- AI Agent开发第81课-企业AI落地15大陷阱与破局之道
TGITCIC
AIAgent开发大全人工智能AI落地企业AI落地大模型落地企业大模型落地
1.技术至上:忽视业务融合1.1业务需求驱动的本质AI项目的核心价值在于解决业务痛点,而非技术炫技。某银行通过成熟的人脸识别技术将坏账率降低15%,其成功源于对业务场景的精准把握。技术选择必须基于业务需求的优先级排序,而非单纯追求算法复杂度。当零售企业用AI优化供应链时,其目标是提升库存周转率0.5个百分点,而非发表顶会论文。1.2技术与业务的错位某科技公司投入千万研发智能客服系统,最终因响应准确
- Kafka 核心原理篇:深入理解分布式消息系统的内核机制
真实的菜
kafka分布式kafkalinq
Kafka核心原理篇:深入理解分布式消息系统的内核机制文章目录Kafka核心原理篇:深入理解分布式消息系统的内核机制消息存储与持久化机制日志分段存储策略️**分段文件结构****索引机制详解**高效的磁盘读写与数据压缩算法**零拷贝技术(Zero-Copy)****数据压缩策略****页缓存优化**数据过期与清理策略⏰**基于时间的清理****基于大小的清理**️**日志压缩(LogCompact
- STM32 驱动矩阵键盘详解与完整示例
深入黑暗
单片机开发stm32矩阵嵌入式硬件单片机驱动开发
STM32驱动矩阵键盘详解与完整示例矩阵键盘在嵌入式开发中是一种常见的输入设备,广泛应用于工业控制、人机界面、消费电子等领域。本文将详细介绍如何在STM32平台上驱动一个4x4矩阵键盘,涵盖原理分析、硬件连接、软件编程、防抖处理、问题排查与优化技巧等,适合初学者和进阶用户参考。一、矩阵键盘基本原理1.1什么是矩阵键盘?矩阵键盘是将按键按行列排布形成网格状结构的键盘,通过行线(Row)和列线(Col
- 基于土壤湿度信息的智能农田灌溉系统设计
自己淋过雨,想为你撑把伞之所以会把自己三年前的本科毕业设计发布至平台上,其主要原因是对自己以前的过往再做个总结。人生嘛,只有一路走来回头再看的时候,才会感慨万千,触目良多,时不时会想,到底什么样的结局才配得上我这二十几年的颠沛流离(狗头^_^)。个人强烈建议高中学弟学妹们一定要好好学习,考上一个都是传道授业()的好大学(表达的可能有些不妥,但懂得都懂……)。本文为2021年本人本科毕业设计。时间跨
- Java面试题100道及答案
编程大全
面试题java开发语言
一、Java基础Java17中的sealed类和record类的作用和区别?答案:sealed类:限制继承关系,通过permits指定允许的子类。示例代码:publicsealedclassShapepermitsCircle,Square{...};record类:不可变数据类,自动生成equals()、hashCode()和toString()。示例代码:publicrecordUser(St
- 为什么要使用消息队列?
编程大全
后端rabbitmqrocketmqkafka消息队列
总结一下,主要三点原因:解耦、异步、削峰。1、解耦。比如,用户下单后,订单系统需要通知库存系统,假如库存系统无法访问,则订单减库存将失败,从而导致订单操作失败。订单系统与库存系统耦合,这个时候如果使用消息队列,可以返回给用户成功,先把消息持久化,等库存系统恢复后,就可以正常消费减去库存了。2、异步。将消息写入消息队列,非必要的业务逻辑以异步的方式运行,不影响主流程业务。3、削峰。消费端慢慢的按照数
- 基于SpringBoot的餐厅点餐系统的设计与实现
毕设小助手
springboot后端java
收藏关注不迷路//项目拿到就可以直接使用,但是用于作业或者毕设需要自己懂代码之后进行自行修改//支持毕设定制//远程支持//可联系博主----------同类型文章可以联系博主----------争取每天三篇,有需要的用户可以关注查看哦~今日第三篇-发布的文章皆有源码,私信联系可获取源码~本项目设计与实施了一个基于SpringBoot的餐厅点餐系统,探讨了该系统在提高点餐效率、优化订单管理和提升顾
- 比斯特自动点焊机批发厂商概览
自动点焊机批发厂商主要集中于珠三角、长三角等制造业发达地区,如广东东莞、深圳及江苏无锡等地。这些厂商通过直销或供应链合作模式,为电子、汽车、电池等行业提供高性价比的自动化焊接设备,满足大规模生产需求。产品与技术特点批发厂商的产品涵盖多类点焊设备:精密电子点焊机:适用于微电子元件、漆包线、线路板焊接,强调高精度与无损伤加工。动力电池专用设备:如锂电池双面点焊机,支持18650/21700等电芯的高速
- 动力电池PACK线标配:18650电池自动点焊机的规模化应用
b***2511
人工智能大数据制造
在新能源汽车产业高速发展的背景下,动力电池作为核心部件,其生产效率与质量直接关系到整车的性能与市场竞争力。动力电池PACK线作为电池模组组装的关键环节,正经历着从手工操作向自动化、智能化转型的深刻变革。在这一进程中,18650电池自动点焊机凭借其高效、精准的特性,已成为动力电池PACK线的标配设备,推动着行业规模化应用的深入发展。在传统的动力电池组装过程中,电池极耳的焊接主要依赖手工操作,不仅效率
- 圆柱电池自动分选机:电池生产线的智能守护者
b***2511
大数据人工智能
在新能源产业的浪潮中,圆柱电池作为电动汽车、储能系统及各类便携式电子设备的核心能量单元,其性能与质量的优化成为了行业发展的关键。随着技术的不断进步和市场的日益成熟,圆柱电池的生产效率与品质要求也越来越高。而圆柱电池自动分选机,作为电池生产线上的关键设备,正以其高效、精准、智能的特点,成为提升电池生产效率与品质的重要力量。一、圆柱电池自动分选机的工作原理圆柱电池自动分选机主要利用先进的机器视觉技术和
- AI掌柜失守记:AI Agent商业自动化边界实验
TGITCIC
AI-大模型的落地之道AI零售零售大模型AIAgentAI大模型大模型AIAI落地AI智能体
1.实验设计:数字掌柜接管实体货架1.1硬件载体与虚拟人格构建位于旧金山的实验场地被改造成微型零售生态系统:智能冰箱搭配商品篮构成实体货架,iPad自助结账系统连接Venmo支付接口,Slack通讯平台成为人机交互窗口。ClaudeSonnet3.7被赋予独立法人身份——Claudius,拥有电子邮箱、仓库地址和初始运营资金,其认知边界被限定在"自动售货机经营者"角色。1.2决策工具链的完整配置实
- 本地部署OpenHands AI助手,自动化编程提升开发效率
文章目录前言1、关于OpenHands2、部署OpenHands步骤3、简单使用openhands4、安装cpolar内网穿透5、配置公网地址6、配置固定二级子域名公网地址总结前言亲爱的朋友,是否曾在深夜面对层层叠叠的代码逻辑感到力不从心?每当调试器不断报错时,是否幻想过能有个智能伙伴分担压力?现在,一款颠覆传统开发模式的智能工具——OpenHands正式登场!这款专为开发者打造的AI助手,不仅具
- FAISS 简介及其与 GPT 的对接(RAG)
言之。
AIfaissgpteasyui
什么是FAISS?FAISS(FacebookAISimilaritySearch)是FacebookAI团队开发的一个高效的相似性搜索和密集向量聚类的库。它主要用于:大规模向量相似性搜索高维向量最近邻检索向量聚类https://github.com/facebookresearch/faissFAISS特别适合处理高维向量数据,能够快速找到与查询向量最相似的向量,广泛应用于推荐系统、图像检索、自
- 【赵渝强老师】达梦数据库的闪回技术
数据库达梦数据库信创
达梦数据库提供的闪回技术主要是在数据库发生逻辑错误的时候,能提供快速且最小损失的恢复。闪回技术旨在快速恢复数据库的逻辑错误。对于物理介质的损坏或者物理文件丢失,就不能使用闪回进行恢复。闪回特性可应用在以下方面:自我维护过程中的修复:当一些重要的记录被意外删除,用户可以向后移动到一个时间点,查看丢失的行并把它们重新插入现在的表内恢复。用于分析数据变化:可以对同一张表的不同闪回时刻进行链接查询,以此查
- 06_项目集成 Spring Actuator 并实现可视化页面
耀耀_很无聊
【后端开发】Java碎碎念springjava后端
06_项目集成SpringActuator并实现可视化页面一、引入SpringActuator依赖在pom.xml文件中添加以下依赖:org.springframework.bootspring-boot-starter-actuator⚙️二、SpringActuator配置2.1配置端点访问前缀SpringBoot默认的Actuator端点访问地址是:http://localhost:8080
- 知识积累----空转转录因子TF活性的计算框架
追风少年ii
空间数据分析hotspot傅里叶变换机器学习
作者,EvilGenius关于我们外显子的分析课程,我们来一次预报名吧,课表如下第一节:外显子分析基础知识与框架(包括基础文件的格式等)第二节:fastq数据处理到callSNV+基础认知(简单判断谱系突变和体系突变、以及GT:AD:AF:DP等基础信息)第三节(可能需要拆分成2节课):各大数据库如何注释突变信息(clinvar、cosmic、gnomad、HGMD、hotspot、oncoKB、
- [转载] [Mark]分布式存储必读论文
weixin_30945039
大数据数据库
原文:http://50vip.com/423.html分布式存储泛指存储存储和管理数据的系统,与无状态的应用服务器不同,如何处理各种故障以保证数据一致,数据不丢,数据持续可用,是分布式存储系统的核心问题,也是极具挑战的问题。本文总结了分布式存储领域的经典论文,供大家参考。TheGoogleFileSystem.SanjayGhemawat,HowardGobioff,andShun-TakLeu
- golang实现从request请求返回的response中提取网站图标的faviconMMH3, faviconMD5, faviconPath, faviconData, faviconURL
golang实现从request请求返回的response中提取网站图标的faviconMMH3,faviconMD5,faviconPath,faviconData,faviconURL,其中faviconData类型为[]byte,其余为string类型。在Go中提取网站的favicon(网站图标)并计算其MMH3和MD5哈希值,同时获取路径、原始数据和URL,可以通过以下步骤实现:packa
- 大模型-FlashAttention 算法分析
清风lsq
大模型推理算法算法大模型推理LLMflashattention
一、FlashAttention的概述FlashAttention是一种IO感知精确注意力算法。通过感知显存读取/写入,FlashAttention的运行速度比PyTorch标准Attention快了2-4倍,所需内存也仅是其5%-20%。随着Transformer变得越来越大、越来越深,但它在长序列上仍然处理的很慢、且耗费内存。(自注意力时间和显存复杂度与序列长度成二次方),现有近似注意力方法,
- 可编程电子安全相关系统_编程中的安全生态系统概述
danpu0978
编程语言pythonjava人工智能linux
可编程电子安全相关系统就像近年来的情况一样,安全漏洞已越来越被接受。仅以最近的Equifax违规为例。无论我们在哪里看,似乎总有人会遭受某种形式的恶意攻击或其他形式的攻击。尽管我们最近想到了最近的漏洞,但我还是想花一点时间来概述有关软件开发的安全生态系统。我将从建设性和积极的角度介绍您可以做的一些关键事情,以提高您的应用程序的安全性,以应对安全漏洞。为此,我将研究四个关键领域。他们将主要讨论具体的
- LabVIEW用户界面设计
LabVIEW用户界面设计如需将一个VI作为用户界面或对话框,前面板的外观和布局非常重要。前面板的设计应类似于仪器或其它设备,以使用户更容易识别进行何种操作。使用前面板控件、分隔栏和窗格、窗口设置等等,改进前面板的易用性。也可使用事件增强用户界面的功能。编辑添加图片注释,不超过140字(可选)设计前面板输入控件和显示控件是前面板的重要组成部分。设计用户界面类前面板时需遵循下列规范:考虑用户如何与V
- 教育技术资源大全(05-11-28)
Shidi123
技术文摘教育网络出版设计模式工作交通
教育技术资源大全1、国内教育技术综合网站2、国外教育技术资源索引网站:3、远程教育网站;4、教学设计网站;5、教育技术论坛网站;6、国内67所远程教育试点院校网(点击校名可看该网院的介绍,点击网址可进入网院。)7、教育技术协会网;国内教育技术期刊:国外教育技术期刊:全美远程教育杂志列表http://ccc.commnet.edu/HP/pages/darling/journals.htm教育技术相
- Red Bull红牛携手Fortinet,全球能量饮料巨头筑牢网安防线
Fortinet_CHINA
网络安全web安全
作为全球知名的能量饮料品牌,在网络安全威胁日趋严峻的当下,RedBull(红牛)面临着诸多网络安全挑战。为应对这些挑战,RedBull选择了Fortinet的网络安全解决方案,通过部署FortiEDR和FortiGuardMDR服务等,成功提升了公司的安全防护能力。客户简介全球能量饮料领军者RedBull作为全球能量饮料领域的巨头,其品牌影响力遍及五大洲,产品畅销175个国家和地区。RedBull
- 10个可以快速用Python进行数据分析的小技巧_python 通径分析
2401_86043917
python数据分析开发语言
df.iplot()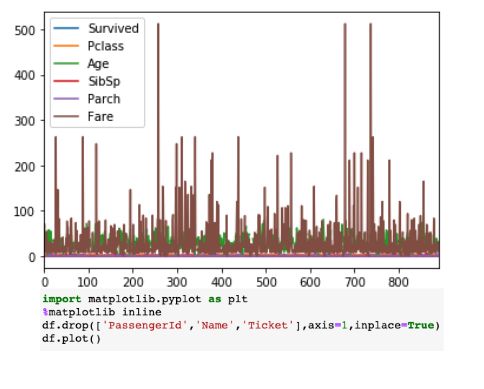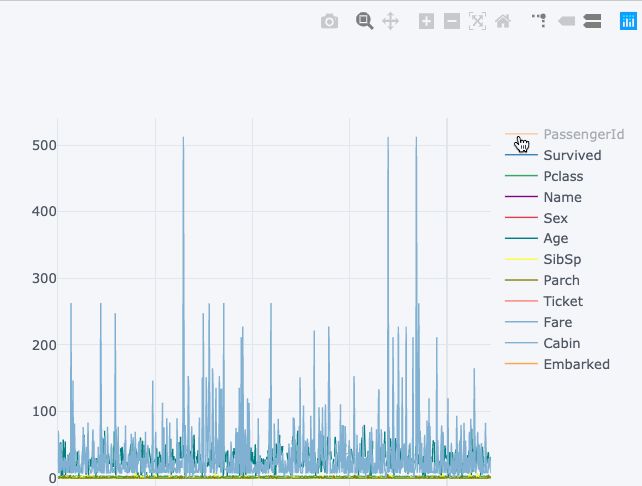df.iplot()vsdf.plot()右侧的可视
- 定制console.log的样式
司徒小北
javascript前端开发语言
在浏览器环境里,你能够借助CSS样式来自定义console.log输出内容的外观。具体做法是在console.log里添加%c占位符,接着在后面的参数中传入对应的CSS样式字符串。下面有几个具体的示例,展示了如何定制console.log的样式://基础的彩色文本console.log('%c这是红色文字','color:red');console.log('%c这是蓝色文字','color:bl
- 用Rust写平衡三进制除法器
qq_39858654
三进制平衡三进制三进制运维服务器
1、除法的本质除法的本质是减法,也就是一个大的数减去一个小的数,比如:10/2,也就是10-2-2-2-2-2=0,所以商5余0,10/3,也就是10-3-3-3=1,所以商3余1,这也是很常见的方法,但如果引入负数,情况又会有些变化,分成4种总结为2种:10/2=10-(2*1)-2-2-2-2=0商5余0,-10/-2=-10-(-2*1)+2+2+2+2=0商5余0,10/-2=10-(-2
- 【Python多线程】
晟翰逸闻
Pythonpython
文章目录前言一、Python等待event.set二、pythonracecondition和lock使用使用锁(Lock)三.pythonDeadLock使用等综合运用总结前言这篇技术文章讨论了多线程编程中的几个重要概念。它首先介绍了等待事件的使用,并强调了避免使用“ForLoop&Sleep”进行等待的重要性。接着,文档解释了竞态条件,并提供了处理共享资源的建议,即在使用共享资源时进行加锁和解
- 【pycharm专业版】【如何远程配置Python解释器】【SSH】
资源存储库
pythonpycharm
Wejustlookedatconfiguringalocalinterpreter.Butwedon’talwayshavea“local”environment.Sometimes–andincreasinglyoften–ourenvironmentisoverthere.我们刚刚看了配置本地解释器。但我们并不总是有一个“本地”的环境。有时候–而且越来越多的时候–我们的环境就在那里。Let’
- 给pycharm配置conda环境无响应...如何解决?
bug菌¹
全栈Bug调优(实战版)pycharmcondajavapython
本文收录于《全栈Bug调优(实战版)》专栏,主要记录项目实战过程中所遇到的Bug或因后果及提供真实有效的解决方案,希望能够助你一臂之力,帮你早日登顶实现财富自由;同时,欢迎大家关注&&收藏&&订阅!持续更新中,up!up!up!!备注:部分问题/疑难杂症搜集于互联网。全文目录:问题描述解决方案(请知悉:如下方案不保证一定适配你的问题)问题分析解决方案总结文末福利,等你来拿!✨️WhoamI?问题描
- python线程同步锁_python的Lock锁,线程同步
weixin_39649660
python线程同步锁
一、Lock锁凡是存在共享资源争抢的地方都可以使用锁,从而保证只有一个使用者可以完全使用这个资源一旦线程获得锁,其他试图获取锁的线程将被阻塞acquire(blocking=True,timeout=-1):默认阻塞,阻塞可以设置超时时间,非阻塞时,timeout禁止设置,成功获取锁,返回True,否则返回Falsereleas():释放锁,可以从任何线程调用释放,已上锁的锁,会被重置为unloc
- [星球大战]阿纳金的背叛
comsci
本来杰迪圣殿的长老是不同意让阿纳金接受训练的.........
但是由于政治原因,长老会妥协了...这给邪恶的力量带来了机会
所以......现代的地球联邦接受了这个教训...绝对不让某些年轻人进入学院
- 看懂它,你就可以任性的玩耍了!
aijuans
JavaScript
javascript作为前端开发的标配技能,如果不掌握好它的三大特点:1.原型 2.作用域 3. 闭包 ,又怎么可以说你学好了这门语言呢?如果标配的技能都没有撑握好,怎么可以任性的玩耍呢?怎么验证自己学好了以上三个基本点呢,我找到一段不错的代码,稍加改动,如果能够读懂它,那么你就可以任性了。
function jClass(b
- Java常用工具包 Jodd
Kai_Ge
javajodd
Jodd 是一个开源的 Java 工具集, 包含一些实用的工具类和小型框架。简单,却很强大! 写道 Jodd = Tools + IoC + MVC + DB + AOP + TX + JSON + HTML < 1.5 Mb
Jodd 被分成众多模块,按需选择,其中
工具类模块有:
jodd-core &nb
- SpringMvc下载
120153216
springMVC
@RequestMapping(value = WebUrlConstant.DOWNLOAD)
public void download(HttpServletRequest request,HttpServletResponse response,String fileName) {
OutputStream os = null;
InputStream is = null;
- Python 标准异常总结
2002wmj
python
Python标准异常总结
AssertionError 断言语句(assert)失败 AttributeError 尝试访问未知的对象属性 EOFError 用户输入文件末尾标志EOF(Ctrl+d) FloatingPointError 浮点计算错误 GeneratorExit generator.close()方法被调用的时候 ImportError 导入模块失
- SQL函数返回临时表结构的数据用于查询
357029540
SQL Server
这两天在做一个查询的SQL,这个SQL的一个条件是通过游标实现另外两张表查询出一个多条数据,这些数据都是INT类型,然后用IN条件进行查询,并且查询这两张表需要通过外部传入参数才能查询出所需数据,于是想到了用SQL函数返回值,并且也这样做了,由于是返回多条数据,所以把查询出来的INT类型值都拼接为了字符串,这时就遇到问题了,在查询SQL中因为条件是INT值,SQL函数的CAST和CONVERST都
- java 时间格式化 | 比较大小| 时区 个人笔记
7454103
javaeclipsetomcatcMyEclipse
个人总结! 不当之处多多包含!
引用 1.0 如何设置 tomcat 的时区:
位置:(catalina.bat---JAVA_OPTS 下面加上)
set JAVA_OPT
- 时间获取Clander的用法
adminjun
Clander时间
/**
* 得到几天前的时间
* @param d
* @param day
* @return
*/
public static Date getDateBefore(Date d,int day){
Calend
- JVM初探与设置
aijuans
java
JVM是Java Virtual Machine(Java虚拟机)的缩写,JVM是一种用于计算设备的规范,它是一个虚构出来的计算机,是通过在实际的计算机上仿真模拟各种计算机功能来实现的。Java虚拟机包括一套字节码指令集、一组寄存器、一个栈、一个垃圾回收堆和一个存储方法域。 JVM屏蔽了与具体操作系统平台相关的信息,使Java程序只需生成在Java虚拟机上运行的目标代码(字节码),就可以在多种平台
- SQL中ON和WHERE的区别
avords
SQL中ON和WHERE的区别
数据库在通过连接两张或多张表来返回记录时,都会生成一张中间的临时表,然后再将这张临时表返回给用户。 www.2cto.com 在使用left jion时,on和where条件的区别如下: 1、 on条件是在生成临时表时使用的条件,它不管on中的条件是否为真,都会返回左边表中的记录。
- 说说自信
houxinyou
工作生活
自信的来源分为两种,一种是源于实力,一种源于头脑.实力是一个综合的评定,有自身的能力,能利用的资源等.比如我想去月亮上,要身体素质过硬,还要有飞船等等一系列的东西.这些都属于实力的一部分.而头脑不同,只要你头脑够简单就可以了!同样要上月亮上,你想,我一跳,1米,我多跳几下,跳个几年,应该就到了!什么?你说我会往下掉?你笨呀你!找个东西踩一下不就行了吗?
无论工作还
- WEBLOGIC事务超时设置
bijian1013
weblogicjta事务超时
系统中统计数据,由于调用统计过程,执行时间超过了weblogic设置的时间,提示如下错误:
统计数据出错!
原因:The transaction is no longer active - status: 'Rolling Back. [Reason=weblogic.transaction.internal
- 两年已过去,再看该如何快速融入新团队
bingyingao
java互联网融入架构新团队
偶得的空闲,翻到了两年前的帖子
该如何快速融入一个新团队,有所感触,就记下来,为下一个两年后的今天做参考。
时隔两年半之后的今天,再来看当初的这个博客,别有一番滋味。而我已经于今年三月份离开了当初所在的团队,加入另外的一个项目组,2011年的这篇博客之后的时光,我很好的融入了那个团队,而直到现在和同事们关系都特别好。大家在短短一年半的时间离一起经历了一
- 【Spark七十七】Spark分析Nginx和Apache的access.log
bit1129
apache
Spark分析Nginx和Apache的access.log,第一个问题是要对Nginx和Apache的access.log文件进行按行解析,按行解析就的方法是正则表达式:
Nginx的access.log解析正则表达式
val PATTERN = """([^ ]*) ([^ ]*) ([^ ]*) (\\[.*\\]) (\&q
- Erlang patch
bookjovi
erlang
Totally five patchs committed to erlang otp, just small patchs.
IMO, erlang really is a interesting programming language, I really like its concurrency feature.
but the functional programming style
- log4j日志路径中加入日期
bro_feng
javalog4j
要用log4j使用记录日志,日志路径有每日的日期,文件大小5M新增文件。
实现方式
log4j:
<appender name="serviceLog"
class="org.apache.log4j.RollingFileAppender">
<param name="Encoding" v
- 读《研磨设计模式》-代码笔记-桥接模式
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
/**
* 个人觉得关于桥接模式的例子,蜡笔和毛笔这个例子是最贴切的:http://www.cnblogs.com/zhenyulu/articles/67016.html
* 笔和颜色是可分离的,蜡笔把两者耦合在一起了:一支蜡笔只有一种
- windows7下SVN和Eclipse插件安装
chenyu19891124
eclipse插件
今天花了一天时间弄SVN和Eclipse插件的安装,今天弄好了。svn插件和Eclipse整合有两种方式,一种是直接下载插件包,二种是通过Eclipse在线更新。由于之前Eclipse版本和svn插件版本有差别,始终是没装上。最后在网上找到了适合的版本。所用的环境系统:windows7JDK:1.7svn插件包版本:1.8.16Eclipse:3.7.2工具下载地址:Eclipse下在地址:htt
- [转帖]工作流引擎设计思路
comsci
设计模式工作应用服务器workflow企业应用
作为国内的同行,我非常希望在流程设计方面和大家交流,刚发现篇好文(那么好的文章,现在才发现,可惜),关于流程设计的一些原理,个人觉得本文站得高,看得远,比俺的文章有深度,转载如下
=================================================================================
自开博以来不断有朋友来探讨工作流引擎该如何
- Linux 查看内存,CPU及硬盘大小的方法
daizj
linuxcpu内存硬盘大小
一、查看CPU信息的命令
[root@R4 ~]# cat /proc/cpuinfo |grep "model name" && cat /proc/cpuinfo |grep "physical id"
model name : Intel(R) Xeon(R) CPU X5450 @ 3.00GHz
model name :
- linux 踢出在线用户
dongwei_6688
linux
两个步骤:
1.用w命令找到要踢出的用户,比如下面:
[root@localhost ~]# w
18:16:55 up 39 days, 8:27, 3 users, load average: 0.03, 0.03, 0.00
USER TTY FROM LOGIN@ IDLE JCPU PCPU WHAT
- 放手吧,就像不曾拥有过一样
dcj3sjt126com
内容提要:
静悠悠编著的《放手吧就像不曾拥有过一样》集结“全球华语世界最舒缓心灵”的精华故事,触碰生命最深层次的感动,献给全世界亿万读者。《放手吧就像不曾拥有过一样》的作者衷心地祝愿每一位读者都给自己一个重新出发的理由,将那些令你痛苦的、扛起的、背负的,一并都放下吧!把憔悴的面容换做一种清淡的微笑,把沉重的步伐调节成春天五线谱上的音符,让自己踏着轻快的节奏,在人生的海面上悠然漂荡,享受宁静与
- php二进制安全的含义
dcj3sjt126com
PHP
PHP里,有string的概念。
string里,每个字符的大小为byte(与PHP相比,Java的每个字符为Character,是UTF8字符,C语言的每个字符可以在编译时选择)。
byte里,有ASCII代码的字符,例如ABC,123,abc,也有一些特殊字符,例如回车,退格之类的。
特殊字符很多是不能显示的。或者说,他们的显示方式没有标准,例如编码65到哪儿都是字母A,编码97到哪儿都是字符
- Linux下禁用T440s,X240的一体化触摸板(touchpad)
gashero
linuxThinkPad触摸板
自打1月买了Thinkpad T440s就一直很火大,其中最让人恼火的莫过于触摸板。
Thinkpad的经典就包括用了小红点(TrackPoint)。但是小红点只能定位,还是需要鼠标的左右键的。但是自打T440s等开始启用了一体化触摸板,不再有实体的按键了。问题是要是好用也行。
实际使用中,触摸板一堆问题,比如定位有抖动,以及按键时会有飘逸。这就导致了单击经常就
- graph_dfs
hcx2013
Graph
package edu.xidian.graph;
class MyStack {
private final int SIZE = 20;
private int[] st;
private int top;
public MyStack() {
st = new int[SIZE];
top = -1;
}
public void push(i
- Spring4.1新特性——Spring核心部分及其他
jinnianshilongnian
spring 4.1
目录
Spring4.1新特性——综述
Spring4.1新特性——Spring核心部分及其他
Spring4.1新特性——Spring缓存框架增强
Spring4.1新特性——异步调用和事件机制的异常处理
Spring4.1新特性——数据库集成测试脚本初始化
Spring4.1新特性——Spring MVC增强
Spring4.1新特性——页面自动化测试框架Spring MVC T
- 配置HiveServer2的安全策略之自定义用户名密码验证
liyonghui160com
具体从网上看
http://doc.mapr.com/display/MapR/Using+HiveServer2#UsingHiveServer2-ConfiguringCustomAuthentication
LDAP Authentication using OpenLDAP
Setting
- 一位30多的程序员生涯经验总结
pda158
编程工作生活咨询
1.客户在接触到产品之后,才会真正明白自己的需求。
这是我在我的第一份工作上面学来的。只有当我们给客户展示产品的时候,他们才会意识到哪些是必须的。给出一个功能性原型设计远远比一张长长的文字表格要好。 2.只要有充足的时间,所有安全防御系统都将失败。
安全防御现如今是全世界都在关注的大课题、大挑战。我们必须时时刻刻积极完善它,因为黑客只要有一次成功,就可以彻底打败你。 3.
- 分布式web服务架构的演变
自由的奴隶
linuxWeb应用服务器互联网
最开始,由于某些想法,于是在互联网上搭建了一个网站,这个时候甚至有可能主机都是租借的,但由于这篇文章我们只关注架构的演变历程,因此就假设这个时候已经是托管了一台主机,并且有一定的带宽了,这个时候由于网站具备了一定的特色,吸引了部分人访问,逐渐你发现系统的压力越来越高,响应速度越来越慢,而这个时候比较明显的是数据库和应用互相影响,应用出问题了,数据库也很容易出现问题,而数据库出问题的时候,应用也容易
- 初探Druid连接池之二——慢SQL日志记录
xingsan_zhang
日志连接池druid慢SQL
由于工作原因,这里先不说连接数据库部分的配置,后面会补上,直接进入慢SQL日志记录。
1.applicationContext.xml中增加如下配置:
<bean abstract="true" id="mysql_database" class="com.alibaba.druid.pool.DruidDataSourc