单库操作
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-data-redisartifactId>
dependency>
spring:
redis:
database: 2
host: 127.0.0.1
port: 6379
password: 123456
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
Jackson2JsonRedisSerializer<Object> jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer<>(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.activateDefaultTyping(LaissezFaireSubTypeValidator.instance, ObjectMapper.DefaultTyping.NON_FINAL, JsonTypeInfo.As.PROPERTY);
JavaTimeModule javaTimeModule = new JavaTimeModule();
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern(DateUtil.DateStyle.YYYY_MM_DD_HH_MM_SS.getValue());
javaTimeModule.addSerializer(LocalDateTime.class, new LocalDateTimeSerializer(dateTimeFormatter));
javaTimeModule.addDeserializer(LocalDateTime.class, new LocalDateTimeDeserializer(dateTimeFormatter));
om.registerModule(javaTimeModule);
jackson2JsonRedisSerializer.setObjectMapper(om);
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(factory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(jackson2JsonRedisSerializer);
template.setHashKeySerializer(jackson2JsonRedisSerializer);
template.setHashValueSerializer(jackson2JsonRedisSerializer);
template.setDefaultSerializer(new StringRedisSerializer());
template.afterPropertiesSet();
return template;
}
@Autowired(required = false)
private RedisTemplate<String, Object> redisTemplate;
@Test
public void testRedis() {
redisTemplate.opsForValue().set("redisTemplate", "redisTemplate");
Object value = redisTemplate.opsForValue().get("redisTemplate");
System.out.println("redis设置的值为:" + value);
}
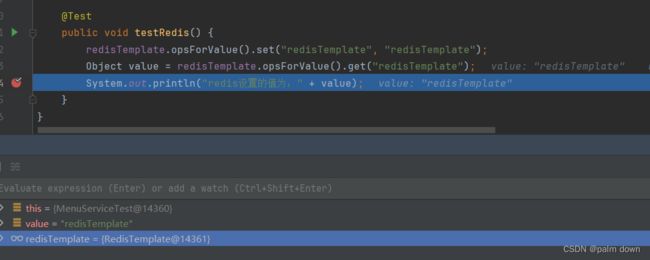
- 能够正常往redis设置数据,也可以正常获取到
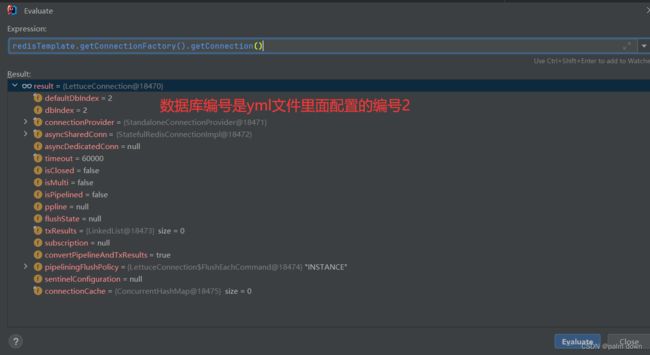
- 可以看到数据库编号是yml里面配置的database编号
多库操作
- 使用lettuce连接池,添加commons-pools依赖
<dependency>
<groupId>org.apache.commonsgroupId>
<artifactId>commons-pool2artifactId>
dependency>
import lombok.Data;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
@Data
@Configuration
public class RedisConfigProperties {
@Value("${spring.redis.host}")
private String host;
@Value("${spring.redis.port}")
private int port;
@Value("${spring.redis.password}")
private String password;
private int timeout = 200;
private int maxActive = 200;
private int maxIdle = 8;
private int minIdle = 0;
private int maxWait = 100;
}
@Bean
public GenericObjectPoolConfig getPoolConfig() {
GenericObjectPoolConfig poolConfig = new GenericObjectPoolConfig();
poolConfig.setMaxTotal(redisConfigProperties.getMaxActive());
poolConfig.setMaxIdle(redisConfigProperties.getMaxIdle());
poolConfig.setMinIdle(redisConfigProperties.getMinIdle());
poolConfig.setMaxWaitMillis(redisConfigProperties.getMaxWait());
return poolConfig;
}
private RedisTemplate<String, Object> redisTemplateFactory(int database) {
RedisStandaloneConfiguration configuration = new RedisStandaloneConfiguration();
configuration.setHostName(redisConfigProperties.getHost());
configuration.setPort(redisConfigProperties.getPort());
configuration.setPassword(RedisPassword.of(redisConfigProperties.getPassword()));
LettucePoolingClientConfiguration clientConfiguration = LettucePoolingClientConfiguration.builder()
.commandTimeout(Duration.ofSeconds(redisConfigProperties.getTimeout())).poolConfig(getPoolConfig()).build();
LettuceConnectionFactory factory = new LettuceConnectionFactory(configuration, clientConfiguration);
factory.setDatabase(database);
factory.afterPropertiesSet();
Jackson2JsonRedisSerializer<Object> jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer<>(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.activateDefaultTyping(LaissezFaireSubTypeValidator.instance, ObjectMapper.DefaultTyping.NON_FINAL, JsonTypeInfo.As.PROPERTY);
JavaTimeModule javaTimeModule = new JavaTimeModule();
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern(DateUtil.DateStyle.YYYY_MM_DD_HH_MM_SS.getValue());
javaTimeModule.addSerializer(LocalDateTime.class, new LocalDateTimeSerializer(dateTimeFormatter));
javaTimeModule.addDeserializer(LocalDateTime.class, new LocalDateTimeDeserializer(dateTimeFormatter));
om.registerModule(javaTimeModule);
jackson2JsonRedisSerializer.setObjectMapper(om);
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(factory);
template.setKeySerializer(new StringRedisSerializer());
template.setValueSerializer(jackson2JsonRedisSerializer);
template.setHashKeySerializer(jackson2JsonRedisSerializer);
template.setHashValueSerializer(jackson2JsonRedisSerializer);
template.setDefaultSerializer(new StringRedisSerializer());
template.afterPropertiesSet();
return template;
}
@Bean
public RedisTemplate<String, Object> redisTemplate0() {
return this.redisTemplateFactory(0);
}
@Bean
public RedisTemplate<String, Object> redisTemplate1() {
return this.redisTemplateFactory(1);
}
@Autowired(required = false)
@Qualifier("redisTemplate0")
private RedisTemplate<String, Object> redisTemplate0;
@Autowired(required = false)
@Qualifier("redisTemplate1")
private RedisTemplate<String, Object> redisTemplate1;
@Test
public void testRedis() {
redisTemplate0.opsForValue().set("redisTemplate0", "redisTemplate0");
Object value0 = redisTemplate0.opsForValue().get("redisTemplate0");
System.out.println("redis0设置的值为:" + value);
redisTemplate1.opsForValue().set("redisTemplate1", "redisTemplate1");
Object value1 = redisTemplate0.opsForValue().get("redisTemplate1");
System.out.println("redis1设置的值为:" + value1);
}