SimpleDialog(简单对话框组件)
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
return MaterialApp(
title: 'SimpleDialog 组件示例',
home:Scaffold(
appBar: AppBar(title: Text('SimpleDialog 组件示例'),),
body:Center(
child: SimpleDialog(
title: const Text('对话框标题'),
children: <Widget>[
SimpleDialogOption(
child: Text('第一行信息'),
onPressed: (){},
),
SimpleDialogOption(
child: Text('第二行信息'),
onPressed: (){},
)
],
),
)
)
);
}
}
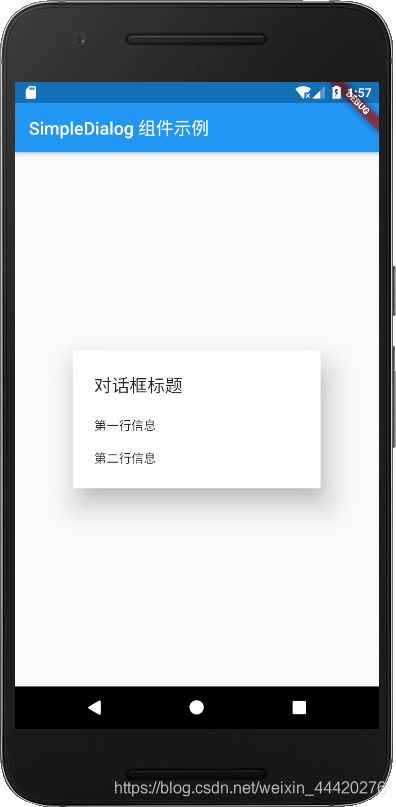
AlertDialong(提示对话框组件)
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
return MaterialApp(
title: 'AlertDialog 组件示例',
home:Scaffold(
appBar: AppBar(title: Text('AltertDialog 组件示例'),),
body:Center(
child: AlertDialog(
title: Text('提示信息'),
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
Text('是否要删除?',textAlign: TextAlign.center, style:new TextStyle(fontSize: 20.0,color: Colors.red,),),
Text('一旦删除数据不可恢复',textAlign: TextAlign.center,)
],
),
),
actions: <Widget>[
FlatButton(child: Text('确定'),onPressed: (){},),
FlatButton(child: Text('取消'),onPressed: (){},)
],
),
)
)
);
}
}
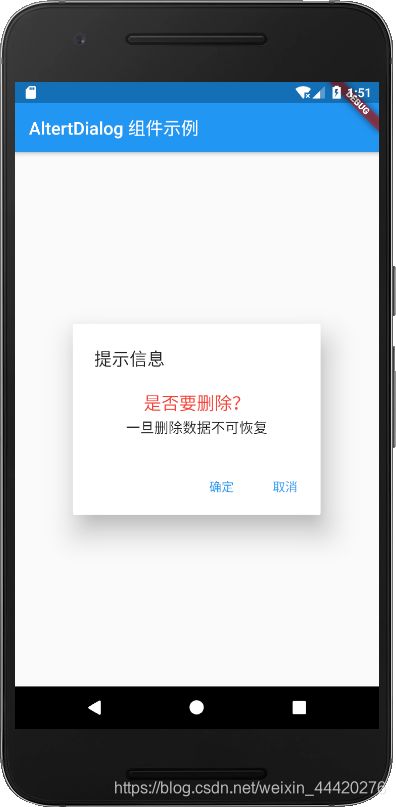
TextField(文本组件)
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget{
@override
Widget build(BuildContext context){
final TextEditingController controller = TextEditingController();
controller.addListener((){
print('您输入的是:${controller.text}');
});
return MaterialApp(
title: 'TextField 组件示例',
home:Scaffold(
appBar: AppBar(title: Text('TextField 组件示例'),),
body:Center(
child:Padding(
padding: const EdgeInsets.all(20.0),
child: TextField(
controller: controller,
maxLength: 30,
maxLines: 1,
autocorrect: true,
autofocus: true,
obscureText: false,
textAlign: TextAlign.center,
style: TextStyle(fontSize: 26.0,color: Colors.green),
onChanged: (text){
print('内容改变时的回调 $text');
},
onSubmitted: (text){
print('内容提交时的回调 $text');
},
enabled: true,
decoration: InputDecoration(
fillColor: Colors.grey.shade200,
filled: true,
helperText: '用户名',
prefixIcon: Icon(Icons.person),
suffixText: '用户名'
),
),
)
)
)
);
}
}
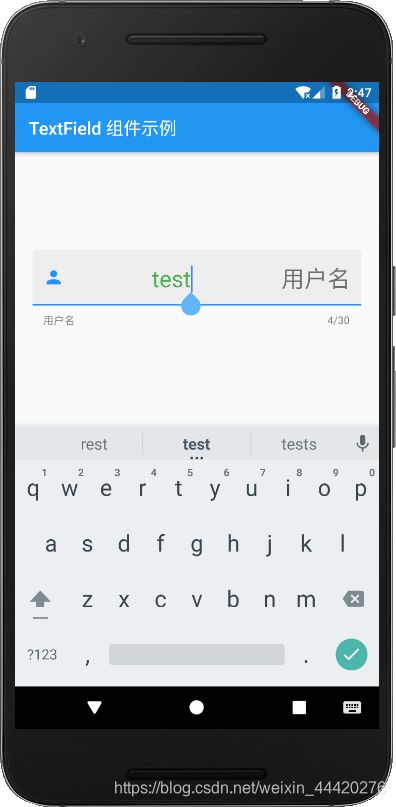
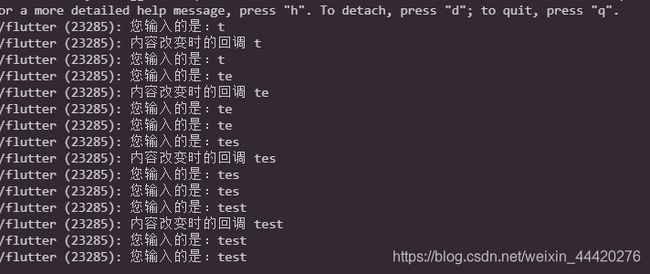
Card(卡片组件)
import 'package:flutter/material.dart';
void main() {
runApp(new MaterialApp(title: 'Card布局', home: new MyApp()));
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
var card = new SizedBox(
height: 250.0,
child: new Column(
children: <Widget>[
new ListTile(
title: new Text('火影忍者',
style: new TextStyle(fontWeight: FontWeight.w300)),
subtitle: new Text('吊车尾逆袭'),
leading: new Icon(Icons.home, color: Colors.lightBlue)),
new Divider(),
new ListTile(
title: new Text('海贼王',
style: new TextStyle(fontWeight: FontWeight.w300)),
subtitle: new Text('出海找宝藏'),
leading: new Icon(Icons.school, color: Colors.lightBlue)),
new Divider(),
],
),
);
return new Scaffold(
appBar: new AppBar(
title: new Text('Card布局'),
),
body: new Center(
child: card,
));
}
}
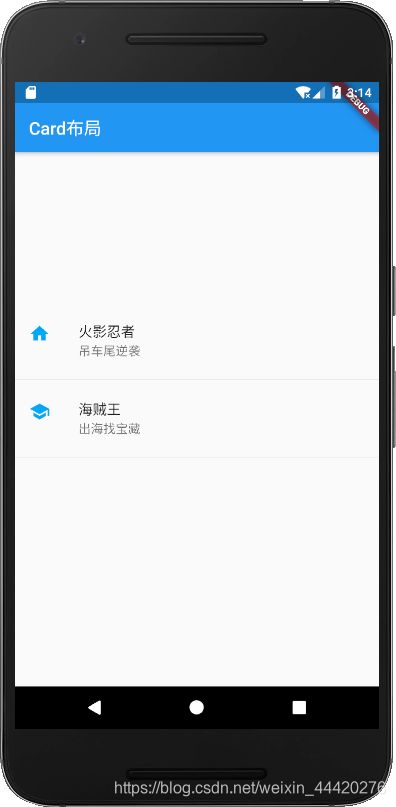