界面:
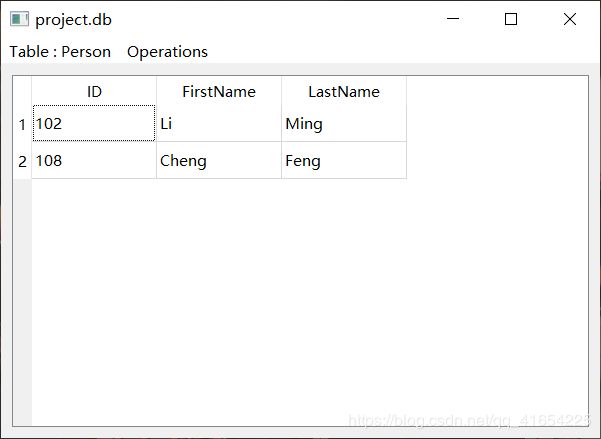


链接:https://pan.baidu.com/s/1DnwMjUlqja6n6D1b9-ZySg
提取码:lwzz
EmbeddedSystem.h
#pragma once
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include "ui_EmbeddedSystem.h"
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include