线程退出
新创建的线程从执行用户定义的函数处开始执行,直到出现以下情况时退出:
- 调用pthread_exit函数退出。
- 调用pthread_cancel函数取消该线程。
- 创建线程的进程退出或者整个函数结束。
- 其中的一个线程执行了exec类函数执行新的进程。

等待线程
退出线程示例
- #include
- #include
- #include
- #include
- void *helloworld(char *argc);
- int main(int argc,int argv[])
- {
- int error;
- int *temptr;
-
- pthread_t thread_id;
-
- pthread_create(&thread_id,NULL,(void *)*helloworld,"helloworld");
- printf("*p=%x,p=%x\n",*helloworld,helloworld);
- if(error=pthread_join(thread_id,(void **)&temptr))
- {
- perror("pthread_join");
- exit(EXIT_FAILURE);
- }
- printf("temp=%x,*temp=%c\n",temptr,*temptr);
- *temptr='d';
- printf("%c\n",*temptr);
- free(temptr);
- return 0;
- }
-
- void *helloworld(char *argc)
- {
- int *p;
- p=(int *)malloc(10*sizeof(int));
- printf("the message is %s\n",argc);
- printf("the child id is %u\n",pthread_self());
- memset(p,'c',10);
- printf("p=%x\n",p);
- pthread_exit(p);
-
- }
运行结果:
- $ ./pthread_exit_test
- *p=80486b9,p=80486b9
- the message is helloworld
- the child id is 3076361024
- p=b6c00468
- temp=b6c00468,*temp=c
- d
线程退出前操作
示例代码:
- #include
- #include
- #include
- #include
-
- void cleanup()
- {
- printf("cleanup\n");
- }
- void *test_cancel(void)
- {
- pthread_cleanup_push(cleanup,NULL);
- printf("test_cancel\n");
- while(1)
- {
- printf("test message\n");
- sleep(1);
- }
- pthread_cleanup_pop(1);
- }
- int main()
- {
- pthread_t tid;
- pthread_create(&tid,NULL,(void *)test_cancel,NULL);
- sleep(2);
- pthread_cancel(tid);
- pthread_join(tid,NULL);
- }
运行结果:
- $ ./pthread_pop_push
- test_cancel
- test message
- test message
- test message
- cleanup
一般来说,Posix的线程终止有两种情况:正常终止和非正常终止。线程主动调用pthread_exit()或者从线程函数中return都将使线程正常退出,这是可预见的退出方式;非正常终止是线程在其他线程的干预下,或者由于自身运行出错(比如访问非法地址)而退出,这种退出方式是不可预见的。
不论是可预见的线程终止还是异常终止,都会存在资源释放的问题,在不考虑因运行出错而退出的前提下,如何保证线程终止时能顺利的释放掉自己所占用的资源,特别是锁资源,就是一个必须考虑解决的问题。
最经常出现的情形是资源独占锁的使用:线程为了访问临界资源而为其加上锁,但在访问过程中被外界取消,如果线程处于响应取消状态,且采用异步方式响应,或者在打开独占锁以前的运行路径上存在取消点,则该临界资源将永远处于锁定状态得不到释放。外界取消操作是不可预见的,因此的确需要一个机制来简化用于资源释放的编程。
在POSIX线程API中提供了一个pthread_cleanup_push()/pthread_cleanup_pop()函数对用于自动释放资源 --从pthread_cleanup_push()的调用点到pthread_cleanup_pop()之间的程序段中的终止动作(包括调用 pthread_exit()和取消点终止)都将执行pthread_cleanup_push()所指定的清理函数。API定义如下:
void pthread_cleanup_push(void (*routine) (void *), void *arg)
void pthread_cleanup_pop(int execute)
pthread_cleanup_push()/pthread_cleanup_pop()采用先入后出的栈结构管理,void routine(void *arg)函数在调用pthread_cleanup_push()时压入清理函数栈,多次对pthread_cleanup_push()的调用将在清理函数栈中形成一个函数链,在执行该函数链时按照压栈的相反顺序弹出。execute参数表示执行到pthread_cleanup_pop()时是否在弹出清理函数的同时执行该函数,为0表示不执行,非0为执行;这个参数并不影响异常终止时清理函数的执行。
pthread_cleanup_push()/pthread_cleanup_pop()是以宏方式实现的,这是pthread.h中的宏定义:
#define pthread_cleanup_push(routine,arg)
{ struct _pthread_cleanup_buffer _buffer;
_pthread_cleanup_push (&_buffer, (routine), (arg));
#define pthread_cleanup_pop(execute)
_pthread_cleanup_pop (&_buffer, (execute)); }
可见,pthread_cleanup_push()带有一个"{",而pthread_cleanup_pop()带有一个"}",因此这两个函数必须成对出现,且必须位于程序的同一级别的代码段中才能通过编译。在下面的例子里,当线程在"do some work"中终止时,将主动调用pthread_mutex_unlock(mut),以完成解锁动作。
work"中终止时,将主动调用pthread_mutex_unlock(mut),以完成解锁动作。
pthread_cleanup_push(pthread_mutex_unlock, (void *) &mut);
pthread_mutex_lock(&mut);
/* do some work */
pthread_mutex_unlock(&mut);
pthread_cleanup_pop(0);
必须要注意的是,如果线程处于PTHREAD_CANCEL_ASYNCHRONOUS状态,上述代码段就有可能出错,因为CANCEL事件有可能在
pthread_cleanup_push()和pthread_mutex_lock()之间发生,或者在pthread_mutex_unlock()和pthread_cleanup_pop()之间发生,从而导致清理函数unlock一个并没有加锁的
mutex变量,造成错误。因此,在使用清理函数的时候,都应该暂时设置成PTHREAD_CANCEL_DEFERRED模式。为此,POSIX的
Linux实现中还提供了一对不保证可移植的pthread_cleanup_push_defer_np()/pthread_cleanup_pop_defer_np()扩展函数,功能与以下
代码段相当:
{ int oldtype;
pthread_setcanceltype(PTHREAD_CANCEL_DEFERRED, &oldtype);
pthread_cleanup_push(routine, arg);
...
pthread_cleanup_pop(execute);
pthread_setcanceltype(oldtype, NULL);
}
上面我用红色标记的部分是这两个函数的关键作用,我的理解就是:
pthread_cleanup_push(pthread_mutex_unlock, (void *) &mut);
pthread_mutex_lock(&mut);
/* do some work */
pthread_mutex_unlock(&mut);
pthread_cleanup_pop(0);
本来do some work之后是有pthread_mutex_unlock(&mut);这句,也就是有解锁操作,但是在do some work时会出现非正常终止,那样的话,系统会根据pthread_cleanup_push中提供的函数,和参数进行解锁操作或者其他操作,以免造成死锁!
补充:
在线程宿主函数中主动调用return,如果return语句包含在pthread_cleanup_push()/pthread_cleanup_pop()对中,则不会引起清理函数的执行,反而会导致segment fault。
为避免这种情况可设置线程的可取消状态为PTHREAD_CANCEL_DISABLE,下面将介绍设置线程的可取消状态。
取消线程
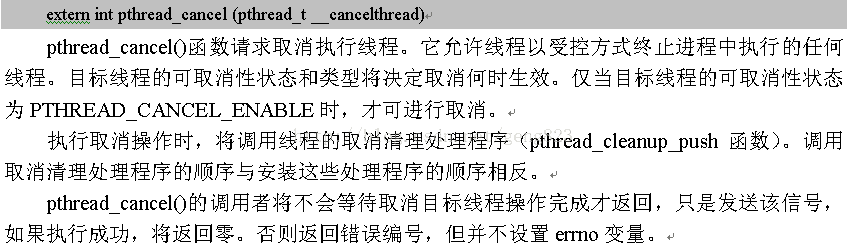
取消线程是指取消一个正在执行线程的操作,当然,一个线程能够被取消并终止执行需要满足以下条件:
该线程是否可以被其它取消,这是可以设置的,在Linux系统下,默认是可以被取消的,可用宏分配是PTHREAD_CANCEL_DISABLE和PTHREAD_CANCEL_ENABLE;
该线程处于可取消点才能取消。也就是说,该线程被设置为可以取消状态,另一个线程发起取消操作,该线程并不是一定马上终止,只能在可取消点才中止执行。可以设置为立即取消和在取消点取消。可用宏为PTHREAD_CANCEL_DEFERRED和PTHREAD_CANCEL_ASYNCHRONOUS。
设置可取消状态 (默认情况下,在创建某个线程时,其可取消性状态为PTHREAD_CANCEL_ENABLE)

可设置的state的合法值:
- 如果目标线程的可取消性状态为PTHREAD_CANCEL_DISABLE,则针对目标线程的取消请求将处于未决状态,启用取消后才执行取消请求。
- 如果目标线程的可取消性状态为PTHREAD_CANCEL_ENABLE,则针对目标线程的取消请求将被传递。默认情况下,在创建某个线程时,其可取消性状态设置为PTHREAD_CANCEL_ENABLE。
设置取消类型
pthread_setcanceltype()函数用来设置取消类型,即允许取消的线程在接收到取消操作后是立即中止还是在取消点中止,该函数声明如下:
- extern int pthread_setcanceltype (int __type, int *__oldtype)
此函数有两个参数,type为调用线程的可取消性类型所要设置的值。oldtype为存储调用线程原来的可取消性类型的地址。type的合法值包括:
如果目标线程的可取消性状态为PTHREAD_CANCEL_ASYNCHRONOUS,则可随时执行新的或未决的取消请求。
如果目标线程的可取消性状态为PTHREAD_CANCEL_DEFERRED,则在目标线程到达一个取消点之前,取消请求将一直处于未决状态。
在创建某个线程时,其可取消性类型设置为PTHREAD_CANCEL_DEFERRED。
示例代码:
- #include
- #include
- #include
- #include
-
- void *thread_function(void *arg);
-
- int main(int argc,char *argv[])
- {
- int res;
- pthread_t a_thread;
- void *thread_result;
-
- res = pthread_create(&a_thread, NULL, thread_function, NULL);
- if (res != 0)
- {
- perror("Thread creation failed");
- exit(EXIT_FAILURE);
- }
-
- printf("Cancelling thread...\n");
- sleep(10);
- res = pthread_cancel(a_thread);
- if (res != 0)
- {
- perror("Thread cancelation failed");
- exit(EXIT_FAILURE);
- }
-
- printf("Waiting for thread to finish...\n");
- sleep(10);
- res = pthread_join(a_thread, &thread_result);
- if (res != 0)
- {
- perror("Thread join failed");
- exit(EXIT_FAILURE);
- }
- exit(EXIT_SUCCESS);
- }
-
- void *thread_function(void *arg)
- {
- int i, res, j;
- sleep(1);
- res = pthread_setcancelstate(PTHREAD_CANCEL_DISABLE, NULL);
- if (res != 0)
- {
- perror("Thread pthread_setcancelstate failed");
- exit(EXIT_FAILURE);
- }
- printf("thread cancle type is disable,can't cancle this thread\n");
- for(i = 0; i <3; i++)
- {
- printf("Thread is running (%d)...\n", i);
- sleep(1);
- }
-
- res = pthread_setcancelstate(PTHREAD_CANCEL_ENABLE, NULL);
- if (res != 0)
- {
- perror("Thread pthread_setcancelstate failed");
- exit(EXIT_FAILURE);
- }
- else
- printf("Now change ths canclestate is ENABLE\n");
- sleep(200);
- pthread_exit(0);
- }
运行结果:
- $ ./pthread_cancle_example
- Cancelling thread...
- thread cancle type is disable,can't cancle this thread
- Thread is running (0)...
- Thread is running (1)...
- Thread is running (2)...
- Now change ths canclestate is ENABLE
- Waiting for thread to finish...
线程与私有数据
在多线程程序中,经常要用全局变量来实现多个函数间的数据共享。由于数据空间是共享的,因此全局变量也为所有进程共有。但有时应用程序设计中必要提供线程私有的全局变量,这个变量仅在线程中有效,但却可以跨过多个函数访问。
比如在程序里可能需要每个线程维护一个链表,而会使用相同的函数来操作这个链表,最简单的方法就是使用同名而不同变量地址的线程相关数据结构。这样的数据结构可以由 Posix 线程库维护,成为线程私有数据 (Thread-specific Data,或称为 TSD)。
主要用于:一个全局的数据,各个线程函数中需要用到这个数据并在线程函数中改变该数据的值,但是不影响主进程中对该数据的使用。假设定义一个全局的key,在主进程A中设置key=0,在A中创建两个线程B,C,线程B中让key=1,线程C中让key=2,那么如果key不设置为线程的私有数据的话,当线程BC结束后key的值就为2了,且如果C对key的赋值在B的后面执行的话,C在对key赋值之前key的值就为1了。而如果把key设置为线程私有数据的话,线程BC结束后key的值仍然为0,而且如果C对key的赋值在B的后面执行的话,C在对key赋值之前key的值仍然为0。
这里主要测试和线程私有数据有关的 4 个函数:
- pthread_key_create(); //创建私有数据,参数一为要创建的私有数据,传入的为地址,参数二为回调函数
- pthread_key_delete(); //参数为创建的私有数据
-
- pthread_getspecific(); //获取私有数据的值
- pthread_setspecific(); //设置私有数据的值
示例代码(使用全局同名变量的情况):
- #include
- #include
- #include
- #include
-
- int key=100;
- void *helloworld_one(char *argc)
- {
- printf("the message is %s\n",argc);
- key=10;
- printf("key=%d,the child id is %u\n",key,pthread_self());
- return 0;
- }
-
- void *helloworld_two(char *argc)
- {
- printf("the message is %s\n",argc);
- sleep(1);
- printf("key=%d,the child id is %u\n",key,pthread_self());
- return 0;
- }
- int main()
- {
-
- pthread_t thread_id_one;
- pthread_t thread_id_two;
-
- pthread_create(&thread_id_one,NULL,(void *)*helloworld_one,"helloworld");
- pthread_create(&thread_id_two,NULL,(void *)*helloworld_two,"helloworld");
- pthread_join(thread_id_one,NULL);
- pthread_join(thread_id_two,NULL);
- }
运行结果:
- $ ./pthread_glob_test
- the message is helloworld
- the message is helloworld
- key=10,the child id is 3075849024
- key=10,the child id is 3067456320
示例代码(使用线程私有数据的情况):
-
-
- #include
- #include
-
- pthread_key_t key=0;
- void echomsg(void *t) //回调函数, t为key
- {
- printf("destructor excuted in thread %u,param=%u\n",pthread_self(),((int *)t));
- }
-
- void * child1(void *arg)
- {
- int i=10;
- int tid=pthread_self();
- printf("\nset key value %d in thread %u\n",i,tid);
- sleep(2);
- //此时key的值仍然为0;
- pthread_setspecific(key,&i);
- printf("thread one sleep 2 until thread two finish\n");
- sleep(2);
- printf("\nthread %u returns %d,add is %u\n",tid,*((int *)pthread_getspecific(key)),(int *)pthread_getspecific(key));
- }
-
- void * child2(void *arg)
- {
- int temp=20;
- int tid=pthread_self();
- printf("\nset key value %d in thread %u\n",temp,tid);
- pthread_setspecific(key,&temp);
- sleep(1);
- printf("thread %u returns %d,add is %u\n",tid,*((int *)pthread_getspecific(key)),(int *)pthread_getspecific(key));
- }
-
- int main(void)
- {
- pthread_t tid1,tid2;
- pthread_key_create(&key,echomsg);//echomsg为回调函数,当线程执行完成后调用echomsg,echomsg的参数t则为key,
- pthread_create(&tid1,NULL,(void *)child1,NULL);
- pthread_create(&tid2,NULL,(void *)child2,NULL);
- pthread_join(tid1,NULL);
- pthread_join(tid2,NULL);
- //此时key的值仍然为0
- pthread_key_delete(key);
- return 0;
- }
运行结果:
- $ ./pthread_key_test
-
- set key value 20 in thread 3067337536
-
- set key value 10 in thread 3075730240
- thread one sleep 2 until thread two finish
- thread 3067337536 returns 20,add is 3067335512
- destructor excuted in thread 3067337536,param=3067335512
-
- thread 3075730240 returns 10,add is 3075728216
- destructor excuted in thread 3075730240,param=3075728216
原文链接:
http://blog.csdn.net/geng823/article/details/41285251