介绍
- 本文功能由canvas实现红包雨功能(
index.tsx
)
- 本文为react的ts版。如有其他版本需求可评论区
- 观赏地址,需过墙
import React, { Component } from 'react';
import moneyx from '@/assets/images/RedEnvelopeRain/ball1.png';
import money1 from '@/assets/images/RedEnvelopeRain/money1.webp';
import money2 from '@/assets/images/pointCon/机器人.png';
import money3 from '@/assets/images/pointCon/无人机.png';
import {
loadImage,
positionX,
randomRange,
} from './utils';
type minMax = [number, number];
const config = {
speedLimit: [1, 4] as minMax,
density: [5, 15] as minMax,
imgInfo: {
w: 50,
h: 50,
randomLimit: [0.5, 1] as minMax,
},
numberMaxLimit: 200,
};
const { speedLimit, imgInfo, numberMaxLimit, density } = config;
export default class CanvasCom extends Component {
ctx: any = null;
wid = 0;
hei = 0;
packedArr: ReturnType<typeof this.createPack>[] = [];
density = {
min: density[0],
max: density[1],
};
clearTime: any = null;
img: any = [];
loadingImageArr = [money1, moneyx, money2, money3]
componentDidMount() {
this.loadingImg();
}
loadingImg() {
Promise.all(this.loadingImageArr.map(v => loadImage(v))).then((res) => {
this.img = res;
this.wid = window.innerWidth;
this.hei = window.innerHeight;
this.initCanvas();
this.start();
});
}
getPixelRatio = (context: any) => {
const backingStore =
context.backingStorePixelRatio ||
context.webkitBackingStorePixelRatio ||
context.mozBackingStorePixelRatio ||
context.msBackingStorePixelRatio ||
context.oBackingStorePixelRatio ||
context.backingStorePixelRatio ||
1;
return (window.devicePixelRatio || 1) / backingStore;
};
initCanvas() {
const canvas: HTMLCanvasElement | any = document.getElementById('canvas');
canvas.width = this.wid;
canvas.height = this.hei;
if (canvas.getContext) {
this.ctx = canvas.getContext('2d');
}
}
createPack() {
const imgRandom = randomRange(...imgInfo.randomLimit);
return {
x: positionX(),
y: 0,
img: this.img[Math.floor(randomRange(0, this.loadingImageArr.length ))],
rotate: randomRange(-45, 45),
direction: Math.random(),
speed: randomRange(...speedLimit),
round: 0,
roundSpeed: randomRange(1, 2),
imgInfo: {
w: imgInfo.w * imgRandom,
h: imgInfo.h * imgRandom,
},
};
}
pushPackArr = () => {
const { max, min } = this.density;
const random = Math.floor(Math.random() * (max - min) + min);
this.packedArr.push(
...new Array(random).fill('').map(() => this.createPack())
);
this.clearTime = setTimeout(() => {
if (this.packedArr.length > numberMaxLimit)
return clearTimeout(this.clearTime);
this.pushPackArr();
}, 300);
};
drawPacked = () => {
this.ctx.clearRect(0, 0, window.innerWidth, window.innerHeight);
this.packedArr.forEach((item, index) => {
const r = ((item.rotate + item.round) * Math.PI) / 180;
const temp = item.y - item.x * Math.tan(r);
const top = temp * Math.cos(r);
const left = item.x / Math.cos(r) + temp * Math.sin(r);
this.ctx.save();
this.ctx.rotate(r);
this.ctx.drawImage(
item.img,
left - item.imgInfo.w / 2,
top - item.imgInfo.h / 2,
item.imgInfo.w,
item.imgInfo.h
);
this.ctx.restore();
if (item.direction < 0.5) {
item.round -= item.roundSpeed;
} else {
item.round += item.roundSpeed;
}
if (item.y + item?.speed <= window.innerHeight) {
item.y = item.y + item?.speed || 0;
} else {
item.y = 0 + item?.speed || 0;
item.x = positionX();
Object.assign(item, this.createPack());
}
});
window.requestAnimationFrame(this.drawPacked);
};
start = () => {
this.pushPackArr();
this.drawPacked();
};
render() {
return (
<div style={{ position: 'absolute', left: 0, top: 0, background: '#000' }}>
<canvas id="canvas"></canvas>
</div>
);
}
}
utils/index.ts
export const loadImage = (url: string) => {
if (!window?.Image) return null;
return new Promise((resolve, reject) => {
const img = new Image();
img.src = url;
img.onload = () => {
resolve(img);
};
img.onerror = () => {
reject(new Error('load image error!'));
};
});
};
export const positionX = () => Math.random() * window.innerWidth;
export const randomRange = (start: number, end: number) => {
const random = (end - start) * Math.random() + start;
const number = 1;
const arr = new Array(number).fill('').map((v, i) => random / (i + 1));
return arr[Math.floor(Math.random() * number)];
};
效果图
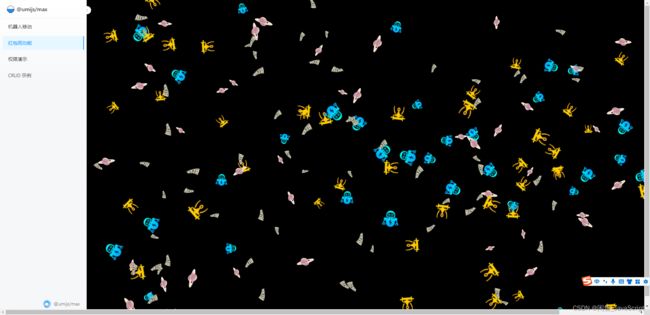
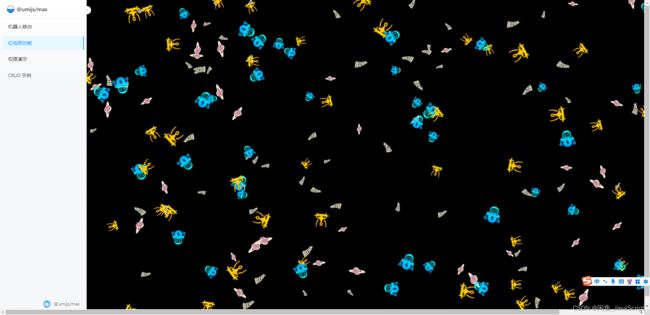