- 中科院C语言应聘机试编程题6,中科院计算所保研笔试+机试+面试经验分享
力气气
中科院C语言应聘机试编程题6
计算所JDL(先进人机交互)实验室9月10号开始联系计算所导师,12号收到导师的回复,大致意思是老师让我提供三位本校推荐老师的联系方式,又问了是否有读博的打算,让我准备到计算所JDL面试,16号收到了他们的正式通知。老实说我这次的复试基本属于裸面,时间很仓促,后来才知道很多同学在暑假之前就开始联系导师了,提前见了导师,咨询清楚了复试内容,暑假有针对性性地复习了一遍。这样的话录取的可能性就很大了,所
- c语言编程机试题及答案,C语言机试题库50道编程题答案(C. The answer of 50 programming questions).doc...
微小蓝
c语言编程机试题及答案
C语言机试题库50道编程题答案(C.Theanswerof50programmingquestions)C语言机试题库50道编程题答案(C.Theanswerof50programmingquestions)1.doublefun(strec*a,strec*b,int*n){inti,j;doubleave,sum=0.0;*n=0;for(i=0;i=ave){b[j]=a[i];j++;(*
- 使用Idea创建springboot项目
奔跑吧邓邓子
SpringBoot深入浅出常见问题解答(FAQ)高效运维javaidea
提示:“奔跑吧邓邓子”的高效运维专栏聚焦于各类运维场景中的实际操作与问题解决。内容涵盖服务器硬件(如IBMSystem3650M5)、云服务平台(如腾讯云、华为云)、服务器软件(如Nginx、Apache、GitLab、Redis、Elasticsearch、Kubernetes、Docker等)、开发工具(如Git、HBuilder)以及网络安全(如挖矿病毒排查、SSL证书配置)等多个方面。无论
- java计算机毕业设计企业销售管理系统源代码+数据库+系统+lw文档
今晚的月亮真好看
java开发语言
java计算机毕业设计企业销售管理系统源代码+数据库+系统+lw文档java计算机毕业设计企业销售管理系统源代码+数据库+系统+lw文档本源码技术栈:项目架构:B/S架构开发语言:Java语言开发软件:ideaeclipse前端技术:Layui、HTML、CSS、JS、JQuery等技术后端技术:JAVA运行环境:Win10、JDK1.8数据库:MySQL5.7/8.0源码地址:https://p
- [特殊字符]【CVPR2024新突破】Logit标准化:知识蒸馏中的自适应温度革命[特殊字符]
☞黑心萝卜三条杠☜
论文人工智能论文阅读
文章信息题目:LogitStandardizationinKnowledgeDistillation论文地址:paper代码地址:code年份:2024年发表于CVPR文章主题文章的核心目标是改进知识蒸馏(KD)中的一个关键问题:传统KD方法假设教师和学生模型共享一个全局温度参数(temperature),这导致学生模型需要精确匹配教师模型的logit范围和方差。这种假设不仅限制了学生模型的性能,
- 【学习】电脑上有多个GPU,命令行指定GPU进行训练。
超好的小白
学习人工智能深度学习
使用如下指令可以指定使用的GPU。CUDA_VISIBLE_DEVICES=1假设要使用第二个GPU进行训练。CUDA_VISIBLE_DEVICES=1pythontrain.py
- SCP传输
超好的小白
vscode+服务器python
scp-P10022yolo_world.taruser@***.88.148.***:/
[email protected]:/home/admin1.将本地文件复制到远程scp/home/data/file.zipusername@remote_host:/home/username/2.将远程文件复制到本地scpusername@rem
- React + TypeScript 实现 SQL 脚本生成全栈实践
i建模
数据建模数据管理前端开发数学建模
React+TypeScript实现数据模型驱动SQL脚本生成全栈实践引言:数据模型与SQL的桥梁革命在现代化全栈开发中,数据模型与数据库的精准映射已成为提升开发效率的关键。传统手动编写SQL脚本的方式存在模式漂移风险高(SchemaDrift)和维护成本大两大痛点。本文将结合React+TypeScript技术栈,解析如何构建智能化的SQL脚本生成系统,并给出2025年最新企业级解决方案。一、技
- VUE实现日历记录事件
yu_zheng5163
vue.jsjavascriptecmascript
el-calendarHTML代码段可以根据自己的需要显示内容,样式-1?'lar-is-selected':'lar-no-selected'"@click="holidayUpdate(data,date)">班-1&&queryDate.indexOf(data.day)!=-1"style="color:#f73131">休 -1?'color:#F73131;text-alig
- 用idea创建低版本springboot2.X的项目
詹皇wm
问题总结intellij-ideajava
文章目录我的环境:创建项目:进入创建项目页面:进入Springboot版本选择和添加依赖页面进入项目打开pom.xml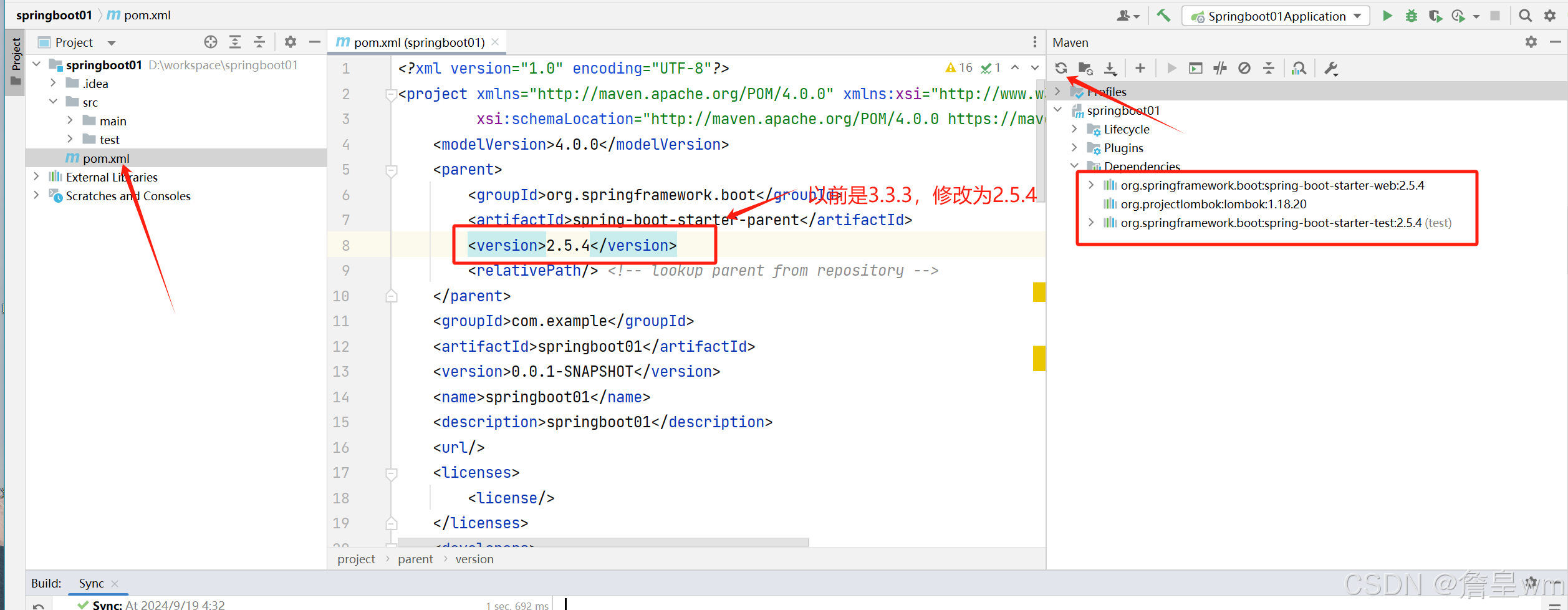*补充*_本文章主要是对于现在idea配置大部分都是自带SpringBoot3.X,怎么创建低版本springboo
- 若依导出PDF
yu_zheng5163
pdfwindows
后端/***导出PDF*@paramphysicalExamination*@paramresponse*@throwsException*/@GetMapping("/exportPDF")publicvoidexportPDF(PhysicalExaminationphysicalExamination,HttpServletResponseresponse)throwsException{/
- 搜索条件下拉展示树状结构
yu_zheng5163
前端javascripthtml
取消确定exportdefault{data(){return{selectedDepartIds:[],selectedOptions:[],dialogVisible:false,treeData:[//树形数据示例{id:1,label:'公司A',children:[{id:2,label:'部门A1'},{id:3,label:'部门A2'},],},{id:4,label:'公司B',
- 【Golang】Go语言Web开发之模板渲染
景天科技苑
Go语言开发零基础到高阶实战golang前端开发语言Go语言模板渲染模板渲染golang模板渲染
✨✨欢迎大家来到景天科技苑✨✨养成好习惯,先赞后看哦~作者简介:景天科技苑《头衔》:大厂架构师,华为云开发者社区专家博主,阿里云开发者社区专家博主,CSDN全栈领域优质创作者,掘金优秀博主,51CTO博客专家等。《博客》:Python全栈,Golang开发,PyQt5和Tkinter桌面开发,小程序开发,人工智能,js逆向,App逆向,网络系统安全,数据分析,Django,fastapi,flas
- 【第八节】C++设计模式(结构型模式)-Decorator(装饰器)模式
攻城狮7号
c++版本设计模式c++设计模式装饰器模式
目录一、问题引出二、模式选择三、代码实现四、总结讨论一、问题引出装饰器模式:动态扩展对象功能的设计模式在面向对象(OO)设计与开发中,我们常面临为已有类添加新职责的需求。传统方法是通过继承创建子类来实现功能扩展,但这种方式容易导致继承层次过深,显著增加系统复杂度。装饰器模式(DecoratorPattern)应运而生,其通过组合替代继承的机制,为功能扩展提供了更灵活的解决方案,从而避免了继承层次过
- Springboot 整合 Java DL4J 实现企业门禁人脸识别系统
伏羲栈
人工智能深度学习JavaDL4J-深度学习实战springbootjavaDeeplearning4jdeeplearning人工智能深度学习spring
博主简介:历代文学网(PC端可以访问:https://literature.sinhy.com/#/literature?__c=1000,移动端可微信小程序搜索“历代文学”)总架构师,15年工作经验,精通Java编程,高并发设计,Springboot和微服务,熟悉Linux,ESXI虚拟化以及云原生Docker和K8s,热衷于探索科技的边界,并将理论知识转化为实际应用。保持对新技术的好奇心,乐于
- Redis---LRU原理与算法实现
lh_freak
redis算法数据库
文章目录LRU概念理解LRU原理基于HashMap和双向链表实现LRURedis中的LRU的实现LRU时钟淘汰策略近似LRU的实现LRU算法的优化RedisLRU的核心代码逻辑RedisLRU的核心代码逻辑RedisLRU的配置参数RedisLRU的优缺点RedisLRU的优缺点LRU概念理解LRU(LeastRecentlyUsed)最近最少使用算法,是一种常用的页面置换算法,广泛应用于操作系统
- HTML---css选择器
lh_freak
htmlcss前端
CSS指层叠样式表,是一种用来为结构化文档(如HTML文档或XML应用)添加样式(字体、间距和颜色等)的计算机语言,CSS文件扩展名为.css。使用CSS我们可以大大提升网页开发的工作效率!插入样式表的方法有三种:外部样式(Externalstylesheet)内部样式(Internalstylesheet)内联样式(Inlinestyle)内联样式(行内样式)hello样式直接写入到本元素标签中
- spark为什么比mapreduce快?
京东云开发者
sparkmapreduce大数据
作者:京东零售吴化斌spark为什么比mapreduce快?首先澄清几个误区:1:两者都是基于内存计算的,任何计算框架都肯定是基于内存的,所以网上说的spark是基于内存计算所以快,显然是错误的2;DAG计算模型减少的是磁盘I/O次数(相比于mapreduce计算模型而言),而不是shuffle次数,因为shuffle是根据数据重组的次数而定,所以shuffle次数不能减少所以总结spark比ma
- 程序员未来的出路:行业趋势与职业发展分析
guzhoumingyue
AIpython
随着技术的发展和行业需求的变化,程序员的职业出路也在不断演变。以下是程序员未来可能的职业发展方向及具体建议:一、技术深耕路线AI与机器学习专家趋势:AI技术在各行业的应用日益广泛,从自动驾驶到智能客服,需求持续增长。技能要求:Python、TensorFlow、PyTorch、数据挖掘、算法优化。发展路径:从机器学习工程师做起,积累项目经验。深入研究深度学习、强化学习等前沿技术。成为AI架构师或数
- 编程参考 - Switch Case Range in GNU C Extensions
夜流冰
编程参考gnu
ExtensionstotheCLanguageFamilyCaseRanges(UsingtheGNUCompilerCollection(GCC))6.32CaseRangesYoucanspecifyarangeofconsecutivevaluesinasinglecaselabel,likethis:caselow...high:Thishasthesameeffectastheprop
- 谈一谈无服务架构降本增效
fxrz12
架构运维云计算serverless无服务器
在当今数字化转型的浪潮中,企业不断寻求创新的方法来优化IT基础设施,降低运营成本并提升业务效率。无服务架构(ServerlessArchitecture)作为一种新兴的计算模式,正在成为众多企业的首选解决方案。本文将探讨无服务架构如何帮助企业实现降本增效,并通过图表对比无服务架构和常规架构。什么是无服务架构?无服务架构是一种云计算执行模型,开发者可以部署代码而无需管理服务器。云服务提供商(如AWS
- 黑客工具介绍
嗨起飞了
网络安全网络安全网络攻击模型
渗透测试红队工具箱深度解析:6大核心工具实战指南法律声明:本文所有工具及技术仅限用于合法授权的安全测试,使用者需遵守《网络安全法》及相关法律法规,擅自攻击他人系统将承担刑事责任。一、Nmap:网络侦察的全能之眼1.1工具原理剖析Nmap(NetworkMapper)采用TCP/IP协议栈指纹识别技术,通过发送定制化数据包分析响应差异,精准识别主机存活状态、开放端口及服务版本。其脚本引擎(NSE)支
- 统计用户输入 C语言
2501_90645732
c语言
从键盘读取用户输入直到遇到#字符,编写程序统计读取的空格数目、读取的换行符数目以及读取的所有其他字符数目。(要求用getchar()输入字符)#includeintmain(){printf("Pleaseinputastringendby#:\n");intc;intspaces=0;intnewlines=0;intother=0;while((c=getchar())!='#'){if(c=
- 解锁Java在客户旅程映射中的无限潜力:从数据收集到优化的全方位指南
墨夶
Java学习资料2javapython开发语言
在当今竞争激烈的市场环境中,了解并优化客户的旅程成为企业成功的关键。通过客户旅程映射(CustomerJourneyMapping,CJM),企业能够识别出客户在与品牌互动过程中遇到的痛点,并据此改进服务。而Java作为一门强大的编程语言,其灵活性和广泛的应用场景使其成为实现这些目标的理想选择。本文将深入探讨如何使用Java进行客户旅程的分析与优化,并提供详尽的代码示例和最佳实践。第一部分:理解客
- 【go语言】复杂数据类型——切片Slice
2302_79952574
golanggolang学习开发语言
1.理解切片Slice(1)切片是什么?切片是数组的一个引用,因此是引用类型。slice通过内部指针和相关属性引用数组片段,以实现变长方案。切片Slice包含三个部分:指向底层数组的指针。切片的长度(len):表示切片当前可见的元素个数。切片的容量(cap):表示从切片起始位置到底层数组末尾的最大可用元素数量。(2)内存布局Go的切片在内存中的结构可以用以下类型表示:typeSliceHeader
- JDBC Java连接数据库
nqqcat~
javaidea
1.准备下载jar——QQ群mysql-connect-java新建工程及目录添加驱动:把jar包添加到项目中,并添加到类库新建数据库新建表2.编写代码目标:创建链接打通连接四个参数驱动;URL;用户名;密码五个步骤加载驱动;创建连接;创建Statement;执行SQL;关闭,释放资源packagecom.wdzl.demo01;importjava.sql.Connection;importja
- MFC—加法器
nqqcat~
MFCmfcc++
1.需要为编辑框添加变量2.在cpp文件中的按钮中添加代码voidCMFCAddtionDlg::OnBnClickedButton1(){//TODO:在此添加控件通知处理程序代码UpdateData(true);//把控件里的值更新给变量m_add=m_add1+m_add2;//加法UpdateData(false);//把控件相加的值赋值给控件}
- 栈的应用(插入一个元素,删除栈顶元素,输出栈元素)数据结构
nqqcat~
数据结构数据结构
一、实验目的:1、掌握栈的特点(先进后出FILO)及基本操作,如入栈、出栈等。2、利用栈的特点解决实际问题,提高编程能力。二、实验内容编程实现顺序栈的各种基本运算,并在此基础上设计一个主程序,完成如下功能:1、初始化顺序栈;2、给定一个元素,将此元素压入此栈中;3、将栈顶一个元素弹出此栈。三、源程序#include#includetypedefintelemtype;#definemaxsize3
- 终端应用开发沉思录
焦糖酒
科技分享javascript前端框架
前言以下所有分析皆是从我的视角出发,探讨下我现行局势下觉得最有可能的实现且有未来发展前景的技术方案。由于本人没有啥开发经验,所以多是纸上谈兵,仅仅记录和分享下我个人想法。移动App的开发模式:在技术选型上,其实好久没这么犹豫过了,最近几天学到ReactNative,但迟迟没有全身心投入,就是在疑虑其和市面上的其他技术相比是否值得学习。目前移动应用开发有以下三条主要道路(原生H5混合)外加一个国内特
- python的pip如何升级
一代码动乾坤
pythonpythonpip开发语言
升级pip的方法如下:打开命令行工具。在Windows系统中,可以通过按下Win+R键,然后输入"cmd"来打开命令提示符;在Mac或Linux系统中,可以直接打开终端。检查当前pip版本。在终端或命令行中输入以下命令:pip--version,这将显示当前安装的版本号。升级pip。使用以下命令来升级pip:pipinstall--upgradepip,这将会检查并安装最新版本的pip,如果已经
- 怎么样才能成为专业的程序员?
cocos2d-x小菜
编程PHP
如何要想成为一名专业的程序员?仅仅会写代码是不够的。从团队合作去解决问题到版本控制,你还得具备其他关键技能的工具包。当我们询问相关的专业开发人员,那些必备的关键技能都是什么的时候,下面是我们了解到的情况。
关于如何学习代码,各种声音很多,然后很多人就被误导为成为专业开发人员懂得一门编程语言就够了?!呵呵,就像其他工作一样,光会一个技能那是远远不够的。如果你想要成为
- java web开发 高并发处理
BreakingBad
javaWeb并发开发处理高
java处理高并发高负载类网站中数据库的设计方法(java教程,java处理大量数据,java高负载数据) 一:高并发高负载类网站关注点之数据库 没错,首先是数据库,这是大多数应用所面临的首个SPOF。尤其是Web2.0的应用,数据库的响应是首先要解决的。 一般来说MySQL是最常用的,可能最初是一个mysql主机,当数据增加到100万以上,那么,MySQL的效能急剧下降。常用的优化措施是M-S(
- mysql批量更新
ekian
mysql
mysql更新优化:
一版的更新的话都是采用update set的方式,但是如果需要批量更新的话,只能for循环的执行更新。或者采用executeBatch的方式,执行更新。无论哪种方式,性能都不见得多好。
三千多条的更新,需要3分多钟。
查询了批量更新的优化,有说replace into的方式,即:
replace into tableName(id,status) values
- 微软BI(3)
18289753290
微软BI SSIS
1)
Q:该列违反了完整性约束错误;已获得 OLE DB 记录。源:“Microsoft SQL Server Native Client 11.0” Hresult: 0x80004005 说明:“不能将值 NULL 插入列 'FZCHID',表 'JRB_EnterpriseCredit.dbo.QYFZCH';列不允许有 Null 值。INSERT 失败。”。
A:一般这类问题的存在是
- Java中的List
g21121
java
List是一个有序的 collection(也称为序列)。此接口的用户可以对列表中每个元素的插入位置进行精确地控制。用户可以根据元素的整数索引(在列表中的位置)访问元素,并搜索列表中的元素。
与 set 不同,列表通常允许重复
- 读书笔记
永夜-极光
读书笔记
1. K是一家加工厂,需要采购原材料,有A,B,C,D 4家供应商,其中A给出的价格最低,性价比最高,那么假如你是这家企业的采购经理,你会如何决策?
传统决策: A:100%订单 B,C,D:0%
&nbs
- centos 安装 Codeblocks
随便小屋
codeblocks
1.安装gcc,需要c和c++两部分,默认安装下,CentOS不安装编译器的,在终端输入以下命令即可yum install gccyum install gcc-c++
2.安装gtk2-devel,因为默认已经安装了正式产品需要的支持库,但是没有安装开发所需要的文档.yum install gtk2*
3. 安装wxGTK
yum search w
- 23种设计模式的形象比喻
aijuans
设计模式
1、ABSTRACT FACTORY—追MM少不了请吃饭了,麦当劳的鸡翅和肯德基的鸡翅都是MM爱吃的东西,虽然口味有所不同,但不管你带MM去麦当劳或肯德基,只管向服务员说“来四个鸡翅”就行了。麦当劳和肯德基就是生产鸡翅的Factory 工厂模式:客户类和工厂类分开。消费者任何时候需要某种产品,只需向工厂请求即可。消费者无须修改就可以接纳新产品。缺点是当产品修改时,工厂类也要做相应的修改。如:
- 开发管理 CheckLists
aoyouzi
开发管理 CheckLists
开发管理 CheckLists(23) -使项目组度过完整的生命周期
开发管理 CheckLists(22) -组织项目资源
开发管理 CheckLists(21) -控制项目的范围开发管理 CheckLists(20) -项目利益相关者责任开发管理 CheckLists(19) -选择合适的团队成员开发管理 CheckLists(18) -敏捷开发 Scrum Master 工作开发管理 C
- js实现切换
百合不是茶
JavaScript栏目切换
js主要功能之一就是实现页面的特效,窗体的切换可以减少页面的大小,被门户网站大量应用思路:
1,先将要显示的设置为display:bisible 否则设为none
2,设置栏目的id ,js获取栏目的id,如果id为Null就设置为显示
3,判断js获取的id名字;再设置是否显示
代码实现:
html代码:
<di
- 周鸿祎在360新员工入职培训上的讲话
bijian1013
感悟项目管理人生职场
这篇文章也是最近偶尔看到的,考虑到原博客发布者可能将其删除等原因,也更方便个人查找,特将原文拷贝再发布的。“学东西是为自己的,不要整天以混的姿态来跟公司博弈,就算是混,我觉得你要是能在混的时间里,收获一些别的有利于人生发展的东西,也是不错的,看你怎么把握了”,看了之后,对这句话记忆犹新。 &
- 前端Web开发的页面效果
Bill_chen
htmlWebMicrosoft
1.IE6下png图片的透明显示:
<img src="图片地址" border="0" style="Filter.Alpha(Opacity)=数值(100),style=数值(3)"/>
或在<head></head>间加一段JS代码让透明png图片正常显示。
2.<li>标
- 【JVM五】老年代垃圾回收:并发标记清理GC(CMS GC)
bit1129
垃圾回收
CMS概述
并发标记清理垃圾回收(Concurrent Mark and Sweep GC)算法的主要目标是在GC过程中,减少暂停用户线程的次数以及在不得不暂停用户线程的请夸功能,尽可能短的暂停用户线程的时间。这对于交互式应用,比如web应用来说,是非常重要的。
CMS垃圾回收针对新生代和老年代采用不同的策略。相比同吞吐量垃圾回收,它要复杂的多。吞吐量垃圾回收在执
- Struts2技术总结
白糖_
struts2
必备jar文件
早在struts2.0.*的时候,struts2的必备jar包需要如下几个:
commons-logging-*.jar Apache旗下commons项目的log日志包
freemarker-*.jar  
- Jquery easyui layout应用注意事项
bozch
jquery浏览器easyuilayout
在jquery easyui中提供了easyui-layout布局,他的布局比较局限,类似java中GUI的border布局。下面对其使用注意事项作简要介绍:
如果在现有的工程中前台界面均应用了jquery easyui,那么在布局的时候最好应用jquery eaysui的layout布局,否则在表单页面(编辑、查看、添加等等)在不同的浏览器会出
- java-拷贝特殊链表:有一个特殊的链表,其中每个节点不但有指向下一个节点的指针pNext,还有一个指向链表中任意节点的指针pRand,如何拷贝这个特殊链表?
bylijinnan
java
public class CopySpecialLinkedList {
/**
* 题目:有一个特殊的链表,其中每个节点不但有指向下一个节点的指针pNext,还有一个指向链表中任意节点的指针pRand,如何拷贝这个特殊链表?
拷贝pNext指针非常容易,所以题目的难点是如何拷贝pRand指针。
假设原来链表为A1 -> A2 ->... -> An,新拷贝
- color
Chen.H
JavaScripthtmlcss
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <HTML> <HEAD>&nbs
- [信息与战争]移动通讯与网络
comsci
网络
两个坚持:手机的电池必须可以取下来
光纤不能够入户,只能够到楼宇
建议大家找这本书看看:<&
- oracle flashback query(闪回查询)
daizj
oracleflashback queryflashback table
在Oracle 10g中,Flash back家族分为以下成员:
Flashback Database
Flashback Drop
Flashback Table
Flashback Query(分Flashback Query,Flashback Version Query,Flashback Transaction Query)
下面介绍一下Flashback Drop 和Flas
- zeus持久层DAO单元测试
deng520159
单元测试
zeus代码测试正紧张进行中,但由于工作比较忙,但速度比较慢.现在已经完成读写分离单元测试了,现在把几种情况单元测试的例子发出来,希望有人能进出意见,让它走下去.
本文是zeus的dao单元测试:
1.单元测试直接上代码
package com.dengliang.zeus.webdemo.test;
import org.junit.Test;
import o
- C语言学习三printf函数和scanf函数学习
dcj3sjt126com
cprintfscanflanguage
printf函数
/*
2013年3月10日20:42:32
地点:北京潘家园
功能:
目的:
测试%x %X %#x %#X的用法
*/
# include <stdio.h>
int main(void)
{
printf("哈哈!\n"); // \n表示换行
int i = 10;
printf
- 那你为什么小时候不好好读书?
dcj3sjt126com
life
dady, 我今天捡到了十块钱, 不过我还给那个人了
good girl! 那个人有没有和你讲thank you啊
没有啦....他拉我的耳朵我才把钱还给他的, 他哪里会和我讲thank you
爸爸, 如果地上有一张5块一张10块你拿哪一张呢....
当然是拿十块的咯...
爸爸你很笨的, 你不会两张都拿
爸爸为什么上个月那个人来跟你讨钱, 你告诉他没
- iptables开放端口
Fanyucai
linuxiptables端口
1,找到配置文件
vi /etc/sysconfig/iptables
2,添加端口开放,增加一行,开放18081端口
-A INPUT -m state --state NEW -m tcp -p tcp --dport 18081 -j ACCEPT
3,保存
ESC
:wq!
4,重启服务
service iptables
- Ehcache(05)——缓存的查询
234390216
排序ehcache统计query
缓存的查询
目录
1. 使Cache可查询
1.1 基于Xml配置
1.2 基于代码的配置
2 指定可搜索的属性
2.1 可查询属性类型
2.2 &
- 通过hashset找到数组中重复的元素
jackyrong
hashset
如何在hashset中快速找到重复的元素呢?方法很多,下面是其中一个办法:
int[] array = {1,1,2,3,4,5,6,7,8,8};
Set<Integer> set = new HashSet<Integer>();
for(int i = 0
- 使用ajax和window.history.pushState无刷新改变页面内容和地址栏URL
lanrikey
history
后退时关闭当前页面
<script type="text/javascript">
jQuery(document).ready(function ($) {
if (window.history && window.history.pushState) {
- 应用程序的通信成本
netkiller.github.com
虚拟机应用服务器陈景峰netkillerneo
应用程序的通信成本
什么是通信
一个程序中两个以上功能相互传递信号或数据叫做通信。
什么是成本
这是是指时间成本与空间成本。 时间就是传递数据所花费的时间。空间是指传递过程耗费容量大小。
都有哪些通信方式
全局变量
线程间通信
共享内存
共享文件
管道
Socket
硬件(串口,USB) 等等
全局变量
全局变量是成本最低通信方法,通过设置
- 一维数组与二维数组的声明与定义
恋洁e生
二维数组一维数组定义声明初始化
/** * */ package test20111005; /** * @author FlyingFire * @date:2011-11-18 上午04:33:36 * @author :代码整理 * @introduce :一维数组与二维数组的初始化 *summary: */ public c
- Spring Mybatis独立事务配置
toknowme
mybatis
在项目中有很多地方会使用到独立事务,下面以获取主键为例
(1)修改配置文件spring-mybatis.xml <!-- 开启事务支持 --> <tx:annotation-driven transaction-manager="transactionManager" /> &n
- 更新Anadroid SDK Tooks之后,Eclipse提示No update were found
xp9802
eclipse
使用Android SDK Manager 更新了Anadroid SDK Tooks 之后,
打开eclipse提示 This Android SDK requires Android Developer Toolkit version 23.0.0 or above, 点击Check for Updates
检测一会后提示 No update were found