1. 效果图
-
点击图片可以进入图片预览的界面
2. 项目分析:
2.1 准备工作:
下载图片预览插件:
- https://www.npmjs.com/package/vue-picture-preview
- npm install vue-picture-preview -S
- 具体使用方式参照上方给出的链接
3. 要操作的结构:
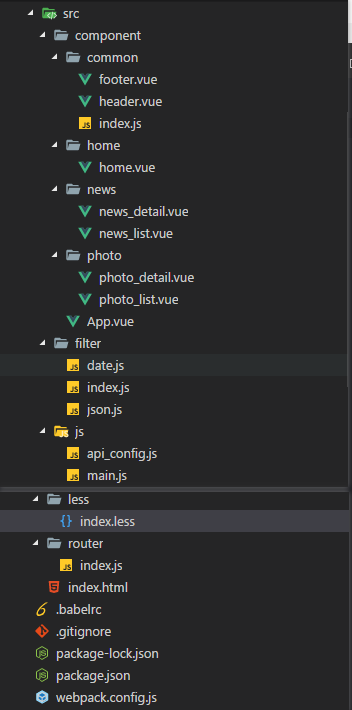
4. 代码:
- src/component/common/footer.vue
- src/component/common/header.vue
- src/component/common/index.js
// 编写属于自己的公共Vue组件库
import HeaderComponent from './header.vue';
import FooterComponent from './footer.vue';
// Vue插件要求提供一个install方法, 这个方法会被注入Vue
// 需要我们调用Vue的filter component directive去扩展功能
export default {
install(Vue) {
Vue.component('app-header', HeaderComponent);
Vue.component('app-footer', FooterComponent);
}
};
- src/component/home/home.vue
-
首页
-
热点新闻
-
图片分享
-
搜索
-
联系我们
-
关于我们
- src/component/news/news_detail.vue
{{ newsDetail.title }}
创建时间: {{ newsDetail.add_time | date }}
点击数: {{ newsDetail.click }}
- src/component/news/news_list.vue
-
{{ item.title }}
创建时间: {{ item.add_time | date }}
点击数: {{ item.click }}
- src/component/photo/photo_detail.vue
{{ photoDetail.title }}
创建时间: {{ photoDetail.add_time | date }}
点击数: {{ photoDetail.click }}
-
- src/component/photo/photo_list.vue
-
全部
-
{{ item.title }}
{{ item.title }}
{{ item.zhaiyao }}
- src/component/App.vue
- src/component/filter/date.js
export default function(time) {
let date = new Date(time);
return `${date.getFullYear()}-${date.getMonth() + 1}-${date.getDate()}`;
};
- src/component/filter/index.js
import DateFilter from './date.js';
export default {
install(Vue) {
Vue.filter('date', DateFilter);
}
};
- src/component/js/api_config.js
const domain = 'http://vue.studyit.io/api';
export default {
// 获取轮播图的接口
getLunbo: `${domain}/getlunbo`,
// 新闻相关接口
getNL: `${domain}/getnewslist`,
getND: `${domain}/getnew/`, // 该接口后面需要一个id
// 图片相关接口
photoC: `${domain}/getimgcategory/`,
photoL: `${domain}/getimages/`, // 该接口后面需要一个分类id: /getimages/:id
photoD: `${domain}/getimageinfo/`, // 该接口后面需要一个图片id: /getimageinfo/:id
photoT: `${domain}/getthumimages/`, // 该接口后面需要一个图片id: /getthumimages/:id
// 商品相关接口
goodsL: `${domain}/getgoods/`, // 该接口后面需要一个页码: /getgoods/?pageindex=number
goodsD: `${domain}/goods/getdesc/`, // 该接口后面需要一个商品id: /getdesc/:id
goodsT: `${domain}/getthumimages/`, // 该接口后面需要一个商品id: /getthumimages/:id
goodsP: `${domain}/getinfo/`, // 该接口后面需要一个商品id: /getinfo/:id
// 购物车相关接口
shopcL: `${domain}/goods/getshopcarlist/`, // 该接口后面需要一串id: /getshopcarlist/:ids
// 评论相关接口
commentL: `${domain}/getcomments/`, // 该接口后面需要一个id: /getcomments/:id
commentS: `${domain}/postcomment/`, // 该接口后面需要一个id: /postcomment/:id, 该需要content内容
};
- src/component/js/main.js
// from后面的路径, 如果含有./ ../那么就相对于当前文件找文件
// 如果没有, 那么就会去node_modules里面找对应的包
// 1.1 导入第三方包
import Vue from 'vue';
import MintUi from 'mint-ui';
import 'mint-ui/lib/style.css';
import Common from '../component/common'; // 自动找到index.js引入
import 'mui/dist/css/mui.css';
import 'mui/examples/hello-mui/css/icons-extra.css';
import axios from 'axios';
import VueRouter from 'vue-router';
import Filter from '../filter' // 自动找到index.js引入
import '../less/index.less';
import VuePP from 'vue-picture-preview';
// 1.2 启用vue插件
Vue.use(MintUi);
Vue.use(Common);
Vue.use(VueRouter);
Vue.use(Filter);
Vue.use(VuePP);
// 2.1 导入配置
import routerConfig from '../router' // 自动找到index.js引入
import apiConfig from './api_config.js'
// 2.2 扩展实例成员
Vue.prototype.axios = axios; // 把axios库放置到原型, 将来其他组件直接可以拿到axios对象
Vue.prototype.api = apiConfig;
// 2.3 导入根组件
import AppComponent from '../component/App.vue';
// 2.4 渲染根组件, 启动项目
new Vue({
el: '#app',
render(createNode) {
return createNode(AppComponent);
},
router: new VueRouter(routerConfig)
});
- src/component/less/index.less
.mui-card-footer img {
width: 100%;
}
- src/component/router/index.js
// 这里对外导出一个路由配置对象
import HomeComponent from '../component/home/home.vue';
import NewsListComponent from '../component/news/news_list.vue';
import NewsDetailComponent from '../component/news/news_detail.vue';
import PhotoListComponent from '../component/photo/photo_list.vue';
import PhotoDetailComponent from '../component/photo/photo_detail.vue';
export default {
routes: [
// 首页路由配置
{ path: "/", redirect: "/index" },
{ name: "i", path: "/index", component: HomeComponent },
// 新闻路由配置
{ name: "nl", path: "/news/list", component: NewsListComponent },
{ name: "nd", path: "/news/detail/:id", component: NewsDetailComponent },
// 图片分享相关路由
{ name: "pl", path: '/photo/list/:id', component: PhotoListComponent },
{ name: "pd", path: '/photo/details/:id', component: PhotoDetailComponent },
]
};
- src/index.html
Document
- .babelrc
{
"presets": [ "env" ],
"plugins":["transform-runtime"]
}
- gitignore
# 忽略第三方包, 他们已经记录在package.json文件中了
/node_modules
# 忽略打包后的文件, 因为我们的项目核心是源代码
/dist
# 忽略隐藏文件
.*
# 不忽略git配置文件和babel配置文件
!.gitignore
!.babelrc
- webpack.config.js
const path = require('path');
const HtmlWP = require('html-webpack-plugin');
const CleanWP = require('clean-webpack-plugin');
module.exports = {
// 打包的入口文件
entry: path.resolve(__dirname, './src/js/main.js'),
// 输出
output: {
path: path.resolve(__dirname, './dist'),
filename: 'bundle.js'
},
// 插件配置
plugins: [
new HtmlWP({
template: './src/index.html',
filename: 'index.html',
inject: 'body'
}),
new CleanWP(['dist'])
],
// 模块配置
module: {
// 配置loader规则
rules: [
// css
{
test: /\.css$/,
use: [ 'style-loader', 'css-loader' ]
},
// less
{
test: /\.less$/,
use: [ 'style-loader', 'css-loader', 'less-loader' ]
},
// html
{
test: /\.(html|tpl)$/,
use: [ 'html-loader' ]
},
// 静态资源引用
{
test: /\.(png|jpeg|gif|jpg|svg|mp3|ttf)$/,
use: [
{ loader: 'url-loader', options: { limit: 10240 } } // 小于10KB的打包
]
},
// js
{
test: /\.js$/,
use: [ 'babel-loader' ],
exclude: path.resolve(__dirname, '../node_modules')
},
// vue
{
test: /\.vue$/,
use: [ 'vue-loader' ]
}
]
}
};
5. 执行webpack-dev-server:
webpack-dev-server