按钮(Button)基本类型与基础使用方法
代码摘自
《EclipseSWT/JFace 核心应用》清华大学出版社。
按钮包含普通按钮(SWT.PUSH)、单选按钮(SWT.RADIO)、多选按钮(SWT.CHECK)、箭头按钮(SWT.ARROW)、切换按钮(SWT.TOGGLE)五种类型;按钮可设置按钮的样式,文字对齐方式的样式有 SWT.LEFT、SWT.RIGHT 和 SWT.CENTER,设置按钮外观风格的样式有 SWT.FLAT 和 SWT.BORDER。
1. 简单应用程序窗口
本次试验包含设定窗口大小、标题栏文字、布局类型,分组标题、布局类型,以及在分组中创建按钮与普通按钮设置。
在这次试验中,建立了一个类,格式是在原 Shell 类上创建新的 Shell 类。
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Shell;
public class ComplexSWT {
public ComplexSWT(Shell sShell){
//Display display = new Display();
//Shell shell = new Shell(display, SWT.CLOSE);
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("Shell");
shell.setLayout(new FillLayout(SWT.VERTICAL)); // 为窗口设置布局(垂直)
Group group = new Group(shell, SWT.SHADOW_ETCHED_OUT); // 在当前窗口中创建分组
group.setText("Group(组)"); // 组名
group.setLayout(new FillLayout(SWT.VERTICAL)); // 为分组设置布局
Button radio1 = new Button(group, SWT.RADIO); // 在当前分组中创建单按钮 1
radio1.setText("First Button"); // 按钮 1 说明
Button radio2 = new Button(group, SWT.RADIO); // 在当前分组中创建单按钮 2
radio2.setText("Second Button"); // 按钮 2 说明
Button button = new Button(shell, SWT.PUSH); // 在当前窗口创建普通按钮
button.setText("Common Button"); // 普通按钮说明
group.layout(); // 分组布局生效
shell.layout(); // 窗口布局生效
shell.open();
/*
while(!shell.isDisposed()){
if(!display.readAndDispatch())
display.sleep();
}
display.dispose();
*/
}
public static void main(String [] args){
//Shell shell = new Shell();
//shell.setSize(200, 200);
//new ComplexSWT();
}
}
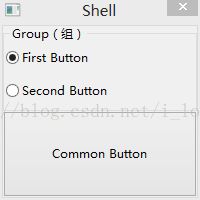
2. 普通按钮(SWT.PUSH)
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class PushButton {
/*
* Shell sShell
*/
public PushButton(Shell sShell) {
//Display display = new Display();
//Shell shell = new Shell(display, SWT.CLOSE);
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.PUSH");
shell.setLayout(new FillLayout(SWT.HORIZONTAL));
// 文字靠左对齐
Button bt1 = new Button(shell, SWT.PUSH|SWT.LEFT);
bt1.setText("SWT.LEFT");
bt1.setToolTipText("SWT.LEFT");
bt1.pack();
// 文字居中对齐
Button bt2 = new Button(shell, SWT.PUSH|SWT.RIGHT);
bt2.setText("SWT.RIGHT");
bt2.setToolTipText("SWT.RIGHT");
bt2.pack();
// 文字靠右对齐
Button bt3 = new Button(shell, SWT.PUSH|SWT.CENTER);
bt3.setText("SWT.CENTER");
bt3.setToolTipText("SWT.CENTER");
// 外观·平坦的(在window8上看不出来?)
Button bt4 = new Button(shell, SWT.PUSH|SWT.FLAT);
bt4.setText("SWT.FLAT");
bt4.setToolTipText("SWT.FLAT");
bt4.pack();
// 外观·下陷的
Button bt5 = new Button(shell, SWT.PUSH|SWT.BORDER);
bt5.setText("SWT.BORDER");
bt5.setToolTipText("SWT.BORDER");
bt5.pack();
shell.layout();
shell.pack();
shell.open();
/*
while(!shell.isDisposed()){
if(!display.readAndDispatch())
display.sleep();
}
display.dispose();*/
}
public static void main(String[] args) {
// TODO Auto-generated method stub
//new PushButton();
}
}

3. 切换按钮(SWT.TOGGLE)
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Shell;
public class ToggleButton {
public ToggleButton(Shell sShell) {
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.TOGGLE");
shell.setLayout(new FillLayout(SWT.HORIZONTAL));
Button bt1 = new Button(shell, SWT.TOGGLE|SWT.LEFT);
bt1.setText("SWT.LEFT");
bt1.setToolTipText("SWT.LEFT");
Button bt2 = new Button(shell, SWT.TOGGLE|SWT.FLAT);
bt2.setText("SWT.FLAT");
bt2.setToolTipText("SWT.FLAT");
Button bt3 = new Button(shell, SWT.TOGGLE|SWT.BORDER);
bt3.setText("SWT.BORDER");
bt3.setToolTipText("SWT.BORDER");
shell.layout();
shell.pack();
shell.open();
}
}

4. 箭头按钮(SWT.ARROW)
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Shell;
public class ArrowButton {
public ArrowButton(Shell sShell) {
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.ARROW");
shell.setLayout(new FillLayout(SWT.HORIZONTAL));
Button bt1 = new Button(shell, SWT.ARROW|SWT.UP);
bt1.setToolTipText("SWT.LEFT");
Button bt2 = new Button(shell, SWT.ARROW|SWT.DOWN);
bt2.setToolTipText("SWT.RIGHT");
Button bt3 = new Button(shell, SWT.ARROW|SWT.LEFT);
bt3.setToolTipText("SWT.CENTER");
Button bt4 = new Button(shell, SWT.ARROW|SWT.RIGHT);
bt4.setToolTipText("SWT.FLAT");
Button bt5 = new Button(shell, SWT.ARROW|SWT.FLAT);
bt5.setToolTipText("SWT.BORDER");
Button bt6 = new Button(shell, SWT.ARROW|SWT.BORDER);
bt6.setToolTipText("SWT.BORDER");
shell.layout();
shell.pack();
shell.open();
}
}
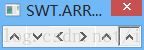
5. 单选按钮(SWT.RADIO)
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Shell;
public class RadioButton {
public RadioButton(Shell sShell) {
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.RADIO");
shell.setLayout(new FillLayout(SWT.VERTICAL));
Group group1 = new Group(shell, SWT.SHADOW_ETCHED_OUT);
group1.setLayout(new FillLayout(SWT.VERTICAL));
group1.setText("One Group");
Button bt1 = new Button(group1, SWT.RADIO|SWT.LEFT);
bt1.setText("SWT.LEFT");
bt1.setToolTipText("SWT.LEFT");
Button bt2 = new Button(group1, SWT.RADIO|SWT.RIGHT);
bt2.setText("SWT.RIGHT");
bt2.setToolTipText("SWT.RIGHT");
Button bt3 = new Button(group1, SWT.RADIO|SWT.CENTER);
bt3.setText("SWT.CENTER");
bt3.setToolTipText("SWT.CENTER");
group1.layout();
group1.pack();
Group group2 = new Group(shell, SWT.SHADOW_ETCHED_OUT);
group2.setLayout(new FillLayout(SWT.VERTICAL));
group2.setText("Another Group");
Button bt4 = new Button(group2, SWT.RADIO|SWT.FLAT);
bt4.setText("SWT.FLAT");
bt4.setToolTipText("SWT.FLAT");
bt4.setSelection(true); // 设置选中状态
// bt4.getSelection(); // 判断一个按钮是否被选中的方法
Button bt5 = new Button(group2, SWT.RADIO|SWT.BORDER);
bt5.setText("SWT.BORDER");
bt5.setToolTipText("SWT.BORDER");
Button bt6 = new Button(group2, SWT.RADIO|SWT.RADIO);
bt6.setText("SWT.RADIO");
bt6.setToolTipText("SWT.RADIO");
group2.layout();
group2.pack();
shell.layout();
shell.pack();
shell.open();
}
}
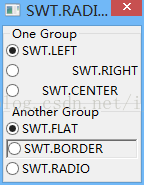
6. 多选按钮(SWT.CHECK)
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Shell;
public class CheckButton {
public CheckButton(Shell sShell){
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.TOGGLE");
shell.setLayout(new FillLayout(SWT.HORIZONTAL));
Group group = new Group(shell, SWT.SHADOW_ETCHED_OUT);
group.setLayout(new FillLayout(SWT.VERTICAL));
group.setText("Check Button");
Button bt1 = new Button(group, SWT.CHECK|SWT.LEFT);
bt1.setText("SWT.LEFT");
bt1.setToolTipText("SWT.LEFT");
Button bt2 = new Button(group, SWT.CHECK|SWT.RIGHT);
bt2.setText("SWT.RIGHT");
bt2.setToolTipText("SWT.RIGHT");
Button bt3 = new Button(group, SWT.CHECK|SWT.CENTER);
bt3.setText("SWT.CENTER");
bt3.setToolTipText("SWT.CENTER");
Button bt4 = new Button(group, SWT.CHECK|SWT.FLAT);
bt4.setText("SWT.FLAT");
bt4.setToolTipText("SWT.FLAT");
Button bt5 = new Button(group, SWT.CHECK|SWT.BORDER);
bt5.setText("SWT.BORDER");
bt5.setToolTipText("SWT.BORDER");
group.layout();
shell.layout();
shell.pack();
shell.open();
}
}
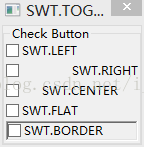
7. 多选按钮案例(CBSample)
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Shell;
public class CheckButtonSample {
public CheckButtonSample(Shell sShell) {
Shell shell = new Shell(sShell, SWT.CLOSE);
shell.setSize(200, 200);
shell.setText("SWT.TOGGLE");
shell.setLayout(new FillLayout(SWT.HORIZONTAL));
// 定义 Shell 的布局
RowLayout layout = new RowLayout(SWT.VERTICAL);
layout.marginWidth = 10;
shell.setLayout(layout);
Group group = new Group(shell, SWT.SHADOW_ETCHED_OUT);
group.setLayout(new FillLayout(SWT.VERTICAL));
group.setText("Preference");
// 定义按钮为数组,要在时间处理类中使用按钮的应用;要设置成 final 形式,事件中要求
final Button button[] = new Button[5];
String items[] = {"Reading", "Swimming", "Music", "Sleeping", "Shopping"};
// 初始化按钮数组
for(int i = 0; i < button.length; i++)
{
button[i] = new Button(group, SWT.CHECK|SWT.CENTER);
button[i].setText(items[i]);
}
// "确定"按钮
Button ok = new Button(group, SWT.PUSH|SWT.CENTER);
ok.setText("Yes");
// 添加"确定"按钮事件
ok.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e){
// 循环所有按钮
for(int i = 0; i < button.length; i++)
{
// 如果被选中,测输出
if(button[i].getSelection())
System.out.println("You choose " + button[i].getText());
// getText() 获取按你文本; getSelection() 判断是否被选中(boolean)
}
}
});
// 选中 CheckButton,发生事件
for(int i = 0; i < button.length; i++)
{
final int j = i;
button[i].addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e){
if(button[j].getSelection())
System.out.println(button[j].getText());
}
});
}
shell.layout();
shell.pack();
shell.open();
}
}
8. 常用方法
Button 常用方法归纳
方法 |
含义 |
setText(String string); getText(); |
设置和获取按钮文本 |
setSelection(boolean selected); getselection(); |
设置和获取按钮是否被选中 |
setImage(Image image); getImage(); |
设置和获取按钮图标,例:button.setImage(display.getSystemImage(SWT.ICON_ERROR))/(new Image(display, "F:\\icon\\edit.gif")); |
setAlignment(int alignment); getAlignment(); |
设置和获取按钮文字对齐方式:SWT.RIGHT, SWT.LEFT, SWT.CENTER |