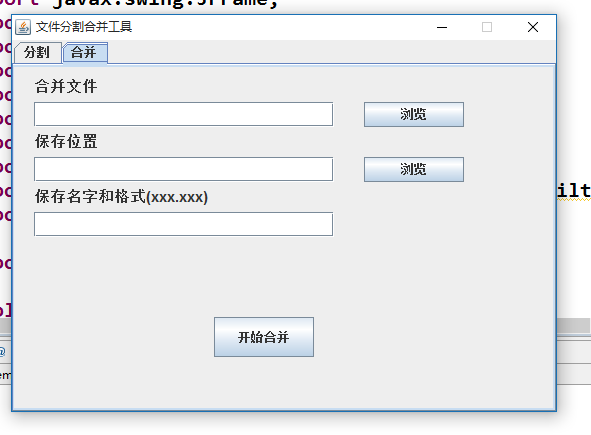
界面
package 文件分割合并;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.nio.file.OpenOption;
import javax.swing.BorderFactory;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTabbedPane;
import javax.swing.JTextField;
import javax.swing.filechooser.FileFilter;
import javax.swing.filechooser.FileNameExtensionFilter;
import javax.swing.text.html.Option;
import 音乐播放器.MusicPlayer;
public class JTabbedPaneDemo extends JFrame {
private JButton jbSplit = null;
private JButton jbMerge = null;
private JPanel jpSplit = null;
private JPanel jpMerge = null;
private JTextField jtfFileName = null, jtfFileName2 = null, jtf1 = null,
jtf2 = null, jtf3;
private JTextField jtfMergeFile1 = null, jtfMergeFile2 = null,
jtfMergeFile3 = null;
private JRadioButton jrb1 = null, jrb2 = null, jrb3=null, jrb4=null;
private File srcFile = null;
private File endFile = null;
private boolean isSplitWay = true;
private String splitWay = "10";
private boolean isSplitName = false;
private String splitName = "请输入",mergeName="";
public JButton jbStart = null, jbStop = null, jbExit = null,jbMergeStart=null;
private boolean isStart = true;
private SplitFile splitFile = null;
private File fileMerge=null,fileMerge2=null;
private boolean isMergeStart=false;
public JTabbedPaneDemo() {
super("文件分割合并工具");
this.setLocation(450, 200);
this.setSize(550, 400);
this.setLayout(new BorderLayout());
JTabbedPane jtp = new JTabbedPane();
jbSplit = new JButton("分割");
addSplit();
jtp.add(jpSplit, "分割");
jbMerge = new JButton("合并");
addMerge();
jtp.add(jpMerge, "合并");
this.getContentPane().add(jtp, BorderLayout.CENTER);
this.setResizable(false);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setVisible(true);
}
public void addSplit() {
jpSplit = new JPanel();
jpSplit.setLayout(null);
JLabel jlFileName = new JLabel("分割文件名");
jlFileName.setFont(new Font("csx", Font.BOLD, 15));
jpSplit.add(jlFileName);
jlFileName.setBounds(20, 10, 100, 20);
jtfFileName = new JTextField(20);
jtfFileName.setBounds(20, 35, 300, 25);
jpSplit.add(jtfFileName);
final JButton jbtSplit1 = new JButton("浏览");
jbtSplit1.setBounds(350, 35, 100, 25);
jpSplit.add(jbtSplit1);
jbtSplit1.setName("浏览1");
jbtSplit1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser jfc = new JFileChooser();
int returnVal = jfc.showOpenDialog(JTabbedPaneDemo.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
srcFile = jfc.getSelectedFile();
jtfFileName.setText(srcFile.getAbsolutePath());
}
}
});
JLabel jlFileName2 = new JLabel("保存位置");
jlFileName2.setFont(new Font("csx", Font.BOLD, 15));
jpSplit.add(jlFileName2);
jlFileName2.setBounds(20, 65, 100, 20);
jtfFileName2 = new JTextField(20);
jtfFileName2.setBounds(20, 90, 300, 25);
jpSplit.add(jtfFileName2);
JButton jbtSplit2 = new JButton("浏览");
jbtSplit2.setBounds(350, 90, 100, 25);
jpSplit.add(jbtSplit2);
jbtSplit2.setName("浏览2");
jbtSplit2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser jfc = new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnVal = jfc.showOpenDialog(JTabbedPaneDemo.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
endFile = jfc.getSelectedFile();
jtfFileName2.setText(endFile.getAbsolutePath());
}
}
});
JPanel jp = new JPanel();
jp.setLayout(new FlowLayout());
jp.setBounds(20, 120, 200, 100);
jp.setBorder(BorderFactory.createTitledBorder("分割方式"));
ButtonGroup bg = new ButtonGroup();
jrb1 = new JRadioButton("每份大小");
jtf1 = new JTextField(5);
jtf1.setText("1024");
JLabel jlb1 = new JLabel("kb");
jrb2 = new JRadioButton("平均分配");
jtf2 = new JTextField(5);
JLabel jlb2 = new JLabel("份");
bg.add(jrb1);
bg.add(jrb2);
jp.add(jrb1);
jp.add(jtf1);
jp.add(jlb1);
jp.add(jrb2);
jp.add(jtf2);
jp.add(jlb2);
jpSplit.add(jp);
jrb1.addActionListener(new action());
jrb2.addActionListener(new action());
JPanel jp2 = new JPanel();
jp2.setLayout(new FlowLayout());
jp2.setBounds(220, 120, 300, 100);
jp2.setBorder(BorderFactory.createTitledBorder("设置名称"));
ButtonGroup bg2 = new ButtonGroup();
jrb3 = new JRadioButton("自定义名字为");
jtf3 = new JTextField(5);
jtf3.setText(splitName);
JLabel jl3 = new JLabel("+ 序号");
jrb4 = new JRadioButton("默认以数字命名(如1.part,2.part...)");
jrb4.setSelected(true);
bg2.add(jrb3);
bg2.add(jrb4);
jp2.add(jrb3);
jp2.add(jtf3);
jp2.add(jl3);
jp2.add(jrb4);
jpSplit.add(jp2);
jrb3.addActionListener(new action());
jrb4.addActionListener(new action());
jbStart = new JButton("开始分割");
jbStart.setBounds(50, 250, 100, 40);
jpSplit.add(jbStart);
jbStart.addActionListener(new action());
jbStop = new JButton("停止分割");
jbStop.setBounds(200, 250, 100, 40);
jpSplit.add(jbStop);
jbStop.addActionListener(new action());
jbExit = new JButton("退出");
jbExit.setBounds(350, 250, 100, 40);
jpSplit.add(jbExit);
jbExit.addActionListener(new action());
}
public void addMerge() {
jpMerge = new JPanel();
jpMerge.setLayout(null);
JLabel jlMergeName = new JLabel("合并文件");
jlMergeName.setFont(new Font("csx", Font.BOLD, 15));
jpMerge.add(jlMergeName);
jlMergeName.setBounds(20, 10, 100, 20);
jtfMergeFile1 = new JTextField(20);
jtfMergeFile1.setBounds(20, 35, 300, 25);
JButton jbMerge1 = new JButton("浏览");
jbMerge1.setBounds(350, 35, 100, 25);
jpMerge.add(jbMerge1);
jpMerge.add(jtfMergeFile1);
jbMerge1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser jfc = new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnVal = jfc.showOpenDialog(JTabbedPaneDemo.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
fileMerge = jfc.getSelectedFile();
jtfMergeFile1.setText(fileMerge.getAbsolutePath());
}
}
});
JLabel jlMergeName2 = new JLabel("保存位置");
jlMergeName2.setFont(new Font("csx", Font.BOLD, 15));
jpMerge.add(jlMergeName2);
jlMergeName2.setBounds(20, 65, 100, 20);
jtfMergeFile2 = new JTextField(20);
jtfMergeFile2.setBounds(20, 90, 300, 25);
JButton jbMerge2 = new JButton("浏览");
jbMerge2.setBounds(350, 90, 100, 25);
jpMerge.add(jbMerge2);
jpMerge.add(jtfMergeFile2);
jbMerge2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser jfc = new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnVal = jfc.showOpenDialog(JTabbedPaneDemo.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
fileMerge2 = jfc.getSelectedFile();
jtfMergeFile2.setText(fileMerge2.getAbsolutePath());
}
}
});
JLabel jlMergeName3 = new JLabel("保存名字和格式(xxx.xxx)");
jlMergeName3.setFont(new Font("csx", Font.BOLD, 15));
jlMergeName3.setBounds(20, 120, 200, 20);
jtfMergeFile3 = new JTextField(20);
jtfMergeFile3.setBounds(20, 145, 300, 25);
jpMerge.add(jlMergeName3);
jpMerge.add(jtfMergeFile3);
jbMergeStart = new JButton("开始合并");
jbMergeStart.setBounds(200, 250, 100, 40);
jpMerge.add(jbMergeStart);
jbMergeStart.addActionListener(new action());
}
class action implements ActionListener {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == jrb1||e.getSource()==jtf1) {
isSplitWay = true;
jtf1.setEditable(true);
jtf2.setEditable(false);
}
if (e.getSource() == jrb2) {
isSplitWay = false;
jtf2.setEditable(true);
jtf1.setEditable(false);
}
if (e.getSource() == jrb3) {
isSplitName = true;
}
if (e.getSource() == jrb4) {
isSplitName = false;
}
if (e.getSource() == jbStart) {
isStart = true;
if (srcFile == null || endFile == null) {
JOptionPane.showMessageDialog(JTabbedPaneDemo.this,
"文件不存在,请重新选择");
return;
}
if(isSplitWay){
splitWay=jtf1.getText();
}else{
splitWay=jtf2.getText();
}
System.out.println(isSplitName);
if(isSplitName){
splitName = jtf3.getText();
}
System.out.println(splitName);
splitFile = new SplitFile(srcFile, endFile, isSplitWay,
splitWay, isSplitName, splitName, isStart,JTabbedPaneDemo.this);
Thread t = new Thread(splitFile);
t.start();
}
if (e.getSource() == jbStop) {
splitFile.isStart = false;
jbStart.setEnabled(true);
}
if (e.getSource() == jbExit) {
System.exit(-1);
}
if(e.getSource()==jbMergeStart){
isMergeStart=true;
mergeName=jtfMergeFile3.getText();
MergeFile merge=new MergeFile(fileMerge,fileMerge2,mergeName,isMergeStart,JTabbedPaneDemo.this);
Thread t=new Thread(merge);
t.start();
}
}
}
public static void main(String[] args) {
new JTabbedPaneDemo();
}
}
切割功能
package 文件分割合并;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.JOptionPane;
public class SplitFile implements Runnable {
private File srcFile = null;
private File endFile = null;
private boolean isSplitWay;
private int splitWay = 0;
private boolean isSplitName;
private String splitName = null;
public boolean isStart;
private JTabbedPaneDemo mainJfram=null;
public SplitFile(File srcFile, File endFile, boolean isSplitWay,
String splitWay, boolean isSplitName, String splitName,
boolean isStart,JTabbedPaneDemo mainJfram) {
this.srcFile = srcFile;
this.endFile = endFile;
this.isSplitWay = isSplitWay;
try {
this.splitWay = Integer.parseInt(splitWay);
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(null, "分割大小错误");
return;
}
this.isSplitName = isSplitName;
this.splitName = splitName;
this.isStart = isStart;
this.mainJfram=mainJfram;
}
public void run() {
while (isStart) {
mainJfram.jbStart.setEnabled(false);
FileInputStream fis = null;
BufferedInputStream bis = null;
FileOutputStream fos = null;
BufferedOutputStream bos = null;
try {
fis = new FileInputStream(srcFile);
bis = new BufferedInputStream(fis);
byte[] buf = null;
if (isSplitWay) {
buf = new byte[splitWay * 1024];
} else {
buf = new byte[(int) (srcFile.length() / splitWay)+10];
}
if (!isSplitName) {
splitName = "";
}
int len = 0;
int count = 0;
while ((len = bis.read(buf)) != -1) {
fos = new FileOutputStream(endFile +"\\"+"_"+splitName + (++count)
+ ".part");
bos = new BufferedOutputStream(fos);
bos.write(buf, 0, len);
bos.flush();
bos.close();
System.out.println(count);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bis != null) {
bis.close();
JOptionPane.showMessageDialog(null, "恭喜你,文件分割完成");
}
} catch (IOException e) {
throw new RuntimeException("关流失败");
}finally{
mainJfram.jbStart.setEnabled(true);
isStart=false;
}
}
}
}
}
合并功能
package 文件分割合并;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.SequenceInputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Enumeration;
import javax.swing.JOptionPane;
public class MergeFile implements Runnable {
private File fileMerge = null;
private File fileMerge2 = null;
private String mergeName = null;
private boolean isMergeStart = false;
JTabbedPaneDemo mainJfram = null;
public MergeFile(File fileMerge, File fileMerge2, String mergeName,
boolean isMergeStart, JTabbedPaneDemo mainJfram) {
this.fileMerge = fileMerge;
this.fileMerge2 = fileMerge2;
this.mergeName = mergeName;
this.isMergeStart = isMergeStart;
this.mainJfram = mainJfram;
System.out.println(mergeName);
}
public void run() {
while (isMergeStart) {
FileOutputStream fos = null;
BufferedOutputStream bos = null;
SequenceInputStream sis = null;
try {
mainJfram.jbMergeStart.setEnabled(false);
File[] file = fileMerge.listFiles();
ArrayList<File> list = new ArrayList<File>();
for (File f : file) {
list.add(f);
}
Collections.sort(list, new Comparator<File>() {
public int compare(File o1, File o2) {
String str1 = o1.getName().split("_")[1].split("\\.")[0];
String str2 = o2.getName().split("_")[1].split("\\.")[0];
return Integer.parseInt(str1) - Integer.parseInt(str2);
}
});
ArrayList<FileInputStream> list2 = new ArrayList<FileInputStream>();
for (File f : list) {
list2.add(new FileInputStream(f));
}
Enumeration<FileInputStream> en = Collections
.enumeration(list2);
sis = new SequenceInputStream(en);
fos = new FileOutputStream(fileMerge2 + "\\" + mergeName);
bos = new BufferedOutputStream(fos);
byte[] buf = new byte[1024];
int len = 0;
System.out.println("测试");
while ((len = sis.read(buf)) != -1) {
bos.write(buf, 0, len);
}
} catch (IOException e) {
JOptionPane.showMessageDialog(null, "合并失败,文件可能不存在或有问题");
} finally {
try {
if (sis != null) {
sis.close();
}
if (bos != null) {
bos.close();
}
} catch (IOException e) {
} finally {
mainJfram.jbMergeStart.setEnabled(true);
isMergeStart = false;
}
}
}
}
}