题目大致意思要求一个字符串所有子字符串(长度从1到总长,但顺序不同,如ab和ba算一个)
First Phone Interview:
1.提出尽可能多的方法使一个method可以返回多个不同type的值
2.reverse string
比如 "I have a dream" -> "dream a have I"
3.判断一个binary tree是不是对称的
Second Phone Interview:
1.给a list of number,返回前top K个(内存足够怎么做,内存不够怎么做)
2.用OOD来电梯
3.找两个链表的交集
Onsite 6轮 1轮HR 1轮午餐 4轮技术 (亚马逊web services team)
1.设计个电话本, 可以用那些数据结构
答案貌似可以用suffix tree或者hashtable
2.问research, OOD 交通灯系统
3.写函数算一个整数的阶层 n!, 又问了n很大,怎么办?
比如99%的n都在400000-900000之间,怎么提高函数的执行速度
4.给一个数组和一个数n,找出数组中的所有的对和等于n的
5.给手机键盘,给定某个按键序列比如'1489',返回这个按键序列生成的所有的正确单词
1.判断一年是否为闰年的方法
- #include"stdio.h"
- intisRun(intnum)
- {
- intret=0;
- if(num%4==0)
- {
- ret=1;
- if(num%100==0)
- {
- ret=0;
- }
- if(num%400==0)
- {
- ret=1;
- }
- }
- returnret;
- }
- intmain()
- {
- intyear;
- scanf("%d",&year);
- if(isRun(year))
- {
- printf("run\n");
- }
- else
- {
- printf("no\n");
- }
- return0;
- }
2 Please write a program which can print all 6 digitsnumbers composed of 1, 2, 2,3,4,5.
- #include"stdio.h"
- voidshowAnother(char*str,intstart,intnum)
- {
- inti=0;
- charc;
- if(str[start]=='\0')
- {
- printf("%s\n",str);
- }
- if(str==NULL)
- return;
- for(i=start;i<num;i++)
- {
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- showAnother(str,start+1,num);
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- }
- }
- voidshowDigit(char*str,intstart,intnum)
- {
- inti=0;
- charc;
- if(str==NULL)
- return;
- for(i=start;i<num;i++)
- {
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- showAnother(str,start+1,num);
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- }
- }
- intmain()
- {
- charstr[]="122345";
- showDigit(str,0,sizeof(str)/sizeof(str[0]));
- }
3 题目:输入一个英文句子,翻转句子中单词的顺序,但单词内字符的顺序不变。句子中单词以空格符隔开。为简单起见,标点符号和普通字母一样处理。
例如输入“I am astudent.”,则输出“student. a am I”。
- #include"stdio.h"
- #include"string.h"
- #defineMAX1024
- #defineCHARLEN256
- voidstrReverse(char*str,intstart,intlen)
- {
- intbegin;
- intend;
- inttemp,i;
- charc;
- if(str==NULL)
- return;
- begin=start;
- for(end=start;end<=len;end++)
- {
- if(str[end]==''||str[end]=='\0')
- {
- temp=end;
- temp-=1;
- for(;begin<temp;begin++,temp--)
- {
- c=str[temp];
- str[temp]=str[begin];
- str[begin]=c;
- }
- begin=end+1;
- }
- }
- end=len-1;
- for(i=start;i<end;i++,end--)
- {
- c=str[i];
- str[i]=str[end];
- str[end]=c;
- }
- }
- intmain()
- {
- charstr[MAX];
- inti;
- intlen;
- gets(str);
- len=strlen(str);
- strReverse(str,0,len);
- printf("%s\n",str);
- return0;
- }
4 题目:输入两个字符串,从第一字符串中删除第二个字符串中所有的字符。例如,输入”Theyare students.”和”aeiou”,则删除之后的第一个字符串变成”Thy r stdnts.”。
- #include"stdio.h"
- #include"string.h"
- #defineMAX1024
- #defineCHARLEN256
- intmain()
- {
- charstr[MAX];
- charstrToMove[MAX];
- intmask[CHARLEN];
- inti;
- intlen;
- intlenToMove;
- memset(mask,0,sizeof(int)*CHARLEN);
- gets(str);
- getchar();
- scanf("%s",strToMove);
- len=strlen(str);
- lenToMove=strlen(strToMove);
- for(i=0;i<lenToMove;i++)
- {
- mask[strToMove[i]]=1;
- }
- for(i=0;i<len;i++)
- {
- if(!mask[str[i]])
- printf("%c",str[i]);
- }
- putchar('\n');
- }
5 求字符串所有子字符串(顺序不同算一个)
- #include"stdio.h"
- #include"string.h"
- #include"math.h"
- #defineMAX1024
- #defineCHARLEN256
- intmain()
- {
- charstr[MAX];
- intlen;
- intnumber;
- inti,j;
- scanf("%s",str);
- len=strlen(str);
- number=pow(2,len);
- printf("Allstrlist\n");
- for(i=1;i<number;i++)
- {
- for(j=0;j<len;j++)
- {
- if((i>>j)&1)
- {
- putchar(str[j]);
- }
- }
- putchar('\n');
- }
- }
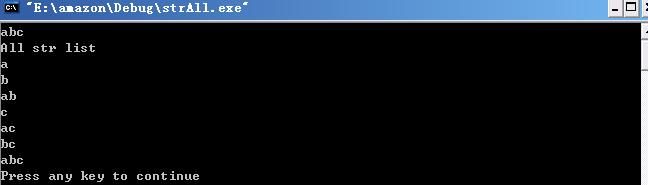
当字符串重复的时候, 先排序nlogn,此时重复的字符会变成连续的,bcaeb 排序以后,abbce, 在用O(n)把排序数组去重,再用上面方法。
6. Swap two nodes in list
SWAP(node *head, node *first, node *second)
这道题目 还要构建节点比较复杂,题目的意思 就是交换两个节点。
当然这道题目 需要指针操作。
不能进行值的交换,因为类型不明哦。
7Find Longest Repeat SubString ,可以overlap,区分大小写
这道题一看就是典型的后缀数组的题目
应该比较简单
我跑一遍试试。
- #include"stdio.h"
- #include"string.h"
- #include"math.h"
- #include"assert.h"
- #defineMAX1024
- #defineCHARLEN256
- intPartion(char*str[MAX],ints,inte)
- {
- inti=s-1;
- intj;
- char*p=str[e];
- char*temp;
- for(j=s;j<e;j++)
- {
- if(strcmp(str[j],p)<0)
- {
- temp=str[i+1];
- str[i+1]=str[j];
- str[j]=temp;
- i+=1;
- }
- }
- temp=str[i+1];
- str[i+1]=p;
- str[e]=temp;
- returni+1;
- }
- voidquickSort(char*str[MAX],intstart,intend)
- {
- intq;
- if(start<end)
- {
- q=Partion(str,start,end);
- quickSort(str,start,q-1);
- quickSort(str,q+1,end);
- }
- }
- intcomLen(char*str1,char*str2)
- {
- intcount=0;
- assert(str1&&str2);
- while(*str1==*str2)
- {
- if(*str1=='\0'||*str2=='\0')
- break;
- count++;
- str1++;
- str2++;
- }
- returncount;
- }
- intmain()
- {
- charstr[MAX];
- charanswer[MAX];
- char*strPoint[MAX];
- inti,len;
- intmaxLen,clen;
- scanf("%s",str);
- len=strlen(str);
- for(i=0;i<len;i++)
- {
- strPoint[i]=str+i;
- }
- quickSort(strPoint,0,len-1);
- maxLen=0;
- for(i=0;i<len-1;i++)
- {
- clen=comLen(strPoint[i],strPoint[i+1]);
- if(clen>maxLen)
- {
- strncpy(answer,strPoint[i],clen);
- answer[clen]='\0';
- maxLen=clen;
- }
- }
- printf("%s\n",answer);
- }
用快排+后缀数组来解决的,还好测试的比较顺利
First Phone Interview:
1.提出尽可能多的方法使一个method可以返回多个不同type的值
2.reverse string
比如 "I have a dream" -> "dream a have I"
3.判断一个binary tree是不是对称的
Second Phone Interview:
1.给a list of number,返回前top K个(内存足够怎么做,内存不够怎么做)
2.用OOD来电梯
3.找两个链表的交集
Onsite 6轮 1轮HR 1轮午餐 4轮技术 (亚马逊web services team)
1.设计个电话本, 可以用那些数据结构
答案貌似可以用suffix tree或者hashtable
2.问research, OOD 交通灯系统
3.写函数算一个整数的阶层 n!, 又问了n很大,怎么办?
比如99%的n都在400000-900000之间,怎么提高函数的执行速度
4.给一个数组和一个数n,找出数组中的所有的对和等于n的
5.给手机键盘,给定某个按键序列比如'1489',返回这个按键序列生成的所有的正确单词
1.判断一年是否为闰年的方法
- #include"stdio.h"
- intisRun(intnum)
- {
- intret=0;
- if(num%4==0)
- {
- ret=1;
- if(num%100==0)
- {
- ret=0;
- }
- if(num%400==0)
- {
- ret=1;
- }
- }
- returnret;
- }
- intmain()
- {
- intyear;
- scanf("%d",&year);
- if(isRun(year))
- {
- printf("run\n");
- }
- else
- {
- printf("no\n");
- }
- return0;
- }
2 Please write a program which can print all 6 digitsnumbers composed of 1, 2, 2,3,4,5.
- #include"stdio.h"
- voidshowAnother(char*str,intstart,intnum)
- {
- inti=0;
- charc;
- if(str[start]=='\0')
- {
- printf("%s\n",str);
- }
- if(str==NULL)
- return;
- for(i=start;i<num;i++)
- {
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- showAnother(str,start+1,num);
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- }
- }
- voidshowDigit(char*str,intstart,intnum)
- {
- inti=0;
- charc;
- if(str==NULL)
- return;
- for(i=start;i<num;i++)
- {
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- showAnother(str,start+1,num);
- c=str[start];
- str[start]=str[i];
- str[i]=c;
- }
- }
- intmain()
- {
- charstr[]="122345";
- showDigit(str,0,sizeof(str)/sizeof(str[0]));
- }
3题目:输入一个英文句子,翻转句子中单词的顺序,但单词内字符的顺序不变。句子中单词以空格符隔开。为简单起见,标点符号和普通字母一样处理。
例如输入“I am astudent.”,则输出“student. a am I”。
- #include"stdio.h"
- #include"string.h"
- #defineMAX1024
- #defineCHARLEN256
- voidstrReverse(char*str,intstart,intlen)
- {
- intbegin;
- intend;
- inttemp,i;
- charc;
- if(str==NULL)
- return;
- begin=start;
- for(end=start;end<=len;end++)
- {
- if(str[end]==''||str[end]=='\0')
- {
- temp=end;
- temp-=1;
- for(;begin<temp;begin++,temp--)
- {
- c=str[temp];
- str[temp]=str[begin];
- str[begin]=c;
- }
- begin=end+1;
- }
- }
- end=len-1;
- for(i=start;i<end;i++,end--)
- {
- c=str[i];
- str[i]=str[end];
- str[end]=c;
- }
- }
- intmain()
- {
- charstr[MAX];
- inti;
- intlen;
- gets(str);
- len=strlen(str);
- strReverse(str,0,len);
- printf("%s\n",str);
- return0;
- }
4题目:输入两个字符串,从第一字符串中删除第二个字符串中所有的字符。例如,输入”Theyare students.”和”aeiou”,则删除之后的第一个字符串变成”Thy r stdnts.”。
- #include"stdio.h"
- #include"string.h"
- #defineMAX1024
- #defineCHARLEN256
- intmain()
- {
- charstr[MAX];
- charstrToMove[MAX];
- intmask[CHARLEN];
- inti;
- intlen;
- intlenToMove;
- memset(mask,0,sizeof(int)*CHARLEN);
- gets(str);
- getchar();
- scanf("%s",strToMove);
- len=strlen(str);
- lenToMove=strlen(strToMove);
- for(i=0;i<lenToMove;i++)
- {
- mask[strToMove[i]]=1;
- }
- for(i=0;i<len;i++)
- {
- if(!mask[str[i]])
- printf("%c",str[i]);
- }
- putchar('\n');
- }
5 求字符串所有子字符串(顺序不同算一个)
题目大致意思要求一个字符串所有子字符串(长度从1到总长,但顺序不同,如ab和ba算一个)
此题有个解法可以利用二进制处理【只能是当字符串中不出现重复字符的时候】
- #include"stdio.h"
- #include"string.h"
- #include"math.h"
- #defineMAX1024
- #defineCHARLEN256
- intmain()
- {
- charstr[MAX];
- intlen;
- intnumber;
- inti,j;
- scanf("%s",str);
- len=strlen(str);
- number=pow(2,len);
- printf("Allstrlist\n");
- for(i=1;i<number;i++)
- {
- for(j=0;j<len;j++)
- {
- if((i>>j)&1)
- {
- putchar(str[j]);
- }
- }
- putchar('\n');
- }
- }
当字符串重复的时候, 先排序nlogn,此时重复的字符会变成连续的,bcaeb 排序以后,abbce, 在用O(n)把排序数组去重,再用上面方法。
6. Swap two nodes in list
SWAP(node *head, node *first, node *second)
这道题目 还要构建节点比较复杂,题目的意思 就是交换两个节点。
当然这道题目 需要指针操作。
不能进行值的交换,因为类型不明哦。
7Find Longest Repeat SubString ,可以overlap,区分大小写
这道题一看就是典型的后缀数组的题目
应该比较简单
我跑一遍试试。
- #include"stdio.h"
- #include"string.h"
- #include"math.h"
- #include"assert.h"
- #defineMAX1024
- #defineCHARLEN256
- intPartion(char*str[MAX],ints,inte)
- {
- inti=s-1;
- intj;
- char*p=str[e];
- char*temp;
- for(j=s;j<e;j++)
- {
- if(strcmp(str[j],p)<0)
- {
- temp=str[i+1];
- str[i+1]=str[j];
- str[j]=temp;
- i+=1;
- }
- }
- temp=str[i+1];
- str[i+1]=p;
- str[e]=temp;
- returni+1;
- }
- voidquickSort(char*str[MAX],intstart,intend)
- {
- intq;
- if(start<end)
- {
- q=Partion(str,start,end);
- quickSort(str,start,q-1);
- quickSort(str,q+1,end);
- }
- }
- intcomLen(char*str1,char*str2)
- {
- intcount=0;
- assert(str1&&str2);
- while(*str1==*str2)
- {
- if(*str1=='\0'||*str2=='\0')
- break;
- count++;
- str1++;
- str2++;
- }
- returncount;
- }
- intmain()
- {
- charstr[MAX];
- charanswer[MAX];
- char*strPoint[MAX];
- inti,len;
- intmaxLen,clen;
- scanf("%s",str);
- len=strlen(str);
- for(i=0;i<len;i++)
- {
- strPoint[i]=str+i;
- }
- quickSort(strPoint,0,len-1);
- maxLen=0;
- for(i=0;i<len-1;i++)
- {
- clen=comLen(strPoint[i],strPoint[i+1]);
- if(clen>maxLen)
- {
- strncpy(answer,strPoint[i],clen);
- answer[clen]='\0';
- maxLen=clen;
- }
- }
- printf("%s\n",answer);
- }
用快排+后缀数组来解决的,还好测试的比较顺利