- 首先效果图:
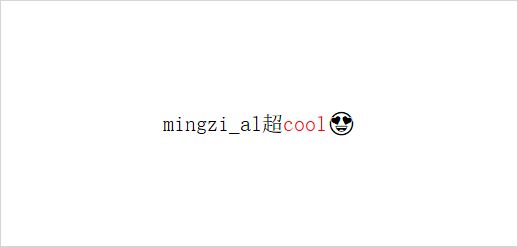
- 接着上通用代码:
public IWorkbook Excel_OpenRead(string excelpath)
{
IWorkbook workbook = null;
bool isCompatible = excelpath.EndsWith(".xls", StringComparison.OrdinalIgnoreCase);
FileStream fsRead = File.OpenRead(excelpath);
if (isCompatible) { workbook = new HSSFWorkbook(fsRead); } else { workbook = new XSSFWorkbook(fsRead); }
fsRead.Close();
return workbook;
}
public void Excel_Write(string excelpath, IWorkbook workbook)
{
Directory.CreateDirectory(Path.GetDirectoryName(excelpath));
FileStream fsWrite = File.Open(excelpath, FileMode.Create, FileAccess.Write);
workbook.Write(fsWrite);
fsWrite.Close();
}
public ICellStyle Excel_Style(IWorkbook workbook, string alignment = "居中")
{
ICellStyle style = workbook.CreateCellStyle();
style.BorderTop = BorderStyle.Thin;
style.BorderBottom = BorderStyle.Thin;
style.BorderLeft = BorderStyle.Thin;
style.BorderRight = BorderStyle.Thin;
style.VerticalAlignment = VerticalAlignment.Center;
style.WrapText = true;
switch (alignment)
{
case "居中":
style.Alignment = HorizontalAlignment.Center;
break;
case "左":
style.Alignment = HorizontalAlignment.Left;
break;
case "右":
style.Alignment = HorizontalAlignment.Right;
break;
}
return style;
}
public IFont Excel_Font(IWorkbook workbook, string name = "宋体", int height = 11, string clor = "黑", bool bold = false)
{
IFont Font = workbook.CreateFont();
Font.FontName = name;
Font.FontHeightInPoints = height;
Font.IsBold = bold;
switch (clor)
{
case "黑":
Font.Color = IndexedColors.Black.Index;
break;
case "红":
Font.Color = IndexedColors.Red.Index;
break;
}
return Font;
}
- NPOI:文件,读/写;操作,字体/单元格 直接上个栗子:
using NPOI.HSSF.UserModel;
using NPOI.SS.UserModel;
using NPOI.SS.Util;
using NPOI.XSSF.UserModel;
using BorderStyle = NPOI.SS.UserModel.BorderStyle;
using HorizontalAlignment = NPOI.SS.UserModel.HorizontalAlignment;
private void button1_Click(object sender, EventArgs e)
{
string filepath = @"D:\test.xls";
IWorkbook workbook = Excel_OpenRead(filepath);
ISheet sheet = workbook.GetSheetAt(0);
int iRowCount = sheet.LastRowNum;
ICellStyle style = Excel_Style(workbook);
IFont Font = Excel_Font(workbook, "宋体", 16);
for (int iRow = 0; iRow <= iRowCount; iRow++)
{
if (sheet.GetRow(iRow) is null) { sheet.CreateRow(iRow).CreateCell(15).SetCellValue("未获取到此行有效数据。"); continue; }
string str = Convert.ToString(sheet.GetRow(iRow).GetCell(0));
sheet.GetRow(iRow).CreateCell(1).SetCellValue("单元格赋值");
sheet.GetRow(iRow).Height = 30 * 20;
style.SetFont(Font);
sheet.GetRow(iRow).GetCell(0).CellStyle = style;
}
Excel_Write(filepath, workbook);
}
ICellStyle style1 = Excel_Style(workbook, "左");
string[] text = new string[] {
"文本1",
"文本2",
"文本3",
"文本4",
"文本5",
"文本6"
};
int r = sheet.LastRowNum;
int[,] re = new int[,] { { 0, 3 }, { 4, 8 }, { 9, 16 } };
CellRangeAddress region;
for (int rr = 0; rr < 2; rr++)
{
for (int cc = 0; cc < 3; cc++)
{
if (sheet.GetRow(r + rr) is null) sheet.CreateRow(r + rr);
sheet.GetRow(r + rr).CreateCell(re[cc, 0]).SetCellValue(text[rr * 3 + cc]);
sheet.GetRow(r + rr).GetCell(re[cc, 0]).CellStyle = style1;
region = new CellRangeAddress(r + rr, r + rr, re[cc, 0], re[cc, 1]);
sheet.AddMergedRegion(region);
RegionUtil.SetBorderLeft(1, region, sheet);
RegionUtil.SetBorderRight(1, region, sheet);
RegionUtil.SetBorderTop(1, region, sheet);
RegionUtil.SetBorderBottom(1, region, sheet);
}
sheet.GetRow(r + rr).Height = 50 * 20;
}
string cool = "mingzi_al超cool”
sheet.GetRow(1).GetCell(0).SetCellValue(cool);
ICell cell = sheet.GetRow(1).GetCell(0);
IFont Font = Excel_Font(workbook, "宋体", 16, "红");
IRichTextString richString = null;
if (sheet.Header.ToString().Contains("XSSF"))
{
richString = new XSSFRichTextString(BT);
}
else
{
richString = new HSSFRichTextString(BT);
}
int cool_Strat = cool.ToString().IndexOf("cool");
int cool_End = cool_Strat + cool .Length;
richString.ApplyFont(cool_Strat , cool_End , Font);
cell.SetCellValue(richString);