Android的编辑框控件EditText在平常编程时会经常用到,有时候会对编辑框增加某些限制,如限制只能输入数字,最大输入的文字个数,不能输入一些非法字符等,这些需求有些可以使用android控件属性直接写在布局xml文件里,比如android:numeric="integer"(只允许输入数字);
对于一些需求,如非法字符限制(例如不允许输入#号,如果输入了#给出错误提示),做成动态判断更方便一些,而且容易扩展;
在Android里使用TextWatcher接口可以很方便的对EditText进行监听;TextWatcher中有3个函数需要重载:
public void beforeTextChanged(CharSequence s, int start,
int count, int after);
public void onTextChanged(CharSequence s, int start, int before, int count);
public void afterTextChanged(Editable s);
从函数名就可以知道其意思,每当敲击键盘编辑框的文字改变时,上面的三个函数都会执行,beforeTextChanged可以给出变化之前的内容,onTextChanged和afterTextChanged给出追加上新的字符之后的文本;
所以对字符的限制判断可以在afterTextChanged函数中进行,如果检查到新追加的字符为认定的非法字符,则在这里将其delete掉,那么他就不会显示在编辑框里了:
private final TextWatcher mTextWatcher = new TextWatcher() {
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
public void onTextChanged(CharSequence s, int start, int before, int count) {
}
public void afterTextChanged(Editable s) {
if (s.length() > 0) {
int pos = s.length() - 1;
char c = s.charAt(pos);
if (c == '#') {
//这里限制在字串最后追加#
s.delete(pos,pos+1);
Toast.makeText(MyActivity.this, "Error letter.",Toast.LENGTH_SHORT).show();
}
}
}
};
注册监听:
EditText mEditor = (EditText)findViewById(R.id.editor_input);
mEditor.addTextChangedListener(mTextWatcher);
android 中如何限制 EditText 最大输入字符数:
方法一:
在 xml 文件中设置文本编辑框属性作字符数限制
如:android:maxLength="10" 即限制最大输入字符个数为10
方法二:
在代码中使用InputFilter 进行过滤
//editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)}); 即限定最大输入字符数为20
[java] view plain copy print ?
- public class TextEditActivity extends Activity {
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
-
- EditText editText = (EditText)findViewById(R.id.entry);
- editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
- }
- }
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText)findViewById(R.id.entry);
editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
}
}
方法三:
利用 TextWatcher 进行监听
[java] view plain copy print ?
- package cie.textEdit;
-
- import android.text.Editable;
- import android.text.Selection;
- import android.text.TextWatcher;
- import android.widget.EditText;
-
-
-
-
- public class MaxLengthWatcher implements TextWatcher {
-
- private int maxLen = 0;
- private EditText editText = null;
-
-
- public MaxLengthWatcher(int maxLen, EditText editText) {
- this.maxLen = maxLen;
- this.editText = editText;
- }
-
- public void afterTextChanged(Editable arg0) {
-
-
- }
-
- public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
- int arg3) {
-
-
- }
-
- public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
-
- Editable editable = editText.getText();
- int len = editable.length();
-
- if(len > maxLen)
- {
- int selEndIndex = Selection.getSelectionEnd(editable);
- String str = editable.toString();
-
- String newStr = str.substring(0,maxLen);
- editText.setText(newStr);
- editable = editText.getText();
-
-
- int newLen = editable.length();
-
- if(selEndIndex > newLen)
- {
- selEndIndex = editable.length();
- }
-
- Selection.setSelection(editable, selEndIndex);
-
- }
- }
-
- }
package cie.textEdit;
import android.text.Editable;
import android.text.Selection;
import android.text.TextWatcher;
import android.widget.EditText;
/*
* 监听输入内容是否超出最大长度,并设置光标位置
* */
public class MaxLengthWatcher implements TextWatcher {
private int maxLen = 0;
private EditText editText = null;
public MaxLengthWatcher(int maxLen, EditText editText) {
this.maxLen = maxLen;
this.editText = editText;
}
public void afterTextChanged(Editable arg0) {
// TODO Auto-generated method stub
}
public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
int arg3) {
// TODO Auto-generated method stub
}
public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
// TODO Auto-generated method stub
Editable editable = editText.getText();
int len = editable.length();
if(len > maxLen)
{
int selEndIndex = Selection.getSelectionEnd(editable);
String str = editable.toString();
//截取新字符串
String newStr = str.substring(0,maxLen);
editText.setText(newStr);
editable = editText.getText();
//新字符串的长度
int newLen = editable.length();
//旧光标位置超过字符串长度
if(selEndIndex > newLen)
{
selEndIndex = editable.length();
}
//设置新光标所在的位置
Selection.setSelection(editable, selEndIndex);
}
}
}
对应的 activity 部分的调用为:
[java] view plain copy print ?
- package cie.textEdit;
-
- import android.app.Activity;
- import android.os.Bundle;
- import android.text.InputFilter;
- import android.widget.EditText;
-
- public class TextEditActivity extends Activity {
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
-
- EditText editText = (EditText) findViewById(R.id.entry);
- editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
-
- }
- }
package cie.textEdit;
import android.app.Activity;
import android.os.Bundle;
import android.text.InputFilter;
import android.widget.EditText;
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText) findViewById(R.id.entry);
editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
}
}
限制输入字符数为10个
main.xml 文件
[html] view plain copy print ?
- <?xml version="1.0" encoding="utf-8"?>
- <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <TextView
- android:id="@+id/label"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:text="Type here:"/>
- <EditText
- android:id="@+id/entry"
- android:singleLine="true"
- android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:background="@android:drawable/editbox_background"
- android:layout_below="@id/label"/>
- <Button
- android:id="@+id/ok"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_below="@id/entry"
- android:layout_alignParentRight="true"
- android:layout_marginLeft="10dip"
- android:text="OK" />
- <Button
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:layout_toLeftOf="@id/ok"
- android:layout_alignTop="@id/ok"
- android:text="Cancel" />
- </RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/label"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Type here:"/>
<EditText
android:id="@+id/entry"
android:singleLine="true"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="@android:drawable/editbox_background"
android:layout_below="@id/label"/>
<Button
android:id="@+id/ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/entry"
android:layout_alignParentRight="true"
android:layout_marginLeft="10dip"
android:text="OK" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toLeftOf="@id/ok"
android:layout_alignTop="@id/ok"
android:text="Cancel" />
</RelativeLayout>
效果为输入了10个字符后,光标停在末尾
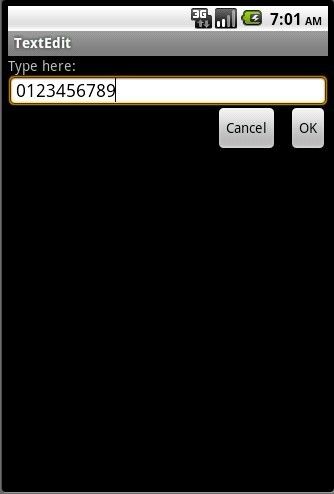