#import module
import numpy
import cartopy
import matplotlib.pyplot as plt
from cartopy import crs
from cartopy.feature import NaturalEarthFeature,COLORS
from matplotlib.cm import get_cmap
from matplotlib.colors import from_levels_and_colors
from matplotlib.animation import FuncAnimation
from IPython.display import HTML
from netCDF4 import Dataset
from xarray import DataArray
from wrf import getvar, interplevel, vertcross, vinterp, ALL_TIMES, CoordPair, xy_to_ll,\
ll_to_xy, to_np, get_cartopy, latlon_coords, cartopy_xlim, cartopy_ylim
import os
import warnings
warnings.filterwarnings('ignore')
#read file data
wrf_dir = "/mnt/"
wrf_file = ["wrfout_d01_2020-03-01_00.nc",
"wrfout_d01_2020-04-01_00.nc",]
for f in wrf_files:
if not os.path.exists(f):
raise ValueError("{} does not exist."
"check for typos or incorrect directory.".format(f))
def single_wrf_file():
global wrf_files
return wrf_files[0]
def multiple_wrf_files():
global wrf_files
return wrf_files
file_path = single_wrf_file()
wrf_f = Dataset(file_path)
terrain = getvar(wrf_f, "ter", timeidx = 0)
cart_proj = get_cartopy(terrain)
lats, lons = latlon_coords(terrain)
#叠加中国地图
china = "/china-boundary/china-boundary.shp"
extent = [70, 140, 10,60] #限定绘图范围
cart_proj = get_cartopy(terrain) #创建投影,对应数据集中terrain的投影
fig = plt.figure(figsize=(10,8))
geo_axes = plt.axes(projection=cart_proj)
states = NaturalEarthFeature(category='cultural',
scale='50m',
facecolor='none',
name='admin_1_states_provinces')
cmap = cartopy.feature.ShapelyFeature(shapereader.Reader(china).geometries(), cart_proj, edgecolor="k", facecolor="none", linewidth=1)
geo_axes.add_feature(cmap, linewidth=0.8)
geo_axes.add_feature(states, linewidth=0.9)
geo_axes.coastlines("50m", linewidth = 0.8)
levels = np.arange(1, 8000, 500)
plt.contour(to_np(lons), to_np(lats), to_np(terrain),
levels=levels, colors="black", transform = crs.PlateCarree())
plt.contourf(to_np(lons), to_np(lats),
to_np(terrain), levels=levels,
transform=crs.PlateCarree(),
cmap=get_cmap("jet"))
#添加经纬度坐标系
plt.rcParams["font.sans-serif"]=["SimHei"]
gl = geo_axes.gridlines(draw_labels = True, linestyle=":", linewidth=1, color="grey")
gl.top_labels = False #关闭上部经纬度标签
gl.right_labels = False
gl.xlabels_bottom = True
gl.xformatter = LONGITUDE_FORMATTER #使横坐标转化为经纬度格式
gl.yformatter = LATITUDE_FORMATTER
gl.xlocator = mticker.FixedLocator(np.arange(70,140,10))
gl.ylocator = mticker.FixedLocator(np.arange(10,60,10))
gl.xlabel_style ={"size":12, "color":"red"} #修改经纬度坐标网格字体大小
gl.ylabel_style ={"size":12, "color":"black"} #修改经纬度坐标网格字体大小
plt.colorbar(ax=geo_axes, shrink=0.58)
#plt.show()
plt.savefig("../wrf-domain.jpg", dpi = 600)
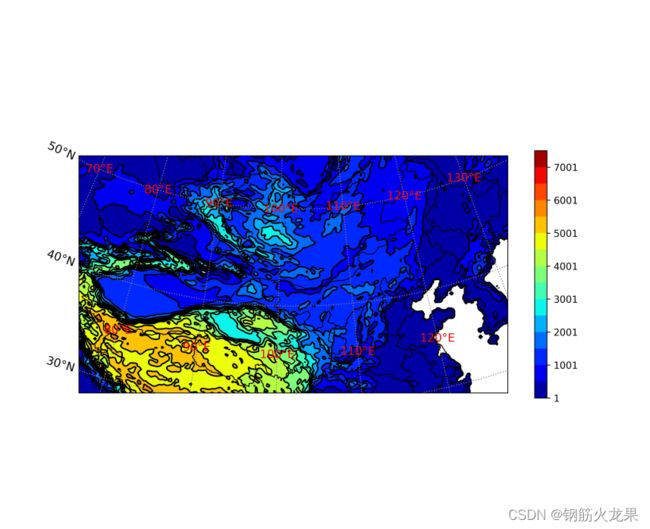