要求:
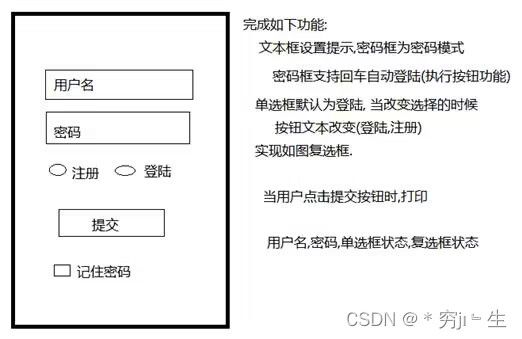
运行结果:
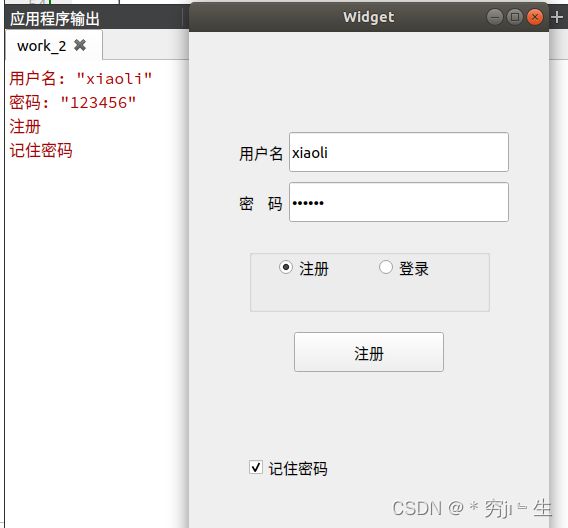
代码:
widget.h
#ifndef WIDGET_H
#define WIDGET_H
#include
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
public slots:
void rdBtnStateChangedSlotFun();
void btnClickedslotFun();
private:
Ui::Widget *ui;
};
#endif // WIDGET_H
widget.cpp
#include "widget.h"
#include "ui_widget.h"
#include
#include
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
ui->btn->setText("登录");
ui->ltxtName->setPlaceholderText("请输入用户名");
ui->ltxtPassword->setPlaceholderText("请输入密码");
ui->ltxtPassword->setEchoMode(QLineEdit::Password);
ui->rdBtnLogon->setChecked(true);
connect(ui->ltxtPassword,SIGNAL(editingFinished()),this,SLOT(btnClickedslotFun()));
connect(ui->rdBtnRegister,SIGNAL(clicked()),this,SLOT(rdBtnStateChangedSlotFun()));
connect(ui->rdBtnLogon,SIGNAL(clicked()),this,SLOT(rdBtnStateChangedSlotFun()));
connect(ui->btn,SIGNAL(clicked()),this,SLOT(btnClickedslotFun()));
}
Widget::~Widget()
{
delete ui;
}
void Widget::rdBtnStateChangedSlotFun()
{
qDebug()<<__func__<<__LINE__;
if(ui->rdBtnLogon->isChecked())
{
ui->btn->setText("登录");
}
if(ui->rdBtnRegister->isChecked())
{
ui->btn->setText("注册");
}
}
void Widget::btnClickedslotFun()
{
qDebug() << "用户名:" << ui->ltxtName->text();
qDebug() << "密码:" << ui->ltxtPassword->text();
if(ui->rdBtnLogon->isChecked())
{
qDebug() << "登录";
}
if(ui->rdBtnRegister->isChecked())
{
qDebug() << "注册";
}
if(ui->cbtnRemberPassword->isChecked())
{
qDebug() << "记住密码";
}
else
{
qDebug() << "取消记住密码";
}
}
ui_widget.h
/********************************************************************************
** Form generated from reading UI file 'widget.ui'
**
** Created by: Qt User Interface Compiler version 5.12.9
**
** WARNING! All changes made in this file will be lost when recompiling UI file!
********************************************************************************/
#ifndef UI_WIDGET_H
#define UI_WIDGET_H
#include
#include
#include
#include
#include
#include
#include
#include
#include
QT_BEGIN_NAMESPACE
class Ui_Widget
{
public:
QLineEdit *ltxtName;
QLineEdit *ltxtPassword;
QLabel *label;
QLabel *label_2;
QPushButton *btn;
QCheckBox *cbtnRemberPassword;
QGroupBox *groupBox;
QRadioButton *rdBtnLogon;
QRadioButton *rdBtnRegister;
void setupUi(QWidget *Widget)
{
if (Widget->objectName().isEmpty())
Widget->setObjectName(QString::fromUtf8("Widget"));
Widget->resize(360, 590);
ltxtName = new QLineEdit(Widget);
ltxtName->setObjectName(QString::fromUtf8("ltxtName"));
ltxtName->setGeometry(QRect(100, 100, 220, 40));
ltxtPassword = new QLineEdit(Widget);
ltxtPassword->setObjectName(QString::fromUtf8("ltxtPassword"));
ltxtPassword->setGeometry(QRect(100, 150, 220, 40));
label = new QLabel(Widget);
label->setObjectName(QString::fromUtf8("label"));
label->setGeometry(QRect(50, 100, 50, 40));
label_2 = new QLabel(Widget);
label_2->setObjectName(QString::fromUtf8("label_2"));
label_2->setGeometry(QRect(50, 150, 50, 40));
btn = new QPushButton(Widget);
btn->setObjectName(QString::fromUtf8("btn"));
btn->setGeometry(QRect(105, 300, 150, 40));
cbtnRemberPassword = new QCheckBox(Widget);
cbtnRemberPassword->setObjectName(QString::fromUtf8("cbtnRemberPassword"));
cbtnRemberPassword->setGeometry(QRect(60, 420, 120, 30));
groupBox = new QGroupBox(Widget);
groupBox->setObjectName(QString::fromUtf8("groupBox"));
groupBox->setGeometry(QRect(60, 200, 241, 80));
rdBtnLogon = new QRadioButton(groupBox);
rdBtnLogon->setObjectName(QString::fromUtf8("rdBtnLogon"));
rdBtnLogon->setGeometry(QRect(130, 20, 80, 30));
rdBtnRegister = new QRadioButton(groupBox);
rdBtnRegister->setObjectName(QString::fromUtf8("rdBtnRegister"));
rdBtnRegister->setGeometry(QRect(30, 20, 80, 30));
retranslateUi(Widget);
QMetaObject::connectSlotsByName(Widget);
} // setupUi
void retranslateUi(QWidget *Widget)
{
Widget->setWindowTitle(QApplication::translate("Widget", "Widget", nullptr));
label->setText(QApplication::translate("Widget", "\347\224\250\346\210\267\345\220\215", nullptr));
label_2->setText(QApplication::translate("Widget", "\345\257\206 \347\240\201", nullptr));
btn->setText(QApplication::translate("Widget", "\346\217\220\344\272\244", nullptr));
cbtnRemberPassword->setText(QApplication::translate("Widget", "\350\256\260\344\275\217\345\257\206\347\240\201", nullptr));
groupBox->setTitle(QString());
rdBtnLogon->setText(QApplication::translate("Widget", "\347\231\273\345\275\225", nullptr));
rdBtnRegister->setText(QApplication::translate("Widget", "\346\263\250\345\206\214", nullptr));
} // retranslateUi
};
namespace Ui {
class Widget: public Ui_Widget {};
} // namespace Ui
QT_END_NAMESPACE
#endif // UI_WIDGET_H
main.cpp
#include "widget.h"
#include
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Widget w;
w.show();
return a.exec();
}