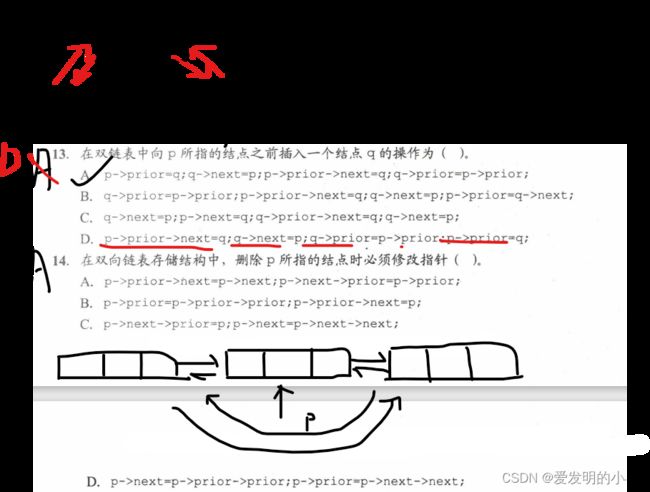
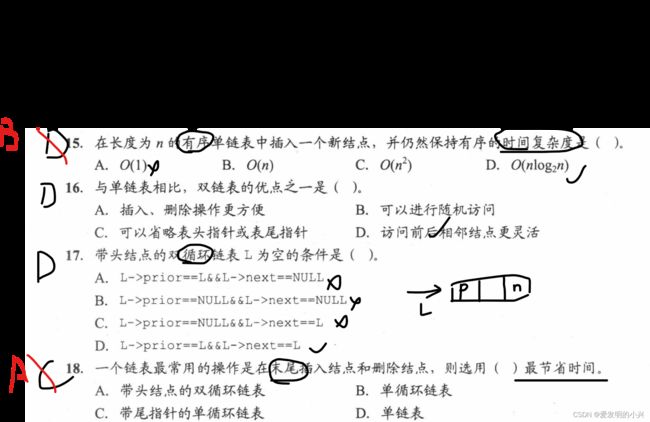


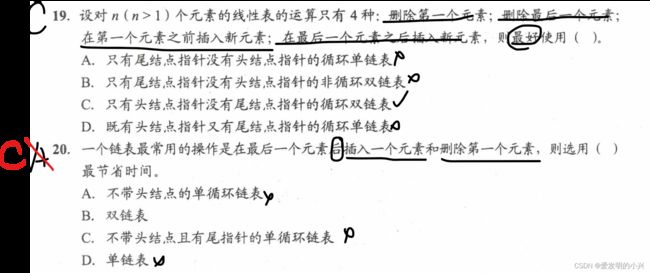
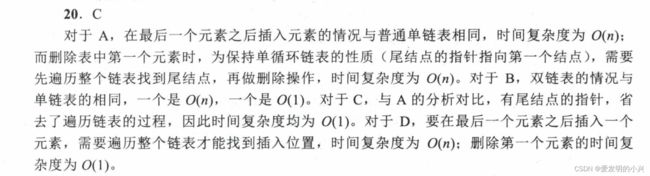
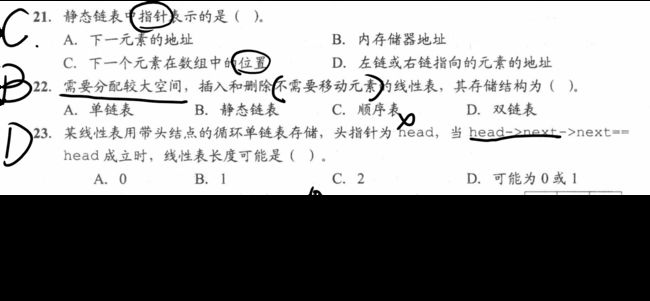
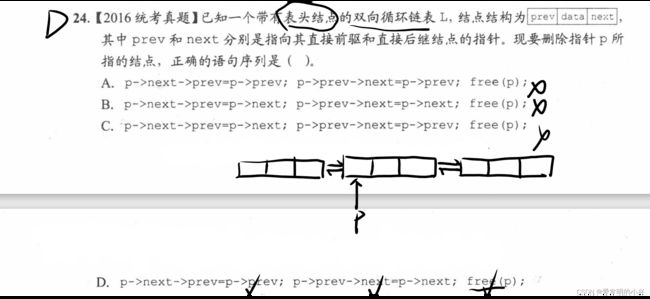
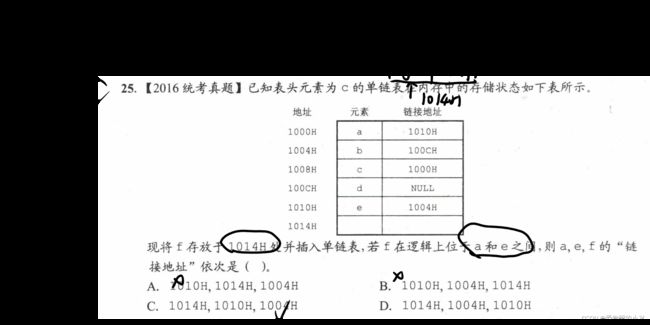
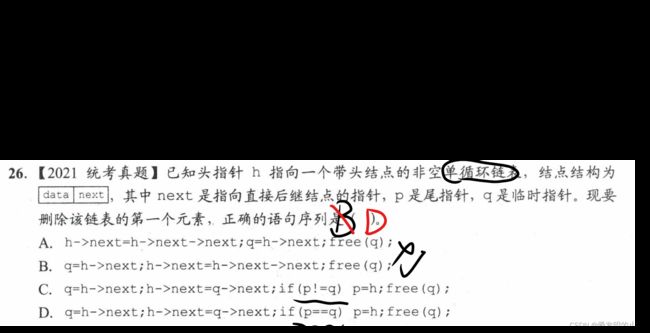
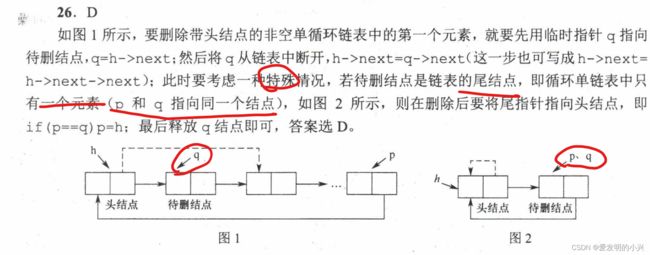

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool ListEmpty(LinkList L)
{
if (L!= NULL)
{
cout << "not empty,不空" << endl;
return OK;
}
else
{
cout << "empty" << endl;
return ERROR;
}
}
LinkList List_HeadInsert(LinkList& L)
{
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L;
L = s;
if (cin.get() == '\n') break;
}
return L;
}
bool Del_X(LinkList& L, Elemtype x)
{
LNode* p = NULL;
if (L == NULL)
return ERROR;
if (L->data == x)
{
p = L;
L = L->next;
free(p);
Del_X(L, x);
}
else
Del_X(L->next, x);
}
int main(void)
{
LinkList L = NULL;
List_HeadInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
ListEmpty(L);
Del_X(L, 3);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
bool Del_X(LinkList& L, Elemtype x)
{
LNode* p = L->next;
LNode* pre = L;
LNode* q = NULL;
if (L->next == NULL)
return ERROR;
while (p != NULL)
{
if (p->data == x)
{
q = p;
p = p->next;
pre->next = p;
free(q);
}
else
{
pre = p;
p = p->next;
}
}
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
Del_X(L, 3);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void R_Print(LinkList L)
{
if (L->next != NULL)
R_Print(L->next);
if (L != NULL) printf("L->data=%d\n",L->data);
}
void R_Ignore_Head(LinkList L)
{
if (L->next != NULL) R_Print(L->next);
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
R_Ignore_Head(L);
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void Delete_Min(LinkList& L)
{
LNode* pre = L, * p = pre->next;
LNode* minpre = pre, * minp = p;
while (p != NULL)
{
if (p->data < minp->data)
{
minp = p;
minpre = pre;
}
pre = p;
p = p->next;
}
minpre->next = minp->next;
free(minp);
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
Delete_Min(L);
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList Reverse_1(LinkList L)
{
LNode* p, * r;
p = L->next;
L->next = NULL;
while (p != NULL)
{
r = p->next;
p->next = L->next;
L->next = p;
p = r;
}
return L;
}
LinkList Reverse_2(LinkList L)
{
LNode* pre, * p = L->next, * r = p->next;
p->next = NULL;
while (r != NULL)
{
pre = p;
p = r;
r = r->next;
p->next = pre;
}
L->next = p;
return L;
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
Reverse_2(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void Sort(LinkList& L)
{
LNode* p = L->next, * pre;
LNode* r = p->next;
p->next = NULL;
p = r;
while (p != NULL)
{
r = p->next;
pre = L;
while (pre->next != NULL && pre->next->data < p->data)
pre = pre->next;
p->next = pre->next;
pre->next = p;
p = r;
}
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
Sort(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void RangeDelete(LinkList& L, int min,int max)
{
LNode* p = L->next, * pre = L, *q = NULL;
while (p != NULL)
{
if ((min < (p->data)) && ((p->data) < max))
{
q = p;
pre->next = p->next;
p = p->next;
free(q);
}
pre = p;
p = p->next;
}
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
RangeDelete(L, 3, 5);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList Search_Common(LinkList L1, LinkList L2)
{
int length1 = 0, length2 = 0;
int distance = 0;
length1=ListLength(L1);
length2=ListLength(L2);
cout << "length1=" << length1 << " length2=" << length2 << endl;
LinkList longList=NULL, shortList= NULL;
if (length1 > length2)
{
longList = L1->next;
shortList = L2->next;
distance = length1 - length2;
}
else
{
longList = L2->next;
shortList = L1->next;
distance = length2 - length1;
}
while (distance--)
longList = longList->next;
while (longList != NULL)
{
if (longList = shortList)
return longList;
else
{
longList = longList->next;
shortList = shortList->next;
}
}
return NULL;
}
int main(void)
{
LinkList L1 = NULL;
List_TailInsert(L1);
cout << L1 << " " << L1->data << " " << L1->next << endl;
cout << L1->next << " " << L1->next->data << " " << L1->next->next << endl;
cout << L1->next->next << " " << L1->next->next->data << " " << L1->next->next->next << endl;
cout << L1->next->next->next << " " << L1->next->next->next->data << " " << L1->next->next->next->next << endl;
cout << L1->next->next->next->next << " " << L1->next->next->next->next->data << " " << L1->next->next->next->next->next << endl;
cout << L1->next->next->next->next->next << " " << L1->next->next->next->next->next->data << " " << L1->next->next->next->next->next->next << endl;
ListEmpty(L1);
LinkList L2 = NULL;
List_TailInsert(L2);
cout << L2 << " " << L2->data << " " << L2->next << endl;
cout << L2->next << " " << L2->next->data << " " << L2->next->next << endl;
cout << L2->next->next << " " << L2->next->next->data << " " << L2->next->next->next << endl;
cout << L2->next->next->next << " " << L2->next->next->next->data << " " << L2->next->next->next->next << endl;
ListEmpty(L2);
cout<<"same: "<<Search_Common(L1, L1)<<endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void Min__Delete(LinkList &head)
{
while (head->next != NULL)
{
LNode* pre = head;
LNode* p = pre->next;
LNode* u;
while (p->next != NULL)
{
if (p->next->data < pre->next->data)
pre = p;
p = p->next;
}
cout<<pre->next->data<<endl;
u = pre->next;
pre->next = u->next;
free(u);
}
free(head);
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << L << " " << L->data << " " << L->next << endl;
cout << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
ListEmpty(L);
Min__Delete(L);
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList DisCreate(LinkList& A)
{
int i = 0;
LinkList B = (LinkList)malloc(sizeof(LNode));
B->next = NULL;
LNode* ra = A, * rb = B, * p;
p = A->next;
A->next = NULL;
while (p != NULL)
{
i++;
if (i % 2 == 0)
{
rb->next = p;
rb = p;
}
else
{
ra->next = p;
ra = p;
}
p = p->next;
}
ra->next = NULL;
rb->next = NULL;
return B;
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << "-------------------------------原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout <<"头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "数据4 : " << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << "数据5 : " << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
cout << "-------------------------------奇数数据-----------------------------"<<endl;
LinkList L1 = NULL;
L1 = DisCreate(L);
L1->data = 0;
cout << "--------节点地址-数据-指针--------" << endl;
cout <<"头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1: " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2: " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3: " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "-------------------------------偶数数据-----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L1 << " " << L1->data << " "<< L1->next << endl;
cout << "数据1: " << L1->next << " " << L1->next->data << " " << L1->next->next << endl;
cout << "数据2: " << L1->next->next << " " << L1->next->next->data << " " << L1->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList DisCreate(LinkList& A)
{
LinkList B = (LinkList)malloc(sizeof(LNode));
B->next = NULL;
LNode* p = A->next, * q;
LNode* ra = A;
while (p != NULL)
{
ra->next = p;
ra = p;
p = p->next;
if (p != NULL)
{
q = p->next;
p->next = B->next;
B->next = p;
p = q;
}
}
ra->next = NULL;
return B;
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << "-------------------------------原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "数据4 : " << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << "数据5 : " << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
cout << "-------------------------------奇数数据-----------------------------" << endl;
LinkList L1 = NULL;
L1 = DisCreate(L);
L1->data = 0;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1: " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2: " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3: " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "-------------------------------偶数数据-----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L1 << " " << L1->data << " " << L1->next << endl;
cout << "数据1: " << L1->next << " " << L1->next->data << " " << L1->next->next << endl;
cout << "数据2: " << L1->next->next << " " << L1->next->next->data << " " << L1->next->next->next << endl;
return 0;
}
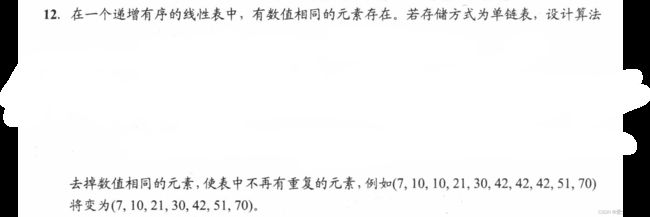
#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
bool Delete_same(LinkList& L)
{
LNode* p = L->next;
LNode* q = NULL;
if (p == NULL)
return ERROR;
while (p->next!= NULL)
{
q = p->next;
if (p->data == q->data)
{
p->next = q->next;
free(q);
}
else
p = p->next;
}
return OK;
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << "-------------------------------原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "数据4 : " << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << "数据5 : " << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
Delete_same(L);
cout << "-------------------------------修改后数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
void MergeList(LinkList& La, LinkList& Lb)
{
LNode* r, * pa = La->next, * pb = Lb->next;
La->next = NULL;
while (pa && pb)
if (pa->data <= pb->data)
{
r = pa->next;
pa->next = La->next;
La->next = pa;
pa = r;
}
else
{
r = pb->next;
pb->next = La->next;
La->next = pb;
pb = r;
}
if (pa)
pb = pa;
while (pb)
{
r = pb->next;
pb->next = La->next;
La->next = pb;
pb = r;
}
free(Lb);
}
int main(void)
{
LinkList L = NULL;
List_TailInsert(L);
cout << "-------------------------------L原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
LinkList L1 = NULL;
List_TailInsert(L1);
cout << "-------------------------------L1原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L1 << " " << L1->data << " " << L1->next << endl;
cout << "数据1 : " << L1->next << " " << L1->next->data << " " << L1->next->next << endl;
cout << "数据2 : " << L1->next->next << " " << L1->next->next->data << " " << L1->next->next->next << endl;
MergeList(L, L1);
cout << "-------------------------------修改后数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << L << " " << L->data << " " << L->next << endl;
cout << "数据1 : " << L->next << " " << L->next->data << " " << L->next->next << endl;
cout << "数据2 : " << L->next->next << " " << L->next->next->data << " " << L->next->next->next << endl;
cout << "数据3 : " << L->next->next->next << " " << L->next->next->next->data << " " << L->next->next->next->next << endl;
cout << "数据4 : " << L->next->next->next->next << " " << L->next->next->next->next->data << " " << L->next->next->next->next->next << endl;
cout << "数据5 : " << L->next->next->next->next->next << " " << L->next->next->next->next->next->data << " " << L->next->next->next->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList Get_Common(LinkList A, LinkList B)
{
LinkList C = (LinkList)malloc(sizeof(LNode));
LNode* pa = A->next;
LNode* pb = B->next;
LNode* r = C;
LNode* s = NULL;
while ((pa != NULL) && (pb != NULL))
{
if (pa->data < pb->data)
pa = pa->next;
else if (pa->data > pb->data)
pb = pb->next;
else
{
LNode* s = (LinkList)malloc(sizeof(LNode));
s->data = pa->data;
r->next = s;
r = s;
pa = pa->next;
pb = pb->next;
}
}
r->next = NULL;
return C;
}
int main(void)
{
LinkList A = NULL;
List_TailInsert(A);
cout << "-------------------------------L原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << A << " " << A->data << " " << A->next << endl;
cout << "数据1 : " << A->next << " " << A->next->data << " " << A->next->next << endl;
cout << "数据2 : " << A->next->next << " " << A->next->next->data << " " << A->next->next->next << endl;
cout << "数据3 : " << A->next->next->next << " " << A->next->next->next->data << " " << A->next->next->next->next << endl;
cout << "数据4 : " << A->next->next->next->next << " " << A->next->next->next->next->data << " " << A->next->next->next->next->next << endl;
cout << "数据5 : " << A->next->next->next->next->next << " " << A->next->next->next->next->next->data << " " << A->next->next->next->next->next->next << endl;
LinkList B = NULL;
List_TailInsert(B);
cout << "-------------------------------L1原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << B << " " << B->data << " " << B->next << endl;
cout << "数据1 : " << B->next << " " << B->next->data << " " << B->next->next << endl;
cout << "数据2 : " << B->next->next << " " << B->next->next->data << " " << B->next->next->next << endl;
cout << "数据3 : " << B->next->next->next << " " << B->next->next->next->data << " " << B->next->next->next->next << endl;
LinkList C = NULL;
C = Get_Common(A, B);
C->data = 0;
cout << "-------------------------------修改后数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << C << " " << C->data << " " << C->next << endl;
cout << "数据1 : " << C->next << " " << C->next->data << " " << C->next->next << endl;
cout << "数据2 : " << C->next->next << " " << C->next->next->data << " " << C->next->next->next << endl;
return 0;
}

#include
using namespace std;
typedef int Elemtype;
#define Maxsize 100
#define ERROR 0
#define OK 1
typedef struct LNode
{
Elemtype data;
struct LNode* next;
}LNode, * LinkList;
bool InitList(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
if (L == NULL)
return ERROR;
L->data = 0;
L->next = NULL;
return OK;
}
bool ListEmpty(LinkList L)
{
if (L->next != NULL)
{
cout << "not empty" << endl;
return OK;
}
else
{
cout << "empty,只有头节点" << endl;
return ERROR;
}
}
int ListLength(LinkList L)
{
LNode* p;
p = L->next;
int i = 0;
while (p != NULL)
{
p = p->next;
i++;
}
return i;
}
LinkList List_HeadInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
s->next = L->next;
L->next = s;
if (cin.get() == '\n') break;
}
return L;
}
LinkList List_TailInsert(LinkList& L)
{
L = (LinkList)malloc(sizeof(LNode));
L->data = 0;
L->next = NULL;
LNode* s = NULL, * r = NULL;
r = L;
int x = 0;
while (cin >> x)
{
s = (LinkList)malloc(sizeof(LNode));
s->data = x;
r->next = s;
r = s;
if (cin.get() == '\n') break;
}
s->next = NULL;
return L;
}
LinkList Get_Common(LinkList A, LinkList B)
{
LNode* pa = A->next;
LNode* pb = B->next;
LNode* u = NULL;
LNode* pc= A;
while ((pa != NULL) && (pb != NULL))
{
if (pa->data == pb->data)
{
pc->next = pa;
pc = pa;
pa = pa->next;
u = pb;
pb = pb->next;
free(u);
}
else if (pa->data < pb->data)
{
u = pa;
pa = pa->next;
free(u);
}
else
{
u = pb;
pb = pb->next;
free(u);
}
}
while (pa)
{
u = pa;
pa = pa->next;
free(u);
}
while (pb)
{
u = pb;
pb = pb->next;
free(u);
}
pc->next = NULL;
free(B);
return A;
}
int main(void)
{
LinkList A = NULL;
List_TailInsert(A);
cout << "-------------------------------L原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << A << " " << A->data << " " << A->next << endl;
cout << "数据1 : " << A->next << " " << A->next->data << " " << A->next->next << endl;
cout << "数据2 : " << A->next->next << " " << A->next->next->data << " " << A->next->next->next << endl;
cout << "数据3 : " << A->next->next->next << " " << A->next->next->next->data << " " << A->next->next->next->next << endl;
cout << "数据4 : " << A->next->next->next->next << " " << A->next->next->next->next->data << " " << A->next->next->next->next->next << endl;
cout << "数据5 : " << A->next->next->next->next->next << " " << A->next->next->next->next->next->data << " " << A->next->next->next->next->next->next << endl;
LinkList B = NULL;
List_TailInsert(B);
cout << "-------------------------------L1原始数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << B << " " << B->data << " " << B->next << endl;
cout << "数据1 : " << B->next << " " << B->next->data << " " << B->next->next << endl;
cout << "数据2 : " << B->next->next << " " << B->next->next->data << " " << B->next->next->next << endl;
cout << "数据3 : " << B->next->next->next << " " << B->next->next->next->data << " " << B->next->next->next->next << endl;
LinkList C = NULL;
C = Get_Common(A, B);
C->data = 0;
cout << "-------------------------------修改后数据----------------------------" << endl;
cout << "--------节点地址-数据-指针--------" << endl;
cout << "头节点: " << C << " " << C->data << " " << C->next << endl;
cout << "数据1 : " << C->next << " " << C->next->data << " " << C->next->next << endl;
cout << "数据2 : " << C->next->next << " " << C->next->next->data << " " << C->next->next->next << endl;
return 0;
}