上一篇简单回顾操作系统相关的知识,这篇正式开始进入编译链接部分。
开发工具IDE一般都把编译和链接都集成进去了,这个过程叫做构建(build),就算是一个简单的gcc hello.c
命令就包含非常复杂的过程。下面介绍下将一步一步介绍这一切是怎么实现的。
编译过程
一个简单Hello World程序
#include
int main()
{
printf("Hello World");
return 0;
}
当使用gcc来编译的时候
gcc hello.c
然后运行该程序
./ a.out
上面总共经历了四个步骤,分别是预处理、编译、汇编和链接。如下图所示:
gcc可以通过参数来指定具体的编译步骤,参数如下:
-o:指定生成的输出文件;
-E:仅执行编译预处理;
-S:将C代码转换为汇编代码;
-wall:显示警告信息;
-c:仅执行编译操作,不进行连接操作。
预编译(输出.i文件)
c文件预处理为.i
文件,cpp预处理为.ii
文件。可以通过参数控制gcc当前操作的步骤。前面讲过用-E
仅仅执行预编译
gcc -E hello.c -o hello.i
由于预编译后的文件内容很长就不贴在这里了,放在了本文你末尾,有兴趣的可以看一看。预编译是处理源代码中的#
开始的预编译指令,比如#include #define
。
规则如下(可以参看预编译后的内容对比):
- 将所有
#define
删除,并且展开所有的宏定义。 - 处理所有的条件预编译指令,如
#if #ifdef #eif #else #endif
- 处理
#include
预编译指令,将被包含的文件Haru到预编译指令的位置。这个过程可能是一个递归的过程,有可能文件中还包含其他文件。 - 删除所有的注释
- 添加行号及文件标识符。如
# 2 "hello.c" 2
,用于编译器编译时产生调试用的行号信息。——格式没有弄懂? - 保留所有的#pragma编译指令,编译器需要
如果当无法判断宏定义是否正确或者文件包含是否正确时,可以查看编译后的文件来查找问题。
根据预编译后的
.i
文件,没弄明白的是为什么预编译之后很多文件会重复引入?
编译(输出汇编代码)
编译是程序构建的核心部分,也最复杂。需要在这个过程中完成词法分析、语法分析、语义分析及优化产生的汇编代码。
gcc -S hello.i -o hello.s
现在一般GCC把预编译和编译都合在一起了。但是也可以分开实现!GCC的命令其实是对一些编译程序的包装而已,它根据不同的参数去调用预编译程序(cc1)、汇编器(as)、链接器(ld)
后面会详细介绍
汇编(输出.o文件)
汇编器将上面生成的汇编代码转为机器可以执行的指令。每一条汇编语句对应一条机器指令。所以汇编过程比编译过程简单,没有复杂的语法、语义,也不需要优化指令。最终输出的是目标文件.o。
as -c hello.s -o hello.o
汇编之后的.o文件是二进制格式的。
链接
由于作者实验的系统是Linux,链接的部分需要用到Linux中的库及.o文件。所以实验在本地做不了
链接总的命令:
如果把路径都省略了
其中很多不知道什么意思,crt1.o、crti.o文件是什么(Mac中能找到),参数lgcc、lgcc_en是什么意思。后面在讲
如果直接执行ld -static helloworld.o
,则会报找不到符号的错误。
ld: warning: -macosx_version_min not specified, assuming 10.11
Undefined symbols for architecture x86_64:
"_printf", referenced from:
_main in helloworld.o
"start", referenced from:
-u command line option
ld: symbol(s) not found for inferred architecture x86_64
编译器(核心)
编译器就是将高级语言翻译为机器语言的一个工具。如果直接使用机器指令或汇编来写,一方面效率低,一方面只能为一种CPU编写,而不能移植。
编译过程一般分为6步(扫描、语法分析、词法分析、语义分析、源代码优化、代码生成和目标代码优化):
下面用这段代码为例:
词法分析(记号)
源代码首先被载入扫描器(语法分析器)。扫描器主要作用就是进行词法分析。运用类似有限状态机的算法将源代码字符序列分隔为一系列的记号。其主要作用就是分隔为一系列的记号。
如上面代码词法分析结果:
把这些记号归类可以有:关键字、标识符、字面量(包含数字、字符串)、特殊符号(加、等号)。然后将这些记号分别存入到对应的表中,以便后续使用。比如标识符放到符号表,数字、字符串放到文字表。
语法分析(语法树)
语法分析器读取上面词法分析的字符流、从中识别出语素、最后生成不同类型的标记。其间一旦发现无效标记,便会报错。
对扫描器产生的记号进行语法分析会生成语法树,语法树就是以表达式为结点的树。一个语句可能由多个表达式组成,如上面的例子。最终产生的语法树如下:
解读一下:整个语句看做是一个赋值表达式,左边是要给数组表达式,右边是一个乘法表达式,数组表达式由连个符号表达式组成。符号和数字是最小的表达式,所以作为整个语法书的结点。同时优先级和含义也会在这个过程中确定下来,比如乘法优先级比加法搞。
如果出现表达式不合法,比如括号不匹配,缺少什么操作符等,编译器就会报语法分析错误。
语义分析(标识类型)
语法分析主要是完成对表达式的语法层面的分析,但是不了解这个语句是否真的有含义。比如c语言两个指针做乘法是没有意义的,但语句是合法的。编译能分析的只能是静态语义,也即是在编译阶段可以确定的语义。动态语义只能在运行期才能确定。
常见的静态语义包括声明和类型匹配、类型转换。比如浮点表达式赋值给整型表达式,隐含着浮点转整型的过程;浮点赋值给指针的时候,语义分析这个类型不匹配,编译器也会报错。动态最长见得就是将0作为除数就是运行期语义错误。
经过语义分析极端,整个语法树表达式都被标识了类型。
中间语言生成(跨平台)
编译器有很多层次优化,并且在源代码就会有优化。这里提到的是源码级别的而优化器。
比如源码级别的优化针对上面的(2+6)表达式就会直接优化为8,因为这个值在编译期就可以确定了。最终生成如下语法树
直接在语法树上做优化比较困难,所以源代码优化器往往把语法树转换为中间代码,他是语法树的顺序表示。非常接近目标代码,但是不保护数据尺寸,变量地址,寄存器名字等。
中间有很多种,比如三地址码和P-代码。
三地址码:x = y op z
表示把变量y和z操作以后赋值给x。因为三个地址码语句有三个变量地址因此得名。
上面的语法树转为三地址码如下:
这里利用几个临时变量,优化程序会将2+6计算出来,得到t1=6然后将t1带入后面的代码。其实还可以省略t3。因为t2可以复用。最终简化如下:
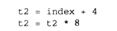

中间代码使得编译器分为前端和后端,前端负责产生平台无关的中间代码,后端将中间代码转为平台相关的代码。如果是跨平台的编译器,可以针对不同的平台使用同一个前端,不同的平台用多个后端。
生成目标代码
中间代码之后就术语编译器后端,主要包含代码生成及目标代优化。
代码生成将中间代码转换为目标的机器代码,这个过程依赖于机器,不同的机器有不同的字长,寄存器,整数数据类型及浮点数类型。上面生成的中间代码会可能会生成如下代码序列(X86下的汇编语言 )
高级编程语言本身非常复杂,如C++的定义极为复杂,目前没有任何一个编译器完整支持C++所有特性。其次CPU异常复杂,本身采用了流水线、多发射、超标量等特性,为了支持这些特性,编译器也异常复杂,尤其是表一起支持多硬件平台。
经过了扫描、语法分析、语义分析,源代码优化、代码生成及目标代码优化,最终编译为了目标代码。但是目标代码中的变量如index、array地址还没确定。如果index、array在同一编译单元里面,那么编译器可以为index和array分配内存空间,确定地址,如果是定义在其他程序模块,则还需要链接其他模块
链接器
符号(Symbol)这个概念随着汇编语言的普及而迅速被使用,用来表示一个地址。可以使一段子程序(函数的其实地址),也可以是一个变量的地址。
重用,划分更小的粒度,最开始程序时写在一个文件中,后来拆分为多个模块。
模块之间如何组合可以归结为模块之前如何通信,最常见的就是C/C++这种静态语言两章方式:一种模块间函数调用,一种是模块间变量访问。函数调用必须知道目标函数的其实地址,变量访问也需要知道变量地址。
最终归结为一种方式,那么就是模块间符号引用,模块间通过符号来通信类似于拼图版,定义符号的模块多出一块区域,引用该符号的模块搞好少了那个区域——拼接过程就是链接
模块拼装
链接的主要内容就是将各个模块之间相互引用的部分都处理好,是模块之间能够正确的衔接。本质就是将依稀额之灵对其他符号地址的引用加以修正。
链接过程包含地址空间分配、符号决议和重定位
每个模块的源代码进过编译器便以为目标文件,目标文件和库一起链接为最终的可执行文件。最常见的就是运行时库(Runtime Library),它是支持程序运行的基本函数集合。库本身是一组目标文件的包,包含一些常用代码编译为目标文件打包之后的集合。
重定位
在链接过程中,对其他定义在目标文件中的函数、变量调用的指令都需要重新调整,结合CPU指令来理解。
假设有个全局变量var目标文件在目标文件A里面定义,现在在目标文件B要访问这个全局变量,比如执行var = 42这段代码。对应的汇编

现在编译目标文件得到这个机器码:
由于目标文件并不知道var的变量地址,所以编译器没有办法确认,这里默认将目标地址置位0, 等待编译器在链接目标文件A、B的时候在将其修正。
加入A、B链接之后,变量var的地址为0x1000,那么连接器就会把这个目标地址修正为0x1000。
上面的这个过程就是重定位,修正的地方叫做重定位入口。
目标文件与可执行文件对比
这里用的工具是hooper,在iOS逆向的时候经常用到
目标文件hello.o的内容
可执行文件a.out的内容
以上对比就能看到,目标文件与可执行文件的差别很大。可执行文件中包含了其他.o文件的符号。
实验数据
原程序
#include
int main()
{
printf("Hello World");
return 0;
}
预编译后的内容
# 1 "hello.c"
# 1 "" 1
# 1 "" 3
# 331 "" 3
# 1 "" 1
# 1 "" 2
# 1 "hello.c" 2
# 1 "/usr/include/stdio.h" 1 3 4
# 64 "/usr/include/stdio.h" 3 4
# 1 "/usr/include/sys/cdefs.h" 1 3 4
# 587 "/usr/include/sys/cdefs.h" 3 4
# 1 "/usr/include/sys/_symbol_aliasing.h" 1 3 4
# 588 "/usr/include/sys/cdefs.h" 2 3 4
# 653 "/usr/include/sys/cdefs.h" 3 4
# 1 "/usr/include/sys/_posix_availability.h" 1 3 4
# 654 "/usr/include/sys/cdefs.h" 2 3 4
# 65 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/Availability.h" 1 3 4
# 190 "/usr/include/Availability.h" 3 4
# 1 "/usr/include/AvailabilityInternal.h" 1 3 4
# 191 "/usr/include/Availability.h" 2 3 4
# 66 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/_types.h" 1 3 4
# 27 "/usr/include/_types.h" 3 4
# 1 "/usr/include/sys/_types.h" 1 3 4
# 33 "/usr/include/sys/_types.h" 3 4
# 1 "/usr/include/machine/_types.h" 1 3 4
# 32 "/usr/include/machine/_types.h" 3 4
# 1 "/usr/include/i386/_types.h" 1 3 4
# 37 "/usr/include/i386/_types.h" 3 4
typedef signed char __int8_t;
typedef unsigned char __uint8_t;
typedef short __int16_t;
typedef unsigned short __uint16_t;
typedef int __int32_t;
typedef unsigned int __uint32_t;
typedef long long __int64_t;
typedef unsigned long long __uint64_t;
typedef long __darwin_intptr_t;
typedef unsigned int __darwin_natural_t;
# 70 "/usr/include/i386/_types.h" 3 4
typedef int __darwin_ct_rune_t;
typedef union {
char __mbstate8[128];
long long _mbstateL;
} __mbstate_t;
typedef __mbstate_t __darwin_mbstate_t;
typedef long int __darwin_ptrdiff_t;
typedef long unsigned int __darwin_size_t;
typedef __builtin_va_list __darwin_va_list;
typedef int __darwin_wchar_t;
typedef __darwin_wchar_t __darwin_rune_t;
typedef int __darwin_wint_t;
typedef unsigned long __darwin_clock_t;
typedef __uint32_t __darwin_socklen_t;
typedef long __darwin_ssize_t;
typedef long __darwin_time_t;
# 33 "/usr/include/machine/_types.h" 2 3 4
# 34 "/usr/include/sys/_types.h" 2 3 4
# 55 "/usr/include/sys/_types.h" 3 4
typedef __int64_t __darwin_blkcnt_t;
typedef __int32_t __darwin_blksize_t;
typedef __int32_t __darwin_dev_t;
typedef unsigned int __darwin_fsblkcnt_t;
typedef unsigned int __darwin_fsfilcnt_t;
typedef __uint32_t __darwin_gid_t;
typedef __uint32_t __darwin_id_t;
typedef __uint64_t __darwin_ino64_t;
typedef __darwin_ino64_t __darwin_ino_t;
typedef __darwin_natural_t __darwin_mach_port_name_t;
typedef __darwin_mach_port_name_t __darwin_mach_port_t;
typedef __uint16_t __darwin_mode_t;
typedef __int64_t __darwin_off_t;
typedef __int32_t __darwin_pid_t;
typedef __uint32_t __darwin_sigset_t;
typedef __int32_t __darwin_suseconds_t;
typedef __uint32_t __darwin_uid_t;
typedef __uint32_t __darwin_useconds_t;
typedef unsigned char __darwin_uuid_t[16];
typedef char __darwin_uuid_string_t[37];
# 1 "/usr/include/sys/_pthread/_pthread_types.h" 1 3 4
# 57 "/usr/include/sys/_pthread/_pthread_types.h" 3 4
struct __darwin_pthread_handler_rec {
void (*__routine)(void *);
void *__arg;
struct __darwin_pthread_handler_rec *__next;
};
struct _opaque_pthread_attr_t {
long __sig;
char __opaque[56];
};
struct _opaque_pthread_cond_t {
long __sig;
char __opaque[40];
};
struct _opaque_pthread_condattr_t {
long __sig;
char __opaque[8];
};
struct _opaque_pthread_mutex_t {
long __sig;
char __opaque[56];
};
struct _opaque_pthread_mutexattr_t {
long __sig;
char __opaque[8];
};
struct _opaque_pthread_once_t {
long __sig;
char __opaque[8];
};
struct _opaque_pthread_rwlock_t {
long __sig;
char __opaque[192];
};
struct _opaque_pthread_rwlockattr_t {
long __sig;
char __opaque[16];
};
struct _opaque_pthread_t {
long __sig;
struct __darwin_pthread_handler_rec *__cleanup_stack;
char __opaque[8176];
};
typedef struct _opaque_pthread_attr_t __darwin_pthread_attr_t;
typedef struct _opaque_pthread_cond_t __darwin_pthread_cond_t;
typedef struct _opaque_pthread_condattr_t __darwin_pthread_condattr_t;
typedef unsigned long __darwin_pthread_key_t;
typedef struct _opaque_pthread_mutex_t __darwin_pthread_mutex_t;
typedef struct _opaque_pthread_mutexattr_t __darwin_pthread_mutexattr_t;
typedef struct _opaque_pthread_once_t __darwin_pthread_once_t;
typedef struct _opaque_pthread_rwlock_t __darwin_pthread_rwlock_t;
typedef struct _opaque_pthread_rwlockattr_t __darwin_pthread_rwlockattr_t;
typedef struct _opaque_pthread_t *__darwin_pthread_t;
# 81 "/usr/include/sys/_types.h" 2 3 4
# 28 "/usr/include/_types.h" 2 3 4
# 39 "/usr/include/_types.h" 3 4
typedef int __darwin_nl_item;
typedef int __darwin_wctrans_t;
typedef __uint32_t __darwin_wctype_t;
# 68 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/sys/_types/_va_list.h" 1 3 4
# 31 "/usr/include/sys/_types/_va_list.h" 3 4
typedef __darwin_va_list va_list;
# 72 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/sys/_types/_size_t.h" 1 3 4
# 30 "/usr/include/sys/_types/_size_t.h" 3 4
typedef __darwin_size_t size_t;
# 73 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/sys/_types/_null.h" 1 3 4
# 74 "/usr/include/stdio.h" 2 3 4
# 1 "/usr/include/sys/stdio.h" 1 3 4
# 39 "/usr/include/sys/stdio.h" 3 4
int renameat(int, const char *, int, const char *) __attribute__((availability(macosx,introduced=10.10)));
int renamex_np(const char *, const char *, unsigned int) __attribute__((availability(macosx,introduced=10.12))) __attribute__((availability(ios,introduced=10.0))) __attribute__((availability(tvos,introduced=10.0))) __attribute__((availability(watchos,introduced=3.0)));
int renameatx_np(int, const char *, int, const char *, unsigned int) __attribute__((availability(macosx,introduced=10.12))) __attribute__((availability(ios,introduced=10.0))) __attribute__((availability(tvos,introduced=10.0))) __attribute__((availability(watchos,introduced=3.0)));
# 76 "/usr/include/stdio.h" 2 3 4
typedef __darwin_off_t fpos_t;
# 88 "/usr/include/stdio.h" 3 4
struct __sbuf {
unsigned char *_base;
int _size;
};
struct __sFILEX;
# 122 "/usr/include/stdio.h" 3 4
typedef struct __sFILE {
unsigned char *_p;
int _r;
int _w;
short _flags;
short _file;
struct __sbuf _bf;
int _lbfsize;
void *_cookie;
int (* _Nullable _close)(void *);
int (* _Nullable _read) (void *, char *, int);
fpos_t (* _Nullable _seek) (void *, fpos_t, int);
int (* _Nullable _write)(void *, const char *, int);
struct __sbuf _ub;
struct __sFILEX *_extra;
int _ur;
unsigned char _ubuf[3];
unsigned char _nbuf[1];
struct __sbuf _lb;
int _blksize;
fpos_t _offset;
} FILE;
extern FILE *__stdinp;
extern FILE *__stdoutp;
extern FILE *__stderrp;
# 231 "/usr/include/stdio.h" 3 4
void clearerr(FILE *);
int fclose(FILE *);
int feof(FILE *);
int ferror(FILE *);
int fflush(FILE *);
int fgetc(FILE *);
int fgetpos(FILE * restrict, fpos_t *);
char *fgets(char * restrict, int, FILE *);
FILE *fopen(const char * restrict __filename, const char * restrict __mode) __asm("_" "fopen" );
int fprintf(FILE * restrict, const char * restrict, ...) __attribute__((__format__ (__printf__, 2, 3)));
int fputc(int, FILE *);
int fputs(const char * restrict, FILE * restrict) __asm("_" "fputs" );
size_t fread(void * restrict __ptr, size_t __size, size_t __nitems, FILE * restrict __stream);
FILE *freopen(const char * restrict, const char * restrict,
FILE * restrict) __asm("_" "freopen" );
int fscanf(FILE * restrict, const char * restrict, ...) __attribute__((__format__ (__scanf__, 2, 3)));
int fseek(FILE *, long, int);
int fsetpos(FILE *, const fpos_t *);
long ftell(FILE *);
size_t fwrite(const void * restrict __ptr, size_t __size, size_t __nitems, FILE * restrict __stream) __asm("_" "fwrite" );
int getc(FILE *);
int getchar(void);
char *gets(char *);
void perror(const char *);
int printf(const char * restrict, ...) __attribute__((__format__ (__printf__, 1, 2)));
int putc(int, FILE *);
int putchar(int);
int puts(const char *);
int remove(const char *);
int rename (const char *__old, const char *__new);
void rewind(FILE *);
int scanf(const char * restrict, ...) __attribute__((__format__ (__scanf__, 1, 2)));
void setbuf(FILE * restrict, char * restrict);
int setvbuf(FILE * restrict, char * restrict, int, size_t);
int sprintf(char * restrict, const char * restrict, ...) __attribute__((__format__ (__printf__, 2, 3))) __attribute__((__availability__(swift, unavailable, message="Use snprintf instead.")));
int sscanf(const char * restrict, const char * restrict, ...) __attribute__((__format__ (__scanf__, 2, 3)));
FILE *tmpfile(void);
__attribute__((__availability__(swift, unavailable, message="Use mkstemp(3) instead.")))
__attribute__((deprecated("This function is provided for compatibility reasons only. Due to security concerns inherent in the design of tmpnam(3), it is highly recommended that you use mkstemp(3) instead.")))
char *tmpnam(char *);
int ungetc(int, FILE *);
int vfprintf(FILE * restrict, const char * restrict, va_list) __attribute__((__format__ (__printf__, 2, 0)));
int vprintf(const char * restrict, va_list) __attribute__((__format__ (__printf__, 1, 0)));
int vsprintf(char * restrict, const char * restrict, va_list) __attribute__((__format__ (__printf__, 2, 0))) __attribute__((__availability__(swift, unavailable, message="Use vsnprintf instead.")));
# 297 "/usr/include/stdio.h" 3 4
char *ctermid(char *);
FILE *fdopen(int, const char *) __asm("_" "fdopen" );
int fileno(FILE *);
# 321 "/usr/include/stdio.h" 3 4
int pclose(FILE *) __attribute__((__availability__(swift, unavailable, message="Use posix_spawn APIs or NSTask instead.")));
FILE *popen(const char *, const char *) __asm("_" "popen" ) __attribute__((__availability__(swift, unavailable, message="Use posix_spawn APIs or NSTask instead.")));
# 342 "/usr/include/stdio.h" 3 4
int __srget(FILE *);
int __svfscanf(FILE *, const char *, va_list) __attribute__((__format__ (__scanf__, 2, 0)));
int __swbuf(int, FILE *);
# 353 "/usr/include/stdio.h" 3 4
inline __attribute__ ((__always_inline__)) int __sputc(int _c, FILE *_p) {
if (--_p->_w >= 0 || (_p->_w >= _p->_lbfsize && (char)_c != '\n'))
return (*_p->_p++ = _c);
else
return (__swbuf(_c, _p));
}
# 379 "/usr/include/stdio.h" 3 4
void flockfile(FILE *);
int ftrylockfile(FILE *);
void funlockfile(FILE *);
int getc_unlocked(FILE *);
int getchar_unlocked(void);
int putc_unlocked(int, FILE *);
int putchar_unlocked(int);
int getw(FILE *);
int putw(int, FILE *);
__attribute__((__availability__(swift, unavailable, message="Use mkstemp(3) instead.")))
__attribute__((deprecated("This function is provided for compatibility reasons only. Due to security concerns inherent in the design of tempnam(3), it is highly recommended that you use mkstemp(3) instead.")))
char *tempnam(const char *__dir, const char *__prefix) __asm("_" "tempnam" );
# 417 "/usr/include/stdio.h" 3 4
# 1 "/usr/include/sys/_types/_off_t.h" 1 3 4
# 30 "/usr/include/sys/_types/_off_t.h" 3 4
typedef __darwin_off_t off_t;
# 418 "/usr/include/stdio.h" 2 3 4
int fseeko(FILE * __stream, off_t __offset, int __whence);
off_t ftello(FILE * __stream);
int snprintf(char * restrict __str, size_t __size, const char * restrict __format, ...) __attribute__((__format__ (__printf__, 3, 4)));
int vfscanf(FILE * restrict __stream, const char * restrict __format, va_list) __attribute__((__format__ (__scanf__, 2, 0)));
int vscanf(const char * restrict __format, va_list) __attribute__((__format__ (__scanf__, 1, 0)));
int vsnprintf(char * restrict __str, size_t __size, const char * restrict __format, va_list) __attribute__((__format__ (__printf__, 3, 0)));
int vsscanf(const char * restrict __str, const char * restrict __format, va_list) __attribute__((__format__ (__scanf__, 2, 0)));
# 442 "/usr/include/stdio.h" 3 4
# 1 "/usr/include/sys/_types/_ssize_t.h" 1 3 4
# 30 "/usr/include/sys/_types/_ssize_t.h" 3 4
typedef __darwin_ssize_t ssize_t;
# 443 "/usr/include/stdio.h" 2 3 4
int dprintf(int, const char * restrict, ...) __attribute__((__format__ (__printf__, 2, 3))) __attribute__((availability(macosx,introduced=10.7)));
int vdprintf(int, const char * restrict, va_list) __attribute__((__format__ (__printf__, 2, 0))) __attribute__((availability(macosx,introduced=10.7)));
ssize_t getdelim(char ** restrict __linep, size_t * restrict __linecapp, int __delimiter, FILE * restrict __stream) __attribute__((availability(macosx,introduced=10.7)));
ssize_t getline(char ** restrict __linep, size_t * restrict __linecapp, FILE * restrict __stream) __attribute__((availability(macosx,introduced=10.7)));
# 458 "/usr/include/stdio.h" 3 4
extern const int sys_nerr;
extern const char *const sys_errlist[];
int asprintf(char ** restrict, const char * restrict, ...) __attribute__((__format__ (__printf__, 2, 3)));
char *ctermid_r(char *);
char *fgetln(FILE *, size_t *);
const char *fmtcheck(const char *, const char *);
int fpurge(FILE *);
void setbuffer(FILE *, char *, int);
int setlinebuf(FILE *);
int vasprintf(char ** restrict, const char * restrict, va_list) __attribute__((__format__ (__printf__, 2, 0)));
FILE *zopen(const char *, const char *, int);
FILE *funopen(const void *,
int (* _Nullable)(void *, char *, int),
int (* _Nullable)(void *, const char *, int),
fpos_t (* _Nullable)(void *, fpos_t, int),
int (* _Nullable)(void *));
# 498 "/usr/include/stdio.h" 3 4
# 1 "/usr/include/secure/_stdio.h" 1 3 4
# 31 "/usr/include/secure/_stdio.h" 3 4
# 1 "/usr/include/secure/_common.h" 1 3 4
# 32 "/usr/include/secure/_stdio.h" 2 3 4
# 42 "/usr/include/secure/_stdio.h" 3 4
extern int __sprintf_chk (char * restrict, int, size_t,
const char * restrict, ...);
# 52 "/usr/include/secure/_stdio.h" 3 4
extern int __snprintf_chk (char * restrict, size_t, int, size_t,
const char * restrict, ...);
extern int __vsprintf_chk (char * restrict, int, size_t,
const char * restrict, va_list);
extern int __vsnprintf_chk (char * restrict, size_t, int, size_t,
const char * restrict, va_list);
# 499 "/usr/include/stdio.h" 2 3 4
# 2 "hello.c" 2
int main()
{
printf("Hello World");
return 0;
}
编译之后的内容
.section __TEXT,__text,regular,pure_instructions
.macosx_version_min 10, 12
.globl _main
.p2align 4, 0x90
_main: ## @main
.cfi_startproc
## BB#0:
pushq %rbp
Lcfi0:
.cfi_def_cfa_offset 16
Lcfi1:
.cfi_offset %rbp, -16
movq %rsp, %rbp
Lcfi2:
.cfi_def_cfa_register %rbp
subq $16, %rsp
leaq L_.str(%rip), %rdi
movl $0, -4(%rbp)
movb $0, %al
callq _printf
xorl %ecx, %ecx
movl %eax, -8(%rbp) ## 4-byte Spill
movl %ecx, %eax
addq $16, %rsp
popq %rbp
retq
.cfi_endproc
.section __TEXT,__cstring,cstring_literals
L_.str: ## @.str
.asciz "Hello World"
.subsections_via_symbols
扩展阅读
gcc命令
gcc 手册
有限状态机
词法分析