- python基于django/flask体育馆管理系统Django-SpringBoot-php-Node.js-flask
QQ_511008285
pythondjangoflaskspringbootphpnode.js
目录技术栈介绍具体实现截图系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研究步骤基本合理,难度适中,本选题是学生所学专业知识的延续,符合学生专业发展方向,对于提高学生的基本知识和技能以及钻研能力有益。该学生能够在预定时间内完成该课题的设计。
- php开发转go的学习计划及课程资料信息
老李要转行
phpgolang学习
以下是为该课程体系整理的配套教材和教程资源清单,包含书籍、视频、官方文档和实战项目资源,帮助你系统化学习:Go语言学习教材推荐(PHP开发者适配版)一、核心教材(按学习阶段分类)1.基础语法阶段(阶段一)资源类型名称推荐理由链接/获取方式官方教程Go语言之旅交互式学习,快速上手基础语法官方免费中文书籍《Go语言入门指南》专为有其他语言经验的开发者编写京东/当当速查手册Go速查表PHP与Go语法对比
- 云贝餐饮独立连锁版v3源码 扫码点餐毕业设计参考好选择 可二开
DeepinThinks
源码分享课程设计php小程序
云贝V3最新版,采用PHP语言和Laravel9框架,前端使用VUE3和小程序uniapp开发。️云贝V3特点:全开源:提供完整的源代码,无版权纠纷。免授权:无需支付额外授权费用。可二开:支持二次开发,满足个性化需求。技术支持:提供开发者技术支持,确保项目顺利进行。最新版本号:V1.7.9新增会员价显示会员价开关(设置-订单设置-商品设置)新增异地外卖下单开关(门店-业务设置-外送设置)新增套餐固
- 最新计算机专业毕设论文选题大全基于BeautifulSoup的毕业设计详细题目100套优质毕设项目分享(源码+论文)✅
会写代码的羊
毕设选题课程设计beautifulsoup毕业设计毕业设计题目毕设题目python网络爬虫
文章目录前言最新毕设选题(建议收藏起来)基于BeautifulSoup的毕业设计选题毕设作品推荐前言2025全新毕业设计项目博主介绍:✌全网粉丝10W+,CSDN全栈领域优质创作者,博客之星、掘金/华为云/阿里云等平台优质作者。技术范围:SpringBoot、Vue、SSM、HLMT、Jsp、PHP、Nodejs、Python、爬虫、数据可视化、小程序、大数据、机器学习等设计与开发。主要内容:免费
- HTML编辑器CKEDITOR支持哪些格式的WORD内容导入?
2501_90699640
html编辑器wordckeditor粘贴wordckeditor导入wordckeditor导入pdfckeditor导入ppt
要求:开源,免费,技术支持编辑器:ckeditor前端:vue2,vue3.vue-cli后端:asp,java,jsp,springboot,php,asp.net,.netcore功能:导入Word,导入Excel,导入PPT(PowerPoint),导入PDF,复制粘贴word,导入微信公众号内容,web截屏平台:Windows,macOS,Linux,RedHat,Ubuntu,CentO
- PHPer看docker容器的管理详解
PHP开源社区
PHP架构dockerpythonmysqlphp
查询容器信息dockerinspcet查询信息,包括运行情况、存贮位置、配置参数、网络设置等。查询容器的运行状态dockerinspect-f{{.State.Status}}【容器】查询容器的IPdockerinspect-f{{.NetworkSettings.IPAddress}}【容器】查询容器日志信息Ωdockerlogs【容器】-f实时打印最新的日志dockerstats实时查看容器所
- 原来的笔记-pear live user最难理解的conf.php的内容
stone5
usersessionpathintegernullemail
最难理解的conf.php的内容Posted三月9th,2007bystone5pear最难懂的conf.php的内容放这里ini_set("include_path",'../libs/PEAR/'.PATH_SEPARATOR.ini_get("include_path"));require_once'MDB2.php';require_once'LiveUser/LiveUser.php';
- python基于django/flask网上书城系统Django-SpringBoot-php-Node.js-flask
QQ_1963288475
pythondjangoflaskspringbootphplaravelnode.js
目录技术栈介绍具体实现截图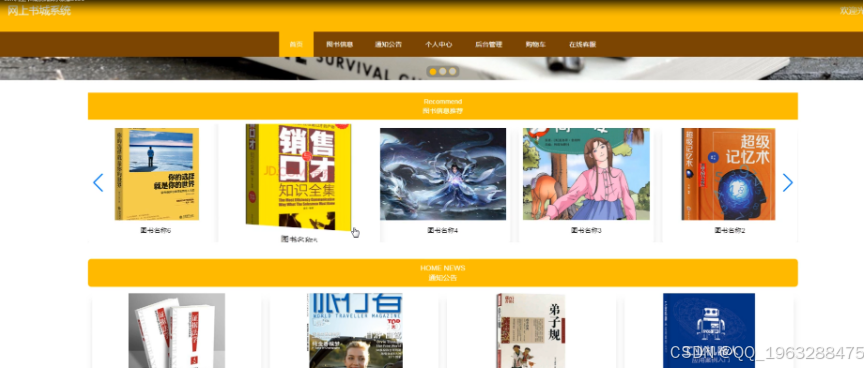系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研
- 在 CentOS 7 上安装 PHP 7.3
wjf63000
centosphplinux
在CentOS7上安装PHP7.3可以按照以下步骤进行操作:1.安装必要的依赖和EPEL仓库EPEL(ExtraPackagesforEnterpriseLinux)是为企业级Linux提供额外软件包的仓库,yum-utils用于管理yum仓库。sudoyuminstall-yepel-releaseyum-utils2.添加Remi仓库Remi仓库包含了丰富的PHP版本,你可以从中选择PHP7.
- GIT日常记录
码农汉子
elasticsearch大数据搜索引擎
sudogitclonehttps://gitee.com/yiketalks/yike_admin.git命令行下载项目命令(进入本地项目地址下)//查看远程仓库地址gitremote-v//切换远程仓库gitremoteset-urloriginhttps://gitee.com/yike_php/yike_work.git分支操作gitbranch//显示分支一览表,同时确认当前所在的分支1
- 【2025年春季】全国CTF夺旗赛-从零基础入门到竞赛,看这一篇就稳了!
白帽子凯哥
web安全学习安全CTF夺旗赛网络安全
基于入门网络安全/黑客打造的:黑客&网络安全入门&进阶学习资源包目录一、CTF简介二、CTF竞赛模式三、CTF各大题型简介四、CTF学习路线4.1、初期1、html+css+js(2-3天)2、apache+php(4-5天)3、mysql(2-3天)4、python(2-3天)5、burpsuite(1-2天)4.2、中期1、SQL注入(7-8天)2、文件上传(7-8天)3、其他漏洞(14-15
- 批量检查微信小程序是否被封的Go代码
微信微信小程序
概述:这段Go代码通过请求接口https://api.52an.fun/xcx/checkxcx.php?appid={appid},批量检查多个微信小程序是否被封禁。接口返回的JSON数据中包含code字段,code为1表示小程序正常,code为0表示小程序被封禁,并且会返回封禁原因。程序会根据返回结果输出每个小程序的状态。Go代码示例:packagemainimport("encoding/j
- 批量检查QQ域名是否被封的C++代码示例
qq
概述:此C++代码示例展示了如何批量请求指定的API接口,检查QQ域名的状态。根据API返回的status值,status为1表示域名正常,status为0表示域名被封禁。我们将使用libcurl来进行HTTP请求,使用jsoncpp解析返回的JSON数据。目标:通过C++编写代码,批量请求https://api.52an.fun/qq/api.php?url=接口,检查多个QQ域名的封禁状态,并
- PHP与数据库连接常见问题及解决办法
奥顺互联_老张
php教程php数据库
PHP与数据库连接常见问题及解决办法在现代Web开发中,PHP与数据库的连接是不可或缺的一部分。无论是构建动态网站、内容管理系统(CMS)还是电子商务平台,PHP与数据库的交互都是核心功能之一。然而,在实际开发过程中,开发者常常会遇到各种与数据库连接相关的问题。本文将探讨PHP与数据库连接中的常见问题,并提供相应的解决办法。1.数据库连接失败问题描述在PHP中,连接数据库时最常见的错误是无法连接到
- AJAX PHP:深入理解与实际应用
wjs2024
开发语言
AJAXPHP:深入理解与实际应用引言随着互联网技术的不断发展,前端与后端交互变得更加频繁。AJAX(AsynchronousJavaScriptandXML)和PHP(HypertextPreprocessor)作为两种流行的技术,在实现动态网页和应用程序方面扮演着重要角色。本文将深入探讨AJAXPHP的工作原理、应用场景以及实际开发中的注意事项。AJAXPHP概述AJAXAJAX是一种基于Ja
- mongodb基本使用(四)
dibisha7239
数据库javascript数据结构与算法ViewUI
MongoDB条件操作符描述条件操作符用于比较两个表达式并从mongoDB集合中获取数据。MongoDB中条件操作符有:(>)大于-$gt(=)大于等于-$gte(db.col.insert({title:'PHP教程',description:'PHP是一种创建动态交互性站点的强有力的服务器端脚本语言。',by:'菜鸟教程',url:'http://www.runoob.com',tags:['
- 树莓派搭php,Raspberry Pi 树莓派搭LAMP服务器
平平无奇的美女
树莓派搭php
目录:为什么要用树莓派?DebianLinux安全性操作系统性能优化配置网络开启sshMakingtheserveravailableontheInternetDNS安装apache安全MySQL安装PHP配置完成本文将会介绍如何把树莓派配置为一台LAMP服务器.这和把XUbuntu配成LAMP服务器有些相似,但是针对树莓派有些需要特殊处理的地方.下面是LAMP服务器的最通用配置:Linux–操作
- Android进行Post提交JSON数据注意事项
kerry1789
Android感慨androidjava单元测试
刚好业务需要写一个测试程序给客户使用,直接入主题吧!请求地址:http://12.32.12.32:91889/testx.php请求参数:{"test1":"顾家家居口味咯咯咯","test2":"把1册","test3":"13512341234","test4":"5rW35Y2X55uR54ux566h55CG5bGA6LCD5bqm5oyH5oyl5bmz5Y","test5":"123
- 树莓派raspberry搭建web服务(基于LAMP)
最古琴
撸了今年阿里、头条和美团的面试,我有一个重要发现.......>>>本文永久地址:https://my.oschina.net/bysu/blog/15502121.安装apachesudoapt-getinstallapache2php-gdphp安装完之后,怎么确认是否安装成功了呢?可以通过以下几种方式确认。a.可以查看是否已有相应的服务ps-ef|grepapache会看到4条服务,其中主进
- lighttpd安装和配置https
hailangnet
Debian笔记httpshttplighttpd
aptinstalllighttpdapt-getinstallphp-cgilighttpd-enable-modfastcgifastcgi-phpservicelighttpdforce-reloadlighttpd配置httpssudonano/etc/lighttpd/lighttpd.conf加入:server.modules+=("mod_openssl")$SERVER["sock
- 基于责任链与策略模式的轻量级PHP日志库设计
苏琢玉
策略模式php责任链模式
你有没有遇到过这样的情况:代码被各种人拷来拷去,散落在不同的服务器上,它们运行着同样的代码,却各有各的脾气。A服务器风平浪静,B服务器炸成烟花,C服务器似乎活着但又不太对劲……而你,每天都在面对来自四面八方的“XX功能炸了”“接口500了”“部署完直接寄了”的灵魂拷问。最离谱的是,它们都会从你这同步最新的代码,但到底是代码问题还是服务器环境问题,你根本没办法第一时间知道。于是,问题就变成了:如何把
- Web 开发都需要学什么?
Duiz33237
前端html5css3web
Web开发是指开发和构建用于互联网的网站和应用程序的过程。它涉及使用各种编程语言、框架和技术来创建功能丰富、用户友好的网站和应用程序。常见的web开发技术包括HTML、CSS和JavaScript。HTML用于创建网页的结构,CSS用于样式和布局,而JavaScript用于实现交互和动态效果。此外,还有许多其他的编程语言和框架,如Python、PHP、Ruby、React、Angular等,用于开
- 2025最新版易支付正版源码开源免授权搭建下载
阿辉博客
开源
搭建教程服务器环境推荐使用宝塔、AMH、XP等面板一键部署服务器环境。PHP版本:>=7.1,推荐7.4或8.0MySQL版本:5.6或5.7伪静态配置直接上传后访问即可完成安装!创建好网站之后,需要配置伪静态才能正常发起支付。以下分别是Nginx、Apache、IIS服务器的伪静态配置方法:Nginx如果是Nginx,伪静态规则在源码包根目录的nginx.txt文件里面。将nginx.txt里面
- PHP语言的区块链扩展性
叶雅茗
包罗万象golang开发语言后端
PHP语言的区块链扩展性引言区块链技术因其去中心化、透明性和不可篡改的特性而备受关注,已被广泛应用于金融、物流、供应链管理、数字身份等多个领域。而在构建区块链应用时,开发语言的选择至关重要。PHP作为一种流行的服务器端脚本语言,凭借其简单易学的特性和丰富的生态系统,逐渐开始在区块链应用开发中崭露头角。本文将探讨PHP语言在区块链应用中的扩展性,包括其优点、局限性,以及如何利用现有的框架和库来构建高
- php中文乱码问号,如何解决PHP中文乱码问题?
Helios-Yang
php中文乱码问号
作为该国家/区域内信息处理的基础,字符编码集起着统一编码的重要作用。字符编码集按长度分为SBCS(单字节字符集),DBCS(双字节字符集)两大类。早期的软件(尤其是操作系统),为了解决本地字符信息的计算机处理,出现了各种本地化版本(L10N),为了区分,引进了LANG,Codepage等概念。但是由于各个本地字符集代码范围重叠,相互间信息交换困难;软件各个本地化版本独立维护成本较高。因此有必要将本
- php中文乱码无法解决_PHP基础|如何解决中文乱码问题?
梦里一只喵
php中文乱码无法解决
为什么会出现中文乱码?很多新手朋友学习PHP的时候,发现程序中的中文在输出的时候会出现乱码的问题,那么为什么会出现这种乱码的情况呢?一般来说,乱码的出现有2种原因,一种是由于编码(charset)设置错误,导致浏览器以错误的编码来解析,从而出现了满屏乱七八糟的“天书”,第二种就是文件被以错误的编码打开,然后保存,比如一个文本文件原先是GB2312编码的,却以UTF-8编码打开再保存,就会出现乱码的
- PHP 处理csv 文件 解决中文乱码
MountainYanYL
PHPcsvphp
/***读取csv格式的数据*@param$file*@returnarray*/publicstaticfunctionread_csv($file){setlocale(LC_ALL,'zh_CN');//linux系统下生效$data=[];//返回的文件数据行if(!is_file($file)&&!file_exists($file)){return$data;}$cvs_file=fo
- PHP编译安装oci8扩展
爬山虎还上班
phpoci8
文档:https://www.php.net/manual/zh/ref.pdo-oci.php如果Oracle数据库与PHP在同一台机器上,则数据库软件已经包含了必要的库。当PHP在另一台机器上时,请使用免费的»OracleInstantClient库点击“OracleInstantClient”进入官网相关地址,根据服务器情况选择对应版本,此处我选择的OracleLinux7(OracleIn
- 解决PHP中文乱码问题
UqConstruction
phpandroidoracle
在PHP开发中,中文乱码是一个常见的问题。当我们在处理中文字符时,有时会遇到显示不正常的情况,这就是中文乱码。本文将介绍一些常见的方法来解决PHP中文乱码问题。设置字符编码PHP中文乱码问题的根本原因是字符编码不匹配。为了正确显示中文字符,我们需要确保在所有的环节中使用相同的字符编码。常见的字符编码包括UTF-8、GBK等。首先,在PHP文件的开头添加以下代码,将文件的字符编码设置为UTF-8:h
- PHP与MySQL的高效数据交互:最佳实践与优化技巧
奥顺互联V
phpphpandroid开发语言
在现代Web开发中,PHP与MySQL的组合仍然是最常见的技术栈之一。PHP作为一种广泛使用的服务器端脚本语言,与MySQL这一强大的关系型数据库管理系统相结合,能够构建出功能强大且高效的Web应用。然而,随着数据量的增长和用户需求的提升,如何实现高效的数据交互成为了开发者必须面对的重要课题。本文将探讨PHP与MySQL高效数据交互的最佳实践与优化技巧,帮助开发者提升系统性能与用户体验。1.使用预
- rust的指针作为函数返回值是直接传递,还是先销毁后创建?
wudixiaotie
返回值
这是我自己想到的问题,结果去知呼提问,还没等别人回答, 我自己就想到方法实验了。。
fn main() {
let mut a = 34;
println!("a's addr:{:p}", &a);
let p = &mut a;
println!("p's addr:{:p}", &a
- java编程思想 -- 数据的初始化
百合不是茶
java数据的初始化
1.使用构造器确保数据初始化
/*
*在ReckInitDemo类中创建Reck的对象
*/
public class ReckInitDemo {
public static void main(String[] args) {
//创建Reck对象
new Reck();
}
}
- [航天与宇宙]为什么发射和回收航天器有档期
comsci
地球的大气层中有一个时空屏蔽层,这个层次会不定时的出现,如果该时空屏蔽层出现,那么将导致外层空间进入的任何物体被摧毁,而从地面发射到太空的飞船也将被摧毁...
所以,航天发射和飞船回收都需要等待这个时空屏蔽层消失之后,再进行
&
- linux下批量替换文件内容
商人shang
linux替换
1、网络上现成的资料
格式: sed -i "s/查找字段/替换字段/g" `grep 查找字段 -rl 路径`
linux sed 批量替换多个文件中的字符串
sed -i "s/oldstring/newstring/g" `grep oldstring -rl yourdir`
例如:替换/home下所有文件中的www.admi
- 网页在线天气预报
oloz
天气预报
网页在线调用天气预报
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transit
- SpringMVC和Struts2比较
杨白白
springMVC
1. 入口
spring mvc的入口是servlet,而struts2是filter(这里要指出,filter和servlet是不同的。以前认为filter是servlet的一种特殊),这样就导致了二者的机制不同,这里就牵涉到servlet和filter的区别了。
参见:http://blog.csdn.net/zs15932616453/article/details/8832343
2
- refuse copy, lazy girl!
小桔子
copy
妹妹坐船头啊啊啊啊!都打算一点点琢磨呢。文字编辑也写了基本功能了。。今天查资料,结果查到了人家写得完完整整的。我清楚的认识到:
1.那是我自己觉得写不出的高度
2.如果直接拿来用,很快就能解决问题
3.然后就是抄咩~~
4.肿么可以这样子,都不想写了今儿个,留着作参考吧!拒绝大抄特抄,慢慢一点点写!
- apache与php整合
aichenglong
php apache web
一 apache web服务器
1 apeche web服务器的安装
1)下载Apache web服务器
2)配置域名(如果需要使用要在DNS上注册)
3)测试安装访问http://localhost/验证是否安装成功
2 apache管理
1)service.msc进行图形化管理
2)命令管理,配
- Maven常用内置变量
AILIKES
maven
Built-in properties
${basedir} represents the directory containing pom.xml
${version} equivalent to ${project.version} (deprecated: ${pom.version})
Pom/Project properties
Al
- java的类和对象
百合不是茶
JAVA面向对象 类 对象
java中的类:
java是面向对象的语言,解决问题的核心就是将问题看成是一个类,使用类来解决
java使用 class 类名 来创建类 ,在Java中类名要求和构造方法,Java的文件名是一样的
创建一个A类:
class A{
}
java中的类:将某两个事物有联系的属性包装在一个类中,再通
- JS控制页面输入框为只读
bijian1013
JavaScript
在WEB应用开发当中,增、删除、改、查功能必不可少,为了减少以后维护的工作量,我们一般都只做一份页面,通过传入的参数控制其是新增、修改或者查看。而修改时需将待修改的信息从后台取到并显示出来,实际上就是查看的过程,唯一的区别是修改时,页面上所有的信息能修改,而查看页面上的信息不能修改。因此完全可以将其合并,但通过前端JS将查看页面的所有信息控制为只读,在信息量非常大时,就比较麻烦。
- AngularJS与服务器交互
bijian1013
JavaScriptAngularJS$http
对于AJAX应用(使用XMLHttpRequests)来说,向服务器发起请求的传统方式是:获取一个XMLHttpRequest对象的引用、发起请求、读取响应、检查状态码,最后处理服务端的响应。整个过程示例如下:
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange
- [Maven学习笔记八]Maven常用插件应用
bit1129
maven
常用插件及其用法位于:http://maven.apache.org/plugins/
1. Jetty server plugin
2. Dependency copy plugin
3. Surefire Test plugin
4. Uber jar plugin
1. Jetty Pl
- 【Hive六】Hive用户自定义函数(UDF)
bit1129
自定义函数
1. 什么是Hive UDF
Hive是基于Hadoop中的MapReduce,提供HQL查询的数据仓库。Hive是一个很开放的系统,很多内容都支持用户定制,包括:
文件格式:Text File,Sequence File
内存中的数据格式: Java Integer/String, Hadoop IntWritable/Text
用户提供的 map/reduce 脚本:不管什么
- 杀掉nginx进程后丢失nginx.pid,如何重新启动nginx
ronin47
nginx 重启 pid丢失
nginx进程被意外关闭,使用nginx -s reload重启时报如下错误:nginx: [error] open() “/var/run/nginx.pid” failed (2: No such file or directory)这是因为nginx进程被杀死后pid丢失了,下一次再开启nginx -s reload时无法启动解决办法:nginx -s reload 只是用来告诉运行中的ng
- UI设计中我们为什么需要设计动效
brotherlamp
UIui教程ui视频ui资料ui自学
随着国际大品牌苹果和谷歌的引领,最近越来越多的国内公司开始关注动效设计了,越来越多的团队已经意识到动效在产品用户体验中的重要性了,更多的UI设计师们也开始投身动效设计领域。
但是说到底,我们到底为什么需要动效设计?或者说我们到底需要什么样的动效?做动效设计也有段时间了,于是尝试用一些案例,从产品本身出发来说说我所思考的动效设计。
一、加强体验舒适度
嗯,就是让用户更加爽更加爽的用你的产品。
- Spring中JdbcDaoSupport的DataSource注入问题
bylijinnan
javaspring
参考以下两篇文章:
http://www.mkyong.com/spring/spring-jdbctemplate-jdbcdaosupport-examples/
http://stackoverflow.com/questions/4762229/spring-ldap-invoking-setter-methods-in-beans-configuration
Sprin
- 数据库连接池的工作原理
chicony
数据库连接池
随着信息技术的高速发展与广泛应用,数据库技术在信息技术领域中的位置越来越重要,尤其是网络应用和电子商务的迅速发展,都需要数据库技术支持动 态Web站点的运行,而传统的开发模式是:首先在主程序(如Servlet、Beans)中建立数据库连接;然后进行SQL操作,对数据库中的对象进行查 询、修改和删除等操作;最后断开数据库连接。使用这种开发模式,对
- java 关键字
CrazyMizzz
java
关键字是事先定义的,有特别意义的标识符,有时又叫保留字。对于保留字,用户只能按照系统规定的方式使用,不能自行定义。
Java中的关键字按功能主要可以分为以下几类:
(1)访问修饰符
public,private,protected
p
- Hive中的排序语法
daizj
排序hiveorder byDISTRIBUTE BYsort by
Hive中的排序语法 2014.06.22 ORDER BY
hive中的ORDER BY语句和关系数据库中的sql语法相似。他会对查询结果做全局排序,这意味着所有的数据会传送到一个Reduce任务上,这样会导致在大数量的情况下,花费大量时间。
与数据库中 ORDER BY 的区别在于在hive.mapred.mode = strict模式下,必须指定 limit 否则执行会报错。
- 单态设计模式
dcj3sjt126com
设计模式
单例模式(Singleton)用于为一个类生成一个唯一的对象。最常用的地方是数据库连接。 使用单例模式生成一个对象后,该对象可以被其它众多对象所使用。
<?phpclass Example{ // 保存类实例在此属性中 private static&
- svn locked
dcj3sjt126com
Lock
post-commit hook failed (exit code 1) with output:
svn: E155004: Working copy 'D:\xx\xxx' locked
svn: E200031: sqlite: attempt to write a readonly database
svn: E200031: sqlite: attempt to write a
- ARM寄存器学习
e200702084
数据结构C++cC#F#
无论是学习哪一种处理器,首先需要明确的就是这种处理器的寄存器以及工作模式。
ARM有37个寄存器,其中31个通用寄存器,6个状态寄存器。
1、不分组寄存器(R0-R7)
不分组也就是说说,在所有的处理器模式下指的都时同一物理寄存器。在异常中断造成处理器模式切换时,由于不同的处理器模式使用一个名字相同的物理寄存器,就是
- 常用编码资料
gengzg
编码
List<UserInfo> list=GetUserS.GetUserList(11);
String json=JSON.toJSONString(list);
HashMap<Object,Object> hs=new HashMap<Object, Object>();
for(int i=0;i<10;i++)
{
- 进程 vs. 线程
hongtoushizi
线程linux进程
我们介绍了多进程和多线程,这是实现多任务最常用的两种方式。现在,我们来讨论一下这两种方式的优缺点。
首先,要实现多任务,通常我们会设计Master-Worker模式,Master负责分配任务,Worker负责执行任务,因此,多任务环境下,通常是一个Master,多个Worker。
如果用多进程实现Master-Worker,主进程就是Master,其他进程就是Worker。
如果用多线程实现
- Linux定时Job:crontab -e 与 /etc/crontab 的区别
Josh_Persistence
linuxcrontab
一、linux中的crotab中的指定的时间只有5个部分:* * * * *
分别表示:分钟,小时,日,月,星期,具体说来:
第一段 代表分钟 0—59
第二段 代表小时 0—23
第三段 代表日期 1—31
第四段 代表月份 1—12
第五段 代表星期几,0代表星期日 0—6
如:
*/1 * * * * 每分钟执行一次。
*
- KMP算法详解
hm4123660
数据结构C++算法字符串KMP
字符串模式匹配我们相信大家都有遇过,然而我们也习惯用简单匹配法(即Brute-Force算法),其基本思路就是一个个逐一对比下去,这也是我们大家熟知的方法,然而这种算法的效率并不高,但利于理解。
假设主串s="ababcabcacbab",模式串为t="
- 枚举类型的单例模式
zhb8015
单例模式
E.编写一个包含单个元素的枚举类型[极推荐]。代码如下:
public enum MaYun {himself; //定义一个枚举的元素,就代表MaYun的一个实例private String anotherField;MaYun() {//MaYun诞生要做的事情//这个方法也可以去掉。将构造时候需要做的事情放在instance赋值的时候:/** himself = MaYun() {*
- Kafka+Storm+HDFS
ssydxa219
storm
cd /myhome/usr/stormbin/storm nimbus &bin/storm supervisor &bin/storm ui &Kafka+Storm+HDFS整合实践kafka_2.9.2-0.8.1.1.tgzapache-storm-0.9.2-incubating.tar.gzKafka安装配置我们使用3台机器搭建Kafk
- Java获取本地服务器的IP
中华好儿孙
javaWeb获取服务器ip地址
System.out.println("getRequestURL:"+request.getRequestURL());
System.out.println("getLocalAddr:"+request.getLocalAddr());
System.out.println("getLocalPort:&quo