Spring Boot + VUE 实现PDF文件的打印预览
<!-- pdf实现 -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
<dependency>
<groupId>com.itextpdf.tool</groupId>
<artifactId>xmlworker</artifactId>
<version>5.5.13</version>
</dependency>
- 我是前端封装了post请求,所以这边请求类型直接使用的PostMapping。通过response返回文件流,前端解析直接加载到弹窗中
@PostMapping("/print")
public void print(@RequestBody Cld cld, HttpServletResponse response) throws Exception {
materialWeighService.print(cld, response);
}
- 制作添加PDF表单域,我的PDF模板文件存放在了项目目录中
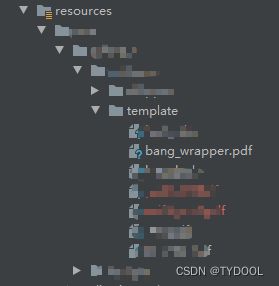
- 下面是具体实现
@Override
public void print(Cld cld, HttpServletResponse response) throws Exception {
String templatePath = "D:\\1\\2\\3\\pdfForm2.pdf";
String fileName = "resPDF";
response.setContentType("application/pdf;charset=UTF-8");
response.setHeader("Content-Disposition", "attachment;fileName="
+ URLEncoder.encode(fileName + ".pdf", "UTF-8"));
Map<String, String> map = new HashMap<>();
map.put("流水号", cld.get流水号());
map.put("供应单位", cld.get供应单位());
map.put("承运单位", cld.get承运单位());
map.put("车牌号", cld.get车牌号());
map.put("材料类别", cld.get进场分类());
map.put("材料规格", cld.get规格());
map.put("毛重", cld.get毛重() + "");
map.put("皮重", cld.get皮重() + "");
if (cld.getHan() == null) {
map.put("含水率", "");
} else {
map.put("含水率", cld.getHan() + "");
}
String kz = cld.get暗扣重量() + cld.get扣杂重量() + "";
map.put("扣除重量", kz);
map.put("扣后净重", cld.get净重() + "");
map.put("材料产地", cld.get生产单位());
map.put("过磅员", cld.get验收人());
map.put("过毛重日期时间", cld.get时间());
map.put("回皮日期时间", cld.get回皮时间());
PdfReader reader;
OutputStream os = null;
ByteArrayOutputStream baos = null;
PdfStamper stamper;
try {
os = response.getOutputStream();
reader = new PdfReader(templatePath);
baos = new ByteArrayOutputStream();
stamper = new PdfStamper(reader, baos);
AcroFields formTexts = stamper.getAcroFields();
BaseFont bf = BaseFont.createFont("C:/WINDOWS/Fonts/SIMSUN.TTC,1",
BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
formTexts.addSubstitutionFont(bf);
for (Map.Entry<String, String> entry : map.entrySet()) {
formTexts.setField(entry.getKey(), entry.getValue());
}
stamper.setFormFlattening(true);
stamper.close();
Document document = new Document();
PdfCopy copy = new PdfCopy(document, os);
document.open();
PdfImportedPage importedPage = copy.getImportedPage(new PdfReader(baos.toByteArray()), 1);
copy.addPage(importedPage);
document.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
baos.close();
os.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
- VUE前端请求
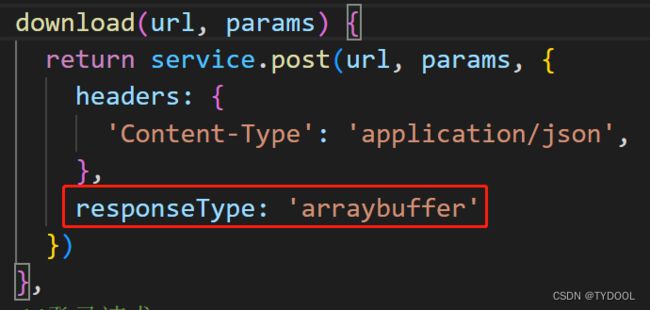
- 其中service是封装的axios请求,其中要注意的是responseType参数,相应类型需指定为arraybuffer,其他类型如blob返回的文件流处理后PDF白屏,没有内容。
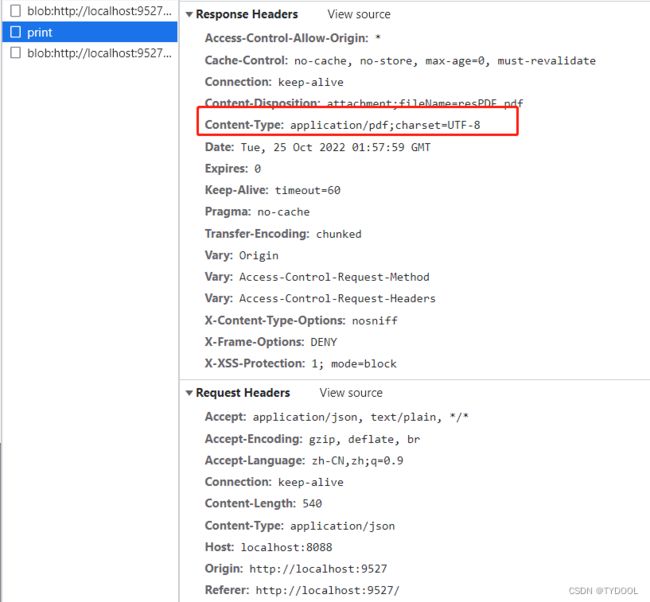
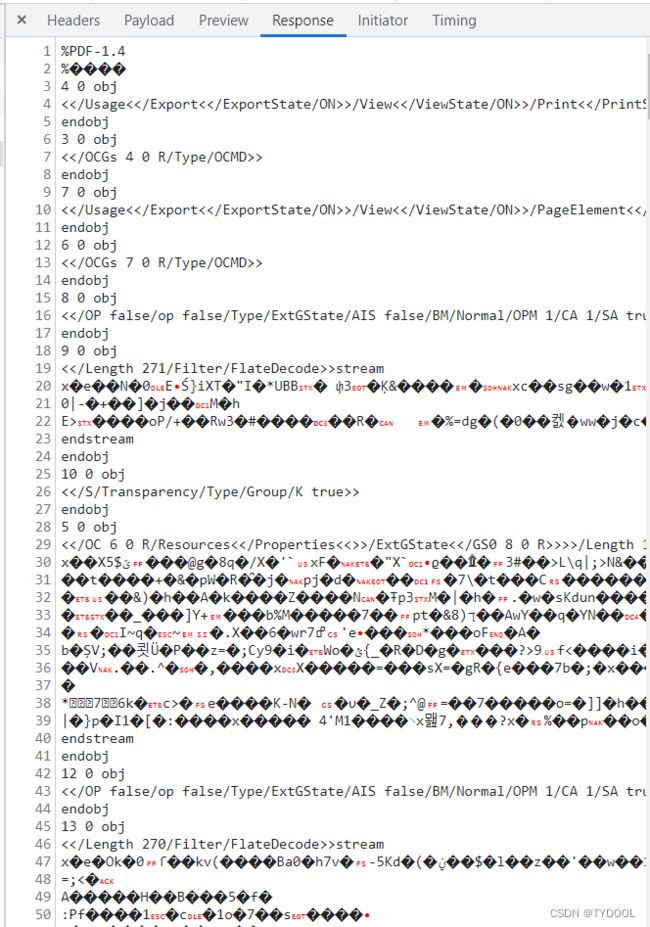
-
请求成功后response会返回如上的文件流
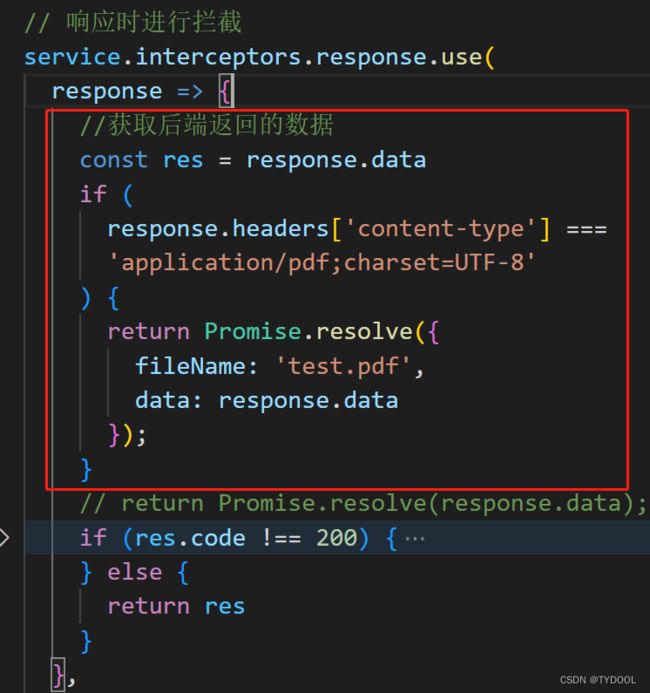
-
请求响应拦截器
-
具体实现
<template slot-scope="scope">
<el-button
type="primary"
size="mini"
round
icon="iconfont icon-a-ziyuan4"
@click="print(scope.row)">打印el-button>
template>
<print-dialog
:title="printDialog.title"
:visible="printDialog.visible"
:height="printDialog.height"
:width="printDialog.width"
@onClose="printClose()"
@onConfirm="printConfirm()">
<div slot="content">
<pdf ref="pdf" :src="pdfUrl">pdf>
div>
print-dialog>
import PrintDialog from '@/components/system/PrintDialog.vue'
import pdf from "vue-pdf-signature";
import CMapReaderFactory from "vue-pdf-signature/src/CMapReaderFactory.js";
export default {
components: {
PrintDialog,
pdf,
},
data() {
return {
printDialog: {
title: 'pdf',
visible: false,
height: 800,
width: 1670,
},
pdfUrl: '',
}
},
methods: {
async print(row) {
let params = {
pdf: row.组装参数,
...
...
}
let res = await materialWeighApi.print(params);
this.getObjectUrl(res.data);
this.printDialog.visible = true
},
},
getObjectUrl(data) {
let url= null;
let file = new Blob([data], {type: "application/pdf" });
if (window.createObjectURL != undefined) {
url = window.createObjectURL(file);
} else if (window.webkitURL != undefined) {
try {
url = window.webkitURL.createObjectURL(file);
} catch (error) {}
} else if (window.URL != undefined) {
try {
url = window.URL.createObjectURL(file);
} catch (error) {}
}
url = pdf.createLoadingTask({ url: url, CMapReaderFactory });
this.pdfUrl = url;
},
printClose() {
this.printDialog.visible = false;
},
printConfirm() {
this.$refs.pdf.print();
this.printDialog.visible = false
},
}