MyBatis-Plus(简称 MP)是一个 MyBatis 的增强工具,在 MyBatis 的基础上只做增强不做改变,为简化开发、提高效率而生。
1.2.为什么要学习Mybatis-Plus
我们已经学习过Mybatis这个框架,我们只需要在dao层定义抽象接口,基于Mybatis零实现的特性,就可以实现对数据库的crud操作。
如下两个接口:
UserMapper接口
public interface UserMapper {
int deleteByPrimaryKey(Long id);
int insert(User user);
List selectList();
User selectByPrimaryKey(Long id);
}
OrderMapper接口
public interface OrderMapper {
int deleteByPrimaryKey(Long id);
int insert(Order order);
List selectList();
User selectByPrimaryKey(Long id);
}
在上面两个业务接口中,我们发现:它们定义了一组类似的crud方法。
在业务类型比较多的时候,我们需要重复的定义这组功能类似的接口方法。
如何解决这个问题呢?
使用Mybatis-plus工具,我们只需要将我们定义的抽象接口,继承一个公用的BaseMapper
使用Mybatis-plus时,甚至都不需要任何的xml映射文件或者接口方法注解,真正的dao层零实现。
2.入门示例
2.1.需求
使用Mybatis-Plus实现对用户的crud操作。
2.2.配置步骤说明
(1)搭建环境(创建项目、导入包)
(2)配置Mybaits-Plus(基于Spring实现)
(3)编写测试代码
2.3.配置步骤
2.3.1.第一部分:搭建环境
2.3.1.1.前提
已经创建好了数据库环境:
CREATE TABLE tb_user
(
id
bigint(20) NOT NULL COMMENT '主键ID',
name
varchar(30) DEFAULT NULL COMMENT '姓名',
age
int(11) DEFAULT NULL COMMENT '年龄',
email
varchar(50) DEFAULT NULL COMMENT '邮箱',
PRIMARY KEY (id
)
)
2.3.1.2.说明
(1)Mybatis-Plus并没有提供单独的jar包,而是通过Maven(或者gradle)来管理jar依赖。本教程需要使用Maven构建项目。
(2)Mybatis-Plus是基于Spring框架实现的,因此使用Mybatis-Plus,必须导入Spring相关依赖。
2.3.1.3.第一步:创建一个Maven项目
因为我们只是测试MybatisPlus框架,所以创建一个jar项目就可以了
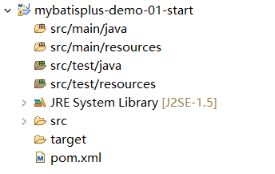
2.3.1.4.第二步:配置pom.xml构建文件
--需要导入依赖,并且配置项目的编码,JDK版本等构建信息
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
org.springframework
spring-jdbc
4.3.24.RELEASE
org.springframework
spring-context
4.3.24.RELEASE
com.baomidou
mybatis-plus
2.3.3
mysql
mysql-connector-java
5.1.47
org.apache.maven.plugins
maven-compiler-plugin
3.8.1
UTF-8
1.8
1.8
org.apache.maven.plugins
maven-surefire-plugin
2.22.2
true
2.3.2.第二部分:配置整合部分
整合MybatisPlus与Spring的配置。创建一个spirng-data.xml配置
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.3.xsd">
2.3.3.第三部分:实现操作功能
说明:完成实验MybatisPlus对数据库增删改查。
2.3.3.1.第一步:编写POJO
说明:使用Mybatis-Plus不使用xml文件,而是基于一组注解来解决实体类和数据库表的映射问题。
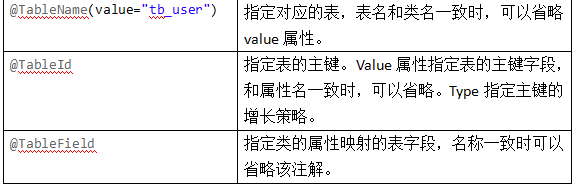
package org.chu.mybatisplus.pojo;
import com.baomidou.mybatisplus.annotations.TableField;
import com.baomidou.mybatisplus.annotations.TableId;
import com.baomidou.mybatisplus.annotations.TableName;
import com.baomidou.mybatisplus.enums.IdType;
@TableName(value="tb_user")
public class User {
@TableId(value="id",type=IdType.AUTO)
private Long id;//BIGINT(20) NOT NULL AUTO_INCREMENT COMMENT '主键ID',
//如果属性名与数据库表的字段名相同可以不写
@TableField(value="name")
private String name;//VARCHAR(30) NULL DEFAULT NULL COMMENT '姓名',
@TableField(value="age")
private Integer age;//INT(11) NULL DEFAULT NULL COMMENT '年龄',
@TableField(value="email")
private String email;//VARCHAR(50) NULL DEFAULT NULL COMMENT '邮箱',
//补全get/set方法
}
2.3.3.2.第二步:编写Mapper接口
package org.chu.mybatisplus.mapper;
import org.chu.mybatisplus.pojo.User;
import com.baomidou.mybatisplus.mapper.BaseMapper;
public interface UserMapper extends BaseMapper
}
2.3.3.3.第三步:测试增删改查
package org.chu.test.mapper;
import java.util.List;
import org.chu.mybatisplus.mapper.UserMapper;
import org.chu.mybatisplus.pojo.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
//注意事项:Maven的单元测试包必须放置测试源码包里面
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations="classpath:spring-data.xml")
public class UserMapperTest {
@Autowired
private UserMapper userMapper;
@Test
public void insert() {
try {
User user=new User();
user.setName("wangwu");
user.setAge(20);
user.setEmail("[email protected]");
Integer insert = userMapper.insert(user);
System.out.println(insert);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Test
public void deleteById() {
try {
Integer count = userMapper.deleteById(1L);
System.out.println(count);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Test
public void deleteByCondition() {
try {
//设置条件
EntityWrapper wrapper=new EntityWrapper<>();
wrapper.like("name", "%wang%");
Integer count = userMapper.delete(wrapper);
System.out.println(count);
} catch (Exception e) {
e.printStackTrace();
}
}
@Test
public void update() {
User user=new User();
user.setName("wangwu");
user.setEmail("[email protected]");
user.setId(2L);
userMapper.updateById(user);
}
@Test
public void findAll() {
List users = userMapper.selectList(null);
for (User user : users) {
System.out.println(user.getName());
}
}
}
3.常用配置
3.1.实体类全局配置
如果在配置文件指定实体类的全局配置,那么可以不需要再配置实体类的关联注解。
--配置文件spring-data.xml的修改
--实体类就可以去掉关联的注解了
package org.chu.mybatisplus.pojo;
public class User {
private Long id;
private String name;
private Integer age;
private String email;
//补全get、set方法
}
3.2.插件配置
Mybatis默认情况下,是不支持物理分页的。默认提供的RowBounds这个分页是逻辑分页来的。
所谓的逻辑分页,就是将数据库里面的数据全部查询出来后,在根据设置的参数返回对应的记录。(分页是在程序的内存中完成)。【表数据过多时,会溢出】
所谓的物理分页,就是根据条件限制,返回指定的记录。(分页在数据库里面已经完成)
MybatisPlus是默认使用RowBounds对象是支持物理分页的。但是需要通过配置Mybatis插件来开启。
配置代码
3.3.自定义SQL语句支持
--实现代码
package org.chu.mybatisplus.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Select;
import org.chu.mybatisplus.pojo.User;
import com.baomidou.mybatisplus.mapper.BaseMapper;
public interface UserMapper extends BaseMapper
@Select(value="select * from tb_user")
List
}
--测试代码
package org.chu.test.mapper;
import java.util.List;
import org.chu.mybatisplus.mapper.UserMapper;
import org.chu.mybatisplus.pojo.User;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
//注意事项:Maven的单元测试包必须放置测试源码包里面
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations="classpath:spring-data.xml")
public class UserMapperTest {
@Autowired
private UserMapper userMapper;
@Test
public void findAll() {
try {
List users = userMapper.findAll();
for (User user : users) {
System.out.println(user.getName());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}