一、框架概述:
框架的优点:开发速度快,规范
后台框架:Hibernate,MyBATIS(iBATIS),EJB
前台框架(MVC):Struts(1/2),SpringMVC,JSF
Spring框架:Spring,Seam
JS框架:JQuery,ExtJS
二、Hibernate框架与ORMapping概念
ORMapping:对象关系映射,通过在类与表之间建立关系,使程序操作类能自动影响到表中的数据。
ORMapping的发展过程:
|
优点 |
缺点 |
JDBC |
好学,执行速度快 |
重复代码比较多,开发速度慢 |
EJB1,2 |
提出了ORMapping |
除了有概念,什么都不行。 |
JDO |
简单 |
连接控制有问题 |
Apache OJB |
无 |
太多 |
Hibernate |
很多 |
执行速度慢 |
MyBATIS |
比Hibernate执行速度快 比JDBC代码简单 |
比Hibernate代码多 比JDBC执行速度慢 |
EJB3 |
使用了Hibernate的源代码 |
架构还是EJB的原始架构 |
Hibernate建立ORMapping关系的方式是使用 XML或Annotation(在Hibernate3.2以上版本才可以使用)
JDBC完成数据库操作的支持类: Hibernate
Connection —> Session
PreparedStatement —> Query
ResultSet —> List
DataBaseConnection —> HibernateSessionFactory
三、Hibernate完成单表数据库CRUD操作
1,在数据库中建立一张数据表,这里建立一个张新闻表(news)
CREATE TABLE news (
id number(8) primary key ,
title varchar2(50) not null,
content varchar2(500) not null,
pub_date date not null
);
在MyEclipse中需要建立项目,并加入Hibernate框架支持。但在这些之前,建议先在MyEclipse里建立与数据库的连接。
建立数据库连接:windows-->show view-->other-->MyEclipse Database-->DB Browser;
然后右击DB Brower 下的空白部分选择New,在Driver template 中选择所需的Oracle(Thin driver),Driver name 随便取,
Connection UrL: jdbc:oracle:thin:@localhost:1521:ORCL, username 和password是数据库连接的姓名和密码;
Driver JARs:添加的是Oracle的驱动Jar包;
点Test Driver,测试是否连接成功。测试成功后如果可以在连接中找到之前建立好的表,表示配置成功,可以开始建立项目。
2、新建项目:FirstHibernateDemo,加入Hibernate支持
右击MyEclipse-->Add Hibernate Capabilities
选择Hibernate版本Hibernate3.3(足够可以用),虽然hibernate最新版已经到4.0+,但是开发中没人用,因为太新;
如果要手工加入框架支持,需要加入以下两部分内容:
1) 支持jar包加入到WEB-INF/lib目录下
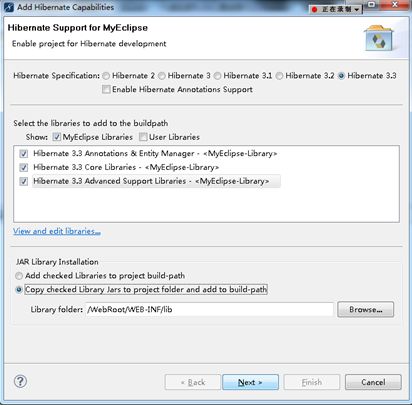
2) Hibernate.cfg.xml(核心配置文件,不推荐改名字)加入到src目录下。
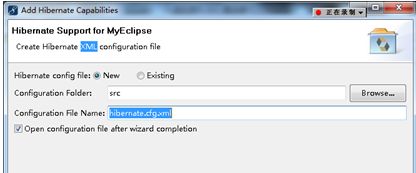
建立数据库连接的类:
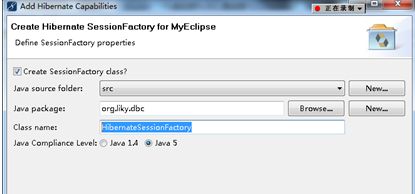
好了,点击Finish,Hibernate支持加好了,项目环境搭建好了;
Hibernate核心配置文件的功能:
1) 配置数据库连接参数(已经配置好了)
2) 加入Hibernate的属性配置,例如:通常加入两个属性property:show_sql,value:true; 格式化sql语句 property:format_sql,value:true;
3、生成的HibernateSessionFactory中,实现了连接池功能,要求掌握连接池的实现原理。
- public class HibernateSessionFactory {
-
- private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
-
- private static final ThreadLocal<Session> threadLocal = new ThreadLocal<Session>();
-
- private static Configuration configuration = new Configuration();
-
- private static org.hibernate.SessionFactory sessionFactory;
-
- private static String configFile = CONFIG_FILE_LOCATION;
-
-
- static {
- try {
-
- configuration.configure(configFile);
-
- sessionFactory = configuration.buildSessionFactory();
- } catch (Exception e) {
- System.err.println("%%%% Error Creating SessionFactory %%%%");
- e.printStackTrace();
- }
- }
-
- private HibernateSessionFactory() {
- }
-
-
-
-
-
- public static Session getSession() throws HibernateException {
-
- Session session = (Session) threadLocal.get();
-
-
- if (session == null || !session.isOpen()) {
- session = sessionFactory.openSession();
-
- threadLocal.set(session);
- }
- return session;
- }
-
-
-
-
- public static void closeSession() throws HibernateException {
- Session session = (Session) threadLocal.get();
-
- threadLocal.set(null);
-
- if (session != null) {
- session.close();
- }
- }
- public static Configuration getConfiguration() {
- return configuration;
- }
- }
4、下面需要根据数据库表,完成Vo对象。.
注意,在一些框架中,VO课程被称为其他的称呼。
TO(EJB1,2),POJO(Hibernate),EntityBean(EJB3)
MyEclipse中提供了根据表自动生成pojo和映射文件的功能。
在DB Browser下,找到对应用户名下table下的news表,选中右击,Hibernate Reverse Engineering -->在java src folders选择对应项目的src;
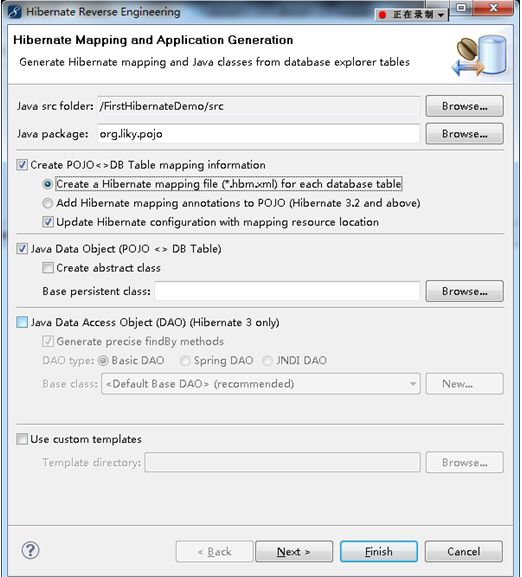
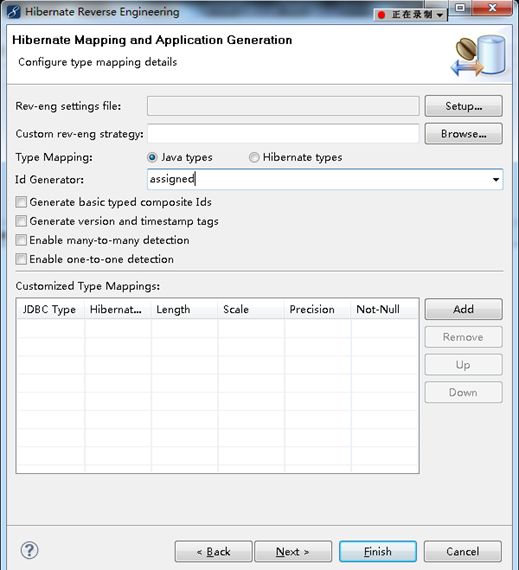
这里需要选择主键生成方式,主要有以下几种:
1) assigned:通过程序添加。
2) sequence:通过Oracle的序列生成主键值
3) native:通过数据库表中自带的关键字生成主键值,例如:MySQL,SQLServer,DB2,HSQL等
4) increment:自增长,通过程序实现自增长功能。
5) UUID:生成一个32的位随机值作为主键。
点击finish即可以生成Hibernate的映射;生成的pojo对象
映射文件要求能看懂,会改。News.hbm.xml:
- <?xml version="1.0" encoding="utf-8"?>
- <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
- "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
- <hibernate-mapping>
- <!--
- News类和SUNXUN用户下的NEWS表映射.
- -->
- <class name="org.liky.pojo.News" table="NEWS" schema="SUNXUN">
- <!--
- 类中的Integer类型的id对应表中的主键
- -->
- <id name="id" type="java.lang.Integer">
- <!--
- 表中主键字段为ID,scale是指小数点后0位,即是整形
- -->
- <column name="ID" precision="8" scale="0" />
- <!--
- 主键生成方式
- -->
- <generator class="assigned" />
- </id>
- <!--
- 类中的String类型的title属性与表中的TITLE字段对应,长度是50,不允许为空
- -->
- <property name="title" type="java.lang.String">
- <column name="TITLE" length="50" not-null="true" />
- </property>
- <property name="content" type="java.lang.String">
- <column name="CONTENT" length="500" not-null="true" />
- </property>
- <property name="pubDate" type="java.util.Date">
- <column name="PUB_DATE" length="7" not-null="true" />
- </property>
- </class>
- </hibernate-mapping>
5、创建一个公共的DAO接口方法,为了方便使用。
-
-
-
-
-
-
-
-
- public interface IDAO<K, V> {
-
- public void doCreate(V vo) throws Exception;
-
- public void doUpdate(V vo) throws Exception;
-
- public void doRemove(K id) throws Exception;
-
- public List<V> findAll() throws Exception;
-
- public V findById(K id) throws Exception;
-
-
-
-
- public List<V> findAll(int pageNo, int pageSize, String keyword,
- String column) throws Exception;
-
-
-
-
- public int getAllCount(String keyword, String column) throws Exception;
-
- }
6、建立新闻的接口,继承公共接口,完成操作。
- public interface INewsDAO extends IDAO<Integer, News> {
- }
7、建立实现类对象
- public class NewsDAOImpl implements INewsDAO {
- public void doCreate(News vo) throws Exception {
- HibernateSessionFactory.getSession().save(vo);
- }
- public void doRemove(Integer id) throws Exception {
-
- HibernateSessionFactory.getSession().delete(findById(id));
- }
- public void doUpdate(News vo) throws Exception {
- HibernateSessionFactory.getSession().update(vo);
- }
- public List<News> findAll() throws Exception {
-
-
-
- String hql = "FROM News";
- Query query = HibernateSessionFactory.getSession().createQuery(hql);
- return query.list();
- }
- public List<News> findAll(int pageNo, int pageSize, String keyword,
- String column) throws Exception {
- String hql = "FROM News AS n WHERE n." + column + " LIKE ?";
-
- Query query = HibernateSessionFactory.getSession().createQuery(hql);
- query.setString(0, "%" + keyword + "%");
-
-
- query.setFirstResult((pageNo - 1) * pageSize);
- query.setMaxResults(pageSize);
-
- return query.list();
- }
- public News findById(Integer id) throws Exception {
-
- return (News) HibernateSessionFactory.getSession().get(News.class, id);
- }
- public int getAllCount(String keyword, String column) throws Exception {
-
- String hql = "SELECT COUNT(n) FROM News AS n WHERE n." + column
- + " LIKE ?";
- Query query = HibernateSessionFactory.getSession().createQuery(hql);
-
- query.setString(0, "%" + keyword + "%"); <p align="left"> <span style="white-space:pre"> </span><span style="color:#cc0000;">
9、建立工厂类
- public class DAOFactory {
- public static INewsDAO getINewsDAOInstance() {
- return new NewsDAOImpl();
- }
- }
10、编写Service层,这里随意定义几个方法
- public interface INewsService {
- public void insert(News news) throws Exception;
- public void delete(int id) throws Exception;
- public News findById(int id) throws Exception;
-
- public Map<String, Object> list(int pageNo, int pageSize, String keyword,
- String column) throws Exception;
- }
11、建立实现类
- public class NewsServiceImpl implements INewsService {
- public void delete(int id) throws Exception {
-
- Transaction tx = HibernateSessionFactory.getSession()
- .beginTransaction();
- try {
- DAOFactory.getINewsDAOInstance().doRemove(id);
- tx.commit();
- } catch (Exception e) {
- e.printStackTrace();
- tx.rollback();
- throw e;
- } finally {
- HibernateSessionFactory.closeSession();
- }
- }
-
- public News findById(int id) throws Exception {
- News news = null;
- try {
- news = DAOFactory.getINewsDAOInstance().findById(id);
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- } finally {
- HibernateSessionFactory.closeSession();
- }
- return news;
- }
-
- public void insert(News news) throws Exception {
-
- Transaction tx = HibernateSessionFactory.getSession()
- .beginTransaction();
- try {
- DAOFactory.getINewsDAOInstance().doCreate(news);
- tx.commit();
- } catch (Exception e) {
- e.printStackTrace();
- tx.rollback();
- throw e;
- } finally {
- HibernateSessionFactory.closeSession();
- }
- }
-
- public Map<String, Object> list(int pageNo, int pageSize, String keyword,
- String column) throws Exception {
- Map<String, Object> map = new HashMap<String, Object>();
- try {
- map.put("allNews", DAOFactory.getINewsDAOInstance().findAll(pageNo,
- pageSize, keyword, column));
- map.put("allCount", DAOFactory.getINewsDAOInstance().getAllCount(
- keyword, column));
- } catch (Exception e) {
- e.printStackTrace();
- throw e;
- } finally {
- HibernateSessionFactory.closeSession();
- }
- return map;
- }
- }
12、工厂类
- public class ServiceFactory {
- public static INewsService getINewsServiceInstance() {
- return new NewsServiceImpl();
- }
- }
13、JUnit测试
针对Service的所有方法,开发中一般要求使用JUnit编写测试用例进行测试。
- public class NewsServiceImplTest {
-
- @Test
- public void testDelete() throws Exception {
- ServiceFactory.getINewsServiceInstance().delete(2);
- }
-
- @Test
- public void testFindById() throws Exception {
- System.out.println(ServiceFactory.getINewsServiceInstance().findById(2)
- .getTitle());
- }
-
- @Test
- public void testInsert() throws Exception {
- News news = new News(3, "测试添加数据032", "测试内容023", new Date());
- ServiceFactory.getINewsServiceInstance().insert(news);
- }
-
- @Test
- public void testList() throws Exception {
- Map<String, Object> map = ServiceFactory.getINewsServiceInstance()
- .list(1, 2, "添加", "title");
- System.out.println(map.get("allCount"));
- System.out.println(map.get("allNews"));
- }
- }
在执行时,控制台中一直提示警告信息,该警告是因为Hibernate中加入的log4j支持造成的。
如果不想显示这个警告,需要自己将log4j的配置文件加入到项目中。
Hibernate中使用到的类和配置:
1) Configuration:读取配置文件
2) SessionFactory:连接池
3) ThreadLocal:实现连接池的支持类
4) Session:数据库连接
a) Save()
b) Update()
c) Delete()
d) Get()
e) createQuery()
f) beginTransaction()
5) Query:查询对象
a) setXxx:设置参数,从 0 开始
b) list():查询多条数据
c) uniqueResult():查询唯一数据,前提是必须只有一条结果,只在查询全部记录数时使用。
6) Transaction:事务处理对象
a) Commit
b) rollback