package ccnu.paint;
import java.awt.Color;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Enumeration;
import java.util.Properties;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import ccnu.util.Answer;
import ccnu.util.Verification;
public class Login extends JFrame
{
private static final long serialVersionUID = 1L;
private Properties pro = new Properties();
private boolean ver_code = false;
private Answer answer = null;
private JPanel p1 = new JPanel();
private JLabel luser = new JLabel("username: ");
private JTextField username = new JTextField(20);
private JPanel p2 = new JPanel();
private JLabel lpwd = new JLabel("password: ");
private JPasswordField pwd = new JPasswordField(20);
private JPanel p4 = new JPanel();
private JLabel lVer = new JLabel("verification: ");
private JTextField ver = new JTextField(10);
private JLabel img = new JLabel();
private JLabel result = new JLabel();
private JPanel p3 = new JPanel();
private JButton ok = new JButton("ok");
private JButton cancel = new JButton("cancel");
private JButton signUp = new JButton("Sign up");
public void initListener()
{
username.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
String name = username.getText();
if (name.equals(""))
{
JOptionPane.showMessageDialog(Login.this, "Please input a userName!");
} else
{
pwd.grabFocus();
}
}
});
pwd.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e)
{
String password = new String(pwd.getPassword());
if(password.equalsIgnoreCase(""))
{
JOptionPane.showMessageDialog(Login.this, "please input a password!");
}else{
ver.grabFocus();
}
}
});
ok.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e)
{
try
{
pro.load(new FileInputStream(new File("src/res/accouts.properties")));
} catch (IOException e1)
{
e1.printStackTrace();
}
check();
}
});
ver.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e)
{
String verCode = ver.getText();
if(verCode.equals(""))
{
JOptionPane.showMessageDialog(Login.this, "Please input a verification!");
}else{
if(verCode.equals(answer.getResult()))
{
result.setIcon(new ImageIcon(Login.this.getClass().getResource("/res/right.jpg")));
ver_code = true;
try
{
pro.load(new FileInputStream(new File("src/res/accouts.properties")));
} catch (IOException e1)
{
e1.printStackTrace();
}
check();
}else{
result.setIcon(new ImageIcon(Login.this.getClass().getResource("/res/error.jpg")));
ver_code = false;
}
}
}
});
img.addMouseListener(new MouseAdapter(){
@Override
public void mouseClicked(MouseEvent e)
{
answer = Verification.verification();
img.setIcon(new ImageIcon(answer.getBufferedImage()));
}
});
cancel.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
int option = JOptionPane.showConfirmDialog(Login.this, "Are you sure to exit?");
if (option == 0)
{
Login.this.dispose();
}
}
});
signUp.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e)
{
new SignUp();
}
});
}
public Login()
{
super("Login");
try
{
pro.load(new FileInputStream(new File("src/res/accouts.properties")));
} catch (IOException e)
{
e.printStackTrace();
}
initListener();
answer = Verification.verification();
img.setIcon(new ImageIcon(answer.getBufferedImage()));
this.setLocation(new Point(200, 200));
this.setSize(500, 300);
this.setLayout(new GridLayout(4, 1, 0, 20));
p1.add(luser);
p1.add(username);
p2.add(lpwd);
p2.add(pwd);
p4.add(this.lVer);
p4.add(this.ver);
p4.add(this.img);
result.setForeground(Color.red);
result.setFont(new Font("楷体", Font.BOLD, 20));
p4.add(result);
p3.add(ok);
p3.add(cancel);
p3.add(signUp);
this.add(p1);
this.add(p2);
this.add(p4);
this.add(p3);
this.getContentPane().setBackground(Color.gray);
this.setResizable(false);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void check()
{
String verCode = ver.getText();
if(verCode.equals(""))
{
JOptionPane.showMessageDialog(Login.this, "Please input a verification!");
return;
}else{
if(verCode.equals(answer.getResult()))
{
result.setIcon(new ImageIcon(Login.this.getClass().getResource("/res/right.jpg")));
ver_code = true;
}else{
result.setIcon(new ImageIcon(Login.this.getClass().getResource("/res/error.jpg")));
ver_code = false;
}
}
if(ver_code == false)
{
JOptionPane.showMessageDialog(this, "verification is error!");
return;
}
String name = username.getText();
String password = new String(pwd.getPassword());
if (isPass(name, password))
{
JOptionPane.showMessageDialog(this, "-^_^- OK...");
this.dispose();
} else
{
JOptionPane.showMessageDialog(this, "userName or password is incorrect!");
username.setText("");
pwd.setText("");
ver.setText("");
answer = Verification.verification();
img.setIcon(new ImageIcon(answer.getBufferedImage()));
result.setIcon(null);
}
}
public boolean isPass(String name, String password)
{
Enumeration en = pro.propertyNames();
while(en.hasMoreElements())
{
String curName = (String)en.nextElement();
if(curName.equalsIgnoreCase(name))
{
if(password.equalsIgnoreCase(pro.getProperty(curName)))
{
return true;
}
}
}
return false;
}
public static void main(String[] args)
{
new Login();
}
}
package ccnu.paint;
import java.awt.GridLayout;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Enumeration;
import java.util.Properties;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class SignUp extends JFrame
{
private static final long serialVersionUID = 3054293481122038909L;
private Properties pro = new Properties();
private JPanel panel = new JPanel();
private JLabel label = new JLabel("username: ");
private JTextField field = new JTextField(15);
private JPanel panel2 = new JPanel();
private JLabel label2 = new JLabel("password: ");
private JPasswordField field2 = new JPasswordField(15);
private JPanel panel3 = new JPanel();
private JLabel label3 = new JLabel("confirmation: ");
private JPasswordField field3 = new JPasswordField(15);
private JPanel panel4 = new JPanel();
private JButton button = new JButton("OK");
private JButton button2 = new JButton("Cancel");
public void initListener()
{
field.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
field2.grabFocus();
}
});
field2.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
field3.grabFocus();
}
});
field3.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
ok_actionPerformed(e);
}
});
button.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
ok_actionPerformed(e);
}
});
button2.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
cancel_actionPerformed(e);
}
});
}
public void ok_actionPerformed(ActionEvent e)
{
String userName = field.getText();
String password = new String(field2.getPassword());
String password2 = new String(field3.getPassword());
if (userName.equals(""))
{
JOptionPane.showMessageDialog(SignUp.this, "username cannot be empty!");
} else
{
if (password.equalsIgnoreCase(""))
{
JOptionPane.showMessageDialog(SignUp.this, "password cannot be empty!");
} else
{
if (password2.equalsIgnoreCase(password))
{
if (isExist(userName))
{
JOptionPane.showMessageDialog(SignUp.this, "username has been existed!");
field.setText("");
field2.setText("");
field3.setText("");
} else
{
pro.setProperty(userName, password);
JOptionPane.showMessageDialog(SignUp.this, "SignUp success!");
writeToPro(userName, password);
SignUp.this.dispose();
}
} else
{
JOptionPane.showMessageDialog(SignUp.this, "password is not consistent!");
field2.setText("");
field3.setText("");
}
}
}
}
public void cancel_actionPerformed(ActionEvent e)
{
System.exit(0);
}
public SignUp()
{
super("Sign up");
try
{
pro.load(new FileInputStream(new File("src/res/accouts.properties")));
} catch (IOException e)
{
e.printStackTrace();
}
initListener();
this.setLocation(new Point(300, 230));
this.setSize(280, 210);
this.setLayout(new GridLayout(4, 1, 0, 20));
panel.add(label);
panel.add(field);
panel2.add(label2);
panel2.add(field2);
panel3.add(label3);
panel3.add(field3);
panel4.add(button);
panel4.add(button2);
this.add(panel);
this.add(panel2);
this.add(panel3);
this.add(panel4);
this.setAlwaysOnTop(true);
this.setResizable(false);
this.setVisible(true);
}
public void writeToPro(String userName, String password)
{
pro.setProperty(userName, password);
try
{
pro.store(new FileOutputStream(new File("src/res/accouts.properties")), "allAccouts");
} catch (IOException e)
{
e.printStackTrace();
}
}
public boolean isExist(String userName)
{
Enumeration enumer = pro.propertyNames();
while (enumer.hasMoreElements())
{
String temp = (String) enumer.nextElement();
if (temp.equals(userName))
{
return true;
}
}
return false;
}
}
package ccnu.util
import java.awt.Color
import java.awt.Font
import java.awt.Graphics
import java.awt.Point
import java.awt.image.BufferedImage
import java.util.Random
// 用于生成验证码
public class Verification
{
private static Answer answer = new Answer()
private static BufferedImage bufferedImage = null
private static String result = null
private static String words = null
private static String words2 = null
// 生成验证码
public static Answer verification()
{
bufferedImage = new BufferedImage(200, 35, BufferedImage.TYPE_INT_RGB)
Graphics g = bufferedImage.getGraphics()
Random rand = new Random()
for (int i = 0
{
Point p1 = new Point(rand.nextInt(200), rand.nextInt(30))
Point p2 = new Point(rand.nextInt(200), rand.nextInt(30))
g.drawLine(p1.x, p1.y, p2.x, p2.y)
}
g.setColor(Color.RED)
g.setFont(new Font("楷体", Font.BOLD, 22))
int plan = 2
switch (rand.nextInt(plan))
{
case 0:
plan(g)
break
case 1:
plan1(g)
break
default:
break
}
answer.setBufferedImage(bufferedImage)
answer.setResult(result)
g.dispose()
return answer
}
// 方案一
private static void plan(Graphics g)
{
words = ReadTxt.read("/res/words.txt")
Random rand = new Random()
String first = String.valueOf(words.charAt(rand.nextInt(words.length())))
String second = String.valueOf(words.charAt(rand.nextInt(words.length())))
String third = String.valueOf(words.charAt(rand.nextInt(words.length())))
g.drawString(first, rand.nextInt(40) + 20, rand.nextInt(12) + 15)
g.drawString(second, rand.nextInt(40) + 80, rand.nextInt(12) + 15)
g.drawString(third, rand.nextInt(40) + 140, rand.nextInt(12) + 15)
result = first + second + third
}
// 方案二
private static void plan1(Graphics g)
{
words2 = ReadTxt.read("/res/words2.txt")
Random rand = new Random()
String first = String.valueOf(words2.charAt(rand.nextInt(words2.length() - 2)))
String second = String.valueOf(words2.charAt(rand.nextInt(2) + 9))
String third = String.valueOf(words2.charAt(rand.nextInt(words2.length() - 2)))
g.drawString(first, rand.nextInt(30) + 20, rand.nextInt(12) + 15)
g.drawString(second, rand.nextInt(40) + 60, rand.nextInt(12) + 15)
g.drawString(third, rand.nextInt(30) + 110, rand.nextInt(12) + 15)
g.drawString("=", rand.nextInt(40) + 150, rand.nextInt(12) + 15)
if(second.equals("+"))
{
result = String.valueOf(Integer.valueOf(first) + Integer.valueOf(third))
}else{
result = String.valueOf(Integer.valueOf(first) - Integer.valueOf(third))
}
}
}
package ccnu.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class ReadTxt
{
public static String read(String path) // 根据指定路径path来读取它,并返回它所包含的内容
{
StringBuffer sb = new StringBuffer();
try
{
BufferedReader br = new BufferedReader(new InputStreamReader(Verification.class.getResourceAsStream(path)));
String temp = null;
while(null != (temp = br.readLine()))
{
sb.append(temp);
}
br.close();
} catch (IOException e)
{
e.printStackTrace();
}
return sb.toString();
}
}
package ccnu.util;
import java.awt.image.BufferedImage;
public class Answer
{
private BufferedImage bufferedImage = null;
private String result = null;
public BufferedImage getBufferedImage()
{
return bufferedImage;
}
public void setBufferedImage(BufferedImage bufferedImage)
{
this.bufferedImage = bufferedImage;
}
public String getResult()
{
return result;
}
public void setResult(String result)
{
this.result = result;
}
}
- 验证码生成汉字识别的问题的文件words.txt
如: 中国湖北省武汉市汉东大学政法学院
- 验证码生成算术运算的问题的文件words2.txt
123456789+-
提示图片

登录效果
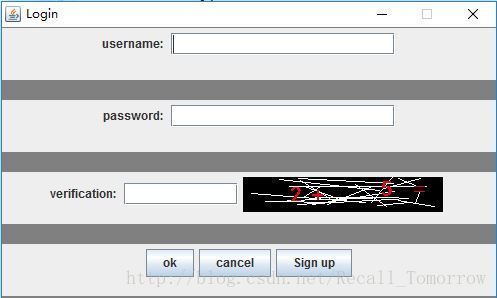