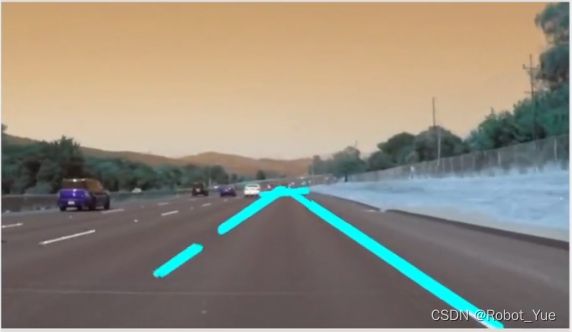
1. mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include
#include
#include
#include
#include
#include
#include
using namespace cv;
using namespace std;
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
void showFrame();
private slots:
void on_btnOpen_clicked();
void on_btnPlay_clicked();
private:
Ui::MainWindow *ui;
QLabel *infoLabel;
VideoCapture videoCap;
QTimer *videoTimer;
};
#endif
2. mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) {
ui->setupUi(this);
infoLabel = new QLabel();
infoLabel->setMinimumWidth(200);
this->statusBar()->addWidget(infoLabel);
videoTimer = new QTimer();
connect(videoTimer, &QTimer::timeout, this, &MainWindow::showFrame);
}
MainWindow::~MainWindow() {
delete ui;
}
void MainWindow::showFrame() {
Mat currentFrame;
videoCap >> currentFrame;
if (!currentFrame.empty()) {
infoLabel->setText("video is playing");
Mat gaussionFrame, grayFrame, cannyFrame;
GaussianBlur(currentFrame, gaussionFrame, Size(5, 5), 1, 0);
cvtColor(gaussionFrame, grayFrame, COLOR_BGR2GRAY);
Canny(grayFrame, cannyFrame, 50, 100);
Mat resultFrame;
Mat maskFrame = Mat::zeros(cannyFrame.size(), cannyFrame.type());
vector<Point>polyPoints;
polyPoints.push_back(Point(0, 368));
polyPoints.push_back(Point(300, 210));
polyPoints.push_back(Point(340, 210));
polyPoints.push_back(Point(640, 368));
fillPoly(maskFrame, vector<vector<Point>>{polyPoints}, Scalar(255, 255, 255));
bitwise_and(cannyFrame, maskFrame, resultFrame);
vector<Vec4i>lines;
HoughLinesP(resultFrame, lines, 1, CV_PI / 180, 5, 40, 20);
for (size_t i = 0; i < lines.size(); i++) {
Vec4i carLine = lines[i];
double lineSlope = (double(carLine[3]) - double(carLine[1])) / (double(carLine[2]) - double(carLine[0]));
if (lineSlope > 0) {
line(currentFrame, Point(carLine[0], carLine[1]), Point(carLine[2], carLine[3]), Scalar(0, 255, 255), 5, LINE_AA);
} else if (lineSlope < 0) {
line(currentFrame, Point(carLine[0], carLine[1]), Point(carLine[2], carLine[3]), Scalar(0, 255, 255), 5, LINE_AA);
}
}
QImage showImg = QImage((const unsigned char*)(currentFrame.data), currentFrame.cols, currentFrame.rows, currentFrame.step, QImage::Format_RGB888);
ui->labelPlay->setPixmap(QPixmap::fromImage(showImg.scaled(ui->labelPlay->size(), Qt::KeepAspectRatio, Qt::SmoothTransformation)));
} else {
infoLabel->setText("video is over");
qDebug() << "video is over!";
videoTimer->stop();
videoCap.release();
}
}
void MainWindow::on_btnOpen_clicked() {
QString videoPath = QFileDialog::getOpenFileName(this, "open video", ".", "video(*.mp4 *.avi, *.flv); All files(*.*)");
ui->lineEdit->setText(videoPath);
infoLabel->setText("video is loaded");
}
void MainWindow::on_btnPlay_clicked() {
ui->labelPlay->clear();
QString videoPath = ui->lineEdit->text();
videoCap.open(videoPath.toStdString());
double rate = videoCap.get(CAP_PROP_FPS);
double videoDelay = 1000 / rate;
videoTimer->start(videoDelay);
}