最简单的类
function Person() {
this.name = "张三";
this.age = 20;
}
var p = new Person();
console.log(p.name);
构造函数和原型链里面增加方法
function Person(){
this.name='张三';
this.age=20;
this.run=function(){
console.log(this.name+'在运动');
}
}
Person.prototype.sex="男";
Person.prototype.work=function(){
console.log(this.name+'在工作');
}
var p=new Person();
console.log(p.name);
p.run();
p.work();
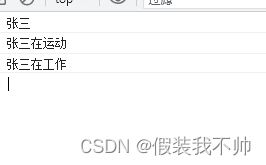
类里面的静态方法
function Person() {
this.name = '张三';
this.age = 20;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.getInfo = function () {
console.log('我是静态方法');
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
var p = new Person();
p.work();
Person.getInfo();
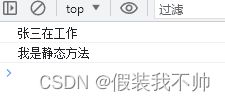
继承
对象冒充实现继承
function Person() {
this.name = '张三';
this.age = 20;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
function Web() {
Person.call(this);
}
var w = new Web();
w.run();
w.work();

原型链实现继承
function Person() {
this.name = '张三';
this.age = 20;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
function Web() {}
Web.prototype = new Person();
var w = new Web();
w.run();
w.work();
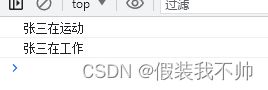
原型链实现继承的问题
function Person(name, age) {
this.name = name;
this.age = age;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
var p = new Person('李四', 20);
p.run();
p.work();

使用构造函数之后就会出现问题
function Person(name, age) {
this.name = name;
this.age = age;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
function Web(name, age) {
}
Web.prototype = new Person();
var w = new Web('赵四', 20);
w.run();
w.work();
var w1=new Web('王五',22);
w1.run();
w1.work();
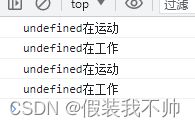
原型链+对象冒充的组合继承模式
function Person(name, age) {
this.name = name;
this.age = age;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
function Web(name, age) {
Person.call(this, name, age);
}
Web.prototype = new Person();
var w = new Web('赵四', 20);
w.run();
w.work();
var w1=new Web('王五',22);
w1.run();
w1.work();
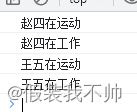
原型链+对象冒充继承的另一种方式
function Person(name, age) {
this.name = name;
this.age = age;
this.run = function () {
console.log(this.name + '在运动');
}
}
Person.prototype.sex = "男";
Person.prototype.work = function () {
console.log(this.name + '在工作');
}
function Web(name, age) {
Person.call(this, name, age);
}
Web.prototype = Person.prototype;
var w = new Web('赵四', 20);
w.run();
w.work();
var w1 = new Web('王五', 22);
w1.run()
w1.work();
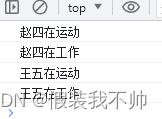