【Java EE】ANT+Xdoclet自动生成Hibernate配置文件
1、下载Xdoclet,网址: http://xdoclet.sourceforge.net/,解压2、新建包com.test.model,存放实体类Group,User
Group.java
1
package
com.test.model;
2
3
import
java.util.Set;
4
5
/**
6
*
7
* @author Administrator
8
*@hibernate.class table="t_group"
9
*/
10
public
class
Group
{
11
/**
12
* @hibernate.id
13
* generator-class="native"
14
*/
15
private int groupid;
16
/**
17
* @hibernate.property
18
*/
19
private String groupname;
20
/**
21
* @hibernate.property
22
*/
23
private String remark;
24
25
/**
26
* @hibernate.set
27
* inverse="true"
28
* @hibernate.key column="groupid"
29
* @hibernate.one-to-many
30
* class="com.test.model.User"
31
*/
32
private Set<User> users;
33
public int getGroupid() {
34
return groupid;
35
}
36
37
public void setGroupid(int groupid) {
38
this.groupid = groupid;
39
}
40
41
public String getGroupname() {
42
return groupname;
43
}
44
45
public void setGroupname(String groupname) {
46
this.groupname = groupname;
47
}
48
49
public String getRemark() {
50
return remark;
51
}
52
53
public void setRemark(String remark) {
54
this.remark = remark;
55
}
56
57
public Set<User> getUsers() {
58
return users;
59
}
60
61
public void setUsers(Set<User> users) {
62
this.users = users;
63
}
64
65
}
User.java

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

1
package
com.test.model;
2
3
import
java.util.Date;
4
5
/**
6
*
7
* @author Administrator
8
*@hibernate.class table="t_user"
9
*/
10
public
class
User
{
11
/**
12
* @hibernate.id
13
* generator-class="native"
14
*/
15
private int userid;
16
/**
17
* @hibernate.property
18
*/
19
private String username;
20
/**
21
* @hibernate.many-to-one
22
* column="groupid"
23
* cascade="all"
24
* class="com.test.model.Group"
25
*/
26
private Group group;
27
/**
28
* @hibernate.property
29
*/
30
private String password;
31
/**
32
* @hibernate.property
33
*/
34
private String email;
35
/**
36
* @hibernate.property
37
*/
38
private String question;
39
/**
40
* @hibernate.property
41
*/
42
private String answer;
43
/**
44
* @hibernate.property
45
*/
46
private Date createtime;
47
/**
48
* @hibernate.property
49
*/
50
private int status;
51
52
public String getEmail() {
53
return email;
54
}
55
56
public void setEmail(String email) {
57
this.email = email;
58
}
59
60
public String getQuestion() {
61
return question;
62
}
63
64
public void setQuestion(String question) {
65
this.question = question;
66
}
67
68
public String getAnswer() {
69
return answer;
70
}
71
72
public void setAnswer(String answer) {
73
this.answer = answer;
74
}
75
76
public Date getCreatetime() {
77
return createtime;
78
}
79
80
public void setCreatetime(Date createtime) {
81
this.createtime = createtime;
82
}
83
84
public int getStatus() {
85
return status;
86
}
87
88
public void setStatus(int status) {
89
this.status = status;
90
}
91
92
public int getUserid() {
93
return userid;
94
}
95
96
public void setUserid(int userid) {
97
this.userid = userid;
98
}
99
100
public String getUsername() {
101
return username;
102
}
103
104
public void setUsername(String username) {
105
this.username = username;
106
}
107
108
public String getPassword() {
109
return password;
110
}
111
112
public void setPassword(String password) {
113
this.password = password;
114
}
115
116
public Group getGroup() {
117
return group;
118
}
119
120
public void setGroup(Group group) {
121
this.group = group;
122
}
123
124
125
}

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

3、在test目录下建立build.xml,其中 < property name ="xdoclet.home" value ="C:/xdoclet-plugins-dist-1.0.4" />为你所解压的xdoclet目录。
1
<?
xml version="1.0" encoding="GBK"
?>
2
<
project
name
="test系统构建脚本"
default
="生成Hibernate配置文件"
basedir
="."
>
3
4
<
property
name
="src.dir"
value
="${basedir}/src"
/>
5
<
property
name
="xdoclet.home"
value
="C:/xdoclet-plugins-dist-1.0.4"
/>
6
7
<!--
build classpath
-->
8
<
path
id
="xdoclet.task.classpath"
>
9
<
fileset
dir
="${xdoclet.home}/lib"
>
10
<
include
name
="**/*.jar"
/>
11
</
fileset
>
12
</
path
>
13
14
<
taskdef
15
name
="xdoclet"
16
classname
="org.xdoclet.ant.XDocletTask"
17
classpathref
="xdoclet.task.classpath"
18
/>
19
20
<
target
name
="生成Hibernate配置文件"
>
21
<
xdoclet
>
22
<
fileset
dir
="${src.dir}/com/test/model"
>
23
<
include
name
="**/*.java"
/>
24
</
fileset
>
25
<
component
26
classname
="org.xdoclet.plugin.hibernate.HibernateConfigPlugin"
27
destdir
="${src.dir}"
28
version
="3.0"
29
hbm2ddlauto
="update"
30
jdbcurl
="jdbc:mysql://localhost/test"
31
jdbcdriver
="com.mysql.jdbc.Driver"
32
jdbcusername
="root"
33
jdbcpassword
="intrl"
34
dialect
="org.hibernate.dialect.MySQLDialect"
35
showsql
="true"
36
/>
37
</
xdoclet
>
38
</
target
>
39
40
<
target
name
="生成hibernate映射文件"
>
41
<
xdoclet
>
42
<
fileset
dir
="${src.dir}/com/test/model"
>
43
<
include
name
="**/*.java"
/>
44
</
fileset
>
45
<
component
46
classname
="org.xdoclet.plugin.hibernate.HibernateMappingPlugin"
47
version
="3.0"
48
destdir
="${src.dir}"
49
/>
50
</
xdoclet
>
51
</
target
>
52
53
</
project
>
4、在MyEclipse中配置ANT,添加刚才所建立的build.xml,并运行其两个功能项,刷新项目目录

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

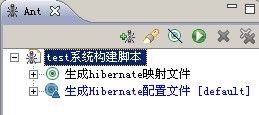
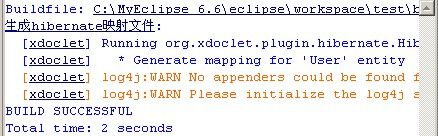
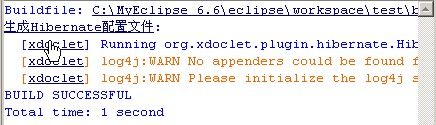
刷新项目目录后的项目目录结构如下:
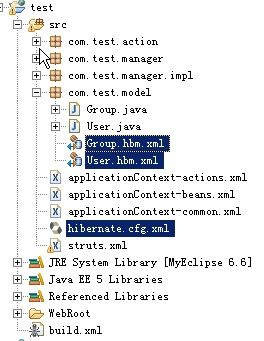
Group.hbm.xml
1
<?
xml version="1.0" encoding="ISO-8859-1"
?>
2
<!
DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"
>
3
4
<
hibernate-mapping
>
5
<
class
table
="t_group"
name
="com.test.model.Group"
>
6
<
id
access
="field"
name
="groupid"
>
7
<
generator
class
="native"
/>
8
</
id
>
9
<
property
name
="groupname"
access
="field"
/>
10
<
property
name
="remark"
access
="field"
/>
11
<
set
inverse
="true"
access
="field"
name
="users"
>
12
<
key
column
="groupid"
/>
13
<
one-to-many
class
="com.test.model.User"
/>
14
</
set
>
15
</
class
>
16
</
hibernate-mapping
>
User.hbm.xml

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

1
<?
xml version="1.0" encoding="ISO-8859-1"
?>
2
<!
DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"
>
3
4
<
hibernate-mapping
>
5
<
class
table
="t_user"
name
="com.test.model.User"
>
6
<
id
access
="field"
name
="userid"
>
7
<
generator
class
="native"
/>
8
</
id
>
9
<
property
name
="username"
access
="field"
/>
10
<
many-to-one
column
="groupid"
cascade
="all"
access
="field"
class
="com.test.model.Group"
name
="group"
/>
11
<
property
name
="password"
access
="field"
/>
12
<
property
name
="email"
access
="field"
/>
13
<
property
name
="question"
access
="field"
/>
14
<
property
name
="answer"
access
="field"
/>
15
<
property
name
="createtime"
access
="field"
/>
16
<
property
name
="status"
access
="field"
/>
17
</
class
>
18
</
hibernate-mapping
>
hibernate.cfg.xml

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

1
<?
xml version="1.0" encoding="ISO-8859-1"
?>
2
<!
DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"
>
3
4
<
hibernate-configuration
>
5
<
session-factory
>
6
<
property
name
="hibernate.connection.driver_class"
>
com.mysql.jdbc.Driver
</
property
>
7
<
property
name
="hibernate.connection.url"
>
jdbc:mysql://localhost/test
</
property
>
8
<
property
name
="hibernate.connection.username"
>
root
</
property
>
9
<
property
name
="hibernate.connection.password"
>
intrl
</
property
>
10
<
property
name
="hibernate.dialect"
>
org.hibernate.dialect.MySQLDialect
</
property
>
11
<
property
name
="hibernate.show_sql"
>
true
</
property
>
12
<
property
name
="hibernate.hbm2ddl.auto"
>
update
</
property
>
13
<
mapping
resource
="com/test/model/Group.hbm.xml"
/>
14
<
mapping
resource
="com/test/model/User.hbm.xml"
/>
15
</
session-factory
>
16
</
hibernate-configuration
>
以上代码语法请自行查找资料学习,在此不作详细介绍。

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

5、资源下载
xdoclet-plugins-dist-1.0.4-bin.zip: http://www.rayfile.com/files/f8462580-66f2-11de-8a67-0014221b798a/
apache-ant-1.7.1-bin.zip: http://www.rayfile.com/files/78e4fa6e-66b6-11de-8110-0014221b798a/