利用python实现星座运势查询APP
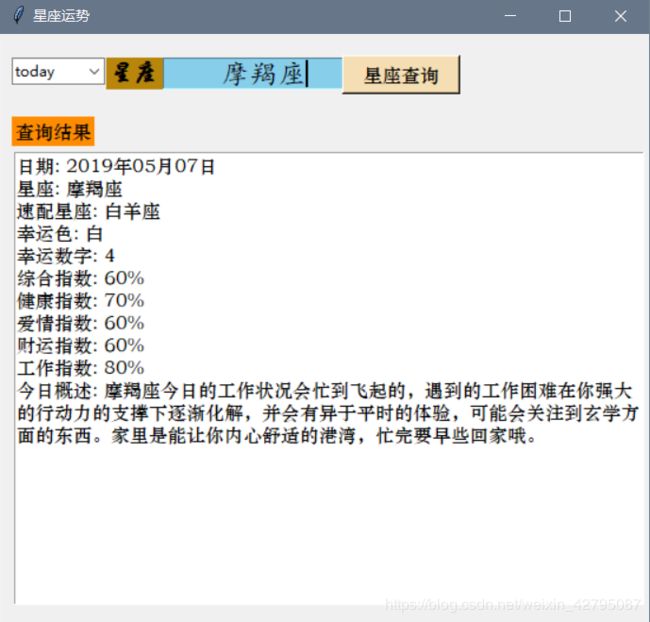
# *_* coding:utf8 *_*
import json
import requests
import tkinter
import tkinter.font as myFont
from tkinter import ttk
app_key = "074ec2ba573ad5cff06dd7addf15b5b5"
url = "http://web.juhe.cn:8080/constellation/getAll?"
def start_parse_fortune():
params = {
"key": app_key,
"consName": input_entry.get(),
"type": pull_value.get()
}
response = requests.get(url, params=params)
response = json.loads(response.text)
if response["resultcode"] == "200":
output_str = ""
output_str += "日期: {datetime}\n" \
"星座: {name}\n" \
"速配星座: {QFriend}\n" \
"幸运色: {color}\n" \
"幸运数字: {number}\n" \
"综合指数: {all}\n" \
"健康指数: {health}\n" \
"爱情指数: {love}\n" \
"财运指数: {money}\n" \
"工作指数: {work}\n" \
"今日概述: {summary}\n"\
.format(**response)
else:
output_str = response["reason"]
result_text.delete(0.0, "end")
result_text.insert("insert", output_str)
root = tkinter.Tk()
root.title("星座运势")
root.geometry("550x500")
entry_font = myFont.Font(size=15, family="华文行楷", weight="bold")
entry_font1 = myFont.Font(size=17, family="楷体")
entry_font2 = myFont.Font(size=12, family="华文中宋")
def go(args):
pull_value.set(pull_list.get())
pull_value = tkinter.StringVar(value="today")
pull_list = ttk.Combobox(root, width=8)
pull_list["values"] = ("today", "tomorrow")
pull_list.current(0)
pull_list.bind("<>", go)
pull_list.place(x=10, y=20)
dram_keyword_lab = tkinter.Label(root, text="星座", justify="center", font=entry_font, bg="DarkGoldenrod")
dram_keyword_lab.place(x=90, y=20)
input_entry = tkinter.Entry(root, justify="center", font=entry_font1, bg="SkyBlue", width=14)
input_entry.place(x=138, y=20)
input_button = tkinter.Button(root, text="星座查询", justify="center", font=entry_font2, bg="Wheat", width=9,
command=start_parse_fortune)
input_button.place(x=290, y=18)
dram_result_lab = tkinter.Label(root, text="查询结果", justify="center", font=entry_font2, bg="DarkOrange")
dram_result_lab.place(x=10, y=70)
result_text = tkinter.Text(root, width=53, height=20, font=entry_font2)
result_text.place(x=12, y=100)
root.mainloop()