文章目录
-
- [参考文章1:Python 绘制 柱状图](https://www.cnblogs.com/shenxiaolin/p/11100094.html)
- 我的代码
参考文章1:Python 绘制 柱状图
plt.figure(figsize=(10, 10), dpi=80)
N = 10
values = (56796,42996,24872,13849,8609,5331,1971,554,169,26)
index = np.arange(N)
width = 0.45
p2 = plt.bar(index, values, width, label="num", color="#87CEFA")
plt.xlabel('clusters')
plt.ylabel('number of reviews')
plt.title('Cluster Distribution')
plt.xticks(index, ('mentioned1cluster', 'mentioned2cluster', 'mentioned3cluster', 'mentioned4cluster', 'mentioned5cluster', 'mentioned6cluster', 'mentioned7cluster', 'mentioned8cluster', 'mentioned9cluster', 'mentioned10cluster'))
plt.legend(loc="upper right")
plt.show()
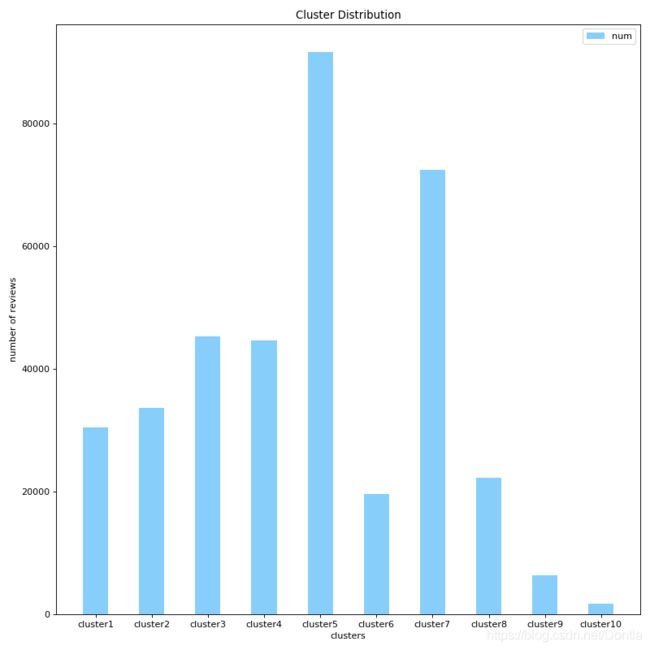
我的代码
"""
@File : 测试拟合平面.py
@Time : 2020/9/8 9:23
@Author : Dontla
@Email : [email protected]
@Software: PyCharm
"""
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from numba import jit
def load_data(file_path):
npzfile = np.load(file_path)
return npzfile['x'], npzfile['y'], npzfile['z']
def cal_flat(x_, y_, z_):
point_num = len(x_)
print('len={}'.format(point_num))
a = 0
A = np.ones((point_num, 3))
for i in range(0, point_num):
A[i, 0] = x_[a]
A[i, 1] = y_[a]
a = a + 1
b = np.zeros((point_num, 1))
a = 0
for i in range(0, point_num):
b[i, 0] = z_[a]
a = a + 1
A_T = A.T
A1 = np.dot(A_T, A)
A2 = np.linalg.inv(A1)
A3 = np.dot(A2, A_T)
X = np.dot(A3, b)
print('平面拟合结果为:z = %.6f * x + %.6f * y + %.6f' % (X[0, 0], X[1, 0], X[2, 0]))
R = 0
for i in range(0, point_num):
R = R + (X[0, 0] * x_[i] + X[1, 0] * y_[i] + X[2, 0] - z_[i]) ** 2
print('方差为:%.*f' % (3, R))
return X
def show_flat(X):
fig1 = plt.figure()
ax1 = fig1.add_subplot(111, projection='3d')
ax1.set_xlabel("x")
ax1.set_ylabel("y")
ax1.set_zlabel("z")
ax1.scatter(x, y, z, c='r', marker='.')
x_p = np.linspace(-200, 1480, 168)
y_p = np.linspace(-200, 920, 112)
x_p, y_p = np.meshgrid(x_p, y_p)
z_p = X[0, 0] * x_p + X[1, 0] * y_p + X[2, 0]
ax1.plot_wireframe(x_p, y_p, z_p, rstride=10, cstride=10)
plt.show()
def cal_error_distribution(x_, y_, z_, coefficient_):
error = z_ - (coefficient_[0, 0] * x_ + coefficient_[1, 0] * y_ + coefficient_[2, 0])
return error
def count_error_number(depth_error_, column_num):
error_max = np.max(depth_error)
error_min = np.min(depth_error)
error_width = (error_max - error_min) / column_num
column_height_sequence = np.zeros(column_num)
for error in depth_error_:
index = int((error - error_min) // error_width)
if index == column_num:
index -= 1
column_height_sequence[index] += 1
return column_height_sequence
def draw_histogram(column_height_sequence_):
plt.figure(figsize=(10, 10), dpi=80)
N = len(column_height_sequence)
values = column_height_sequence_
index = np.arange(N)
width = 1
p2 = plt.bar(index, values, width, label="num", color="#87CEFA")
plt.xlabel('error/mm')
plt.ylabel('number of points')
plt.title('D435 error Distribution(500mm)')
index_new = []
for index_ in index:
if index_ % 100 == 0:
index_new.append(index_)
index_new_content = []
for index in index_new:
error_max = np.max(depth_error)
error_min = np.min(depth_error)
index_new_content.append(error_min + (error_max - error_min) * index / N)
index_new_content_string = [str(i) for i in np.around(index_new_content, decimals=1)]
plt.xticks(index_new, index_new_content_string)
plt.legend(loc="upper right")
plt.show()
if __name__ == '__main__':
x, y, z = load_data('./data/500mm/4_1599623292.4111953.npz')
coefficient = cal_flat(x, y, z)
depth_error = cal_error_distribution(x, y, z, coefficient)
column_height_sequence = count_error_number(depth_error, 1000)
draw_histogram(column_height_sequence)
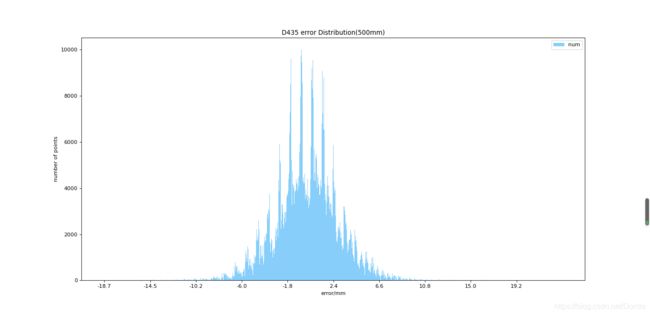
代码中深度数据:
https://download.csdn.net/download/Dontla/12833241