一、生成验证码
(参考博文链接:https://www.cnblogs.com/nanyangke-cjz/p/7049281.html)
package com.hd.orig;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.awt.image.RenderedImage;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
import javax.imageio.ImageIO;
public class CodeUtil {
private static int width = 140;// 定义图片的width
private static int height = 40;// 定义图片的height
private static int codeCount = 4;// 定义图片上显示验证码的个数
private static int xx = 25;
private static int fontHeight = 36;
private static int codeY = 32;
private static char[] codeSequence = { 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R',
'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9' };
public static Map map ;
/**
* 生成一个map集合
* code为生成的验证码
* codePic为生成的验证码BufferedImage对象
* @return
*/
public static Map generateCodeAndPic() {
// 定义图像buffer
BufferedImage buffImg = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
// Graphics2D gd = buffImg.createGraphics();
// Graphics2D gd = (Graphics2D) buffImg.getGraphics();
Graphics gd = buffImg.getGraphics();
// 创建一个随机数生成器类
Random random = new Random();
// 将图像填充为白色
gd.setColor(Color.WHITE);
gd.fillRect(0, 0, width, height);
// 创建字体,字体的大小应该根据图片的高度来定。
Font font = new Font("Fixedsys", Font.BOLD, fontHeight);
// 设置字体。
gd.setFont(font);
// 画边框。
gd.setColor(Color.BLACK);
gd.drawRect(0, 0, width - 1, height - 1);
// 随机产生40条干扰线,使图象中的认证码不易被其它程序探测到。
gd.setColor(Color.BLACK);
for (int i = 0; i < 30; i++) {
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(12);
int yl = random.nextInt(12);
gd.drawLine(x, y, x + xl, y + yl);
}
// randomCode用于保存随机产生的验证码,以便用户登录后进行验证。
StringBuffer randomCode = new StringBuffer();
int red = 0, green = 0, blue = 0;
// 随机产生codeCount数字的验证码。
for (int i = 0; i < codeCount; i++) {
// 得到随机产生的验证码数字。
String code = String.valueOf(codeSequence[random.nextInt(36)]);
// 产生随机的颜色分量来构造颜色值,这样输出的每位数字的颜色值都将不同。
red = random.nextInt(255);
green = random.nextInt(255);
blue = random.nextInt(255);
// 用随机产生的颜色将验证码绘制到图像中。
gd.setColor(new Color(red, green, blue));
gd.drawString(code, (i + 1) * xx, codeY);
// 将产生的四个随机数组合在一起。
randomCode.append(code);
}
Map map =new HashMap();
//存放验证码
map.put("code", randomCode);
//存放生成的验证码BufferedImage对象
map.put("codePic", buffImg);
return map;
}
public static void run() throws Exception {
//创建文件输出流对象
map = CodeUtil.generateCodeAndPic();
OutputStream out = new FileOutputStream("D://img/"+map.get("code")+".jpg");
ImageIO.write((RenderedImage) map.get("codePic"), "jpeg", out);
}
public static void main(String[] args) throws Exception {
run();
new View().run();
}
}
二、编写界面(自己写的,多多指教)
package com.hd.orig;
import java.awt.Font;
import java.awt.Panel;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
import com.hd.identifyCode.FailView;
import com.hd.identifyCode.SuccessView;
public class View {
JFrame frame = new JFrame("验证码");
Panel panel = new Panel();
CodeUtil codeUtil = new CodeUtil();
private Object code;
public void run() {
try {
code=codeUtil.map.get("code");
} catch (Exception e) {
e.printStackTrace();
}
frame.setBounds(400, 200, 565, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setIconImage(new ImageIcon("img/success.jpg").getImage());
frame.setResizable(false);
//请输入验证码
JLabel label = new JLabel("请输入验证码:");
label.setFont(new Font("宋体", Font.BOLD, 26));
label.setBounds(30, 25, 190, 30);
panel.add(label);
//验证码输入框
JTextField codeIn = new JTextField();
codeIn.setBounds(230, 25, 100, 30);
panel.add(codeIn);
//添加图片按钮
JButton imgBtn = new JButton(new ImageIcon("D://img/"+code+".jpg"));
imgBtn.setBounds(360, 20, 140,40);
imgBtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
code=codeUtil.map.get("code");
} catch (Exception e1) {
e1.printStackTrace();
}
imgBtn.setIcon(new ImageIcon("D://img/"+code+".jpg"));
}
});
panel.add(imgBtn);
//添加提交按钮
JButton submit = new JButton("提交");
submit.setFont(new Font("宋体", Font.BOLD, 30));
submit.setBounds(230, 90, 100,40);
submit.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String coIn = codeIn.getText().trim();
if(coIn.equals(code.toString())){
frame.dispose();
new SuccessView();
}else{
new FailView();
}
}
});
panel.add(submit);
panel.setLayout(null);
frame.add(panel);
frame.setVisible(true);
}
}
三、运行展示
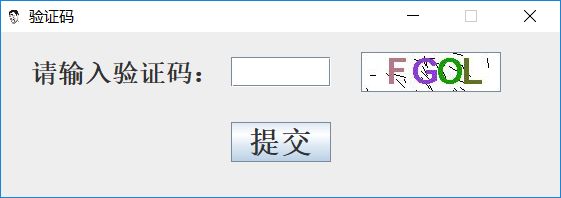