1. 模板样式
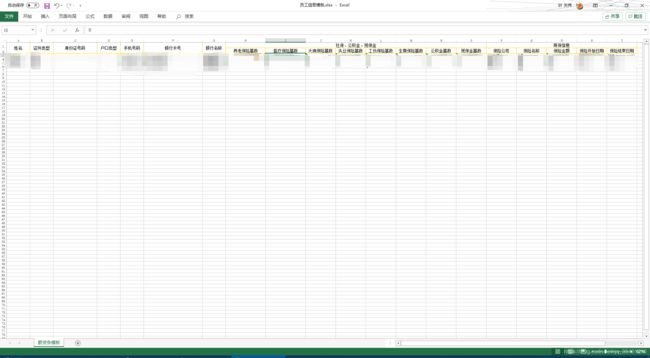
2. class
/**
* 员工信息模板
*/
@Data
public class CompanyStaffExcelDTO {
private static final String GN_FSIAOHF = "社保、公积金、残保金";
private static final String GN_INSURANCE = "商保信息";
@Excel(name = "姓名")
@NotBlank(message = "姓名不能为空")
private String name;
@Excel(name = "身份证号码")
@NotBlank(message = "身份证号码不能为空")
private String idCard;
@Excel(name = "手机号码")
@NotBlank(message = "手机号码不能为空")
private String phone;
@Excel(name = "银行卡号")
@NotBlank(message = "银行卡号不能为空")
private String bankCard;
@Excel(name = "银行名称")
@NotBlank(message = "银行名称不能为空")
private String bankName;
@Excel(name = "养老保险基数", groupName = GN_FSIAOHF)
private BigDecimal endowmentInsurance;
@Excel(name = "医疗保险基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "1", groupName = GN_FSIAOHF)
private BigDecimal medicalInsurance;
@Excel(name = "大病保险基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "2", groupName = GN_FSIAOHF)
private BigDecimal illnessInsurance;
@Excel(name = "失业保险基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "3", groupName = GN_FSIAOHF)
private BigDecimal unemploymentInsurance;
@Excel(name = "工伤保险基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "4", groupName = GN_FSIAOHF)
private BigDecimal employmentInjuryInsurance;
@Excel(name = "生育保险基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "5", groupName = GN_FSIAOHF)
private BigDecimal maternityInsurance;
@Excel(name = "公积金基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "6", groupName = GN_FSIAOHF)
private BigDecimal accumulationFund;
@Excel(name = "残保金基数", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "7", groupName = GN_FSIAOHF)
private BigDecimal residualPremium;
@Excel(name = "保险公司", groupName = GN_INSURANCE)
private String insuranceCompany;
@Excel(name = "保险名称", orderNum = "1", groupName = GN_INSURANCE)
private String insuranceName;
@Excel(name = "保险金额", type = XkjCnst.EXCEL_CELL_TYPE_NUM, numFormat = XkjCnst.EXCEL_CELL_NUM_FORMAT, orderNum = "2", groupName = GN_INSURANCE)
private BigDecimal insuranceAmount;
@Excel(name = "保险开始日期", orderNum = "3", groupName = GN_INSURANCE)
private String insuranceStartTime;
@Excel(name = "保险结束日期", orderNum = "4", groupName = GN_INSURANCE)
private String insuranceEndTime;
@ApiModelProperty(value = "作为错误信息的返回字段,不参与任何Excel的下载和上传")
private Map errors;
public static final String[] MUST_FILL = new String[]{"姓名", "身份证号码", "手机号码", "银行卡号", "银行名称"};
}
3. 实现
/**
* 公司员工导入
*/
void companyStaffImport(@RequestParam("file") MultipartFile file) {
ImportParams importParams = new ImportParams();
// 因为Excel是从第3行开始才有正式数据,所以我们这里从第2行开始读取数据
importParams.setHeadRows(2);
// 这里是做非空验证,DTO中的数组必须不为空
importParams.setImportFields(CompanyStaffExcelDTO.MUST_FILL);
// 获取模板
ExcelImportResult data;
try {
data = ExcelImportUtil.importExcelMore(file.getInputStream(), CompanyStaffExcelDTO.class, importParams);
} catch (Exception e) {
log.error(e.getMessage(), e);
return Response.failure(BizError.EXCEL_ERROR);
}
// 获取模板数据
List successList = data.getList();
int total = successList.size();
if (total == 0) {
// 如果数据量为0直接返回错误信息,不继续执行
return Response.failure(BizError.EXCEL_NO_DATA);
}
// 循环数据
for (CompanyStaffExcelDTO excelDTO : successList) {
// 取数据方式
excelDTO.getName()
// 新增员工信息
CompanyStaff companyStaff = new CompanyStaff();
// 新增员工商保信息
CompanyStaffInsurance companyStaffInsurance = new CompanyStaffInsurance();
// 新增员工社保信息
CompanyStaffSocial companyStaffSocial = new CompanyStaffSocial();
}
// 看情况返回信息
return Response.success(vo);
}