BubbleSort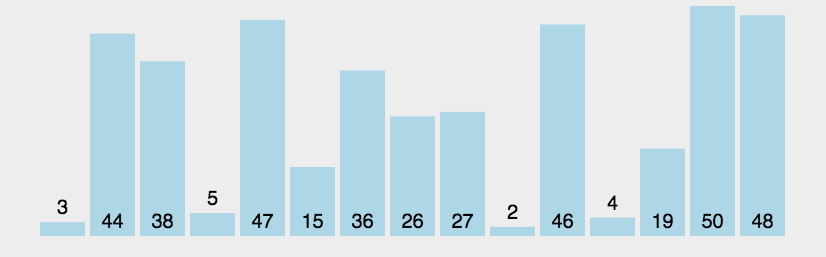
package algorithm;
public class Sort {
public static void main(String[] args) {
double[] un_sortedArray = randomArray(10,0,100);
double[] sortedArray = bubbleSort(copyArray(un_sortedArray));
System.out.println("*----------un_sortedArray----------*");
showArray(un_sortedArray);
System.out.println();
System.out.println("*----------sortedArray----------*");
showArray(sortedArray);
}
public static double[] bubbleSort(double[] arr) {
for (int i = 1; i < arr.length; i++) {
for (int j = 0; j < arr.length - i; j++) {
if (arr[j] > arr[j+1]) {
double temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
return arr;
}
public static double[] randomArray(int length, double min, double max) {
double[] randomArray = new double[length];
for (int i = 0; i < randomArray.length; i++) {
randomArray[i] = min + (max - min) * Math.random();
}
return randomArray;
}
public static double[] copyArray(double arr[]) {
double[] copyArray = new double[arr.length];
for (int i = 0; i < arr.length; i++) {
copyArray[i] = arr[i];
}
return copyArray;
}
public static void showArray(double arr[]) {
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + ",");
}
}
}