C语言实现二叉树的四种遍历和求深度与叶子结点个数
-
- 使用链式存储实现二叉树建立
-
- 1、**定义存储数据类型和链式二叉树**
- 2、**根据输入结点初始化并建立二叉树**
- 构造访问输出Visit函数
- 二叉树的先序遍历
- 二叉树的中序遍历
- 二叉树的后序遍历
- 求二叉树的深度和叶子结点个数
-
- 1、**求二叉树的深度**
- 2、**求二叉树的叶子结点个数**
- 定义顺序队列并初始化
-
- 1、**定义一个顺序队列**
- 2、**初始化队列**
- 构造队列相关函数
-
- 1、**判断队列是否为空**
- 2、**队列入队操作**
- 3、**队列出队操作**
- 使用队列实现二叉树的层序遍历
- 整个项目的完整代码
- 运行效果图
使用链式存储实现二叉树建立
1、定义存储数据类型和链式二叉树
typedef char ElemType;
typedef struct BiTNode {
ElemType data;
struct BiTNode *lchild, *rchild;
} BiTNode, *BiTree;
2、根据输入结点初始化并建立二叉树
bool CreateBiTree(BiTree &T) {
ElemType ch;
scanf("%c", &ch);
getchar();
if (ch == ' ') {
T = NULL;
} else {
if (!(T = (BiTree) malloc(sizeof(BiTNode)))) {
return false;
}
T->data = ch;
printf("请输入%c的左子树:", ch);
CreateBiTree(T->lchild);
printf("请输入%c的右子树:", ch);
CreateBiTree(T->rchild);
}
return true;
}
构造访问输出Visit函数
void Visit(BiTree T) {
printf("%c", T->data);
}
二叉树的先序遍历
void PreOrder(BiTree T) {
if (T != NULL) {
Visit(T);
PreOrder(T->lchild);
PreOrder(T->rchild);
}
}
二叉树的中序遍历
void InOrder(BiTree T) {
if (T != NULL) {
InOrder(T->lchild);
Visit(T);
InOrder(T->rchild);
}
}
二叉树的后序遍历
void PosOrder(BiTree T) {
if (T) {
PosOrder(T->lchild);
PosOrder(T->rchild);
Visit(T);
}
}
求二叉树的深度和叶子结点个数
1、求二叉树的深度
int TreeDeep(BiTree T) {
int deep = 0;
if (T != NULL) {
int leftDeep = TreeDeep(T->lchild);
int rightDeep = TreeDeep(T->rchild);
deep = leftDeep >= rightDeep ? leftDeep + 1 : rightDeep + 1;
}
return deep;
}
2、求二叉树的叶子结点个数
int LeafCount(BiTree T, int &num) {
if (T) {
if (T->lchild == NULL && T->rchild == NULL) {
num++;
}
LeafCount(T->lchild, num);
LeafCount(T->rchild, num);
}
return num;
}
定义顺序队列并初始化
1、定义一个顺序队列
typedef struct {
BiTree BNode[MaxSize];
int front, rear;
} SqQueue;
2、初始化队列
void InitQueue(SqQueue &Q) {
Q.front = Q.rear = 0;
}
构造队列相关函数
1、判断队列是否为空
bool IsEmpty(SqQueue Q) {
if (Q.front == Q.rear)
return true;
else
2、队列入队操作
bool EnQueue(SqQueue &Q, BiTree T) {
if ((Q.rear + 1) % MaxSize == Q.front)
return false;
Q.BNode[Q.rear] = T;
Q.rear = (Q.rear + 1) % MaxSize;
return true;
}
3、队列出队操作
bool DeQueue(SqQueue &Q, BiTree &T) {
if (Q.front == Q.rear)
return false;
T = Q.BNode[Q.front];
Q.front = (Q.front + 1) % MaxSize;
return true;
}
使用队列实现二叉树的层序遍历
void LevelOrder(BiTree T, SqQueue &Q) {
if (T != NULL) {
EnQueue(Q, T);
}
while (IsEmpty(Q) == false) {
BiTree T1;
DeQueue(Q, T1);
Visit(T1);
if (T1->lchild != NULL)
EnQueue(Q, T1->lchild);
if (T1->rchild != NULL)
EnQueue(Q, T1->rchild);
}
}
整个项目的完整代码
#include
#include
#define MaxSize 255
typedef char ElemType;
typedef struct BiTNode {
ElemType data;
struct BiTNode *lchild, *rchild;
} BiTNode, *BiTree;
typedef struct {
BiTree BNode[MaxSize];
int front, rear;
} SqQueue;
bool CreateBiTree(BiTree &T) {
ElemType ch;
scanf("%c", &ch);
getchar();
if (ch == ' ') {
T = NULL;
} else {
if (!(T = (BiTree) malloc(sizeof(BiTNode)))) {
return false;
}
T->data = ch;
printf("请输入%c的左子树:", ch);
CreateBiTree(T->lchild);
printf("请输入%c的右子树:", ch);
CreateBiTree(T->rchild);
}
return true;
}
void InitQueue(SqQueue &Q) {
Q.front = Q.rear = 0;
}
bool IsEmpty(SqQueue Q) {
if (Q.front == Q.rear)
return true;
else
return false;
}
bool EnQueue(SqQueue &Q, BiTree T) {
if ((Q.rear + 1) % MaxSize == Q.front)
return false;
Q.BNode[Q.rear] = T;
Q.rear = (Q.rear + 1) % MaxSize;
return true;
}
bool DeQueue(SqQueue &Q, BiTree &T) {
if (Q.front == Q.rear)
return false;
T = Q.BNode[Q.front];
Q.front = (Q.front + 1) % MaxSize;
return true;
}
void Visit(BiTree T) {
printf("%c", T->data);
}
void PreOrder(BiTree T) {
if (T != NULL) {
Visit(T);
PreOrder(T->lchild);
PreOrder(T->rchild);
}
}
void InOrder(BiTree T) {
if (T != NULL) {
InOrder(T->lchild);
Visit(T);
InOrder(T->rchild);
}
}
void PosOrder(BiTree T) {
if (T) {
PosOrder(T->lchild);
PosOrder(T->rchild);
Visit(T);
}
}
void LevelOrder(BiTree T, SqQueue &Q) {
if (T != NULL) {
EnQueue(Q, T);
}
while (IsEmpty(Q) == false) {
BiTree T1;
DeQueue(Q, T1);
Visit(T1);
if (T1->lchild != NULL)
EnQueue(Q, T1->lchild);
if (T1->rchild != NULL)
EnQueue(Q, T1->rchild);
}
}
int TreeDeep(BiTree T) {
int deep = 0;
if (T != NULL) {
int leftDeep = TreeDeep(T->lchild);
int rightDeep = TreeDeep(T->rchild);
deep = leftDeep >= rightDeep ? leftDeep + 1 : rightDeep + 1;
}
return deep;
}
int LeafCount(BiTree T, int &num) {
if (T) {
if (T->lchild == NULL && T->rchild == NULL) {
num++;
}
LeafCount(T->lchild, num);
LeafCount(T->rchild, num);
}
return num;
}
int main() {
BiTree T;
SqQueue Q;
InitQueue(Q);
int num = 0;
printf("请先输入树根结点(空格代表空结点):");
CreateBiTree(T);
printf("该二叉树的先序遍历结果为:");
PreOrder(T);
printf("\n");
printf("该二叉树的中序遍历结果为:");
InOrder(T);
printf("\n");
printf("该二叉树的后序遍历结果为:");
PosOrder(T);
printf("\n");
printf("该二叉树的层次遍历结果为:");
LevelOrder(T, Q);
printf("\n");
printf("该二叉树的深度为:");
printf("%d\n", TreeDeep(T));
printf("该二叉树的叶子结点个数为:");
printf("%d\n", LeafCount(T, num));
return 0;
}
运行效果图
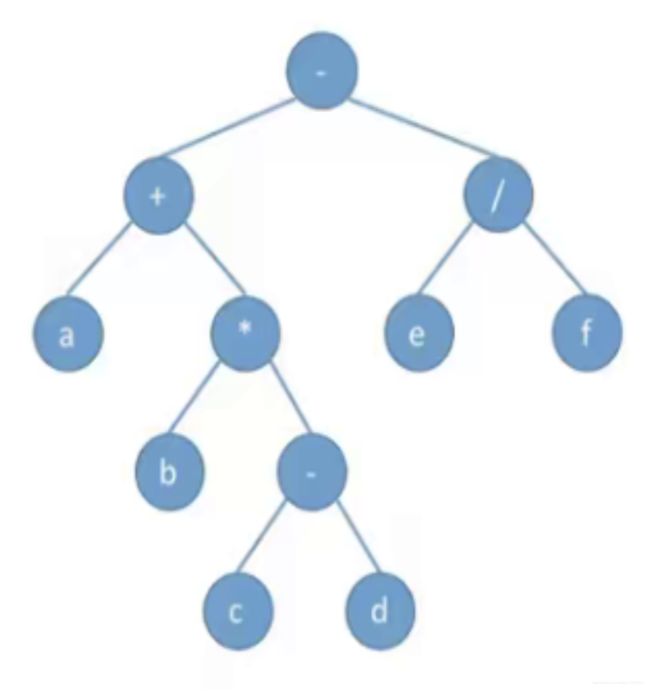
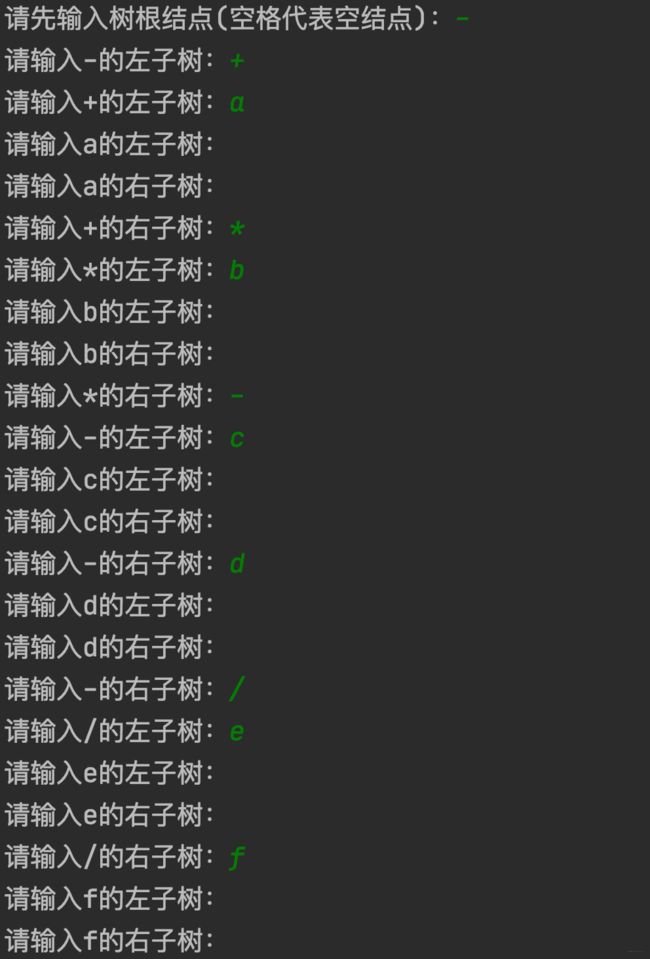
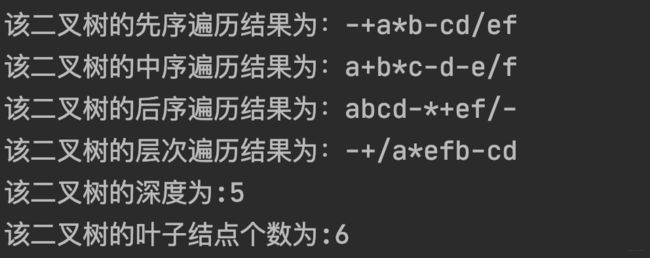