字符流
解析:
1.一个字符一个字符的读
2.只能用来操作字符(不能写图片 音频 视频)
Windows系统 一个中文字符 占2字节
默认使用GBK的编码表(简体中文)
FileWirter
构造方法(绑定写入的路径)
2.测试字符流输入的内容
public class text {
public static void main(String[] args) throws IOException {
FileWriter f = new FileWriter("/Users/lanou/Desktop/test/haha.txt");
f.write(100);
f.flush();
char[] c = {'a','b','c','d','a','q'};
f.write(c);
f.flush();
f.write(c,1,3);
f.flush();
f.write("写个格式\n格式单位");
f.flush();
f.write("大小多少",1,2);
f.flush();
f.close();
}
}
注意:关闭程序一定要写不然写入无法停止
4.循环读取
public class Demo05 {
public static void main(String[] args) throws IOException{
FileReader fr = new FileReader("/Users/lanou/Desktop/test/haha.txt");
int len;
char[] ch = new char[1024];
while((len = fr.read(ch))!= -1) {
System.out.println(new String(ch, 0, len));
}
}
}
注意:
写的时候可以直接写入字符串
读的时候不能 因为 字符串很难界定到哪里结束 不太容易判断一个字符串
3.复制文件的时间(使用模板设计模式)
public class Demo11 {
}
abstract class Fun{
public static long getFun1() {
System.out.println("系统计时已开始");
long start = System.currentTimeMillis();
return start;
}
public abstract void copy(File file1,File file2);
public static long getFun2() {
System.out.println("系统计时已结束");
long stop = System.currentTimeMillis();
return stop;
}
}
class Fun1 extends Fun{
@Override
public void copy(File file1,File file2) {
try {
FileWriter fw = new FileWriter(file2);
FileReader fr = new FileReader(file1);
int len = 0;
char[] cs = new char[1024];
while((len = fr.read(cs)) != -1) {
fw.write(cs);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
4.字符流的两种读取文件方式(单个字节读/字符数组读)
public class Demo10 {
public static void main(String[] args) {
readByCha(new File("/Users/lanou/Desktop/test/znb.txt"));
}
public static void readByCha(File file) {
FileReader fr = null;
try {
fr = new FileReader(file);
int len = 0;
char[] c = new char[1024];
while((len = fr.read(c))!= -1) {
System.out.println(new String(c, 0, len));
}
} catch (FileNotFoundException e) {
throw new RuntimeException("打开失败");
} catch (IOException e) {
throw new RuntimeException("读取失败");
}finally {
if(fr != null) {
try {
fr.close();
} catch (IOException e) {
System.out.println("文件关闭失败");
}
}
}
}
public static void readByChar(File file) {
FileReader fr = null;
try {
fr = new FileReader(file);
int length = 0;
while((length = fr.read())!= -1) {
System.out.println((char)length);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("连接文件失败");
} catch (IOException e) {
throw new RuntimeException("读取文件失败");
}finally {
if(fr != null) {
try {
fr.close();
} catch (IOException e) {
throw new RuntimeException("关闭异常");
}
}
}
}
@SuppressWarnings("resource")
public static void readFromFileByByt(File file) {
FileInputStream fis = null;
try {
fis = new FileInputStream(file);
int len = 0;
byte[] bs = new byte[1024];
while((len = fis.read(bs)) != -1) {
System.out.println(new String(bs,0,len));
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件不存在");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}finally {
if(fis != null) {
try {
fis.close();
} catch (IOException e) {
System.out.println("文件关闭失败");
}
}
}
}
@SuppressWarnings("resource")
public void readFromFileByByte(File file) {
FileInputStream fis = null;
try {
fis = new FileInputStream(file);
int len = 0;
while((len = fis.read()) != -1) {
System.out.println((char)len);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件不存在");
} catch (IOException e) {
throw new RuntimeException("文件读取失败");
}finally {
if(file != null) {
try {
fis.close();
} catch (IOException e) {
System.out.println("文件关闭失败");
}
}
}
}
}
6.将一个文件夹下的所有txt文件 复制 到另一个文件夹下 并且把txt改成java
public class Demo09 {
public static void main(String[] args) throws IOException {
File file1 = new File("/Users/lanou/Desktop/test");
File file2 = new File("/Users/lanou/Desktop/test3");
copyDirToAnotherFile(file1, file2);
}
public static void copyDirToAnotherFile(File src,File dest) throws IOException {
File[] files = src.listFiles(new MyFileFilter());
for (File subFile : files) {
if(subFile.isFile()) {
String name = subFile.getName();
int lastIndexOf = name.lastIndexOf(".");
String substring = name.substring(0, lastIndexOf);
String string = substring + ".java";
File file = new File(dest,string);
file.createNewFile();
}else {
copyDirToAnotherFile(subFile, dest);
}
}
}
}
class MyFileFilter implements FileFilter{
@Override
public boolean accept(File pathname) {
if(pathname.isDirectory()) {
return true;
}
return pathname.getName().endsWith("txt");
}
}
2.转换流
OutputStreamWriter(字符流转向字节流)
作用:不同编码格式写入
需要使用到 FileOutputStream 类
OutputStream 字节流父类
Writer 字符流父类
InputStreamReader
作用:可以读取不同编码格式的文件
需要使用到FileInputStream
public class Demo07 {
public static void main(String[] args) throws IOException {
readGBK();
}
public static void readUTF8() throws IOException {
FileInputStream fls = new FileInputStream("/Users/lanou/Desktop/test/utf8.txt");
InputStreamReader isr = new InputStreamReader(fls);
char[] c = new char[1024];
int len;
while((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
public static void readGBK() throws IOException {
FileInputStream fis = new FileInputStream("/Users/lanou/Desktop/test/gbk.txt");
InputStreamReader isr = new InputStreamReader(fis, "GBK");
char[] c = new char[1024];
int len;
while((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
public static void getUTF8() throws IOException{
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/test/utf8.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos);
osw.write("窗前");
osw.close();
}
public static void getGBK() throws IOException {
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/test/gbk.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "GBK");
osw.write("明月");
osw.close();
}
}
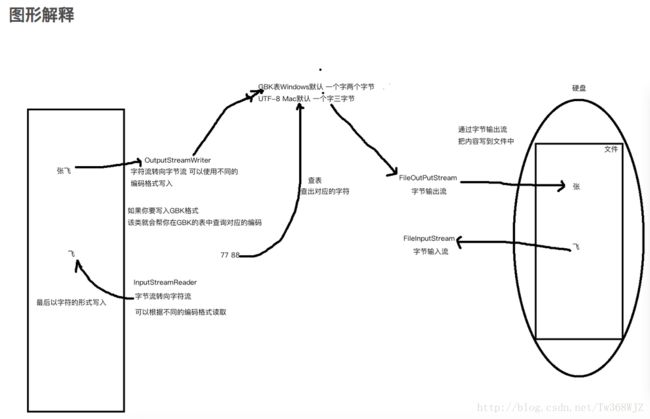