本文来自http://blog.csdn.net/hellogv/,引用必须注明出处!
上次介绍了Activity以及Intent的使用,这次就介绍Service,如果把Activity比喻为前台程序,那么Service就是后台程序,Service的整个生命周期都只会在后台执行。Service跟Activity一样也由Intent调用。在工程里想要添加一个Service,先新建继承Service的类,然后到AndroidManifest.xml -> Application ->Application Nodes中的Service标签中添加。
Service要由Activity通过startService 或者 bindService来启动,Intent负责传递参数。先贴出本文程序运行截图:
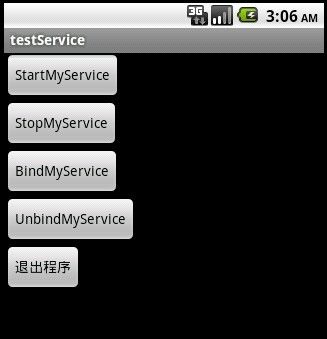
本文主要讲解Service的调用,以及其生命周期。
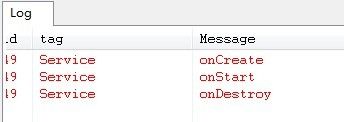
上图是startService之后再stopService的Service状态变化。
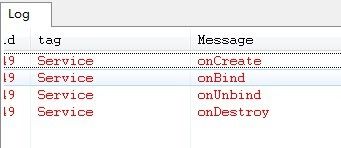
上图是bindService之后再unbindService的Service状态变化。
startService与bindService都可以启动Service,那么它们之间有什么区别呢?它们两者的区别就是使Service的周期改变。由startService启动的Service必须要有stopService来结束Service,不调用stopService则会造成Activity结束了而Service还运行着。bindService启动的Service可以由unbindService来结束,也可以在Activity结束之后(onDestroy)自动结束。
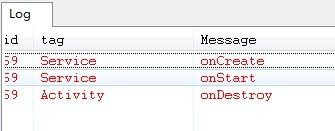
上图是startService之后再Activity.finish()的Service状态变化,Service还在跑着。
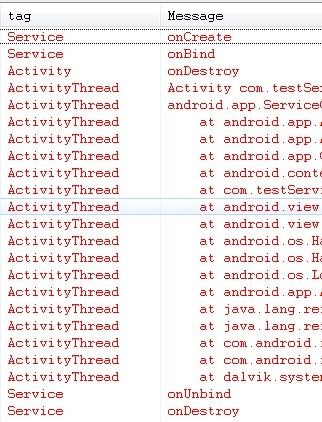
上图是bindService之后再Activity.finish()的Service状态变化,Service最后自动unbindService。
main.xml代码:
- <?xmlversion="1.0"encoding="utf-8"?>
- <LinearLayoutxmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <Buttonandroid:layout_width="wrap_content"
- android:layout_height="wrap_content"android:id="@+id/btnStartMyService"
- android:text="StartMyService"></Button>
- <Buttonandroid:layout_width="wrap_content"
- android:layout_height="wrap_content"android:id="@+id/btnStopMyService"
- android:text="StopMyService"></Button>
- <Buttonandroid:layout_width="wrap_content"
- android:layout_height="wrap_content"android:id="@+id/btnBindMyService"
- android:text="BindMyService"></Button>
- <Buttonandroid:layout_width="wrap_content"
- android:layout_height="wrap_content"android:id="@+id/btnUnbindMyService"
- android:text="UnbindMyService"></Button>
- <Buttonandroid:layout_width="wrap_content"
- android:layout_height="wrap_content"android:id="@+id/btnExit"
- android:text="退出程序"></Button>
- </LinearLayout>
testService.java的源码:
- packagecom.testService;
-
- importandroid.app.Activity;
- importandroid.app.Service;
- importandroid.content.ComponentName;
- importandroid.content.Intent;
- importandroid.content.ServiceConnection;
- importandroid.os.Bundle;
- importandroid.os.IBinder;
- importandroid.util.Log;
- importandroid.view.View;
- importandroid.widget.Button;
-
- publicclasstestServiceextendsActivity{
- ButtonbtnStartMyService,btnStopMyService,btnBindMyService,btnUnbindMyService,btnExit;
- @Override
- publicvoidonCreate(BundlesavedInstanceState){
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- btnStartMyService=(Button)this.findViewById(R.id.btnStartMyService);
- btnStartMyService.setOnClickListener(newClickEvent());
-
- btnStopMyService=(Button)this.findViewById(R.id.btnStopMyService);
- btnStopMyService.setOnClickListener(newClickEvent());
-
- btnBindMyService=(Button)this.findViewById(R.id.btnBindMyService);
- btnBindMyService.setOnClickListener(newClickEvent());
-
- btnUnbindMyService=(Button)this.findViewById(R.id.btnUnbindMyService);
- btnUnbindMyService.setOnClickListener(newClickEvent());
-
- btnExit=(Button)this.findViewById(R.id.btnExit);
- btnExit.setOnClickListener(newClickEvent());
- }
- @Override
- publicvoidonDestroy()
- {
- super.onDestroy();
- Log.e("Activity","onDestroy");
- }
-
- privateServiceConnection_connection=newServiceConnection(){
- @Override
- publicvoidonServiceConnected(ComponentNamearg0,IBinderarg1){
-
- }
-
- @Override
- publicvoidonServiceDisconnected(ComponentNamename){
-
- }
- };
- classClickEventimplementsView.OnClickListener{
-
- @Override
- publicvoidonClick(Viewv){
- Intentintent=newIntent(testService.this,MyService.class);
- if(v==btnStartMyService){
- testService.this.startService(intent);
- }
- elseif(v==btnStopMyService){
- testService.this.stopService(intent);
- }
- elseif(v==btnBindMyService){
- testService.this.bindService(intent,_connection,Service.BIND_AUTO_CREATE);
- }
- elseif(v==btnUnbindMyService){
- if(MyService.ServiceState=="onBind")
- testService.this.unbindService(_connection);
- }
- elseif(v==btnExit)
- {
- testService.this.finish();
- }
-
- }
-
- }
- }
MyService.java的源码:
- packagecom.testService;
-
- importandroid.app.Service;
- importandroid.content.Intent;
- importandroid.os.IBinder;
- importandroid.util.Log;
-
- publicclassMyServiceextendsService{
- staticpublicStringServiceState="";
- @Override
- publicIBinderonBind(Intentarg0){
- Log.e("Service","onBind");
- ServiceState="onBind";
- returnnull;
- }
- @Override
- publicbooleanonUnbind(Intentintent){
- super.onUnbind(intent);
- Log.e("Service","onUnbind");
- ServiceState="onUnbind";
- returnfalse;
-
- }
- @Override
- publicvoidonCreate(){
- super.onCreate();
- Log.e("Service","onCreate");
- ServiceState="onCreate";
- }
- @Override
- publicvoidonDestroy(){
- super.onDestroy();
- Log.e("Service","onDestroy");
- ServiceState="onDestroy";
- }
- @Override
- publicvoidonStart(Intentintent,intstartid){
- super.onStart(intent,startid);
- Log.e("Service","onStart");
- ServiceState="onStart";
- }
-
- }