可视化作业
作业1代码
x = np.linspace(-10,10,50)
y = np.sin(x)
plt.plot(x,y,'bo:',markerfacecolor = 'r', markersize = 10)
plt.title('My Matplotlib pictures')
plt.xlabel('x-axis')
plt.ylabel('y-axis')
plt.annotate("(-5,0)",xy=(-5,0),xytext=(-2.5,0.25),arrowprops=dict(facecolor='green',headwidth = 20, headlength = 20))
plt.annotate("(0,0)",xy=(0,0))
plt.grid()
plt.show()
结果如下:
作业2
plt.figure(dpi=600)
plt.title('不同果酱面包配料百分比')
elements = ['面粉','砂糖','奶油','果酱','坚果']
a = [0.4,0.15,0.2,0.1,0.15]
b = [0.3,0.25,0.2,0.1,0.15]
colors = ['skyblue','green','blue','orange','pink']
plt.pie(a,autopct="%1d%%",colors = colors,pctdistance=0.8,
wedgeprops = {'linewidth':1, 'edgecolor':'white'})
plt.pie(b,radius=0.7,autopct="%1d%%",colors = colors,pctdistance=0.8,
wedgeprops = {'linewidth':1.5, 'edgecolor':'white'})
plt.pie([1],colors='w',radius=0.4)
plt.legend(elements, fontsize = 10, title = '配料表')
plt.show()
结果如下:
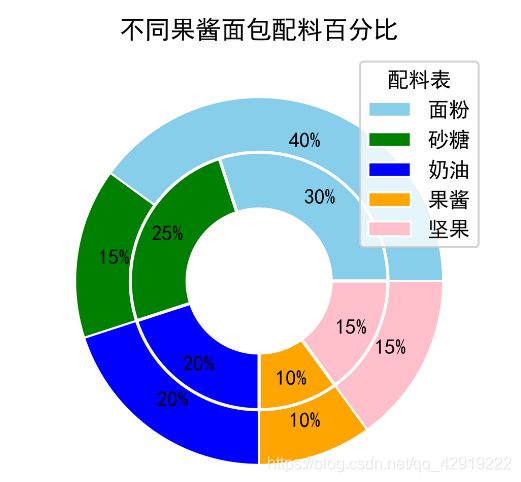
作业3
ax1 = plt.subplot2grid((3,3),(0,1))
ax2 = plt.subplot2grid((3,3),(1,1))
ax3 = plt.subplot2grid((3,3),(0,0),rowspan=3)
ax4 = plt.subplot2grid((3,3),(2,1),colspan=2)
ax5 = plt.subplot2grid((3,3),(0,2),rowspan=2)
结果如下:
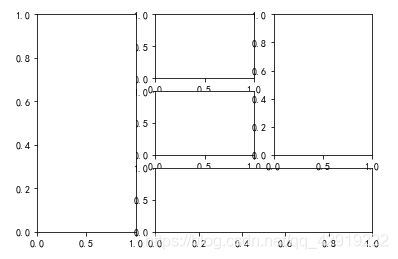
作业4
import numpy as np
from matplotlib.patches import Circle, Wedge, Polygon, Ellipse
from matplotlib.collections import PatchCollection
import matplotlib.pyplot as plt
fig,ax = plt.subplots()
patches = []
leftstripe = Wedge((0.46,0.5),0.15,90,100)
midstripe = Wedge((0.5,0.5),0.15,85,95)
rightstripe = Wedge((0.54,0.5),0.15,80,90)
lefteye = Wedge((0.36,0.46),0.06, 0, 360,width=0.03)
righteye = Wedge((0.63,0.46),0.06, 0, 360,width=0.03)
nose = Wedge((0.5,0.32),0.08,75,105,width=0.03)
mouthleft = Wedge((0.44,0.4),0.08,240,320,width=0.01)
mouthright = Wedge((0.56,0.4),0.08,220,300,width=0.01)
patches += [leftstripe, midstripe, rightstripe,
lefteye, righteye, nose,
mouthleft, mouthright]
leftiris = Circle((0.36,0.46),0.04)
rightiris = Circle((0.63,0.46),0.04)
patches += [leftiris,rightiris]
leftear = Polygon([[0.2,0.6],[0.3,0.8],[0.4,0.64]],True)
rightear = Polygon([[0.6,0.64],[0.7,0.8],[0.8,0.6]],True)
topleftwhisker = Polygon([[0.01,0.4],[0.18,0.38],[0.17,0.42]],True)
bottomleftwhisker = Polygon([[0.01,0.3],[0.18,0.32],[0.2,0.28]],True)
toprightwhisker = Polygon([[0.99,0.41],[0.82,0.39],[0.82,0.43]],True)
bottomrightwhisker = Polygon([[0.99,0.31],[0.82,0.33],[0.81,0.29]],True)
patches += [leftear,rightear,
topleftwhisker,bottomleftwhisker,
toprightwhisker,bottomrightwhisker]
body= Ellipse((0.5,-0.18),0.6,0.8)
patches.append(body)
colors = 100*np.random.rand(len(patches))
p = PatchCollection(patches, alpha = 0.4)
p.set_array(np.array(colors))
ax.add_collection(p)
plt.show()
结果如下:
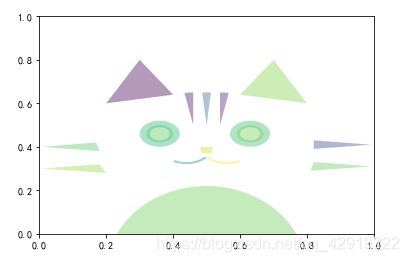