Vuex模块化(modules)与namespaced(命名空间)的搭配
Vuex模块化(modules)格式
- 原理:可以对Vuex的actions,mutations,state,getters四个属性综合成一个部分,做成一个模块使用
- 优点:可以将一些特殊的功能独立,进行调用
const a = {
actions : {
方法名(context, value){
......对value的操作
context.commit('mutations方法名', value)
}
},
mutations : {
方法名(state, value){
......对state更新,并赋值给value
}
},
state : {
对象名 : 100
},
getters : {
方法名(state){
return ......
}
}
}
export default new Vuex.Store({
modules : {
aModule : a
a
}
})
namespaced(命名空间)用法
- 功能:用来控制dispatch和commit的全访问能力
注意点1:模块区分
- 在模块化的基础上,对于dispatch或者是commit在调用时需要做好命名空间(namespaced)
- 原因:在没有做模块化处理的时候,通过dispatch或者是commit调用时,是将一整个文件进行搜索,找寻与之对应的方法名。(做个小实验,如下)
<template>
<div>
<button @click="A1">A1 BUTTON</button>
</div>
</template>
<script>
export default {
name : 'A',
methods : {
A1(){
this.$store.dispatch('A1')
}
}
}
</script>
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const a = {
actions : {
A1(){
console.log('A1 actions');
}
}
}
const b = {
actions : {
A1(){
console.log('B1 actions');
}
}
}
export default new Vuex.Store({
modules : {
aModule : a,
bModule : b,
}
})
- 以上store.js文件中,分别定义了a和b模块,在两个模块的actions中都有A1方法,执行效果如下:
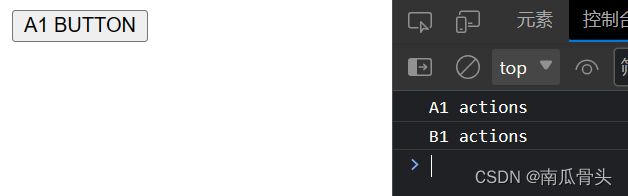
- 点击A1按钮,都触发了a和b模块中的A1方法。当我们只需要执行一个模块的时候,就可以使用命名空间(namespaced),在模块中的第一行加上
namespaced : true
,可以防止一次性全部访问到
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const a = {
namespaced : true,
actions : {
A1(){
console.log('A1 actions');
}
}
}
const b = {
namespaced : true,
actions : {
A1(){
console.log('B1 actions');
}
}
}
export default new Vuex.Store({
modules : {
aModule : a,
bModule : b,
}
})
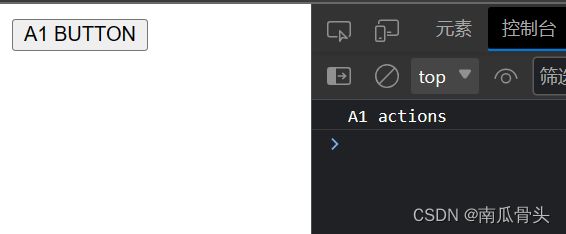
注意点2:使用namespace模块区分后,在xxx.vue调用模块方法上要做出的改变
- state:
- 没有namespace:{{$store.state.对象名}}
- 用了namespace:{{$store.state.模块名.对象名}}
- actions:
- 没有namespace:this.$store.dispatch(‘actions方法名’, 值)
- 用了namespace:this.$store.dispatch(‘模块名/actions方法名’, 值)
- mutations:
- 没有namespace:this.$store.commit(‘mutations方法名’, 值)
- 用了namespace:this.$store.commit(‘模块名/mutations方法名’, 值)
- getters:
- 没有namespace:{{$store.getters.getters方法名}}
- 用了namespace:{{$store.getters[‘模块名/getters方法名’]}}
<template>
<div>
<h3>state:{{$store.state.aModule.对象名}}</h3>
<h3>getters:{{$store.getters['aModule/getters方法名']}}</h3>
</div>
</template>
<script>
export default {
name : 'xxx',
methods : {
方法名(){
this.$store.dispatch('aModule/actions方法名', 值)
},
方法名(){
this.$store.commit('aModule/mutations方法名', 值)
}
}
}
</script>
const a = {
namespaced : true,
actions : {
方法名(context, value){
......对value的操作
context.commit('mutations方法名', value)
}
},
mutations : {
方法名(state, value){
......对state更新,并赋值给value
}
},
state : {
对象名 : 100
},
getters : {
方法名(state){
return ......
}
}
}
const b = {跟上面一样}
export default new Vuex.Store({
modules : {
aModule : a
a
}
})
导入导出式模板(简洁)
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
import aModule from './a'
export default new Vuex.Store({
modules : {
aModule
}
})
export default {
namespaced : true,
actions : {
......
},
mutations : {
......
},
state : {
......
},
getters : {
......
}
}