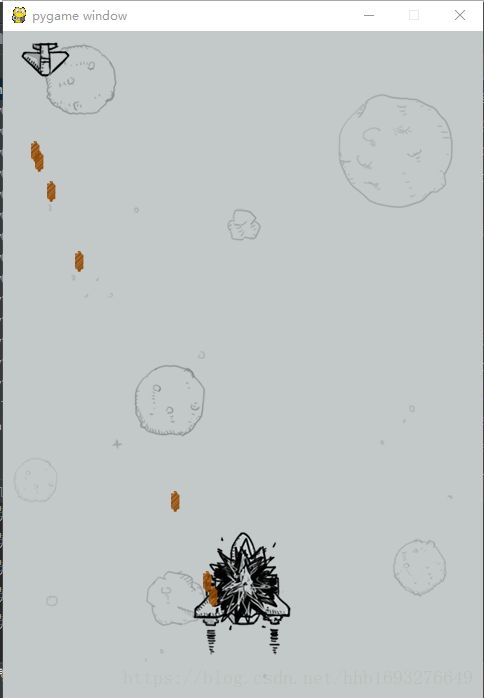
import pygame
from pygame.locals import *
import time
import random
bomb_flag = 0
class Plane(object):
def __init__(self,screen,image_path,x,y):
self.screen = screen
self.x = x
self.y = y
self.image = pygame.image.load(image_path)
self.bullet_list = []
def display(self):
self.screen.blit(self.image,(self.x,self.y))
bullet_list_remove = []
for bullet in self.bullet_list:
bullet.display()
bullet.move()
if bullet.judge():
bullet_list_remove.append(bullet)
for i in bullet_list_remove:
self.bullet_list.remove(i)
class HeroPlane(Plane):
def __init__(self,screen):
image_path = 'feiji/hero1.png'
super(HeroPlane, self).__init__(screen,image_path,190,500)
self.bomb_image_list = []
self.__get_bomb_image()
self.isbomb = False
self.image_num = 0
self.image_index = 0
def display(self):
if self.isbomb:
bomb_image = self.bomb_image_list[self.image_index]
self.screen.blit(bomb_image,(self.x,self.y))
self.image_num += 1
if self.image_num ==(self.image_length + 1):
self.image_num = 0
self.image_index += 1
if self.image_index > (self.image_length-1):
self.image_index = 5
time.sleep(2)
exit()
else:
Plane.display(self)
for bullet in self.bullet_list:
if bullet.judge_jizhong(self.enemy):
self.enemy.bomb(True)
def bomb(self, isbomb):
self.isbomb = isbomb
def __get_bomb_image(self):
for i in range(1, 4):
im_path = 'feiji/hero_blowup_n' + str(i) + '.png'
self.bomb_image_list.append(pygame.image.load(im_path))
self.image_length = len(self.bomb_image_list)
def send_bullet(self,enemy):
self.enemy = enemy
self.bullet_list.append(Bullet(self.screen,self.x,self.y))
def move_left(self):
self.x -= 5
def move_right(self):
self.x += 5
def move_up(self):
self.y -= 5
def move_down(self):
self.y += 5
class EnemyPlane(Plane):
def __init__(self,screen):
image_path = 'feiji/enemy0.png'
self.direction = 'right'
super(EnemyPlane, self).__init__(screen,image_path,10,10)
self.bomb_image_list = []
self.__get_bomb_image()
self.isbomb = False
self.image_num = 0
self.image_index = 0
def bomb(self,isbomb):
self.isbomb = isbomb
def __get_bomb_image(self):
for i in range(1,4):
im_path = 'feiji/enemy0_down' + str(i) + '.png'
self.bomb_image_list.append(pygame.image.load(im_path))
self.image_length = len(self.bomb_image_list)
def display(self):
if self.isbomb:
bomb_image = self.bomb_image_list[self.image_index]
self.screen.blit(bomb_image,(self.x,self.y))
self.image_num += 1
if self.image_num ==(self.image_length + 1):
self.image_num = 0
self.image_index += 1
if self.image_index > (self.image_length-1):
self.image_index = 5
time.sleep(2)
exit()
else:
Plane.display(self)
for bullet in self.bullet_list:
if bullet.judge_jizhong(self.hero):
self.hero.bomb(True)
def send_bullet(self,hero):
self.hero = hero
random_num = random.randint(1,100)
if random_num == 10 or random_num == 20:
self.bullet_list.append(EnemyBullet(self.screen,self.x,self.y))
def move(self):
if self.direction == 'right':
self.x += 2
elif self.direction == 'left':
self.x -= 2
if self.x > 480-51:
self.direction = 'left'
elif self.x < 0:
self.direction = 'right'
class BaseBullet(object):
def __init__(self,screen,image_path,x,y):
self.screen = screen
self.image = pygame.image.load(image_path)
self.x = x
self.y = y
def display(self):
self.screen.blit(self.image,(self.x,self.y))
class EnemyBullet(BaseBullet):
def __init__(self,screen,x,y):
image_path = 'feiji/bullet1.png'
super(EnemyBullet, self).__init__(screen,image_path,x + 20, y + 30)
def judge_jizhong(self,hero):
if self.x > hero.x and self.x < hero.x + 50:
if self.y > hero.y and self.y < hero.y + 20:
print('英雄死亡')
return True
else:
return False
def move(self):
self.y += 5
def judge(self):
if self.y > 700:
return True
else:
return False
class Bullet(BaseBullet):
def __init__(self,screen,x,y):
image_path = 'feiji/bullet.png'
super(Bullet, self).__init__(screen,image_path,x+40,y-20)
def judge_jizhong(self,enemy):
if self.x > enemy.x and self.x < enemy.x + 56:
if self.y > enemy.y and self.y < enemy.y + 31:
print('击中敌机')
return True
else:
return False
def move(self):
self.y -= 5
def judge(self):
if self.y < 0:
return True
else:
return False
class PlaneGame(object):
def key_control(self):
for event in pygame.event.get():
if event.type == QUIT:
print('exit')
exit()
if event.type == KEYDOWN:
if event.key == K_LEFT or event.key == K_a:
print('left')
self.hero.x -= 5
elif event.key == K_RIGHT or event.key == K_d:
print('right')
self.hero.x += 5
elif event.key == K_UP or event.key == K_w:
print("up")
self.hero.y -= 5
elif event.key == K_DOWN or event.key == K_s:
print("down")
self.hero.y += 5
elif event.key == K_SPACE:
print("space-空格建")
self.hero.send_bullet(self.enemy)
elif event.key == K_b:
print("爆炸")
self.enemy.isbomb = True
def main(self):
pygame.init()
pygame.key.set_repeat(True)
self.screen = pygame.display.set_mode((480, 700), 0, 32)
self.background = pygame.image.load("feiji/background.png")
self.hero = HeroPlane(self.screen)
self.enemy = EnemyPlane(self.screen)
while True:
self.key_control()
self.screen.blit(self.background, (0, 0))
self.hero.display()
self.enemy.display()
self.enemy.move()
self.enemy.send_bullet(self.hero)
pygame.display.update()
time.sleep(0.01)
if __name__ == "__main__":
PlaneGame().main()