【IT168 评论】 JSON是专门为浏览器中的网页上运行的JavaScript代码而设计的一种数据格式。在网站应用中使 用JSON的场景越来越多,本文介绍ASP.NET中JSON的序列化和反序列化,主要对JSON的简单介绍,ASP.NET如何序列化和反序列化的处 理,在序列化和反序列化对日期时间、集合、字典的处理。
一、JSON简介
JSON(JavaScript Object Notation,JavaScript对象表示法)是一种轻量级的数据交换格式。
JSON是“名值对”的集合。结构由大括号'{}',中括号'[]',逗号',',冒号':',双引号'“”'组成,包含的数据类型有Object,Number,Boolean,String,Array, NULL等。
JSON具有以下的形式:
对象(Object)是一个无序的“名值对”集合,一个对象以”{”开始,”}”结束。每个“名”后跟着一个”:”,多个“名值对”由逗号分隔。如:
var user
=
{
"
name
"
:
"
张三
"
,
"
gender
"
:
"
男
"
,
"
birthday
"
:
"
1980-8-8
"
}
数组(Array)是值的有序集合,一个数组以“[”开始,以“]”结束,值之间使用“,”分隔。如:
var userlist
=
[{
"
user
"
:{
"
name
"
:
"
张三
"
,
"
gender
"
:
"
男
"
,
"
birthday
"
:
"
1980-8-8
"
}},{
"
user
"
:{
"
name
"
:
"
李四
"
,
"
gender
"
:
"
男
"
,
"
birthday
"
:
"
1985-5-8
"
}}];
字符串(String)是由双引号包围的任意数量的Unicode字符的集合,使用反斜线转义。
二、对JSON数据进行序列化和反序列化
可以使用DataContractJsonSerializer类将类型实例序列化为JSON字符串,并将JSON字符串反序列化为类型实例。 DataContractJsonSerializer在System.Runtime.Serialization.Json命名空间下,.NET Framework 3.5包含在System.ServiceModel.Web.dll中,需要添加对其的引用;.NET Framework 4在System.Runtime.Serialization中。
利用DataContractJsonSerializer序列化和反序列化的代码:
1
: using System;
2
: using System.Collections.Generic;
3
: using System.Linq;
4
: using System.Web;
5
: using System.Runtime.Serialization.Json;
6
: using System.IO;
7
: using System.Text;
8
:
9
:
///
10
:
///
JSON序列化和反序列化辅助类
11
:
///
12
:
public
class JsonHelper
13
: {
14
:
///
15
:
///
JSON序列化
16
:
///
17
:
public
static
string
JsonSerializer(T t)
18
: {
19
: DataContractJsonSerializer ser
=
new
DataContractJsonSerializer(typeof(T));
20
: MemoryStream ms
=
new
MemoryStream();
21
: ser.WriteObject(ms, t);
22
:
string
jsonString
=
Encoding.UTF8.GetString(ms.ToArray());
23
: ms.Close();
24
: return jsonString;
25
: }
26
:
27
:
///
28
:
///
JSON反序列化
29
:
///
30
:
public
static T JsonDeserialize(
string
jsonString)
31
: {
32
: DataContractJsonSerializer ser
=
new
DataContractJsonSerializer(typeof(T));
33
: MemoryStream ms
=
new
MemoryStream(Encoding.UTF8.GetBytes(jsonString));
34
: T obj
=
(T)ser.ReadObject(ms);
35
: return obj;
36
: }
37
: }
序列化Demo:
简单对象Person:
1
:
public
class Person
2
: {
3
:
public
string
Name {
get
;
set
; }
4
:
public
int
Age {
get
;
set
; }
5
: }
序列化为JSON字符串:
1
: protected void Page_Load(
object
sender, EventArgs e)
2
: {
3
: Person p
=
new
Person();
4
: p.Name
=
"
张三
"
;
5
: p.Age
=
28
;
6
:
7
:
string
jsonString
=
JsonHelper.JsonSerializer(p);
8
: Response.Write(jsonString);
9
: }
输出结果:
{
"
Age
"
:
28
,
"
Name
"
:
"
张三
"
}
反序列化Demo:
1
: protected void Page_Load(
object
sender, EventArgs e)
2
: {
3
:
string
jsonString
=
"
{\
"
Age
\
"
:28,\
"
Name
\
"
:\
"
张三
\
"
}
"
;
4
: Person p
=
JsonHelper.JsonDeserialize(jsonString);
5
: }
运行结果:
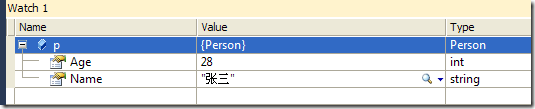
ASP.NET中的JSON序列化和反序列化还可以使用JavaScriptSerializer,在 System.Web.Script.Serializatioin命名空间下,需引用System.Web.Extensions.dll.也可以使用 JSON.NET.
三、JSON序列化和反序列化日期时间的处理
JSON格式不直接支持日期和时间。 DateTime值值显示为“/Date(700000+0500)/”形式的JSON字符串,其中第一个数字(在提供的示例中为 700000)是 GMT 时区中自 1970 年 1 月 1 日午夜以来按正常时间(非夏令时)经过的毫秒数。该数字可以是负数,以表示之前的时间。示例中包括“+0500”的部分可选,它指示该时间属于Local 类型,即它在反序列化时应转换为本地时区。如果没有该部分,则会将时间反序列化为Utc。
修改Person类,添加LastLoginTime:
1
:
public
class Person
2
: {
3
:
public
string
Name {
get
;
set
; }
4
:
public
int
Age {
get
;
set
; }
5
:
public
DateTime LastLoginTime {
get
;
set
; }
6
: }
1
: Person p
=
new
Person();
2
: p.Name
=
"
张三
"
;
3
: p.Age
=
28
;
4
: p.LastLoginTime
=
DateTime.Now;
5
:
6
:
string
jsonString
=
JsonHelper.JsonSerializer(p);
序列化结果:
{
"
Age
"
:
28
,
"
LastLoginTime
"
:
"
\/Date(1294499956278+0800)\/
"
,
"
Name
"
:
"
张三
"
}
1 、在后台使用正则表达式对其替换处理。修改JsonHelper:
1
: using System;
2
: using System.Collections.Generic;
3
: using System.Linq;
4
: using System.Web;
5
: using System.Runtime.Serialization.Json;
6
: using System.IO;
7
: using System.Text;
8
: using System.Text.RegularExpressions;
9
:
10
:
///
11
:
///
JSON序列化和反序列化辅助类
12
:
///
13
:
public
class JsonHelper
14
: {
15
:
///
16
:
///
JSON序列化
17
:
///
18
:
public
static
string
JsonSerializer(T t)
19
: {
20
: DataContractJsonSerializer ser
=
new
DataContractJsonSerializer(typeof(T));
21
: MemoryStream ms
=
new
MemoryStream();
22
: ser.WriteObject(ms, t);
23
:
string
jsonString
=
Encoding.UTF8.GetString(ms.ToArray());
24
: ms.Close();
25
:
//
替换Json的Date字符串
26
:
string
p
=
@
"
\\/Date\((\d+)\+\d+\)\\/
"
;
27
: MatchEvaluator matchEvaluator
=
new
MatchEvaluator(ConvertJsonDateToDateString);
28
: Regex reg
=
new
Regex(p);
29
: jsonString
=
reg.Replace(jsonString, matchEvaluator);
30
: return jsonString;
31
: }
32
:
33
:
///
34
:
///
JSON反序列化
35
:
///
36
:
public
static T JsonDeserialize(
string
jsonString)
37
: {
38
:
//
将
"
yyyy-MM-dd HH:mm:ss
"
格式的字符串转为
"
\/Date(1294499956278+0800)\/
"
格式
39
:
string
p
=
@
"
\d{4}-\d{2}-\d{2}\s\d{2}:\d{2}:\d{2}
"
;
40
: MatchEvaluator matchEvaluator
=
new
MatchEvaluator(ConvertDateStringToJsonDate);
41
: Regex reg
=
new
Regex(p);
42
: jsonString
=
reg.Replace(jsonString, matchEvaluator);
43
: DataContractJsonSerializer ser
=
new
DataContractJsonSerializer(typeof(T));
44
: MemoryStream ms
=
new
MemoryStream(Encoding.UTF8.GetBytes(jsonString));
45
: T obj
=
(T)ser.ReadObject(ms);
46
: return obj;
47
: }
48
:
49
:
///
50
:
///
将Json序列化的时间由
/
Date
(
1294499956278
+
0800
)转为字符串
51
:
///
52
:
private
static
string
ConvertJsonDateToDateString(Match m)
53
: {
54
:
string
result
=
string
.Empty;
55
: DateTime dt
=
new
DateTime(
1970
,
1
,
1
);
56
: dt
=
dt.AddMilliseconds(
long
.Parse(m.Groups[
1
].Value));
57
: dt
=
dt.ToLocalTime();
58
: result
=
dt.ToString(
"
yyyy-MM-dd HH:mm:ss
"
);
59
: return result;
60
: }
61
:
62
:
///
63
:
///
将时间字符串转为Json时间
64
:
///
65
:
private
static
string
ConvertDateStringToJsonDate(Match m)
66
: {
67
:
string
result
=
string
.Empty;
68
: DateTime dt
=
DateTime.Parse(m.Groups[
0
].Value);
69
: dt
=
dt.ToUniversalTime();
70
: TimeSpan ts
=
dt
-
DateTime.Parse(
"
1970-01-01
"
);
71
: result
=
string
.Format(
"
\\/Date({0}+0800)\\/
"
,ts.TotalMilliseconds);
72
: return result;
73
: }
74
: }
序列化Demo:
1
: Person p
=
new
Person();
2
: p.Name
=
"
张三
"
;
3
: p.Age
=
28
;
4
: p.LastLoginTime
=
DateTime.Now;
5
:
6
:
string
jsonString
=
JsonHelper.JsonSerializer(p);
运行结果:
{
"
Age
"
:
28
,
"
LastLoginTime
"
:
"
2011-01-09 01:00:56
"
,
"
Name
"
:
"
张三
"
}
反序列化Demo:
string
json
=
"
{\
"
Age
\
"
:28,\
"
LastLoginTime
\
"
:\
"
2011
-
01
-
09
00
:
30
:
00
\
"
,\
"
Name
\
"
:\
"
张三
\
"
}
"
;
p
=
JsonHelper.JsonDeserialize(json);
运行结果:

在后台替换字符串适用范围比较窄,如果考虑到全球化的有多种语言还会更麻烦。
2、利用JavaScript处理
1
:
function
ChangeDateFormat(jsondate) {
2
: jsondate
=
jsondate.replace(
"
/Date(
"
,
""
).replace(
"
)/
"
,
""
);
3
:
if
(jsondate.indexOf(
"
+
"
)
>
0
) {
4
: jsondate
=
jsondate.substring(
0
, jsondate.indexOf(
"
+
"
));
5
: }
6
:
else
if
(jsondate.indexOf(
"
-
"
)
>
0
) {
7
: jsondate
=
jsondate.substring(
0
, jsondate.indexOf(
"
-
"
));
8
: }
9
:
10
: var
date
=
new
Date
(parseInt(jsondate,
10
));
11
: var
month
=
date
.getMonth()
+
1
<
10
?
"
0
"
+
(
date
.getMonth()
+
1
) :
date
.getMonth()
+
1
;
12
: var currentDate
=
date
.getDate()
<
10
?
"
0
"
+
date
.getDate() :
date
.getDate();
13
: return
date
.getFullYear()
+
"
-
"
+
month
+
"
-
"
+
currentDate;
14
: }
简单Demo :
ChangeDateFormat(
"
\/Date(1294499956278+0800)\/
"
);
结果:
2011-1-8
四、JSON序列化和反序列化集合、字典、数组的处理
在JSON数据中,所有的集合、字典和数组都表示为数组。
List序列化:
1
: List list
=
new
List()
2
: {
3
:
new
Person(){ Name
=
"
张三
"
, Age
=
28
},
4
:
new
Person(){ Name
=
"
李四
"
, Age
=
25
}
5
: };
6
:
7
:
string
jsonString
=
JsonHelper.JsonSerializer
>
(list);
序列化结果:
"
[{\
"
Age
\
"
:28,\
"
Name
\
"
:\
"
张三
\
"
},{\
"
Age
\
"
:25,\
"
Name
\
"
:\
"
李四
\
"
}]
"
字典不能直接用于JSON,Dictionary字典转化为JSON并不是跟原来的字典格式一致,而是形式以Dictionary的Key作为名称”Key“的值,以Dictionary的Value作为名称为”Value“的值 。如:
1
: Dictionary dic
=
new
Dictionary();
2
: dic.Add(
"
Name
"
,
"
张三
"
);
3
: dic.Add(
"
Age
"
,
"
28
"
);
4
:
5
:
string
jsonString
=
JsonHelper.JsonSerializer
<
Dictionary
>
(dic);
序列化结果:
1
:
"
[{\
"
Key
\
"
:\
"
Name
\
"
,\
"
Value
\
"
:\
"
张三
\
"
},{\
"
Key
\
"
:\
"
Age
\
"
,\
"
Value
\
"
:\
"
28
\
"
}]
"
http://tech.it168.com/a2011/0412/1177/000001177095.shtml