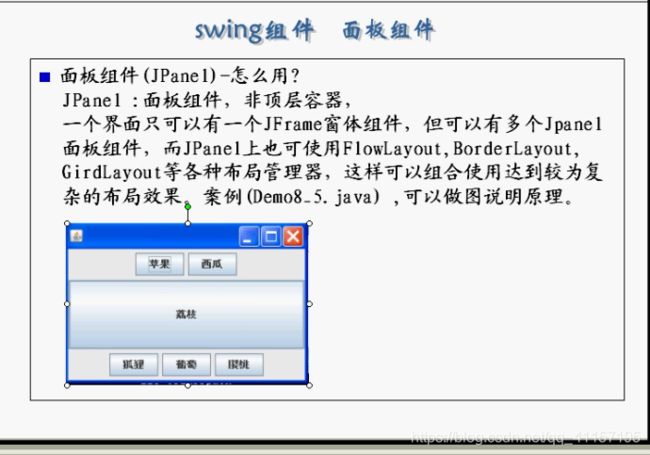
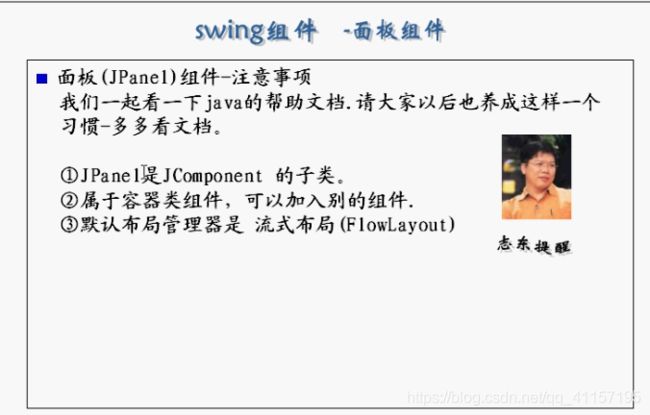
import java.awt.*;
import javax.swing.*;
public class Demo8_5 extends JFrame {
JPanel jp1,jp2;
JButton jb1,jb2,jb3,jb4,jb5,jb6;
public static void main(String[] args) {
Demo8_5 demo8_5 = new Demo8_5();
}
public Demo8_5(){
jp1 = new JPanel();
jp2 = new JPanel();
jb1 = new JButton("西瓜");
jb2 = new JButton("苹果");
jb3 = new JButton("荔枝");
jb4 = new JButton("葡萄");
jb5 = new JButton("桔子");
jb6 = new JButton("香蕉");
jp1.add(jb1);
jp1.add(jb2);
jp2.add(jb3);
jp2.add(jb4);
jp2.add(jb5);
this.add(jp1,BorderLayout.NORTH);
this.add(jp2,BorderLayout.SOUTH);
this.add(jb6,BorderLayout.CENTER);
this.setTitle("JPanel组件");
this.setSize(400,200);
this.setLocation(300,200);
this.setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
}
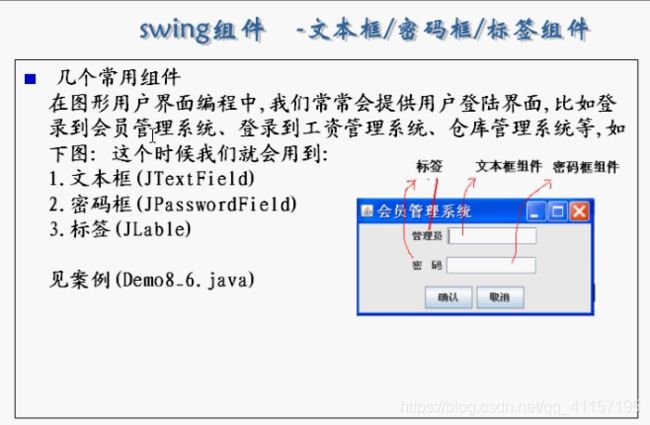
import java.awt.*;
import javax.swing.*;
public class Demo8_6 extends JFrame{
JPanel jp1,jp2,jp3;
JButton jb1,jb2;
JLabel lab1,lab2;
JTextField Jtext;
JPasswordField Jpwd;
public static void main(String[] args) {
Demo8_6 demo8_6 = new Demo8_6();
}
public Demo8_6() {
jp1 = new JPanel();
jp2 = new JPanel();
jp3 = new JPanel();
jb1 = new JButton("确定");
jb2 = new JButton("取消");
lab1 = new JLabel("管理员");
lab2 = new JLabel("密 码");
Jtext = new JTextField(10);
Jpwd = new JPasswordField(10);
this.setLayout(new GridLayout(3,1));
jp1.add(lab1);
jp1.add(Jtext);
jp2.add(lab2);
jp2.add(Jpwd);
jp3.add(jb1);
jp3.add(jb2);
this.add(jp1);
this.add(jp2);
this.add(jp3);
this.setTitle("会员管理系统");
this.setSize(300,150);
this.setLocation(300,200);
this.setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
}
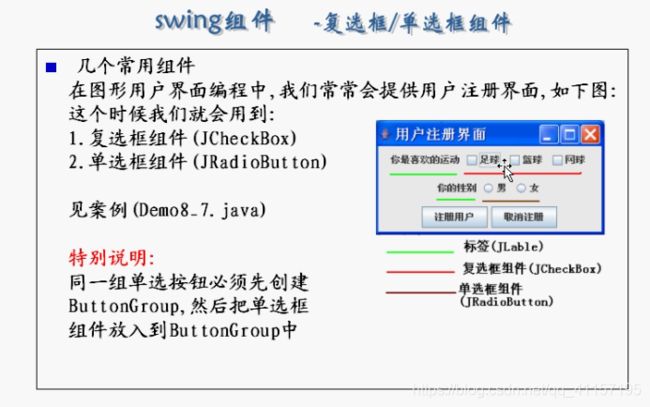
import java.awt.*;
import javax.swing.*;
public class Demo8_7 extends JFrame{
JPanel jp1,jp2,jp3;
JLabel jl1,jl2;
JButton jb1,jb2;
JCheckBox jcb1,jcb2,jcb3;
JRadioButton jrb1,jrb2;
ButtonGroup gb;
public static void main(String[] args) {
Demo8_7 demo8_7 = new Demo8_7();
}
public Demo8_7(){
jp1 = new JPanel();
jp2 = new JPanel();
jp3 = new JPanel();
jl1 = new JLabel("你喜欢的运动");
jl2 = new JLabel("你的性别");
jb1 = new JButton("注册用户");
jb2 = new JButton("取消注册");
jcb1 = new JCheckBox("足球");
jcb2 = new JCheckBox("篮球");
jcb3 = new JCheckBox("网球");
jrb1 = new JRadioButton("男");
jrb2 = new JRadioButton("女");
ButtonGroup bg = new ButtonGroup();
bg.add(jrb1);
bg.add(jrb2);
this.setLayout(new GridLayout(3,1));
jp1.add(jl1);
jp1.add(jcb1);
jp1.add(jcb2);
jp1.add(jcb3);
jp2.add(jl2);
jp2.add(jrb1);
jp2.add(jrb2);
jp3.add(jb1);
jp3.add(jb2);
this.add(jp1);
this.add(jp2);
this.add(jp3);
this.setTitle("用户注册界面");
this.setSize(300,150);
this.setLocation(300,200);
this.setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
}
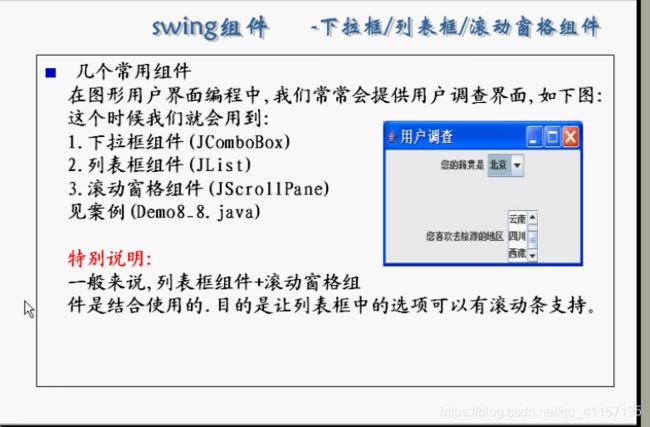
import java.awt.*;
import javax.swing.*;
public class Demo8_8 extends JFrame {
JPanel jp1,jp2,jp3;
JLabel jl1,jl2;
JComboBox jcb1;
JList jlist;
JScrollPane jsp;
public static void main(String[] args) {
Demo8_8 demo8_8 = new Demo8_8();
}
public Demo8_8() {
jp1 = new JPanel();
jp2 = new JPanel();
jp3 = new JPanel();
jl1 = new JLabel("你的籍贯");
jl2 = new JLabel("旅游地点");
String [] jg = {"北京" , "上海 ", "天津 ", "长沙"};
jcb1 = new JComboBox(jg);
String [] dd = {"西安", "武汉 ", "成都 " ,"广东 "};
jlist = new JList(dd);
jsp = new JScrollPane(jlist);
jlist.setVisibleRowCount(2);
this.setLayout(new GridLayout(3,1));
jp1.add(jl1);
jp1.add(jcb1);
jp2.add(jl2);
jp2.add(jsp);
this.add(jp1);
this.add(jp2);
this.setTitle("用户注册界面");
this.setSize(300,300);
this.setLocation(300,200);
this.setResizable(false);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
}
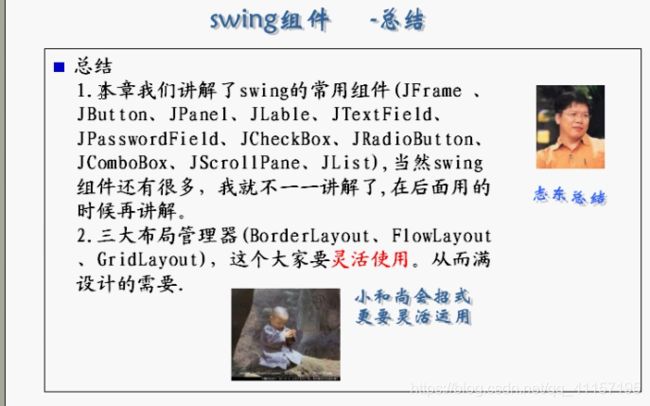