- 金融风控算法透明度与可解释性优化
智能计算研究中心
其他
内容概要金融风控算法的透明化研究面临模型复杂性提升与监管合规要求的双重挑战。随着深度学习框架在特征提取环节的广泛应用,算法可解释性与预测精度之间的平衡成为核心议题。本文从联邦学习架构下的数据协作机制出发,结合特征工程优化与超参数调整技术,系统性分析逻辑回归、随机森林等传统算法在召回率、F1值等关键指标上的表现差异。研究同时探讨数据预处理流程对风控决策鲁棒性的影响,并提出基于注意力机制的特征权重可视
- 联邦学习算法安全优化与可解释性研究
智能计算研究中心
其他
内容概要本研究围绕联邦学习算法的安全性优化与模型可解释性增强展开系统性探索。首先,针对联邦学习中数据隐私泄露与模型性能损耗的固有矛盾,提出一种融合差分隐私与动态权重聚合的协同优化框架,通过分层加密机制降低敏感信息暴露风险。其次,引入可解释性算法(如LIME与SHAP)构建透明化决策路径,结合注意力机制实现特征贡献度的可视化映射,有效提升模型在医疗影像异常检测与金融欺诈识别场景中的可信度。此外,研究
- 8Manage竞价系统如何帮助优化汽车零部件采购管理?
Sadie_d
汽车软件需求软件工程
在汽车零部件制造行业,采购管理是企业运营中的核心环节之一。随着市场竞争的加剧和供应链复杂性的提升,如何高效、透明地完成询比价流程,成为许多企业关注的重点。特别是对于一家汽车零部件制造商来说,从发布采购需求到最终选择供应商,整个过程需要兼顾效率、成本和质量。而引入一个智能化的采购竞价系统,或许能为这一需求提供理想的解决方案。汽车零部件采购的痛点对于汽车零部件制造商而言,采购环节往往面临多重挑战。首先
- 实现图片处理功能鸿蒙示例代码
本文原创发布在华为开发者社区。介绍本项目基于OpenHarmony三方库ImageKnife进行图片处理场景开发使用:支持不同类型的本地与网络图片展示。支持拉起相机拍照展示与图库照片选择展示。支持图片单一种变换效果。支持本地/在线图片格式:JPG、PNG、SVG、GIF、DPG、WEBP、BMP实现图片处理功能源码链接效果预览使用说明下载安装根目录下的oh-package.json5中depend
- 2025健康保障新选择 众托帮用“互助力量”为家庭健康加码
创新
近期,《健康中国2030规划纲要》中期评估报告发布,明确提出“鼓励社会互助机制创新,完善多层次医疗保障”。面对医保目录外用药、突发重疾等潜在风险,越来越多家庭开始关注“基础医保+互助保障”的双重防护模式。众托帮作为国内领先的大病互助平台,以灵活参与、透明运作的特点,成为千万家庭的健康“备选项”。**社会互助成健康中国“新解法”**2025年国家多部门联合印发《关于引导社会力量参与医疗保障的指导意见
- 区块链赋能:用Python开发去中心化投票系统
Echo_Wish
Python!实战!区块链python去中心化
区块链赋能:用Python开发去中心化投票系统在这个互联网迅猛发展的时代,投票系统不仅仅停留在政务领域,它已成为社区治理、企业决策甚至区块链DAO(去中心化自治组织)中重要的机制。然而,传统投票系统往往集中化,存在信任和数据安全问题。区块链技术以其不可篡改性和透明性为去中心化投票提供了理想的解决方案。在这篇文章中,我将通过Python语言,结合区块链智能合约,教你如何从零开发一个去中心化的投票系统
- 【从零开始学习计算机科学】信息安全(十三)区块链
贫苦游商
学习区块链hash公有链私有链信息安全网络安全
【从零开始学习计算机科学】信息安全(十三)区块链区块链区块链概述区块链的主要特性开放,共识交易透明,双方匿名不可篡改,可追溯区块链的主要类别公有链私有链联盟链区块链核心技术Hash指针Merkle(梅根)树SPV交易验证过程区块链网络分叉解决机制51%攻击问题基于比特币的区块链的优势与不足常用的区块链区块链区块链概述能否在互联网环境(开放环境)下,创造一种技术,使得在无法保证人们相互信任的前提下,
- Python赋能区块链溯源系统:从技术实现到应用落地
Echo_Wish
Python!实战!python区块链开发语言
Python赋能区块链溯源系统:从技术实现到应用落地在供应链管理、食品安全、药品追踪等多个领域,产品的来源和流通过程正成为消费者和企业关注的重点。传统溯源系统往往缺乏数据透明性和不可篡改性,而区块链技术的引入解决了这些痛点,将溯源信息永久记录在分布式账本上,实现全流程可追溯。那么问题来了:如何用Python这把“瑞士军刀”构建一个高效的区块链溯源系统?本文将围绕这一主题,深入探讨Python在区块
- DApp开发需要多少钱?全面解析DApp开发成本
Lovely_xwys
区块链开发区块链人工智能web3
随着区块链技术的不断发展和普及,去中心化应用(DApp)逐渐成为金融科技领域的新宠。DApp以其去中心化、透明性和安全性等特点,吸引了众多开发者和投资者的关注。然而,对于想要涉足DApp开发的人来说,最关心的问题之一就是:DApp开发需要多少钱?本文将从多个角度全面解析DApp的开发成本。一、DApp开发成本概述DApp的开发成本并非一成不变,它受到多种因素的影响,包括项目的复杂度、功能需求、技术
- TDE透明加密技术:免改造实现华为云ECS中数据库和文件加密存储
安 当 加 密
华为云数据库
在数字经济与云计算深度融合的今天,华为云ECS(弹性云服务器)已成为企业数字化转型的核心载体,承载着数据库、文件存储、AI训练等关键业务。然而,云上数据安全形势日益严峻:2024年全球云环境勒索攻击同比激增210%,密钥泄露、权限失控、合规失效成为企业上云的三大痛点。作为国内数据安全领域的领军者,上海安当推出的TDE透明加密技术,以“存储层无感加密、密钥全生命周期管理、动态防勒索”为核心,为华为云
- uni-app全局弹窗的实现方案
-Dayer-
uni-app前端javascript全局弹窗
背景为了解决uni-app任意位置出现弹窗解决方案一、最初方案受限于uni-app调用组件需要每个页面都引入注册才可以使用,此方案繁琐,每个页面都要写侵入性比较强二、改进方案app端:新建一个页面进行跳转,可以实现伪弹窗(其实是打开一个背景透明的页面)web端:全局挂载body插入一个弹窗三、初步实现方案就是利用条件编译,web端写组件、app端写页面,利用不同的生命周期,完成通用的逻辑四、详细实
- SVN a peg revision is not allowed here 解决办法
男子峰
svnSVNbug
默认情况下,同名图片,更新的时候,后来更行的是会直接替换原图,所以会出现相应的英文提示。然后执行上面所说的svnresolved指令的时候,会出现一些问题(以名为btn@2x的图片为例):svnresolved项目名/图片资源文件夹名字/btn@2x然后,svn会报错!E200009:'项目名/图片文件夹名/
[email protected]':apegrevisionisnotallowedhere解决:在图
- ubuntu为pycharm添加系统快捷启动图标
金大大诶
Linux
一、首先,在桌面创建一个文件:pycharm.desktop二、编辑文件,添加以下内容:(Exec是sh文件位置,icon是图标文件位置)[DesktopEntry]Version=1.0Type=ApplicationName=PycharmIcon=/home/du/Documents/pycharm-community-2017.3.3/bin/pycharm.pngExec=/home/d
- QILSTE H4-108LB高亮蓝光LED灯珠 发光二极管LED
QILSTE
QILSTELED灯珠发光二极管LED
#H4-108LB:高亮蓝光LED的参数解析与应用挑战在电子设备的照明领域,H4-108LB型号的高亮蓝光LED以其独特的光电特性占据一席之地。这款LED产品尺寸仅为1.6×0.8×0.4mm,却蕴含着复杂的参数设计,使其在众多应用场景中表现出色。然而,要真正理解其性能优势,就必须深入剖析其参数的复杂性。##一、尺寸与封装:紧凑与透明的结合H4-108LB的外观尺寸为1.6×0.8×0.4mm,这
- 医疗影像联邦学习可解释性算法研究
智能计算研究中心
其他
内容概要医疗影像分析领域的联邦学习技术正面临数据隐私保护与模型可解释性的双重挑战。本研究以跨机构医疗影像协作场景为核心,系统性探讨联邦学习框架下可解释性算法的创新路径,重点解决医疗AI模型在分布式训练中的透明度缺失问题。通过引入动态特征选择机制与可解释性注意力模块,算法在保持数据本地化处理的同时,实现了关键病灶特征的跨域关联与可视化解析。研究同步整合自动化数据增强流程与多维度评估指标(如F1值、召
- 什么是数据库的分区技术?
破碎的天堂鸟
学习教程数据库
数据库分区技术详解数据库分区技术是一种通过将大型表或索引分割成多个逻辑独立、物理可分离的单元(即分区)来优化性能和管理效率的核心策略。以下是其核心要点和应用的全面解析:1.定义与基本原理数据库分区(Partitioning)是一种物理数据库设计技术,通过特定规则(如范围、列表、哈希等)将表或索引划分为更小、更易管理的逻辑单元。每个分区可独立存储于不同物理位置(如磁盘或服务器),但对应用层透明,逻辑
- TDE透明加密:重塑文件传输与网盘存储的安全新范式
安 当 加 密
安全
在数据要素价值持续释放的今天,企业文件传输与存储系统正面临**“既要跨域流动,又要严防泄露”的双重挑战。传统加密方案往往陷入两难困境:离线传输依赖手工解密导致效率低下,网盘存储依赖平台方加密存在密钥失控风险。作为国内数据安全领域的创新者,上海安当推出的TDE透明加密技术**,以**“端到端无感加密、全链路权限管控、跨平台无缝兼容”**为核心,为企业构建从文件生成、传输到存储的全生命周期防护体系。本
- 扫盲系列--Web3智能合约+Solidity简介
「已注销」
前端框架
前言这几天web3智能合约这个概念,频繁映入我的眼帘。web3.0这个概念我听说过,核心特征是去中心化、开放性、隐私保护和数据所有权回归个人。Web1.0是信息浏览时代,Web2.0是用户参与和社交网络时代,Web3.0是去中心化与智能化时代。在Web3.0这一新的互联网架构下,用户不再仅仅是内容的消费者,更是自己数字身份和数据的拥有者。Web3.0旨在构建一个更加透明、安全且高效的信息网络。我对
- 无人自助空间智能管理系统解决方案(深度优化版)
ALLSectorSorft
大数据人工智能网络自动化小程序uni-appandroid
无人自助空间智能管理系统解决方案(深度优化版)一、行业痛点与系统价值传统管理依赖人工:人工管理模式下,易出现人为失误,如计费错误、资源分配不当等。同时,人工操作效率低下,在高峰时段难以快速响应客户需求。且夜间运营需额外安排人力,增加运营成本,导致夜间运营困难。资源利用率不透明:由于缺乏有效的数据监测与分析手段,空间资源的空置率难以实时掌握,造成资源浪费。这也使得收益难以准确预测,不利于商家制定合理
- 手机租赁系统开发核心技术解析
红点租赁系统开发
其他
内容概要如果把手机租赁系统比作一台精密运转的智能管家,那它的骨架可不是用代码随便搭的乐高积木。这玩意儿得同时搞定三件事:让用户像刷短视频一样流畅下单,让风控系统比小区门禁还难糊弄,还得让物流信息比外卖小哥的定位更透明。想象一下,当你在APP里滑动挑选最新款折叠屏手机时,后台其实正在上演三重加密的信用评分大战——你的芝麻信用分、电商平台消费记录甚至社交账号活跃度,都被塞进算法熔炉里炼成租赁权限的通行
- 链上赋能:智能合约重塑供应链管理
Echo_Wish
前沿技术人工智能智能合约linux运维
链上赋能:智能合约重塑供应链管理供应链是现代经济活动的核心,而复杂的供应链环节常常面临诸多挑战:数据孤岛、信息不透明、操作低效甚至信任危机。这些问题不仅增加了运营成本,还导致资源浪费。随着区块链技术的兴起,供应链管理迎来了新的解决方案,其中智能合约(SmartContract)作为区块链的重要组成部分,正在颠覆传统的供应链管理模式。在本文中,我将结合Python开发与智能合约,探讨智能合约在供应链
- 程序化广告行业(27/89):供应商筛选、比稿流程与广告透明化要点
lilye66
程序化广告sqlserver数据库cloudera大数据
程序化广告行业(27/89):供应商筛选、比稿流程与广告透明化要点在数字化营销浪潮中,程序化广告已成为企业精准触达目标受众的重要手段。一直以来,我都期望和大家一同深入钻研技术领域知识,实现共同进步。今天,咱们继续剖析程序化广告行业,聚焦在供应商筛选、比稿流程以及广告透明化这些关键环节,帮助大家更好地理解这一行业的运作机制。供应商筛选与比稿流程详解供应商入库评估要点在程序化广告投放中,供应商的选择至
- 利用deepseek AI制作视频的小白教程
银行金融科技
银行信息系统架构详解机器学习人工智能deepseek
以下是基于DeepSeekAI的完整视频制作教程,涵盖从剧本到成片的全流程操作(附关键技巧):一、前期准备(1天)1.分镜优化根据之前的分镜脚本,用Notion或Excel整理出AI友好型分镜表(示例):画面描述关键词时长动态水墨展开成卷轴水墨山水、花瓣特效、东晋风格15秒Q版人物在曲水流觞放纸船全息投影、透明茶具、童声配音45秒2.素材预生成文本转图像:bash#用DeepSeekCoder生成
- 8Manage采购管理软件如何助力原材料厂商实现闭环管理?
Sadie_d
大数据软件工程软件需求
在原材料生产行业,尤其是像高分子材料、半导体、纳米材料、高性能复合材料这样的高技术领域,采购管理的效率直接关系到企业的生产进度和成本控制。对于一家拥有数百家外部供应商的厂商来说,采购流程不仅复杂,还需要高度的协调性和透明度。从供应商管理到采购订单下达,再到对账付款,如何实现整个采购流程的闭环管理,成为许多企业关注的重点。本文将围绕这一需求,探讨采购管理软件如何助力企业优化流程,并提供一些实用的选型
- 使用 WebP 优化 GPU 纹理占用
泫凝
javascript前端
WebP格式相比JPEG/PNG文件更小,可以减少GPU纹理内存占用,提高WebGL/Three.js/3D渲染的性能。为什么WebP能减少GPU内存占用?文件更小→WebP比JPG/PNG压缩率更高,减少纹理上传带宽,提高渲染速度。支持透明度(RGBA)→比PNG更小,适用于UI贴图/透明纹理。减少Mipmaps体积→WebP生成的mipmap纹理占用的GPU内存更少。⚠️但WebP仍然是8-b
- Python计算DEM(tif格式)坡度和坡向
见贤思齐547
Python地理数据处理python
本文根据山东省DEM图获取坡度、坡向图,使用了三种方式:PythonGDAL工具自带的函数处理、Python中自己编写函数实现和arcgis中实现。一.Python中实现(针对TIF格式的DEM数据)1.利用gdal工具处理(1)代码fromosgeoimportgdal,osr#获取影像信息infoDEM=gdal.Info(r"D:\ProfessionalProfile\DEMdata\2_
- PyTorch 生态概览:为什么选择动态计算图框架?
小诸葛IT课堂
pytorch人工智能python
一、PyTorch的核心价值PyTorch作为深度学习框架的后起之秀,通过动态计算图技术革新了传统的静态图模式。其核心优势体现在:动态灵活性:代码即模型,支持即时调试Python原生支持:无缝衔接Python生态高效的GPU加速:通过CUDA实现透明的硬件加速活跃的社区生态:GitHub贡献者超1.8万人,日均更新100+次二、动态计算图VS静态计算图对比#动态计算图示例(PyTorch)impo
- uniapp移动端图片比较器组件,仿英伟达官网rtx光追图片比较器功能
独断万古的伊莉雅
uni-appjavascript前端组件
组件下载地址:https://ext.dcloud.net.cn/plugin?id=22609已测试h5和微信小程序,理论支持全平台亮点:简单易用使用js计算而不是resize属性,定制化程度更高组件挂在后可播放指示线动画,提示用户可以拖拽比较图片左右下角可配置文字,且指引线距离文字过近时文字会变透明使用示例RTXOFF效果示例:
- python基于django/flask网上书城系统Django-SpringBoot-php-Node.js-flask
QQ_1963288475
pythondjangoflaskspringbootphplaravelnode.js
目录技术栈介绍具体实现截图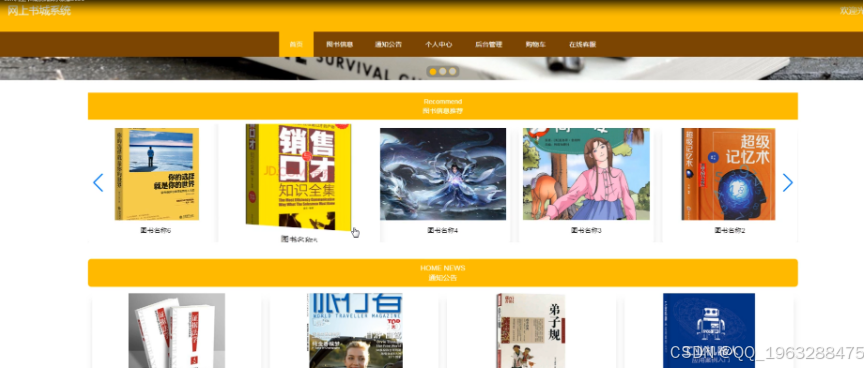系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研
- 人工智能伦理与可持续发展
CarlowZJ
人工智能
前言人工智能(AI)技术正在深刻地改变我们的生活和工作方式。从自动驾驶汽车到智能医疗系统,从个性化推荐到自动化决策,AI的应用无处不在。然而,随着技术的快速发展,其伦理和社会影响也引发了广泛的关注。人工智能伦理不仅涉及技术本身的公平性、透明性和安全性,还涉及到更广泛的社会、经济和环境影响。本文将探讨人工智能伦理的核心问题,并从可持续发展的角度提出应对策略。一、人工智能伦理的核心问题1.1数据隐私与
- 用MiddleGenIDE工具生成hibernate的POJO(根据数据表生成POJO类)
AdyZhang
POJOeclipseHibernateMiddleGenIDE
推荐:MiddlegenIDE插件, 是一个Eclipse 插件. 用它可以直接连接到数据库, 根据表按照一定的HIBERNATE规则作出BEAN和对应的XML ,用完后你可以手动删除它加载的JAR包和XML文件! 今天开始试着使用
- .9.png
Cb123456
android
“点九”是andriod平台的应用软件开发里的一种特殊的图片形式,文件扩展名为:.9.png
智能手机中有自动横屏的功能,同一幅界面会在随着手机(或平板电脑)中的方向传感器的参数不同而改变显示的方向,在界面改变方向后,界面上的图形会因为长宽的变化而产生拉伸,造成图形的失真变形。
我们都知道android平台有多种不同的分辨率,很多控件的切图文件在被放大拉伸后,边
- 算法的效率
天子之骄
算法效率复杂度最坏情况运行时间大O阶平均情况运行时间
算法的效率
效率是速度和空间消耗的度量。集中考虑程序的速度,也称运行时间或执行时间,用复杂度的阶(O)这一标准来衡量。空间的消耗或需求也可以用大O表示,而且它总是小于或等于时间需求。
以下是我的学习笔记:
1.求值与霍纳法则,即为秦九韶公式。
2.测定运行时间的最可靠方法是计数对运行时间有贡献的基本操作的执行次数。运行时间与这个计数成正比。
- java数据结构
何必如此
java数据结构
Java 数据结构
Java工具包提供了强大的数据结构。在Java中的数据结构主要包括以下几种接口和类:
枚举(Enumeration)
位集合(BitSet)
向量(Vector)
栈(Stack)
字典(Dictionary)
哈希表(Hashtable)
属性(Properties)
以上这些类是传统遗留的,在Java2中引入了一种新的框架-集合框架(Collect
- MybatisHelloWorld
3213213333332132
//测试入口TestMyBatis
package com.base.helloworld.test;
import java.io.IOException;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibat
- Java|urlrewrite|URL重写|多个参数
7454103
javaxmlWeb工作
个人工作经验! 如有不当之处,敬请指点
1.0 web -info 目录下建立 urlrewrite.xml 文件 类似如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE u
- 达梦数据库+ibatis
darkranger
sqlmysqlibatisSQL Server
--插入数据方面
如果您需要数据库自增...
那么在插入的时候不需要指定自增列.
如果想自己指定ID列的值, 那么要设置
set identity_insert 数据库名.模式名.表名;
----然后插入数据;
example:
create table zhabei.test(
id bigint identity(1,1) primary key,
nam
- XML 解析 四种方式
aijuans
android
XML现在已经成为一种通用的数据交换格式,平台的无关性使得很多场合都需要用到XML。本文将详细介绍用Java解析XML的四种方法。
XML现在已经成为一种通用的数据交换格式,它的平台无关性,语言无关性,系统无关性,给数据集成与交互带来了极大的方便。对于XML本身的语法知识与技术细节,需要阅读相关的技术文献,这里面包括的内容有DOM(Document Object
- spring中配置文件占位符的使用
avords
1.类
<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.o
- 前端工程化-公共模块的依赖和常用的工作流
bee1314
webpack
题记: 一个人的项目,还有工程化的问题嘛? 我们在推进模块化和组件化的过程中,肯定会不断的沉淀出我们项目的模块和组件。对于这些沉淀出的模块和组件怎么管理?另外怎么依赖也是个问题? 你真的想这样嘛? var BreadCrumb = require(‘../../../../uikit/breadcrumb’); //真心ugly。
- 上司说「看你每天准时下班就知道你工作量不饱和」,该如何回应?
bijian1013
项目管理沟通IT职业规划
问题:上司说「看你每天准时下班就知道你工作量不饱和」,如何回应
正常下班时间6点,只要是6点半前下班的,上司都认为没有加班。
Eno-Bea回答,注重感受,不一定是别人的
虽然我不知道你具体从事什么工作与职业,但是我大概猜测,你是从事一项不太容易出现阶段性成果的工作
- TortoiseSVN,过滤文件
征客丶
SVN
环境:
TortoiseSVN 1.8
配置:
在文件夹空白处右键
选择 TortoiseSVN -> Settings
在 Global ignote pattern 中添加要过滤的文件:
多类型用英文空格分开
*name : 过滤所有名称为 name 的文件或文件夹
*.name : 过滤所有后缀为 name 的文件或文件夹
--------
- 【Flume二】HDFS sink细说
bit1129
Flume
1. Flume配置
a1.sources=r1
a1.channels=c1
a1.sinks=k1
###Flume负责启动44444端口
a1.sources.r1.type=avro
a1.sources.r1.bind=0.0.0.0
a1.sources.r1.port=44444
a1.sources.r1.chan
- The Eight Myths of Erlang Performance
bookjovi
erlang
erlang有一篇guide很有意思: http://www.erlang.org/doc/efficiency_guide
里面有个The Eight Myths of Erlang Performance: http://www.erlang.org/doc/efficiency_guide/myths.html
Myth: Funs are sl
- java多线程网络传输文件(非同步)-2008-08-17
ljy325
java多线程socket
利用 Socket 套接字进行面向连接通信的编程。客户端读取本地文件并发送;服务器接收文件并保存到本地文件系统中。
使用说明:请将TransferClient, TransferServer, TempFile三个类编译,他们的类包是FileServer.
客户端:
修改TransferClient: serPort, serIP, filePath, blockNum,的值来符合您机器的系
- 读《研磨设计模式》-代码笔记-模板方法模式
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
- 配置心得
chenyu19891124
配置
时间就这样不知不觉的走过了一个春夏秋冬,转眼间来公司已经一年了,感觉时间过的很快,时间老人总是这样不停走,从来没停歇过。
作为一名新手的配置管理员,刚开始真的是对配置管理是一点不懂,就只听说咱们公司配置主要是负责升级,而具体该怎么做却一点都不了解。经过老员工的一点点讲解,慢慢的对配置有了初步了解,对自己所在的岗位也慢慢的了解。
做了一年的配置管理给自总结下:
1.改变
从一个以前对配置毫无
- 对“带条件选择的并行汇聚路由问题”的再思考
comsci
算法工作软件测试嵌入式领域模型
2008年上半年,我在设计并开发基于”JWFD流程系统“的商业化改进型引擎的时候,由于采用了新的嵌入式公式模块而导致出现“带条件选择的并行汇聚路由问题”(请参考2009-02-27博文),当时对这个问题的解决办法是采用基于拓扑结构的处理思想,对汇聚点的实际前驱分支节点通过算法预测出来,然后进行处理,简单的说就是找到造成这个汇聚模型的分支起点,对这个起始分支节点实际走的路径数进行计算,然后把这个实际
- Oracle 10g 的clusterware 32位 下载地址
daizj
oracle
Oracle 10g 的clusterware 32位 下载地址
http://pan.baidu.com/share/link?shareid=531580&uk=421021908
http://pan.baidu.com/share/link?shareid=137223&uk=321552738
http://pan.baidu.com/share/l
- 非常好的介绍:Linux定时执行工具cron
dongwei_6688
linux
Linux经过十多年的发展,很多用户都很了解Linux了,这里介绍一下Linux下cron的理解,和大家讨论讨论。cron是一个Linux 定时执行工具,可以在无需人工干预的情况下运行作业,本文档不讲cron实现原理,主要讲一下Linux定时执行工具cron的具体使用及简单介绍。
新增调度任务推荐使用crontab -e命令添加自定义的任务(编辑的是/var/spool/cron下对应用户的cr
- Yii assets目录生成及修改
dcj3sjt126com
yii
assets的作用是方便模块化,插件化的,一般来说出于安全原因不允许通过url访问protected下面的文件,但是我们又希望将module单独出来,所以需要使用发布,即将一个目录下的文件复制一份到assets下面方便通过url访问。
assets设置对应的方法位置 \framework\web\CAssetManager.php
assets配置方法 在m
- mac工作软件推荐
dcj3sjt126com
mac
mac上的Terminal + bash + screen组合现在已经非常好用了,但是还是经不起iterm+zsh+tmux的冲击。在同事的强烈推荐下,趁着升级mac系统的机会,顺便也切换到iterm+zsh+tmux的环境下了。
我为什么要要iterm2
切换过来也是脑袋一热的冲动,我也调查过一些资料,看了下iterm的一些优点:
* 兼容性好,远程服务器 vi 什么的低版本能很好兼
- Memcached(三)、封装Memcached和Ehcache
frank1234
memcachedehcachespring ioc
本文对Ehcache和Memcached进行了简单的封装,这样对于客户端程序无需了解ehcache和memcached的差异,仅需要配置缓存的Provider类就可以在二者之间进行切换,Provider实现类通过Spring IoC注入。
cache.xml
<?xml version="1.0" encoding="UTF-8"?>
- Remove Duplicates from Sorted List II
hcx2013
remove
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.
For example,Given 1->2->3->3->4->4->5,
- Spring4新特性——注解、脚本、任务、MVC等其他特性改进
jinnianshilongnian
spring4
Spring4新特性——泛型限定式依赖注入
Spring4新特性——核心容器的其他改进
Spring4新特性——Web开发的增强
Spring4新特性——集成Bean Validation 1.1(JSR-349)到SpringMVC
Spring4新特性——Groovy Bean定义DSL
Spring4新特性——更好的Java泛型操作API
Spring4新
- MySQL安装文档
liyong0802
mysql
工作中用到的MySQL可能安装在两种操作系统中,即Windows系统和Linux系统。以Linux系统中情况居多。
安装在Windows系统时与其它Windows应用程序相同按照安装向导一直下一步就即,这里就不具体介绍,本文档只介绍Linux系统下MySQL的安装步骤。
Linux系统下安装MySQL分为三种:RPM包安装、二进制包安装和源码包安装。二
- 使用VS2010构建HotSpot工程
p2p2500
HotSpotOpenJDKVS2010
1. 下载OpenJDK7的源码:
http://download.java.net/openjdk/jdk7
http://download.java.net/openjdk/
2. 环境配置
▶
- Oracle实用功能之分组后列合并
seandeng888
oracle分组实用功能合并
1 实例解析
由于业务需求需要对表中的数据进行分组后进行合并的处理,鉴于Oracle10g没有现成的函数实现该功能,且该功能如若用JAVA代码实现会比较复杂,因此,特将SQL语言的实现方式分享出来,希望对大家有所帮助。如下:
表test 数据如下:
ID,SUBJECTCODE,DIMCODE,VALUE
1&nbs
- Java定时任务注解方式实现
tuoni
javaspringjvmxmljni
Spring 注解的定时任务,有如下两种方式:
第一种:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http
- 11大Java开源中文分词器的使用方法和分词效果对比
yangshangchuan
word分词器ansj分词器Stanford分词器FudanNLP分词器HanLP分词器
本文的目标有两个:
1、学会使用11大Java开源中文分词器
2、对比分析11大Java开源中文分词器的分词效果
本文给出了11大Java开源中文分词的使用方法以及分词结果对比代码,至于效果哪个好,那要用的人结合自己的应用场景自己来判断。
11大Java开源中文分词器,不同的分词器有不同的用法,定义的接口也不一样,我们先定义一个统一的接口:
/**
* 获取文本的所有分词结果, 对比