- 企业内网系统:从传统开发到智能赋能的进化之路
飞算JavaAI开发助手
科技人工智能大数据java
在当今数字化浪潮中,企业内网系统作为支撑日常运营的核心基础设施,其开发效率与质量直接关系到企业的竞争力。传统开发模式下,程序员需要手动完成需求分析、架构设计、代码编写、测试调试等全流程工作,不仅耗时费力,还容易因人为疏忽导致质量隐患。而随着人工智能技术的突破性进展,以飞算JavaAI为代表的智能开发工具正在重塑企业内网系统的开发范式,为程序员提供从设计到落地的全链路智能支持。一、传统企业内网系统开
- MCP协议采用客户端-服务器架构的深层逻辑与架构对比分析
一、架构选择的核心动因1.功能解耦与安全边界的强制性要求MCP采用客户端-服务器(C/S)架构的核心动因源于AI系统与真实世界交互的特殊性:权限分层控制:主机(Host)作为协调层,严格划分客户端(Client)与服务端(Server)的操作权限。例如医疗场景中,诊断模型(Client)仅能通过医院授权的主机访问脱敏病历服务器,无法直接接触原始数据。沙箱隔离需求:每个MCP服务器运行在独立容器中(
- TDengine 集群部署及启动、扩容、缩容常见问题与解决方案
TDengine (老段)
TDengine运维tdengine大数据时序数据库数据库物联网涛思数据iot
一、部署阶段常见问题及解决方案1.dockerentrypoint.sh相关bug问题描述:在特定docker-compose.yaml文件下无法自动建立集群,原因是Docker镜像entrypoint文件/usr/bin/entrypoint.sh执行代码无法抓取出FIRST_EP信息(TDengine3.3.6.3版本)。解决方案:需检查taosd-C执行结果是否正常获取FIRST_EP,并确
- 时序数据库 TDengine × SSRS:专为工业、能源场景打造的报表解决方案
每当听到“做报表”三个字,是不是内心都会先叹口气?尤其在工业、能源、制造等场景,面对那些结构固定、字段繁多、格式要求严苛的报表任务,用Excel手动拼,真的是既费时又容易出错。现在解决方案来了——时序数据库TDengine与SQLServerReportingServices(SSRS)已经完成无缝集成!高性能时序数据库+企业级报表平台,帮你用更少的操作、更高的效率,制作出更稳定、更规范的专业报表
- 京东携手HarmonyOS SDK首发家电AR高精摆放功能
在电商行业的演进中,商品的呈现方式不断升级:从文字、图片到视频,再到如今逐渐兴起的3D与AR技术。作为XR应用探索的先行者,京东正站在这场体验革新的最前沿,不断突破商品展示的边界,致力于通过创新技术让消费者的选购过程更加直观、真实和高效。“3D技术能够提供更逼真的视觉呈现、更沉浸的交互体验,让消费者"所见即所得”,帮助品牌更好实现与用户的深入连接,“3D信息流"将成为下一代内容形态的重要载体。”-
- Docker 高级管理 -- 容器通信技术与数据持久化
婷儿z
docker容器运维
目录第一节:容器通信技术一:Docker容器的网络模式1:Bridge模式2:Host模式3:Container模式4:None模式5:Overlay模式6:Macvlan模式7:自定义网络模式二:端口映射关键对比三:容器互联关键对比四:容器间通信实现案例1.网络创建选项2.容器通信实现步骤3.通信方式对比第二节:数据持久化技术一:Docker的数据管理1.数据卷核心概念2.数据卷核心作用3.数据
- Seaborn高阶玩法全解析:从复杂图表到多图布局的可视化实战指南
数据可视化就像给数据“画肖像”——初级阶段是勾勒轮廓,高级阶段则是赋予灵魂。在Python可视化生态中,Seaborn凭借“一行代码出美图”的优雅,成为数据分析的“画笔利器”。但你是否遇到过这样的场景:想同时展示数据分布与统计量,却被基础图表限制;想批量绘制分面图,手动拼接效率低下;想让图表更具设计感,却对颜色搭配和注解技巧一知半解?本文将带你解锁Seaborn的高阶玩法,从复杂图表绘制到多图布局
- 从0到1:SQL注入与XSS攻防实战——数据库安全加固全攻略
小张在编程
sqlxss数据库
引言2023年某电商平台用户数据泄露事件中,黑客仅用一行username='OR'1'='1的登录输入,就拖走了百万用户信息;另一家社交网站更离谱,用户在评论区输入alert('xss'),竟让千万级用户的浏览器成了“提线木偶”。这些看似简单的攻击,为何能撕开企业安全防线?今天我们就来拆解SQL注入与XSS的“作案手法”,并给出一套可落地的数据库安全加固方案——毕竟,防住这两类攻击,能解决80%的
- 使用 p6spy,拦截到持久层执行的sql及参数
Peter-OK
一些问题p6spysql
声明:文章内容是自己使用后整理,大部分工具代码出自大牛,但因无法确认出处,故仅在此处由衷的对无私分享源代码的作者表示感谢与致敬!本人在拦截到sql的基础上加了分析功能和异常告警功能1、导入p6spy的jar包,如果是maven项目引入pomp6spyp6spy3.9.12、修改datasource数据源的driverClassName驱动和url地址为com.p6spy.engine.spy.P6
- 2.Spring Cloud生态全景解析:核心组件、能力边界与定位
碎风影
SpringCloud深度解析springcloudspring后端
导语:SpringCloud并非单一框架,而是基于SpringBoot构建的分布式系统工具集。它通过标准化封装,将服务发现、配置管理、熔断限流等复杂基础设施转化为开箱即用的组件,让开发者聚焦业务逻辑。本文将系统解析其核心组成、与SpringBoot的共生关系,并客观审视其能力边界,助您构建清晰的微服务技术选型地图。一、核心基石:SpringBoot与SpringCloud的共生关系关键结论:Spr
- uniapp [安卓苹果App端] - 实现获取请求手机位置权限+开启定位获取经纬度/省市区地址等,检测权限手机定位是否开启并引导用户同意授权,uniApp app端调用本机开启本机定位权限及IP属地
前端开发大师鸭
+Uniapp开发问题汇总uni-app手机定位权限手机位置权限uniapp安卓苹果系统权限用户拒绝定位权限后怎么办开启位置并获取IP定位数据经纬度及省市区县详细地址数据
前言网上的教程乱七八糟且兼容性太差,本文提供优质示例。在uni-appApp端(安卓APP|苹果APP)开发中,详解在app平台端实现获取手机位置权限及开启定位功能(原生实现不依赖第三方插件和地图),有权限则开启位置定位获取用户经纬度+IP属地+省市区县详细地址数据等操作,反之无权限则提示开启位置权限与引导用户授权操作,uniAppapp端实现判断是否拥有定位权限及提示引导用户授权同意,完美兼容安
- 从实验到文化 - “混沌日”与持续混沌
weixin_42587823
混沌数据库混沌
从实验到文化-“混沌日”与持续混沌第一部分:锻炼团队的“免疫系统”-混沌日(GameDay)什么是“混沌日”?混沌日是一场有计划、有组织的演习活动。在活动中,团队成员们齐聚一堂(无论是线上还是线下),在一个受控的环境中(理想情况是生产环境,但从预生产环境开始是更安全的选择),主动触发一次模拟的真实故障场景。它就像一次针对技术团队和系统的消防演习。它的价值何在?混沌实验不仅仅测试机器,它同样也测试人
- Zsh中PATH环境变量错误的报错与别名配置实战指南
喜欢编程就关注我
javapython前端Zsh中PATH环境变量错误的报错与别名配置实战指南代码
Zsh中PATH环境变量错误的报错与别名配置实战指南一、PATH环境变量错误诊断矩阵1.1常见错误类型错误现象典型报错信息根本原因解决方案命令未找到zsh:commandnotfound:xxxPATH未包含命令所在目录检查PATH配置路径重复无报错但路径列表冗余多次添加相同路径使用数组去重权限问题zsh:permissiondenied:/usr/local路径目录无执行权限调整目录权限特殊字符
- scanpy保存图片的常用方法汇总
Bio Coder
空间转录组&单细胞scanpy保存图片汇总
在使用Scanpy(一个用于单细胞RNA测序数据分析的Python库)时,保存图片(如可视化结果)是常见的操作。Scanpy的绘图功能主要基于Matplotlib和Seaborn,保存图片的方法也与这些库的保存机制一致。以下是Scanpy保存图片的详细方法及注意事项:1.基本保存图片的方法Scanpy的绘图函数(如sc.pl.umap、sc.pl.tsne、sc.pl.pca等)通常会返回Matp
- 服务注册和发现组件的详细对比与选型建议(详细版)
古龙飞扬
springcloudspring后端
服务注册和发现组件Eureka、Consul、ZooKeeper、Etcd和Nacos的区别与选型建议在微服务架构中,服务注册与发现是一个核心组件,它解决了服务实例的动态管理和自动发现的问题。目前,市场上存在多种服务注册与发现组件,其中Eureka、Consul、ZooKeeper、Etcd和Nacos较为常见。作为资深的软件架构师,本文将详细分析这些组件的区别,并提供选型建议。一、EurekaE
- k8s深度讲解----宏观架构与集群之脑 - API Server 和 etcd
weixin_42587823
云原生kubernetes架构etcd
宏观架构与集群之脑-APIServer和etcd宏观架构:数据中心的操作系统在开始之前,让我们先建立一个高层视角。你可以将Kubernetes想象成一个管理整个数据中心的分布式操作系统。在这个操作系统中:控制平面(ControlPlane)就是它的“内核”,负责管理和决策。工作节点(WorkerNodes)就是它的“CPU和内存”,是真正运行应用程序的地方。我们常用的kubectl就是与这个“内核
- MCP Streamable HTTP 样例(qbit)
pythonagent
前言模型上下文协议(ModelContextProtocol,MCP),是由Anthropic推出的开源协议,旨在实现大语言模型与外部数据源和工具的集成,用来在大模型和数据源之间建立安全双向的连接。本文代码技术栈Python3.11.8FastMCP2.10.3MCP的传输机制StandardInput/Output(stdio)StreamableHTTPServer-SentEvents(SS
- Spring框架中的Component与Bean注解
SpringBoot中的@Bean与@Component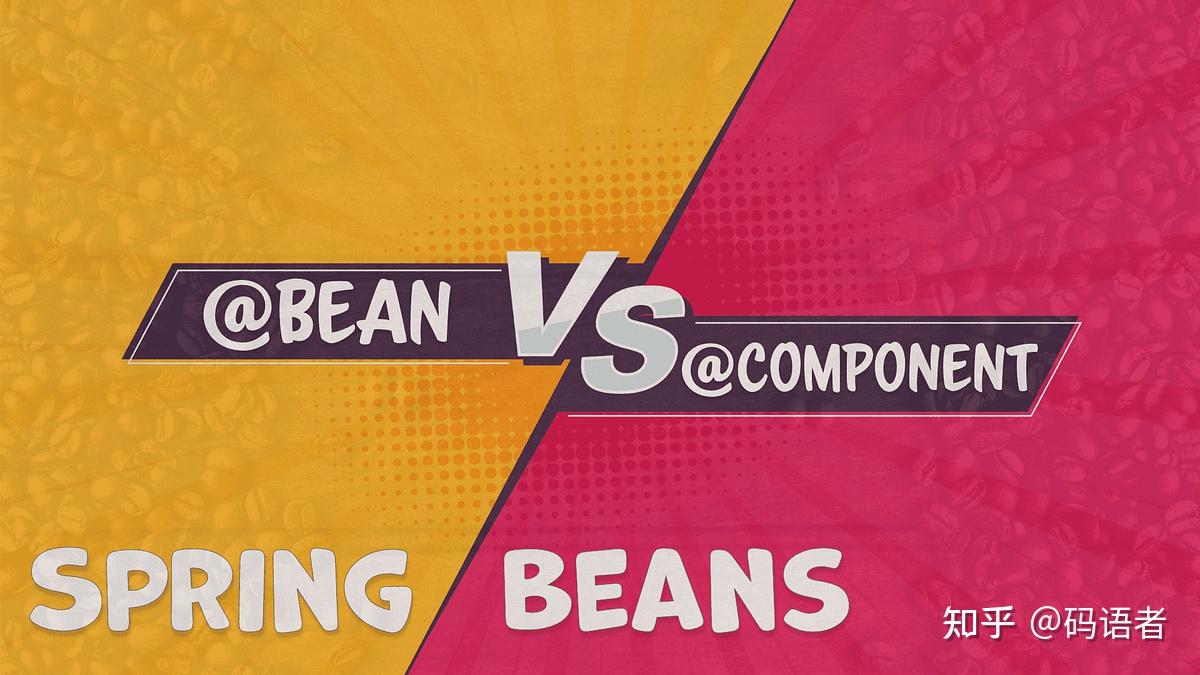Spring的@Component和@Bean注解的关键区别在于:@Bean注解可用于暴露您自己编写的JavaBeans,而@Component注解可用于暴露源代码由他人维护的JavaBeans。Sprin
- C++ 工厂模式与抽象工厂:创建对象的灵活设计
海派程序猿
c++javajvm
C++工厂模式与抽象工厂:让对象“流水线”更优雅想象一下,你是一家玩具工厂的老板,主要生产两种玩具:小汽车和积木。最初,你的生产流程很简单,需要什么就直接用new创建什么://生产小汽车Car*myCar=newCar();//生产积木Block*myBlock=newBlock();简单粗暴,效率很高,就像直接从仓库里抓取零件组装一样。但问题也随之而来:耦合度高:生产代码直接依赖于具体的Car和
- 掌握变量命名与Python继承机制
掌握变量命名与Python继承机制背景简介在编程中,变量命名和继承是基础且重要的概念。良好的命名习惯可以提升代码的可读性,而继承则是一种代码复用的重要机制。本文将结合具体的书籍章节内容,深入解析变量命名规则和Python继承机制。变量命名规则变量命名是编程中最基础的部分,而正确的命名习惯能够帮助其他开发者(或未来的自己)更好地理解代码。根据书籍提供的内容,我们应当遵守以下规则:变量名只包含数字、下
- uiautomatorviewer工具在Android 9.0上的应用及优势
小馬锅
本文还有配套的精品资源,点击获取简介:uiautomatorviewer是AndroidSDK中的自动化测试和UI分析工具,特别适用于Android9.0版本。它支持扫描和分析应用UI控件,获取关键UI元素信息以编写自动化测试脚本。工具采用XPath定位技术,对于复杂布局中的UI元素精确定位尤为有效。同时,uiautomatorviewer与Appium自动化测试框架在功能上具有重叠,但各有优势。
- 从实践到自动化:现代运维管理的转型与挑战
运维
从实践到自动化:现代运维管理的转型与挑战在信息化快速发展的今天,企业IT系统的稳定性、可用性和安全性已成为衡量公司竞争力的重要因素之一。运维(IT运维)管理作为确保企业IT系统健康、稳定运行的关键职能,一直是企业技术团队关注的重点。然而,随着业务的复杂化、用户需求的变化以及技术的不断创新,传统的运维方式已逐渐无法满足企业对于高效、高可用、高安全的需求。如何提升运维效率、减少人为错误、提高运维系统的
- 华为手机手机与计算机传输,如何将华为手机的视频传到华为的电脑上?手机与电脑数据互传操作步骤...
人人保
华为手机手机与计算机传输
手机与电脑数据互传操作bai步骤如下:1、手机du通过原装USB数据线与电脑相连,待zhi电脑自行dao安装驱动,并确认驱动安装成功,如下图所示:注:如驱动未安装成功,可通过安装HiSuite软件进行辅助驱动安装或者通过选择端口模式中的帮助进行电脑驱动安装。(1)在手机端弹出的对话框选择“是,访问数据”(2)在手机下拉菜单中USB连接方式中选择设备文件管理(MTP)注:关于设备文件管理(MTP)和
- DMA技术与音频数据的存储和播放
曹小满2579
Android基础音视频Android
基本概念采样率:每秒采集的采样点次数。如480000HZ,就是我们常见的48KHZ采样点(Sample):每一个采样点代表一个时间点的声音幅度值。对于立体声,每个采样点包含了两个声道(左声道,右声道)的数据。帧:一帧就是一个时刻采集的数据,如果音频是立体声则会产生2个采样点,如果是更复杂的比如5.1,则会产生更多的采样点。例如PCM数据是48KHZ,16bit的,立体声,则一秒的PCM数据有48K
- 稀缺工具,效率拉满!
在办公场景中,图像和文档是最常接触的两类文件类型。日常工作中经常需要对多个文件进行批量处理,如图片转文档、PDF文件空白页删除、PDF转双层、图片校正等操作。这些重复性操作如果逐个处理不但效率极低下,还可能出现错误,而利用批量操作工具。可以快速完成大量文件的批量操作;分享一款高效的文档、图片批量操作工具:图档批处理助手v1.2.1;图档批处理助手是一款专注于高效处理文档与图像批任务的轻量级工具,软
- Flex与Spring集成
hkmw
Flex配置springflexapplicationdependenciescomponentsaccess
Flex与Spring集成UsingFlexwithSpringUPDATE(1/12/2007):IputtogetheraTomcat-basedTestDriveServerthatincludesthesamplesdescribedbelowrunningout-of-thebox.Readthispostformoreinfo.WhatisSpring?Springisoneofthe
- Ubuntu 与 Windows 实现文件夹共享
懒羊羊大王呀
LinuxwindowsLinuxSamba文件夹共享
Ubuntu20.04与Windows实现文件夹共享Linux中Samba的下载与配置sudoupdateapt#更新工具包sudoaptinstallsamba#下载Sambasudocp/etc/samba/smb.conf/etc/samba/smb.conf.bak#尽量备份一下sudovim/etc/samba/smb.conf#修改配置文件#添加以下内容,其中[shared]#共享文件
- 量子计算的数学地基:解码希尔伯特空间的魔法
牧之112
量子计算
在科技圈,“量子计算”早已不是陌生的名词。从谷歌的“量子霸权”实验到IBM的量子云服务,从药物研发的分子模拟到密码学的革命性突破,量子计算正以颠覆式的姿态重塑着人类对计算的认知。但在这些令人惊叹的应用背后,藏着一个关键的数学基石——希尔伯特空间(HilbertSpace)。它像一片隐形的“量子舞台”,支撑着量子比特的叠加、纠缠与计算,是理解量子计算本质绕不开的概念。一、从“普通空间”到“量子空间”
- 【视频观看系统】- 技术与架构选型
✅项目技术选型方案一、整体架构风格项目层级技术选型说明架构风格微服务架构(SpringCloud)独立部署、易扩展、易维护服务通信HTTP(RestTemplate或Feign)+RocketMQ同步调用+异步事件注册中心Nacos服务注册、发现、配置中心配置中心Nacos配置管理多服务统一配置API网关SpringCloudGateway路由转发、权限验证、限流服务监控SpringBootAdm
- Readr 项目安装与配置指南
芮奕滢Kirby
Readr项目安装与配置指南readr项目地址:https://gitcode.com/gh_mirrors/rea/readr1.项目基础介绍readr是一个R语言的开源项目,由HadleyWickham创建和维护。该项目的主要目的是提供一种快速且友好的方式来读取分隔文件(如CSV和TSV)中的矩形数据。readr能够解析多种数据类型,并在解析过程中提供详细的错误报告,以便用户能够快速识别和解决
- 关于旗正规则引擎下载页面需要弹窗保存到本地目录的问题
何必如此
jsp超链接文件下载窗口
生成下载页面是需要选择“录入提交页面”,生成之后默认的下载页面<a>标签超链接为:<a href="<%=root_stimage%>stimage/image.jsp?filename=<%=strfile234%>&attachname=<%=java.net.URLEncoder.encode(file234filesourc
- 【Spark九十八】Standalone Cluster Mode下的资源调度源代码分析
bit1129
cluster
在分析源代码之前,首先对Standalone Cluster Mode的资源调度有一个基本的认识:
首先,运行一个Application需要Driver进程和一组Executor进程。在Standalone Cluster Mode下,Driver和Executor都是在Master的监护下给Worker发消息创建(Driver进程和Executor进程都需要分配内存和CPU,这就需要Maste
- linux上独立安装部署spark
daizj
linux安装spark1.4部署
下面讲一下linux上安装spark,以 Standalone Mode 安装
1)首先安装JDK
下载JDK:jdk-7u79-linux-x64.tar.gz ,版本是1.7以上都行,解压 tar -zxvf jdk-7u79-linux-x64.tar.gz
然后配置 ~/.bashrc&nb
- Java 字节码之解析一
周凡杨
java字节码javap
一: Java 字节代码的组织形式
类文件 {
OxCAFEBABE ,小版本号,大版本号,常量池大小,常量池数组,访问控制标记,当前类信息,父类信息,实现的接口个数,实现的接口信息数组,域个数,域信息数组,方法个数,方法信息数组,属性个数,属性信息数组
}
&nbs
- java各种小工具代码
g21121
java
1.数组转换成List
import java.util.Arrays;
Arrays.asList(Object[] obj); 2.判断一个String型是否有值
import org.springframework.util.StringUtils;
if (StringUtils.hasText(str)) 3.判断一个List是否有值
import org.spring
- 加快FineReport报表设计的几个心得体会
老A不折腾
finereport
一、从远程服务器大批量取数进行表样设计时,最好按“列顺序”取一个“空的SQL语句”,这样可提高设计速度。否则每次设计时模板均要从远程读取数据,速度相当慢!!
二、找一个富文本编辑软件(如NOTEPAD+)编辑SQL语句,这样会很好地检查语法。有时候带参数较多检查语法复杂时,结合FineReport中生成的日志,再找一个第三方数据库访问软件(如PL/SQL)进行数据检索,可以很快定位语法错误。
- mysql linux启动与停止
墙头上一根草
如何启动/停止/重启MySQL一、启动方式1、使用 service 启动:service mysqld start2、使用 mysqld 脚本启动:/etc/inint.d/mysqld start3、使用 safe_mysqld 启动:safe_mysqld&二、停止1、使用 service 启动:service mysqld stop2、使用 mysqld 脚本启动:/etc/inin
- Spring中事务管理浅谈
aijuans
spring事务管理
Spring中事务管理浅谈
By Tony Jiang@2012-1-20 Spring中对事务的声明式管理
拿一个XML举例
[html]
view plain
copy
print
?
<?xml version="1.0" encoding="UTF-8"?>&nb
- php中隐形字符65279(utf-8的BOM头)问题
alxw4616
php中隐形字符65279(utf-8的BOM头)问题
今天遇到一个问题. php输出JSON 前端在解析时发生问题:parsererror.
调试:
1.仔细对比字符串发现字符串拼写正确.怀疑是 非打印字符的问题.
2.逐一将字符串还原为unicode编码. 发现在字符串头的位置出现了一个 65279的非打印字符.
 
- 调用对象是否需要传递对象(初学者一定要注意这个问题)
百合不是茶
对象的传递与调用技巧
类和对象的简单的复习,在做项目的过程中有时候不知道怎样来调用类创建的对象,简单的几个类可以看清楚,一般在项目中创建十几个类往往就不知道怎么来看
为了以后能够看清楚,现在来回顾一下类和对象的创建,对象的调用和传递(前面写过一篇)
类和对象的基础概念:
JAVA中万事万物都是类 类有字段(属性),方法,嵌套类和嵌套接
- JDK1.5 AtomicLong实例
bijian1013
javathreadjava多线程AtomicLong
JDK1.5 AtomicLong实例
类 AtomicLong
可以用原子方式更新的 long 值。有关原子变量属性的描述,请参阅 java.util.concurrent.atomic 包规范。AtomicLong 可用在应用程序中(如以原子方式增加的序列号),并且不能用于替换 Long。但是,此类确实扩展了 Number,允许那些处理基于数字类的工具和实用工具进行统一访问。
 
- 自定义的RPC的Java实现
bijian1013
javarpc
网上看到纯java实现的RPC,很不错。
RPC的全名Remote Process Call,即远程过程调用。使用RPC,可以像使用本地的程序一样使用远程服务器上的程序。下面是一个简单的RPC 调用实例,从中可以看到RPC如何
- 【RPC框架Hessian一】Hessian RPC Hello World
bit1129
Hello world
什么是Hessian
The Hessian binary web service protocol makes web services usable without requiring a large framework, and without learning yet another alphabet soup of protocols. Because it is a binary p
- 【Spark九十五】Spark Shell操作Spark SQL
bit1129
shell
在Spark Shell上,通过创建HiveContext可以直接进行Hive操作
1. 操作Hive中已存在的表
[hadoop@hadoop bin]$ ./spark-shell
Spark assembly has been built with Hive, including Datanucleus jars on classpath
Welcom
- F5 往header加入客户端的ip
ronin47
when HTTP_RESPONSE {if {[HTTP::is_redirect]}{ HTTP::header replace Location [string map {:port/ /} [HTTP::header value Location]]HTTP::header replace Lo
- java-61-在数组中,数字减去它右边(注意是右边)的数字得到一个数对之差. 求所有数对之差的最大值。例如在数组{2, 4, 1, 16, 7, 5,
bylijinnan
java
思路来自:
http://zhedahht.blog.163.com/blog/static/2541117420116135376632/
写了个java版的
public class GreatestLeftRightDiff {
/**
* Q61.在数组中,数字减去它右边(注意是右边)的数字得到一个数对之差。
* 求所有数对之差的最大值。例如在数组
- mongoDB 索引
开窍的石头
mongoDB索引
在这一节中我们讲讲在mongo中如何创建索引
得到当前查询的索引信息
db.user.find(_id:12).explain();
cursor: basicCoursor 指的是没有索引
&
- [硬件和系统]迎峰度夏
comsci
系统
从这几天的气温来看,今年夏天的高温天气可能会维持在一个比较长的时间内
所以,从现在开始准备渡过炎热的夏天。。。。
每间房屋要有一个落地电风扇,一个空调(空调的功率和房间的面积有密切的关系)
坐的,躺的地方要有凉垫,床上要有凉席
电脑的机箱
- 基于ThinkPHP开发的公司官网
cuiyadll
行业系统
后端基于ThinkPHP,前端基于jQuery和BootstrapCo.MZ 企业系统
轻量级企业网站管理系统
运行环境:PHP5.3+, MySQL5.0
系统预览
系统下载:http://www.tecmz.com
预览地址:http://co.tecmz.com
各种设备自适应
响应式的网站设计能够对用户产生友好度,并且对于
- Transaction and redelivery in JMS (JMS的事务和失败消息重发机制)
darrenzhu
jms事务承认MQacknowledge
JMS Message Delivery Reliability and Acknowledgement Patterns
http://wso2.com/library/articles/2013/01/jms-message-delivery-reliability-acknowledgement-patterns/
Transaction and redelivery in
- Centos添加硬盘完全教程
dcj3sjt126com
linuxcentoshardware
Linux的硬盘识别:
sda 表示第1块SCSI硬盘
hda 表示第1块IDE硬盘
scd0 表示第1个USB光驱
一般使用“fdisk -l”命
- yii2 restful web服务路由
dcj3sjt126com
PHPyii2
路由
随着资源和控制器类准备,您可以使用URL如 http://localhost/index.php?r=user/create访问资源,类似于你可以用正常的Web应用程序做法。
在实践中,你通常要用美观的URL并采取有优势的HTTP动词。 例如,请求POST /users意味着访问user/create动作。 这可以很容易地通过配置urlManager应用程序组件来完成 如下所示
- MongoDB查询(4)——游标和分页[八]
eksliang
mongodbMongoDB游标MongoDB深分页
转载请出自出处:http://eksliang.iteye.com/blog/2177567 一、游标
数据库使用游标返回find的执行结果。客户端对游标的实现通常能够对最终结果进行有效控制,从shell中定义一个游标非常简单,就是将查询结果分配给一个变量(用var声明的变量就是局部变量),便创建了一个游标,如下所示:
> var
- Activity的四种启动模式和onNewIntent()
gundumw100
android
Android中Activity启动模式详解
在Android中每个界面都是一个Activity,切换界面操作其实是多个不同Activity之间的实例化操作。在Android中Activity的启动模式决定了Activity的启动运行方式。
Android总Activity的启动模式分为四种:
Activity启动模式设置:
<acti
- 攻城狮送女友的CSS3生日蛋糕
ini
htmlWebhtml5csscss3
在线预览:http://keleyi.com/keleyi/phtml/html5/29.htm
代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>攻城狮送女友的CSS3生日蛋糕-柯乐义<
- 读源码学Servlet(1)GenericServlet 源码分析
jzinfo
tomcatWebservlet网络应用网络协议
Servlet API的核心就是javax.servlet.Servlet接口,所有的Servlet 类(抽象的或者自己写的)都必须实现这个接口。在Servlet接口中定义了5个方法,其中有3个方法是由Servlet 容器在Servlet的生命周期的不同阶段来调用的特定方法。
先看javax.servlet.servlet接口源码:
package
- JAVA进阶:VO(DTO)与PO(DAO)之间的转换
snoopy7713
javaVOHibernatepo
PO即 Persistence Object VO即 Value Object
VO和PO的主要区别在于: VO是独立的Java Object。 PO是由Hibernate纳入其实体容器(Entity Map)的对象,它代表了与数据库中某条记录对应的Hibernate实体,PO的变化在事务提交时将反应到实际数据库中。
实际上,这个VO被用作Data Transfer
- mongodb group by date 聚合查询日期 统计每天数据(信息量)
qiaolevip
每天进步一点点学习永无止境mongodb纵观千象
/* 1 */
{
"_id" : ObjectId("557ac1e2153c43c320393d9d"),
"msgType" : "text",
"sendTime" : ISODate("2015-06-12T11:26:26.000Z")
- java之18天 常用的类(一)
Luob.
MathDateSystemRuntimeRundom
System类
import java.util.Properties;
/**
* System:
* out:标准输出,默认是控制台
* in:标准输入,默认是键盘
*
* 描述系统的一些信息
* 获取系统的属性信息:Properties getProperties();
*
*
*
*/
public class Sy
- maven
wuai
maven
1、安装maven:解压缩、添加M2_HOME、添加环境变量path
2、创建maven_home文件夹,创建项目mvn_ch01,在其下面建立src、pom.xml,在src下面简历main、test、main下面建立java文件夹
3、编写类,在java文件夹下面依照类的包逐层创建文件夹,将此类放入最后一级文件夹
4、进入mvn_ch01
4.1、mvn compile ,执行后会在