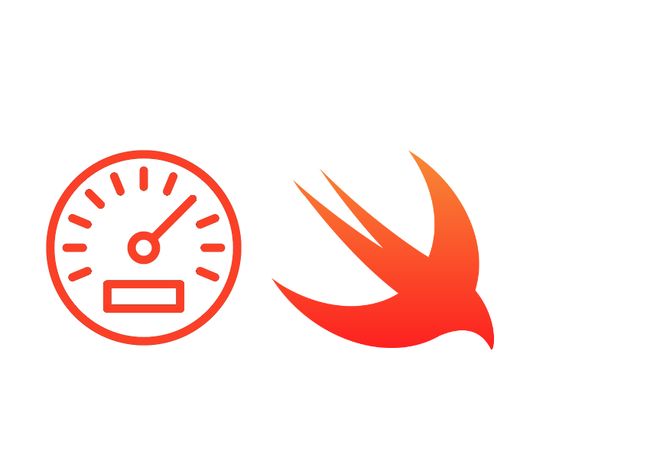
Swift具有优雅,简单和“设计安全”的特点,是历史上发展最快的语言之一。 实际上,Swift的官方口号是“使简单的事情变得容易,而困难的事情变得可能”。 在本文中,您将通过重构代码来学习如何充分利用Swift。
尽管许多代码优化是隐式的,并且显然是语言设计所固有的,但某些重构策略可以使您的代码更易读,更可靠且性能更好。 在本文中,您将学习使用Swift 4重构代码的八种方法。
本文目的
在本文中,您将学习一些使用Swift 4更好地优化和重构代码的方法。我们将介绍以下内容:
- 用
zip
优雅地处理字典中的重复键 - 设置字典的默认值
- 将字典合并为一个
- 将数据字典值直接过滤到另一个字典对象中
- 使用Codable将自定义对象保存到JSON
- 交换可变数组中的值
- 处理多行文字
- 在集合中查找随机元素
1.词典中的重复键
首先,Swift 4使用通用功能zip
以一种优雅的方式来处理重复键,从而进一步增强了字典。 zip
适用于词典,而且实际上它使您可以从符合Sequence的两个基础集合中构建自己的序列类型。
例如,假设您有一个具有以下值的数组,请注意两个包含相同键的元素:
let locations = [ "Springfield", "Chicago", "Springfield", "Jackson"]
var dictionary: Dictionary
通过使用zip
,您可以创建一系列唯一对:
let locations = [ "Springfield", "Chicago", "Springfield", "Jackson"]
var dictionary = Dictionary(zip(locations, repeatElement(1, count: locations.count)), uniquingKeysWith: +)
print(dictionary) // ["Jackson": 1, "Chicago": 1, "Springfield": 2]
上面的代码中的uniquingKeysWith
元素允许您通过使用数学运算符来创建唯一值。 在这种情况下,只要找到重复的条目,我们就使用+
递增值。 当然,您也可以决定采用自己的自定义增量逻辑。
2.字典的默认值
Swift 4的另一个强大功能是能够使用新添加的下标为字典设置默认值。 在以下代码中,当您访问字典中某个键的值时,返回的值是一个optional
值,如果该键不存在,则将返回nil:
let locationsAndPopulations = [ "Springfield": 115715, "Chicago": 2705000, "Aurora": 201110]
print(locationsAndPopulations["Springfield"]) //value is optional
print(locationsAndPopulations["New York"]) //this key doesn't exist, returns nil
通常,您应该处理可选值中nil
的可能性,但是Swift 4通过新的下标使您更加方便,该下标允许您添加默认参数,而不是强制您保护或取消包装可选项。
print(locationsAndPopulations["New York", default: 0])
在这种情况下,由于我们在初始数组中没有纽约的条目,它将返回默认参数0。如果需要,您还可以注入动态值而不是静态文字值,这当然可以使下标功能更加强大。
3.合并字典
Swift 4还通过使用merge(_:uniquingKeysWith:)
使将两个词典合并为一个更加容易。 在以下示例中,我们将第二个字典合并到第一个字典中,并且通过使用新学习的参数uniquingKeysWith
,我们可以确保在出现任何重复项时都将对其进行处理:
var locationsAndPopulationsIL = [ “Springfield”: 115715, “Chicago”: 2705000, “Aurora”: 201110]
let location2 = [ “Rockford”: 152871, “Naperville”: 141853, “Champaign”: 81055]
locationsAndPopulationsIL.merge(location2, uniquingKeysWith: +)
print(locationsAndPopulationsIL)
如果没有此功能,通常必须手动遍历字典的所有值,并在将两个字典合并为一个字典时实现自定义逻辑。
4.将字典值过滤到另一个字典中
除了合并两个字典外,您还可以动态过滤字典,并将结果定向回另一个相同类型的字典中。 在下面的代码片段中,我们通过特定值过滤位置字典,该值作为字典返回:
let locationsAndPopulationsIL = [ "Springfield": 115715, "Chicago": 2705000, "Aurora": 201110]
let filteredmillionPlusPopulation = locationsAndPopulationsIL.filter{ $0.value > 1000000 }
print(filteredmillionPlusPopulation) //["Chicago": 2705000]
因此,除了简单的过滤之外,您还可以利用filter
闭包来提供自定义过滤器,这些过滤器最终将产生新的字典结果。
5.将自定义对象保存到JSON
如果您曾经序列化(和反序列化)数据,那么它可能会涉及到很多问题,必须使用NSObject
子类化类,并实现NSCoding
。 使用Swift 4,您可以通过使用Codable
来更有效地序列化类。 当您想通过将自己的自定义对象序列化为JSON对象以传递给API甚至使用UserDefaults
在本地持久化而持久化时,此功能特别有用:
// a class to serialize
class Location: Codable{
var name: String
var population: Int
init(name: String, population: Int){
self.name = name
self.population = population
}
}
//create an instance
let chicago = Location(name: "chicago", population: 2705000)
//serialize to JSON
let jsonData = try? JSONEncoder().encode(chicago)
//deserialize and output
if let data = jsonData{
let jsonString = String(data: data, encoding: .utf8)
print(jsonString!) //{"name":"chicago","population":2705000}
}
如您所见,通过将类或结构设置为Codable
,您可以轻松地将数据序列化为JSON,将数据保留在某个位置,然后反序列化。
6.交换可变数组中的值
切换到数组,Swift 4的另一个受欢迎的功能是能够通过使用swapAt(_:_:)
在可变数组中直接交换两个值。 这对于诸如排序算法之类的功能最有用,并且使用起来非常简单:
var locations = [ "Springfield", "Chicago", "Champaign", "Aurora"]
locations.swapAt(0, 2)
print(locations) //["Champaign", "Chicago", "Springfield", "Aurora"]
以前,您必须使用临时变量在两个元素位置之间交换,但是使用此方法,您可以更简洁地对数组进行排序。
7.处理多行文字
Swift 4的另一个很酷的功能是能够将多行字符串文字存储在您的值中,这使得拆分文字内容变得更加容易表现出来变得非常容易。 通过使用符号"""
打开和关闭文本块,您可以创建多行内容,甚至可以引用动态变量,如下所示,当我们动态添加日期时。
let illinoisIntro = """
Illinois is a state in the Midwestern United States. It is the 5th most
populous and 25th largest state, and is often noted as a microcosm of
the country. With Chicago and its suburbs in the northeast, small industrial
cities and great agricultural productivity in central and northern Illinois,
and natural resources like coal, timber, and petroleum in the south, Illinois
has a diverse economic base and is a major transportation hub.
(Source: Wikipedia - Dated \(Date())
"""
print(illinoisIntro)
8.选择集合中的随机元素
Swift 4.2中的新功能是可以使用randomElement
函数在集合中选择随机元素。 实际上,不仅数组,而且符合Collection
协议的任何对象都可以使用此漂亮功能。 以下示例使用我们的位置数组,它将从该数组中打印出一个随机城市:
let locations = [ "Springfield", "Chicago", "Springfield", "Jackson"]
let randomElement = locations.randomElement()
print(randomElement!)
结论
在本文中,您学习了Swift 4带来的一些有用的技术,以帮助创建更紧凑,更集中和更优化的代码。 在第四次迭代中,Swift在帮助您重构代码以使其更具可读性和可靠性方面取得了长足的进步。 Swift 4.x尚未完成,我鼓励您关注Swift的官方演变页面以记录即将讨论的所有新建议。
翻译自: https://code.tutsplus.com/tutorials/8-new-ways-to-refactor-your-code-with-swift-4--cms-31742