使用访问图像三种办法,指针,迭代器,动态地址计算
指针访问
方法一
#include
#include
#include
#include
using namespace std;
using namespace cv;
#include
#include
using namespace cv;
#include
#include
void colorReduce(Mat& inputImage, Mat& outputImage, int div);
int main()
{
Mat srcImage = imread("1.jpg");
imshow("原始图像", srcImage);
Mat dstImage;
dstImage.create(srcImage.rows, srcImage.cols, srcImage.type());
double time0 = static_cast<double>(getTickCount());
colorReduce(srcImage, dstImage, 32);
time0 = ((double)getTickCount() - time0) / getTickFrequency(); cout << "此方法运行时间为: " << time0 << "秒" << endl;
imshow("效果图", dstImage);
waitKey(0);
}
void colorReduce(Mat& inputImage, Mat& outputImage, int div)
{
outputImage = inputImage.clone();
int rowNumber = outputImage.rows;
int colNumber = outputImage.cols * outputImage.channels();
for (int i = 0; i < rowNumber; i++)
{
uchar* data = outputImage.ptr<uchar>(i);
for (int j = 0; j < colNumber; j++)
{
data[j] = data[j] / div * div + div / 2;
}
}
}
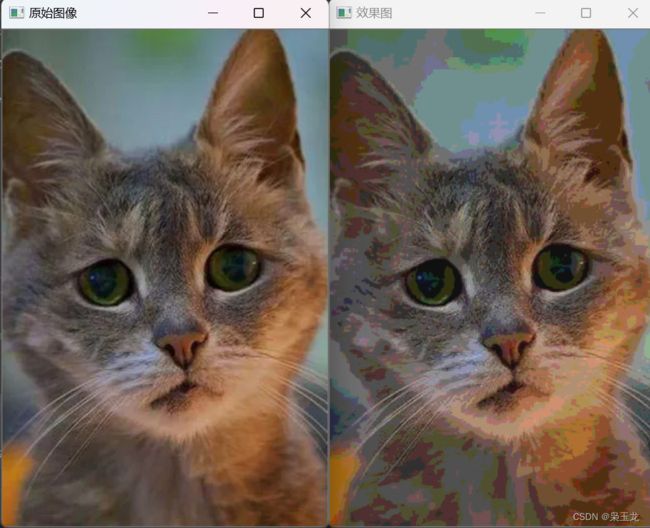
指针访问方法二
#include
int main() {
cv::Mat image = cv::imread("path/to/your/image.jpg");
if (image.empty()) {
std::cerr << "Error: Could not read the image." << std::endl;
return -1;
}
int rows = image.rows;
int cols = image.cols;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
cv::Vec3b& pixel = image.at<cv::Vec3b>(i, j);
pixel[0] = 0;
pixel[1] = pixel[1];
pixel[2] = pixel[2];
}
}
cv::imshow("Modified Image", image);
cv::waitKey(0);
return 0;
}

使用迭代器调用图片
#include
#include
#include
#include
using namespace std;
using namespace cv;
#include
#include
using namespace cv;
#include
#include
void colorReduce(Mat& inputImage, Mat& outputImage, int div);
int main()
{
Mat srcImage = imread("1.jpg");
imshow("原始图像", srcImage);
Mat dstImage;
dstImage.create(srcImage.rows, srcImage.cols, srcImage.type());
double time0 = static_cast<double>(getTickCount());
colorReduce(srcImage, dstImage, 32);
time0 = ((double)getTickCount() - time0) / getTickFrequency(); cout << "此方法运行时间为: " << time0 << "秒" << endl;
imshow("效果图", dstImage);
waitKey(0);
}
void colorReduce(Mat& inputImage, Mat & outputImage, int div)
{
outputImage = inputImage. clone();
Mat_<Vec3b>::iterator it = outputImage. begin<Vec3b>();
Mat_<Vec3b>::iterator itend = outputImage. end<Vec3b>();
for(;it != itend;++it)
{
(*it) [0] = (*it) [0]/div*div + div/2;
(*it) [1] = (*it) [1]/div*div + div/2;
(*it) [2] = (*it) [2]/div*div + div/2;
}
}
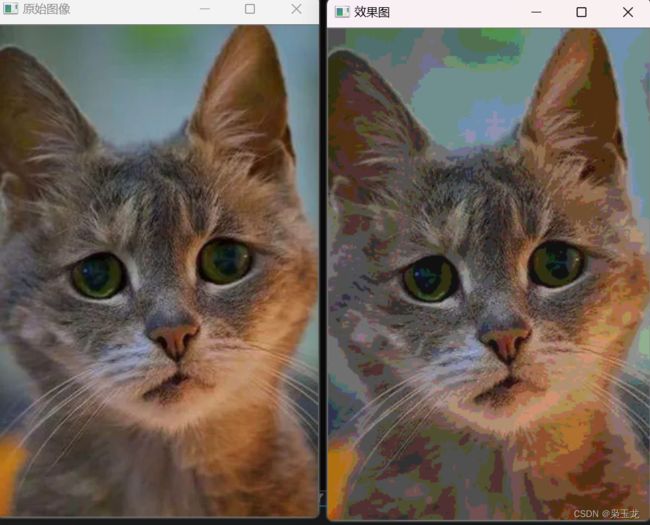
方法二使用迭代器调用图片
#include
int main() {
cv::Mat image = cv::imread("1.jpg");
if (image.empty()) {
std::cerr << "Error: Could not read the image." << std::endl;
return -1;
}
for (cv::MatIterator_<cv::Vec3b> it = image.begin<cv::Vec3b>(); it != image.end<cv::Vec3b>(); ++it) {
cv::Vec3b& pixel = (*it);
pixel[0] = 0;
pixel[1] = pixel[1];
pixel[2] = pixel[2];
}
cv::imshow("Modified Image", image);
cv::waitKey(0);
return 0;
}
使用动态地址调用图片
#include
#include
#include
#include
using namespace std;
using namespace cv;
#include
#include
using namespace cv;
#include
#include
void colorReduce(Mat& inputImage, Mat& outputImage, int div);
int main()
{
Mat srcImage = imread("1.jpg");
imshow("原始图像", srcImage);
Mat dstImage;
dstImage.create(srcImage.rows, srcImage.cols, srcImage.type());
double time0 = static_cast<double>(getTickCount());
colorReduce(srcImage, dstImage, 32);
time0 = ((double)getTickCount() - time0) / getTickFrequency(); cout << "此方法运行时间为: " << time0 << "秒" << endl;
imshow("效果图", dstImage);
waitKey(0);
}
void colorReduce(Mat& inputImage, Mat& outputImage, int div) {
outputImage = inputImage.clone();
int rowNumber = outputImage.rows;
int colNumber = outputImage.cols;
for (int i = 0; i < rowNumber; i++)
{
for (int j = 0; j < colNumber; j++)
{
outputImage.at<Vec3b>(i, j)[0] =
outputImage.at<Vec3b>(i, j)[0] / div * div + div / 2;
outputImage.at<Vec3b>(i, j)[1] = outputImage.at<Vec3b>(i, j)[1] / div * div + div / 2;
outputImage.at<Vec3b>(i, j)[2] =
outputImage.at<Vec3b>(i, j)[2] / div * div + div / 2;
}
}
}
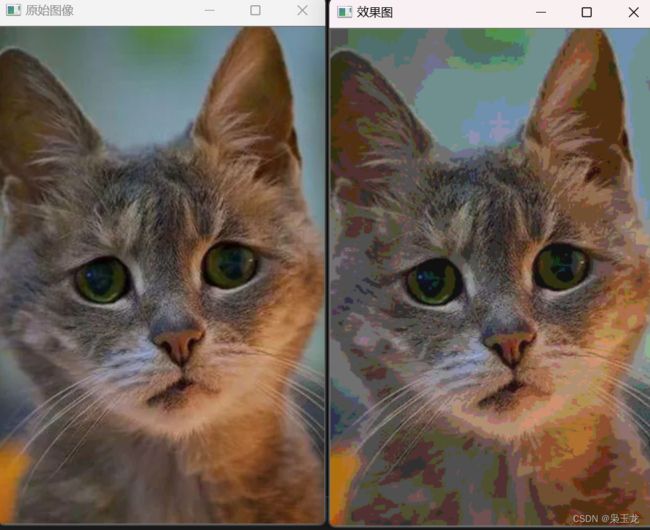
方法二动态地址计算
#include
int main() {
cv::Mat image = cv::imread("1.jpg");
if (image.empty()) {
std::cerr << "Error: Could not read the image." << std::endl;
return -1;
}
int rows = image.rows;
int cols = image.cols;
for (int i = 0; i < rows; ++i) {
uchar* rowPtr = image.ptr<uchar>(i);
for (int j = 0; j < cols; ++j) {
int offset = j * image.channels();
rowPtr[offset] = 0;
rowPtr[offset + 1] = rowPtr[offset + 1];
rowPtr[offset + 2] = rowPtr[offset + 2];
}
}
cv::imshow("Modified Image", image);
cv::waitKey(0);
return 0;
}
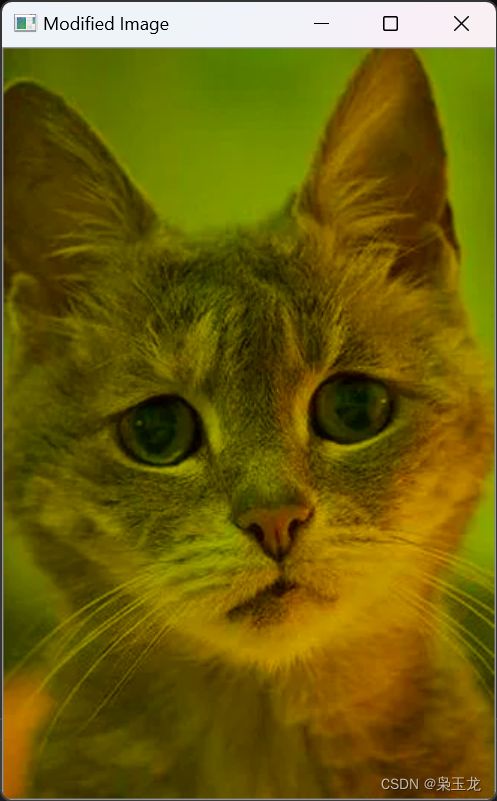