- 系统编程05-线程(pthread_create、pthread_join、pthread_exit)
JAN JM
系统编程linux服务器ubuntu
目录一、守护进程1.概念(简答题)1)怎样成为守护进程2.守护进程编写步骤1)忽略SIGHUP2)产生子进程3)创建新会话4)产生孙子进程5)进入新进程组6)关闭文件资源7)关闭文件权限掩码8)切换进程工作路径二、linux最小资源单位--线程。1.线程与进程2.线程函数接口特点?1)由于线程函数接口都是封装在一个线程库,所以我们是看不到源码的,查看线程的函数,都是在第3手册:man3xxxx2)
- C语言的网络编程
AI向前看
包罗万象golang开发语言后端
C语言的网络编程引言随着互联网的发展,网络编程逐渐成为计算机科学和软件工程领域中一个重要的研究方向与应用实践。C语言作为一种高效、灵活的编程语言,广泛应用于系统编程和网络编程中。网络编程涉及到的知识面较广,包括网络协议、套接字编程、数据传输等方面。本文将深入探讨C语言的网络编程,帮助读者了解基本概念、相关技术及应用实例。网络编程基础1.网络协议在进行网络编程之前,了解网络协议是非常重要的。网络协议
- C语言的语法糖
AI向前看
包罗万象golang开发语言后端
C语言的语法糖引言在程序开发的过程中,语言的设计和编写风格往往会直接影响开发效率和代码可读性。C语言作为一种广泛应用于系统编程和嵌入式开发的编程语言,其设计虽然追求简洁与高效,但在某些方面同样存在可以提高编程体验的“语法糖”。本文将深入探讨C语言中的语法糖概念及其应用对程序员的帮助。一、什么是语法糖“语法糖”(SyntacticSugar)一词最早由计算机科学家希伯特·马克·古尔德(PeterJ.
- C/C++中的 void*
wudi_demaxiya
C++c++c语言指针
在看《Unix/Linux系统编程》中关于POSIXThread部分的时候发现C语言中用void*传递了int类型变量,很疑惑,于是查了些资料并汇总了一下。介绍了C语言和C++中关于void*的用法,涉及到了C++中的reinterpret_cast如果哪里有错误欢迎指正!参考资料参考资料1.C/C++中的void*与其他指针类型转换1.1C中void*与其他指针类型转换C语言对指针类型的转换要求
- C语言的数据库交互
疯狂小小小码农
包罗万象golang开发语言后端
C语言的数据库交互引言在现代的软件开发中,数据库是应用程序的重要组成部分。无论是小型的个人项目还是大型的企业级应用,数据的存储、查询和管理都需要有效的数据库系统。在众多编程语言中,C语言以其高效、灵活和底层操作的特性,被广泛应用于系统编程、嵌入式开发等领域。当然,C语言在数据库交互方面也是非常强大的。本文将深入探讨C语言与数据库的交互,包括常用的数据库及其驱动,数据库操作的基本流程,常见的数据库操
- Intel系统编程指南第八章——8.8 多核架构
zenny_chen
操作系统及嵌入式开发编程cache多线程存储工具扩展
本小节描述了支持双核和四核技术的Intel64和IA-32处理器的架构。本讨论可应用于Intel奔腾处理器至尊版、奔腾D处理器、Intel酷睿Duo、双核Intel至强处理器、Intel酷睿2Quad处理器,以及四核Intel至强处理器。通常而言,每个处理器核心具有专用的架构资源,与底层的不带硬件多线程性能微架构的一单个处理器实现相同。一个双核处理器中的每个逻辑处理器(不管是否支持Intel超线程
- 【Linux网络编程】第九弹---深入解析TCP服务、IOService与Jsoncpp的应用与实现
小林熬夜学编程
Linux网络编程linux网络运维tcp/ipC语言c++服务器
✨个人主页:熬夜学编程的小林系列专栏:【C语言详解】【数据结构详解】【C++详解】【Linux系统编程】【Linux网络编程】目录1、TcpService.hpp1.1、TcpServer类基本结构1.2、构造析构函数1.3、Loop()1.3.1、内部类1.3.2、Execute()2、Service.hpp2.1、IOService类基本结构2.2、构造析构函数2.3、IOExcute()3、
- C语言中的内存管理:`malloc`、`free`和内存泄漏
cc++c#后端go
C语言是一种非常强大的低级编程语言,提供了直接操作计算机内存的能力,这使得它在系统编程、嵌入式开发、以及高性能计算等领域得到了广泛应用。然而,这种能力同时也带来了复杂的内存管理问题。如何正确、有效地分配和释放内存是每一个C程序员都必须掌握的基本技能。本文将详细探讨C语言中的内存管理,重点关注内存分配函数malloc、内存释放函数free,以及常见的内存管理错误,如内存泄漏。1.动态内存分配:mal
- FPGA器件在线配置方法概述
fpga和matlab
FPGA其他fpga开发FPGA在线配置
目录1.配置电路结构和原理2.ICR控制电路软件3.几种常见的FPGA在线配置方法3.1动态部分重配置(PartialReconfiguration,PR)3.2在系统编程(In-SystemProgramming,ISP)3.3多比特流配置(Multi-BitstreamConfiguration)3.4远程更新与配置3.5使用OpenCL或HLS工具FPGA(Field-Programmabl
- Rust中的所有权和借用规则详解
代码云1
rust开发语言后端
Rust是一种系统编程语言,其设计目标包括内存安全、并发安全以及性能。为了实现这些目标,Rust引入了一系列独特的编程概念,其中最为核心的就是所有权(Ownership)和借用(Borrowing)规则。本文将详细解释Rust中的所有权和借用规则,以及它们如何确保内存安全和并发安全。一、所有权规则在Rust中,每一个值都有一个与之关联的所有者。这个所有者可以是变量、数据结构或者是其他形式的存储。所
- Rust 函数
lly202406
开发语言
Rust函数Rust是一种系统编程语言,以其安全性、速度和并发性而闻名。在Rust中,函数是一等公民,是组织代码和实现功能的基本单位。本文将深入探讨Rust中的函数,包括其定义、特性、参数、返回值以及高级用法。函数定义在Rust中,函数使用fn关键字定义。函数可以有参数和返回值。下面是一个简单的函数定义示例:fngreet(name:&str)->String{format!("Hello,{}!
- Rust:重塑编程世界的利器,对前端开发的深远影响
大鱼前端
前端
引言:在编程语言的海洋中,有一颗璀璨的明星正在冉冉升起——那就是Rust。它不仅在后端领域展现出强大的实力,更在前端领域带来了颠覆性的变革。本文将带你深入了解Rust的魅力,探讨其独特的优势,以及Rust如何影响前端开发,并通过案例分析展示Rust在前端应用的实力。一、Rust:编程语言的新星Rust,这门由Mozilla主导开发的系统编程语言,自诞生之初就以其独特的设计理念和出色的性能赢得了众多
- Rust在Web开发中的并发模型
编程小智星
网络
Rust是一种系统编程语言,以其高效、安全和并发性而著称。随着Web应用规模的不断扩大和复杂性的增加,对并发处理能力的需求也日益突出。Rust作为一种新兴的编程语言,在Web开发中展现出了强大的并发处理能力。本文将深入探讨Rust在Web开发中的并发模型,分析其核心特性以及如何在Web应用中发挥优势。一、Rust并发模型概述Rust的并发模型主要基于其独特的所有权系统和无锁数据结构。所有权系统通过
- Rust编程语言到底是不是炒作的噱头?
极道亦有道
rust开发语言后端
Rust是一种系统编程语言,由于独特的安全、性能和并发性支持,近年来备受关注。作为一名开发人员,你可能想知道Rust是否适合下一个项目。我们在这篇博文中将探讨2024年Rust流行背后的原因以及它如何改善你的开发体验。关于Rust编程语言的几个惊人事实据StackOverflow开发者调查显示,Rust连续第八年被评为最受喜爱的编程语言。据Tiobe公司撰写的IEEESpectrum开发报告显示,
- Linux内核以后会分块逐步用Rust重写吗?
纵然间
linuxrust运维
Linux内核已经积累了大量的代码,包括数百万行的C和C++代码。要想重写这些代码需要巨大的人力和时间投入,且存在很高的风险。这些代码已经过长时间的测试和验证,具有很高的稳定性和可靠性。Rust虽然是一种强调安全性和性能的系统编程语言,但其相对于C和C++来说仍然较新,在Linux内核开发领域的应用还相对较少。用Rust重写Linux内核需要开发者具备深厚的Rust编程技能和经验,以及对Linux
- Linux :epoll ET 模式下文件描述符出现饥饿的情况
技术探索者
linux知识epoll
背景(饥饿问题)使用epollET模式时,epoll_wait返回就绪文件描述符集合,然后我们循环处理,但这时如果有一个文件描述符上有大量输入(不间断的输入流),因为我们ET模式需要一直读到返回EAGIN/EWOULDBLOCK,那我们就会一直在处理这个文件描述符,而导致其他文件描述符得不到处理,这就是采用边缘触发时有可能出现的文件描述符饥饿情况处理方法参考Linux/Unix系统编程手册63.4
- 程式语言区分
白总Server
htmlpythonjavac++开发语言
程序语言有很多种,每种都有其特定的用途和特点。以下是一些广泛使用的编程语言:1.Python:易于学习,广泛用于数据科学、机器学习、网络开发、自动化等领域。2.Java:广泛应用于企业级应用、安卓开发、大型系统开发等。3.C:一种基础语言,广泛用于系统编程、嵌入式开发、操作系统等领域。4.C++:C语言的扩展,支持面向对象编程,用于游戏开发、高性能应用等。5.JavaScript:主要用于网页前端
- Linux系统编程之事件驱动
weixin_34342905
c/c++ui
通常,我们写服务器处理模型的程序时,有以下几种模型:(1)每收到一个请求,创建一个新的进程,来处理该请求;(2)每收到一个请求,创建一个新的线程,来处理该请求;(3)每收到一个请求,放入一个事件列表,让主进程通过非阻塞I/O方式来处理请求分析:第(1)中方法,由于创建新的进程的开销比较大,所以,会导致服务器性能比较差,但实现比较简单。第(2)种方式,由于要涉及到线程的同步,有可能会面临死锁等问题。
- C语言和C++有什么区别(笔记)
Jennifer_28
笔记c++c#
C语言和C++有什么区别C语言和C++是两种常见的编程语言,它们有很多相似之处,但也存在一些不同之处。本文将从语言历史、语法、特性、应用领域等方面探讨一下C语言和C++之间的区别。语言历史C语言是由DennisRitchie在20世纪70年代开发的一种通用编程语言,主要用于系统编程和操作系统开发。C++是由BjarneStroustrup在20世纪80年代初期开发的一种面向对象的编程语言,它在C语
- 【Rust光年纪】从心理学计算到机器学习:Rust语言数据科学库全方位解读!
friklogff
Rust光年纪机器学习rust人工智能
Rust语言的数据科学和机器学习库大揭秘:核心功能、使用指南一网打尽!前言随着数据科学和机器学习在各个领域的广泛应用,使用高效、稳定的编程语言来实现这些功能变得尤为重要。Rust语言作为一种安全且高性能的系统编程语言,正逐渐成为数据科学和机器学习领域的热门选择。本文将介绍几个优秀的Rust库,它们分别用于心理学计算、统计分析、数据科学和机器学习,让我们一同探索它们的核心功能、使用场景和API概览。
- 【图书介绍】《Rust编程与项目实战》
夏天又到了
操作系统与编程语言rust开发语言后端
本书目的系统讲解Rust编程语言,帮助读者掌握Rust网络服务器、游戏开发、数据分析等方面的基础编程技能。学习Rust编程,做一下技术储备。内容简介Rust是一门系统编程语言,专注于安全,尤其是并发安全,它也是支持函数式、命令式以及泛型等编程范式的多范式语言。标准Rust在语法和性能上和标准C++类似,设计者可以在保证性能的同时提供更好的内存安全。本书详解Rust编程技巧,配套示例源码、PPT课件
- [Linux系统编程]进程组和会话,守护进程
SlanderMC
linux运维服务器
一.进程组进程组,也称之为作业。BSD于1980年前后向Unix中增加的一个新特性。代表一个或多个进程的集合。每个进程都属于一个进程组。在waitpid.函数和kill函数的参数中都曾使用到。操作系统设计的进程组的概念,是为了简化对多个进程的管理。当父进程,创建子进程的时候,默认子进程与父进程属于同一进程组。进程组ID=第一个进程ID(组长进程)。可以使用kill-9-进程组ID(负数)来将整个进
- 什么是Rust语言?探索安全系统编程的未来
教IT的小王A
rust安全开发语言
什么是Rust语言?探索安全系统编程的未来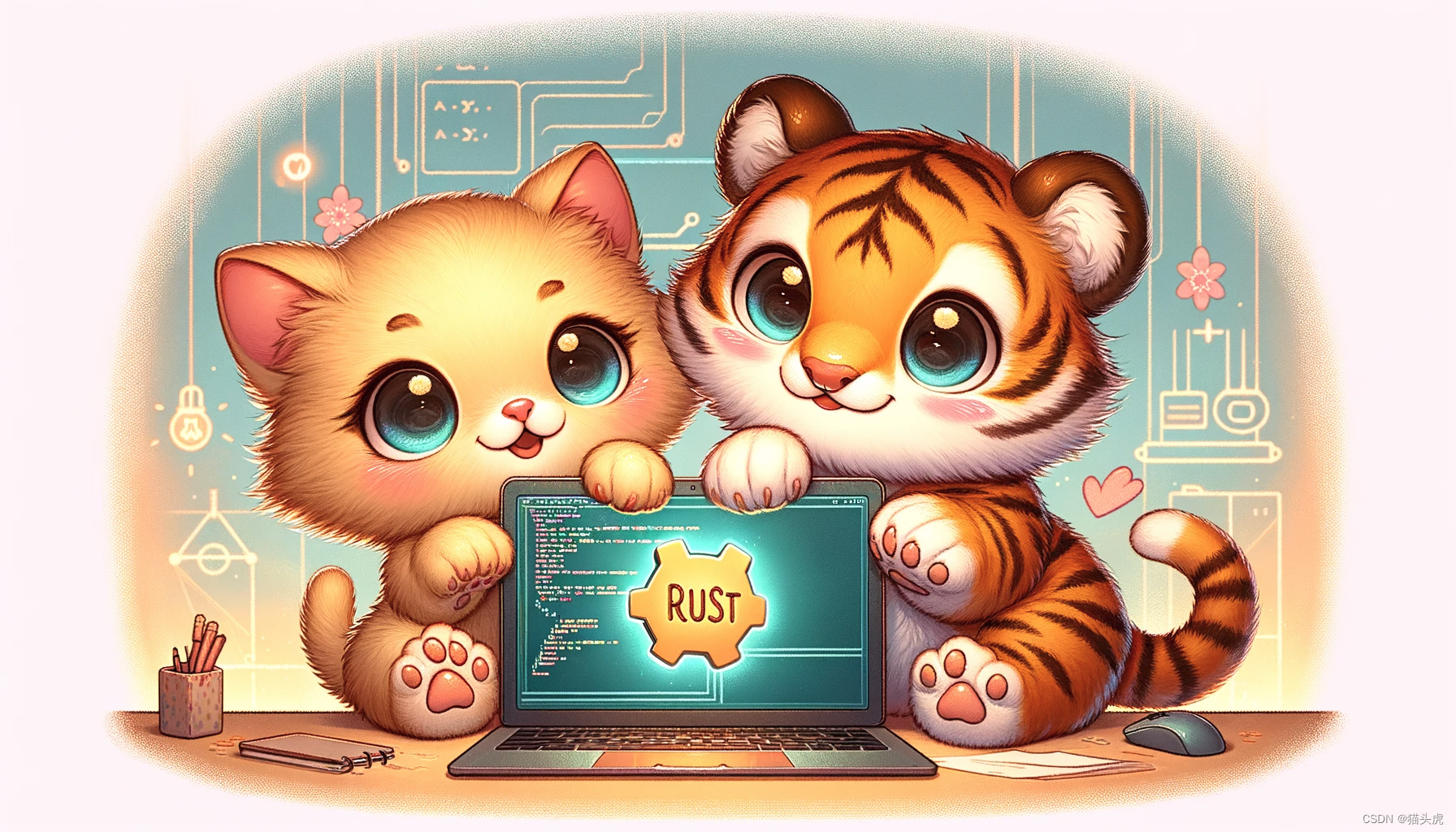文章目录*什么是Rust语言?探索安全系统编程的未来*摘要引言正文*Rust语言简介发展历程Rust的技术意义和优势Rust解决的问题详细代码示例和操作命令QA环节小结参考资料表格总结本文
- Linux系统编程(10)线程资源回收和互斥锁
流殇258
java开发语言
一、pthread_cancel函数pthread_cancel函数用于请求取消一个线程。当调用pthread_cancel时,它会向指定的线程发送一个取消请求。#includeintpthread_cancel(pthread_tthread);thread:要发送取消请求的线程标识符。成功时,返回0。失败时,返回一个错误号二、pthread_detach函数pthread_detach用于将线
- 第十五章 rust中进行windows系统开发
余识-
Rust从入门到精通rustwindows开发语言
注意本系列文章已升级、转移至我的自建站点中,本章原文为:rust中进行Windows系统开发目录注意一、前言二、指针三、windows四、字符串一、前言作为系统级语言,rust可以很容易的和C++一样进行系统级编程,但实际操作后我相信你会遇到相当多的问题。比如以本文要介绍的windows系统编程为例,由于windows系统底层使用的C/C++语言,导致其大量api的参数都是指针,而rust作为一门
- 【Rust光年纪】地理信息处理不再困扰:Rust语言库大揭秘
friklogff
Rust光年纪rust开发语言后端
地理信息处理不再难:Rust语言下的六大利器前言随着地理信息技术的不断发展,对于地理空间数据的处理和分析需求也日益增加。Rust语言作为一种快速、并发、内存安全的系统编程语言,越来越受到开发者的关注和青睐。本文将介绍一些用于Rust语言的地理信息处理库,以及它们的核心功能、使用场景、安装与配置方法以及API概览。欢迎订阅专栏:Rust光年纪文章目录地理信息处理不再难:Rust语言下的六大利器前言1
- 重头开始嵌入式第二十七天(Linux系统编程 信号通信)
FLPGYH
Linux系统高级编程c语言linuxvim
目录进程间通信===》1.信号通信1.信号的五种类型:2.kill1、信号kill-l==>前32个有具体含义的信号3.信号注册函数原型:1.自定义信号处理:2、在所有的信号中有如下两个特列:2.共享内存信号量集1.key创建方式有三种:共享内存===》效率最高的进程间通信方式1、申请对象:2.映射对象:shmat()3.读写共享内存:类似堆区内存的直接读写:4.撤销映射:shmdt5.删除对象:
- 重头开始嵌入式第二十八天(Linux系统编程 网络通信 套接字)
FLPGYH
linuxvimc语言
目录1.网络编程1.OSI(OpenSystemInterconnection)模型即开放式系统互联通信参考模型。TFTP(TrivialFileTransferProtocol)即简单文件传输协议。2.TCP/IP模型也叫网际互联模型共分为4层:也叫协议栈3、TCP/IP协议族:4.DNS(DomainNameSystem,域名系统)是互联网的一项重要服务。4、网络基础(ABCDE类)5.网络相
- 重头开始嵌入式第二十一天(Linux系统编程 文件相关函数)
FLPGYH
vimlinuxc语言
目录1.getpwuid2.getpwnam3.getgrgid4.symlink在Linux和类Unix系统中,创建软链接(符号链接)的常用指令是ln-s。5.remove6.rename7.link8.truncate9.perror10.strerror11.error1.makefile2.gdbstrtok1.getpwuidgetpwuid函数是C语言标准库中的一个函数,用于通过用户I
- 122、Rust微服务:打造高性能分布式系统
多多的编程笔记
Rust之Web开发rust微服务开发语言
Rust分布式系统:了解CAP定理、BASE理论,掌握微服务架构的设计和实现引言分布式系统是计算机科学中一个广泛研究的领域。随着互联网的快速发展,分布式系统已经成为现代软件工程中不可或缺的一部分。Rust是一种系统编程语言,由于其安全性、速度和并发性而逐渐受到关注。本文将介绍Rust在分布式系统中的应用,重点关注CAP定理、BASE理论以及微服务架构的设计和实现。CAP定理CAP定理是分布式系统中
- Spring中@Value注解,需要注意的地方
无量
springbean@Valuexml
Spring 3以后,支持@Value注解的方式获取properties文件中的配置值,简化了读取配置文件的复杂操作
1、在applicationContext.xml文件(或引用文件中)中配置properties文件
<bean id="appProperty"
class="org.springframework.beans.fac
- mongoDB 分片
开窍的石头
mongodb
mongoDB的分片。要mongos查询数据时候 先查询configsvr看数据在那台shard上,configsvr上边放的是metar信息,指的是那条数据在那个片上。由此可以看出mongo在做分片的时候咱们至少要有一个configsvr,和两个以上的shard(片)信息。
第一步启动两台以上的mongo服务
&nb
- OVER(PARTITION BY)函数用法
0624chenhong
oracle
这篇写得很好,引自
http://www.cnblogs.com/lanzi/archive/2010/10/26/1861338.html
OVER(PARTITION BY)函数用法
2010年10月26日
OVER(PARTITION BY)函数介绍
开窗函数 &nb
- Android开发中,ADB server didn't ACK 解决方法
一炮送你回车库
Android开发
首先通知:凡是安装360、豌豆荚、腾讯管家的全部卸载,然后再尝试。
一直没搞明白这个问题咋出现的,但今天看到一个方法,搞定了!原来是豌豆荚占用了 5037 端口导致。
参见原文章:一个豌豆荚引发的血案——关于ADB server didn't ACK的问题
简单来讲,首先将Windows任务进程中的豌豆荚干掉,如果还是不行,再继续按下列步骤排查。
&nb
- canvas中的像素绘制问题
换个号韩国红果果
JavaScriptcanvas
pixl的绘制,1.如果绘制点正处于相邻像素交叉线,绘制x像素的线宽,则从交叉线分别向前向后绘制x/2个像素,如果x/2是整数,则刚好填满x个像素,如果是小数,则先把整数格填满,再去绘制剩下的小数部分,绘制时,是将小数部分的颜色用来除以一个像素的宽度,颜色会变淡。所以要用整数坐标来画的话(即绘制点正处于相邻像素交叉线时),线宽必须是2的整数倍。否则会出现不饱满的像素。
2.如果绘制点为一个像素的
- 编码乱码问题
灵静志远
javajvmjsp编码
1、JVM中单个字符占用的字节长度跟编码方式有关,而默认编码方式又跟平台是一一对应的或说平台决定了默认字符编码方式;2、对于单个字符:ISO-8859-1单字节编码,GBK双字节编码,UTF-8三字节编码;因此中文平台(中文平台默认字符集编码GBK)下一个中文字符占2个字节,而英文平台(英文平台默认字符集编码Cp1252(类似于ISO-8859-1))。
3、getBytes()、getByte
- java 求几个月后的日期
darkranger
calendargetinstance
Date plandate = planDate.toDate();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
Calendar cal = Calendar.getInstance();
cal.setTime(plandate);
// 取得三个月后时间
cal.add(Calendar.M
- 数据库设计的三大范式(通俗易懂)
aijuans
数据库复习
关系数据库中的关系必须满足一定的要求。满足不同程度要求的为不同范式。数据库的设计范式是数据库设计所需要满足的规范。只有理解数据库的设计范式,才能设计出高效率、优雅的数据库,否则可能会设计出错误的数据库.
目前,主要有六种范式:第一范式、第二范式、第三范式、BC范式、第四范式和第五范式。满足最低要求的叫第一范式,简称1NF。在第一范式基础上进一步满足一些要求的为第二范式,简称2NF。其余依此类推。
- 想学工作流怎么入手
atongyeye
jbpm
工作流在工作中变得越来越重要,很多朋友想学工作流却不知如何入手。 很多朋友习惯性的这看一点,那了解一点,既不系统,也容易半途而废。好比学武功,最好的办法是有一本武功秘籍。研究明白,则犹如打通任督二脉。
系统学习工作流,很重要的一本书《JBPM工作流开发指南》。
本人苦苦学习两个月,基本上可以解决大部分流程问题。整理一下学习思路,有兴趣的朋友可以参考下。
1 首先要
- Context和SQLiteOpenHelper创建数据库
百合不是茶
androidContext创建数据库
一直以为安卓数据库的创建就是使用SQLiteOpenHelper创建,但是最近在android的一本书上看到了Context也可以创建数据库,下面我们一起分析这两种方式创建数据库的方式和区别,重点在SQLiteOpenHelper
一:SQLiteOpenHelper创建数据库:
1,SQLi
- 浅谈group by和distinct
bijian1013
oracle数据库group bydistinct
group by和distinct只了去重意义一样,但是group by应用范围更广泛些,如分组汇总或者从聚合函数里筛选数据等。
譬如:统计每id数并且只显示数大于3
select id ,count(id) from ta
- vi opertion
征客丶
macoprationvi
进入 command mode (命令行模式)
按 esc 键
再按 shift + 冒号
注:以下命令中 带 $ 【在命令行模式下进行】,不带 $ 【在非命令行模式下进行】
一、文件操作
1.1、强制退出不保存
$ q!
1.2、保存
$ w
1.3、保存并退出
$ wq
1.4、刷新或重新加载已打开的文件
$ e
二、光标移动
2.1、跳到指定行
数字
- 【Spark十四】深入Spark RDD第三部分RDD基本API
bit1129
spark
对于K/V类型的RDD,如下操作是什么含义?
val rdd = sc.parallelize(List(("A",3),("C",6),("A",1),("B",5))
rdd.reduceByKey(_+_).collect
reduceByKey在这里的操作,是把
- java类加载机制
BlueSkator
java虚拟机
java类加载机制
1.java类加载器的树状结构
引导类加载器
^
|
扩展类加载器
^
|
系统类加载器
java使用代理模式来完成类加载,java的类加载器也有类似于继承的关系,引导类是最顶层的加载器,它是所有类的根加载器,它负责加载java核心库。当一个类加载器接到装载类到虚拟机的请求时,通常会代理给父类加载器,若已经是根加载器了,就自己完成加载。
虚拟机区分一个Cla
- 动态添加文本框
BreakingBad
文本框
<script> var num=1; function AddInput() { var str=""; str+="<input 
- 读《研磨设计模式》-代码笔记-单例模式
bylijinnan
java设计模式
声明: 本文只为方便我个人查阅和理解,详细的分析以及源代码请移步 原作者的博客http://chjavach.iteye.com/
public class Singleton {
}
/*
* 懒汉模式。注意,getInstance如果在多线程环境中调用,需要加上synchronized,否则存在线程不安全问题
*/
class LazySingleton
- iOS应用打包发布常见问题
chenhbc
iosiOS发布iOS上传iOS打包
这个月公司安排我一个人做iOS客户端开发,由于急着用,我先发布一个版本,由于第一次发布iOS应用,期间出了不少问题,记录于此。
1、使用Application Loader 发布时报错:Communication error.please use diagnostic mode to check connectivity.you need to have outbound acc
- 工作流复杂拓扑结构处理新思路
comsci
设计模式工作算法企业应用OO
我们走的设计路线和国外的产品不太一样,不一样在哪里呢? 国外的流程的设计思路是通过事先定义一整套规则(类似XPDL)来约束和控制流程图的复杂度(我对国外的产品了解不够多,仅仅是在有限的了解程度上面提出这样的看法),从而避免在流程引擎中处理这些复杂的图的问题,而我们却没有通过事先定义这样的复杂的规则来约束和降低用户自定义流程图的灵活性,这样一来,在引擎和流程流转控制这一个层面就会遇到很
- oracle 11g新特性Flashback data archive
daizj
oracle
1. 什么是flashback data archive
Flashback data archive是oracle 11g中引入的一个新特性。Flashback archive是一个新的数据库对象,用于存储一个或多表的历史数据。Flashback archive是一个逻辑对象,概念上类似于表空间。实际上flashback archive可以看作是存储一个或多个表的所有事务变化的逻辑空间。
- 多叉树:2-3-4树
dieslrae
树
平衡树多叉树,每个节点最多有4个子节点和3个数据项,2,3,4的含义是指一个节点可能含有的子节点的个数,效率比红黑树稍差.一般不允许出现重复关键字值.2-3-4树有以下特征:
1、有一个数据项的节点总是有2个子节点(称为2-节点)
2、有两个数据项的节点总是有3个子节点(称为3-节
- C语言学习七动态分配 malloc的使用
dcj3sjt126com
clanguagemalloc
/*
2013年3月15日15:16:24
malloc 就memory(内存) allocate(分配)的缩写
本程序没有实际含义,只是理解使用
*/
# include <stdio.h>
# include <malloc.h>
int main(void)
{
int i = 5; //分配了4个字节 静态分配
int * p
- Objective-C编码规范[译]
dcj3sjt126com
代码规范
原文链接 : The official raywenderlich.com Objective-C style guide
原文作者 : raywenderlich.com Team
译文出自 : raywenderlich.com Objective-C编码规范
译者 : Sam Lau
- 0.性能优化-目录
frank1234
性能优化
从今天开始笔者陆续发表一些性能测试相关的文章,主要是对自己前段时间学习的总结,由于水平有限,性能测试领域很深,本人理解的也比较浅,欢迎各位大咖批评指正。
主要内容包括:
一、性能测试指标
吞吐量、TPS、响应时间、负载、可扩展性、PV、思考时间
http://frank1234.iteye.com/blog/2180305
二、性能测试策略
生产环境相同 基准测试 预热等
htt
- Java父类取得子类传递的泛型参数Class类型
happyqing
java泛型父类子类Class
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import org.junit.Test;
abstract class BaseDao<T> {
public void getType() {
//Class<E> clazz =
- 跟我学SpringMVC目录汇总贴、PDF下载、源码下载
jinnianshilongnian
springMVC
----广告--------------------------------------------------------------
网站核心商详页开发
掌握Java技术,掌握并发/异步工具使用,熟悉spring、ibatis框架;
掌握数据库技术,表设计和索引优化,分库分表/读写分离;
了解缓存技术,熟练使用如Redis/Memcached等主流技术;
了解Ngin
- the HTTP rewrite module requires the PCRE library
流浪鱼
rewrite
./configure: error: the HTTP rewrite module requires the PCRE library.
模块依赖性Nginx需要依赖下面3个包
1. gzip 模块需要 zlib 库 ( 下载: http://www.zlib.net/ )
2. rewrite 模块需要 pcre 库 ( 下载: http://www.pcre.org/ )
3. s
- 第12章 Ajax(中)
onestopweb
Ajax
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/
- Optimize query with Query Stripping in Web Intelligence
blueoxygen
BO
http://wiki.sdn.sap.com/wiki/display/BOBJ/Optimize+query+with+Query+Stripping+in+Web+Intelligence
and a very straightfoward video
http://www.sdn.sap.com/irj/scn/events?rid=/library/uuid/40ec3a0c-936
- Java开发者写SQL时常犯的10个错误
tomcat_oracle
javasql
1、不用PreparedStatements 有意思的是,在JDBC出现了许多年后的今天,这个错误依然出现在博客、论坛和邮件列表中,即便要记住和理解它是一件很简单的事。开发者不使用PreparedStatements的原因可能有如下几个: 他们对PreparedStatements不了解 他们认为使用PreparedStatements太慢了 他们认为写Prepar
- 世纪互联与结盟有感
阿尔萨斯
10月10日,世纪互联与(Foxcon)签约成立合资公司,有感。
全球电子制造业巨头(全球500强企业)与世纪互联共同看好IDC、云计算等业务在中国的增长空间,双方迅速果断出手,在资本层面上达成合作,此举体现了全球电子制造业巨头对世纪互联IDC业务的欣赏与信任,另一方面反映出世纪互联目前良好的运营状况与广阔的发展前景。
众所周知,精于电子产品制造(世界第一),对于世纪互联而言,能够与结盟