Abstract Factory Pattern, UML diagram, Java Example
- 定义
- 类图
- 参与者
- 例子: Financial Tools Factory
- 例子: Class Diagram
- 例子: Java sample code
- 好处
- 使用
Definition
提供一个接口为生成相类似或依赖对象而没指定它们的具体实现类
Class Diagram
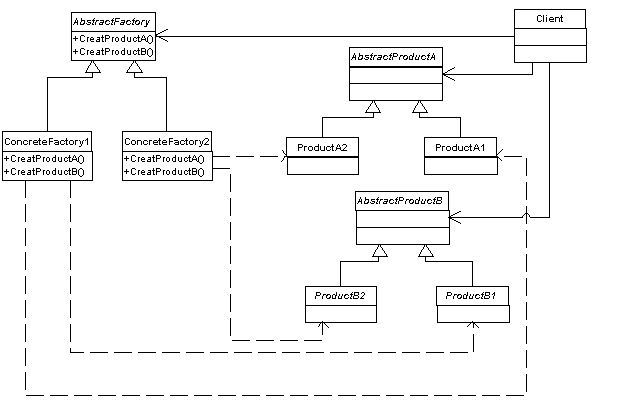
参与者
- 抽象工厂 (FinancialToolsFactory)
- 具体工厂 (EuropeFinancialToolsFactory, CanadaFinancialToolsFactory)
- 抽象产品 (TaxProcessor, ShipFeeProcessor)
- declares an interface for a type of product object
- 具体产品 (EuropeTaxProcessor, CanadaTaxProcessor, EuropeShipFeeProcessor, CanadaShipFeeProcessor)
- defines a product object to be created by the corresponding concrete factory implements the AbstractProduct interface
- 使用者(OrderProcessor)
- uses interfaces declared by AbstractFactory and AbstractProduct classes
Example: Financial Tools Factory
Example: Class Diagram
Example: Java sample code
// Factories
package com.apwebco.patterns.gof.abstractfactory;
public abstract class FinancialToolsFactory {
public abstract TaxProcessor createTaxProcessor();
public abstract ShipFeeProcessor createShipFeeProcessor();
}
public class CanadaFinancialToolsFactory extends FinancialToolsFactory {
public TaxProcessor createTaxProcessor() {
return new CanadaTaxProcessor();
}
public ShipFeeProcessor createShipFeeProcessor() {
return new CanadaShipFeeProcessor();
}
}
public class EuropeFinancialToolsFactory extends FinancialToolsFactory {
public TaxProcessor createTaxProcessor() {
return new EuropeTaxProcessor();
}
public ShipFeeProcessor createShipFeeProcessor() {
return new EuropeShipFeeProcessor();
}
}
// Products
public abstract class ShipFeeProcessor {
abstract void calculateShipFee(Order order);
}
public abstract class TaxProcessor {
abstract void calculateTaxes(Order order);
}
public class EuropeShipFeeProcessor extends ShipFeeProcessor {
public void calculateShipFee(Order order) {
// insert here Europe specific ship fee calculation
}
}
public class CanadaShipFeeProcessor extends ShipFeeProcessor {
public void calculateShipFee(Order order) {
// insert here Canada specific ship fee calculation
}
}
public class EuropeTaxProcessor extends TaxProcessor {
public void calculateTaxes(Order order) {
// insert here Europe specific taxt calculation
}
}
public class CanadaTaxProcessor extends TaxProcessor {
public void calculateTaxes(Order order) {
// insert here Canada specific taxt calculation
}
}
// Client
public class OrderProcessor {
private TaxProcessor taxProcessor;
private ShipFeeProcessor shipFeeProcessor;
public OrderProcessor(FinancialToolsFactory factory) {
taxProcessor = factory.createTaxProcessor();
shipFeeProcessor = factory.createShipFeeProcessor();
}
public void processOrder (Order order) {
// ....
taxProcessor.calculateTaxes(order);
shipFeeProcessor.calculateShipFee(order);
// ....
}
}
// Integration with the overall application
public class Application {
public static void main(String[] args) {
// .....
String countryCode = "EU";
Customer customer = new Customer();
Order order = new Order();
OrderProcessor orderProcessor = null;
FinancialToolsFactory factory = null;
if (countryCode == "EU") {
factory = new EuropeFinancialToolsFactory();
} else if (countryCode == "CA") {
factory = new CanadaFinancialToolsFactory();
}
orderProcessor = new OrderProcessor(factory);
orderProcessor.processOrder(order);
}
}
好处
- 隔离具体实现类
- 允许并容易改变同一系列的产品
- 促进产品的一致性
用法
- When the system needs to be independent of how its products are created composed and represented.
- When the system needs to be configured with one of multiple families of products.
- When a family of products need to be used together and this constraint needs to be enforced.
- When you need to provide a library of products, expose their interfaces not the implementation.