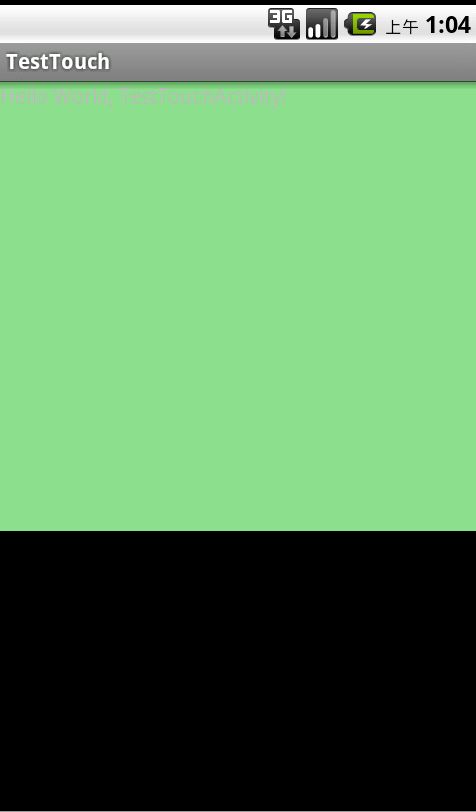
package com.domo.touch;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnTouchListener;
import android.widget.TextView;
public class TestTouchActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView textView = (TextView) findViewById(R.id.textview);
textView.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
// TODO Auto-generated method stub
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "TextView: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "TextView : ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
return false;//注意:return false
}
});
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
// TODO Auto-generated method stub
switch (ev.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "Activity_dis: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "Activity_dis: ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
Log.v("TAG", "Activity_dis: " + super.dispatchTouchEvent(ev));
return super.dispatchTouchEvent(ev);//注意
}
}
代码图如上:
当dispatchTouchEvent的返回值为super.dispatchTouchEvent(ev)时,获取到super.dispatchTouchEvent(ev)为False;点击Textview的显示的结果为
04-28 01:09:32.978: V/TAG(5733): Activity_dis: ACTION_DOWN0
04-28 01:09:32.978: V/TAG(5733): TextView: ACTION_DOWN0
04-28 01:09:32.978: V/TAG(5733): Activity_dis: false
04-28 01:09:32.978: V/TAG(5733): TextView: ACTION_DOWN0 //TextView只能获取到Down事件
04-28 01:09:33.068: V/TAG(5733): Activity_dis: false
04-28 01:09:33.088: V/TAG(5733): Activity_dis: ACTION_UP1
04-28 01:09:33.088: V/TAG(5733): Activity_dis: false
当
dispatchTouchEvent的返回值为
false时;点击Textview的显示的结果为
public boolean dispatchTouchEvent(MotionEvent ev) {
// TODO Auto-generated method stub
switch (ev.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "Activity_dis: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "Activity_dis: ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
Log.v("TAG", "Activity_dis: " + super.dispatchTouchEvent(ev));
return false; //即这里改为False时
}
1 04-28 01:14:25.467: V/TAG(5884): Activity_dis: ACTION_DOWN0
2 04-28 01:14:25.467: V/TAG(5884): TextView: ACTION_DOWN0 //只能获取到一次Down事件
3 04-28 01:14:25.467: V/TAG(5884): Activity_dis: false
4 04-28 01:14:25.571: V/TAG(5884): Activity_dis: ACTION_UP1
5 04-28 01:14:25.571: V/TAG(5884): Activity_dis: false
当
dispatchTouchEvent的返回值为
true时;点击Textview的显示的结果为:
1 04-28 01:17:00.398: V/TAG(5995): Activity_dis: ACTION_DOWN0
2 04-28 01:17:00.398: V/TAG(5995): TextView: ACTION_DOWN0 // 效果没见发生变化
3 04-28 01:17:00.398: V/TAG(5995): Activity_dis: false
4 04-28 01:17:00.418: V/TAG(5995): Activity_dis: ACTION_UP1
5 04-28 01:17:00.418: V/TAG(5995): Activity_dis: false
下面在
Activity中添加
onThouchEvent事件详细代码见下面
package com.domo.touch;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnTouchListener;
import android.widget.TextView;
public class TestTouchActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
TextView textView = (TextView) findViewById(R.id.textview);
textView.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
// TODO Auto-generated method stub
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "TextView: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "TextView : ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
return false;
}
});
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
// TODO Auto-generated method stub
switch (ev.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "Activity_dis: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "Activity_dis: ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
Log.v("TAG", "Activity_dis: " + super.dispatchTouchEvent(ev));
return true;//注意
}
@Override
public boolean onTouchEvent(MotionEvent event) {//在Activity中添加了onTouchEvent事件
// TODO Auto-generated method stub
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "onTouchEvent: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "onTouchEvent: ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
Log.v("TAG", "onTouchEvent: " + super.onTouchEvent(event));
return super.onTouchEvent(event);//注意
}
}
点击Textview结果:
04-28 01:22:11.957: V/TAG(6152): Activity_dis: ACTION_DOWN0
04-28 01:22:11.957: V/TAG(6152): TextView: ACTION_DOWN0 //还是只有一次
04-28 01:22:11.957: V/TAG(6152): onTouchEvent: ACTION_DOWN0
04-28 01:22:11.957: V/TAG(6152): onTouchEvent: false
04-28 01:22:11.957: V/TAG(6152): Activity_dis: false
04-28 01:22:12.047: V/TAG(6152): Activity_dis: ACTION_UP1
04-28 01:22:12.047: V/TAG(6152): onTouchEvent: ACTION_UP1
04-28 01:22:12.058: V/TAG(6152): onTouchEvent: false
04-28 01:22:12.058: V/TAG(6152): Activity_dis: false
把return
super.onTouchEvent(event);改为
return false;
点击Textview结果:
04-28 01:25:17.378: V/TAG(6152): Activity_dis: ACTION_DOWN0
04-28 01:25:17.378: V/TAG(6152): TextView: ACTION_DOWN0 //依然只有一次
04-28 01:25:17.378: V/TAG(6152): onTouchEvent: ACTION_DOWN0
04-28 01:25:17.378: V/TAG(6152): onTouchEvent: false
04-28 01:25:17.378: V/TAG(6152): Activity_dis: false
04-28 01:25:17.468: V/TAG(6152): Activity_dis: ACTION_UP1
04-28 01:25:17.468: V/TAG(6152): onTouchEvent: ACTION_UP1
04-28 01:25:17.468: V/TAG(6152): onTouchEvent: false
04-28 01:25:17.468: V/TAG(6152): Activity_dis: false
再把public boolean dispatchTouchEvent(MotionEvent ev) { 也返回 return false;
04-28 01:27:16.178: V/TAG(6304): Activity_dis: ACTION_DOWN0
04-28 01:27:16.178: V/TAG(6304): TextView: ACTION_DOWN0 //依然只有一次
04-28 01:27:16.178: V/TAG(6304): onTouchEvent: ACTION_DOWN0
04-28 01:27:16.178: V/TAG(6304): onTouchEvent: false
04-28 01:27:16.178: V/TAG(6304): Activity_dis: false
04-28 01:27:16.298: V/TAG(6304): Activity_dis: ACTION_UP1
04-28 01:27:16.298: V/TAG(6304): onTouchEvent: ACTION_UP1
04-28 01:27:16.298: V/TAG(6304): onTouchEvent: false
04-28 01:27:16.298: V/TAG(6304): Activity_dis: false
textView.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent event) {
// TODO Auto-generated method stub
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.v("TAG", "TextView: ACTION_DOWN" + MotionEvent.ACTION_DOWN);
break;
case MotionEvent.ACTION_UP:
Log.v("TAG", "TextView : ACTION_UP" + MotionEvent.ACTION_UP);
break;
default:
break;
}
return true; //注意
把这里改成true
Textview才能监听到 TextView: ACTION_DOWN0及TextView : ACTION_UP1的动作。
04-28 01:36:26.057: V/TAG(6628): Activity_dis: ACTION_DOWN0
04-28 01:36:26.057: V/TAG(6628): TextView: ACTION_DOWN0
04-28 01:36:26.057: V/TAG(6628): Activity_dis: true
04-28 01:36:26.148: V/TAG(6628): Activity_dis: ACTION_UP1
04-28 01:36:26.148: V/TAG(6628): TextView : ACTION_UP1//监听成功
04-28 01:36:26.148: V/TAG(6628): Activity_dis: true