package com.example.mytabmp3;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.annotation.SuppressLint;
import android.content.ContentResolver;
import android.database.Cursor;
import android.media.MediaParser;
import android.media.MediaPlayer;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.os.Message;
import android.provider.MediaStore;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.ProgressBar;
import android.widget.TabHost;
import android.widget.TextView;
import android.widget.Toast;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.MalformedURLException;
import java.net.Socket;
import java.net.URL;
import java.net.URLConnection;
public class MainActivity extends AppCompatActivity implements OnClickListener {
ImageView nextIv,playIv,lastIv;
TextView singerTv,songTv;
RecyclerView musicRv;
List<LocalMusicBean> mDatas;
private LocalMusicAdater adapter;
private int position;
int currnetPalyPosition=-1;
int currentPausePositionInSong=0;
MediaPlayer mediaPlayer;
TextView tv;
ListView ls;
Button btn;
EditText edt;
String NeedFindWords;
String[] strArr;
private static final String Path="http://192.168.1.7/song/2.DJ - 侧脸 (言寺).mp3";
private ProgressBar progressBar;
private TextView textView;
private Button button;
private int FileLength;
private int DownedFileLength=0;
private InputStream inputStream;
private URLConnection connection;
private OutputStream outputStream;
private final int HANDLER_MSG_TELL_RECV = 0x124;
@SuppressLint("HandlerLeak")
Handler handler = new Handler(){
public void handleMessage(Message msg){
System.out.println((msg.obj).toString());
strArr = (msg.obj).toString().split("\n");
ArrayAdapter<String> arrayAdapter= new ArrayAdapter<String> (
MainActivity.this, android.R.layout.simple_list_item_1,strArr);
ls.setAdapter(arrayAdapter);
}
};
private void startNetThread() {
new Thread() {
@Override
public void run() {
try {
Socket socket = new Socket("192.168.1.7", 666);
InputStream is = socket.getInputStream();
OutputStream out = socket.getOutputStream();
out.write(NeedFindWords.getBytes());
out.flush();
byte[] bytes = new byte[1024];
int n = is.read(bytes);
Message msg = handler.obtainMessage(HANDLER_MSG_TELL_RECV, new String(bytes, 0, n));
msg.sendToTarget();
is.close();
socket.close();
} catch (Exception e) {
}
}
}.start();
}
private Handler handler2=new Handler()
{
public void handleMessage(Message msg)
{
if (!Thread.currentThread().isInterrupted()) {
switch (msg.what) {
case 0:
progressBar.setMax(FileLength);
Log.i("文件长度----------->", progressBar.getMax()+"");
break;
case 1:
progressBar.setProgress(DownedFileLength);
int x=DownedFileLength*100/FileLength;
textView.setText(x+"%");
break;
case 2:
String[] strs=Path.split("/");
int lastSTR=0;
for(int i=0,len=strs.length;i<len;i++){
lastSTR=len;
System.out.println(strs[i].toString());
}
Toast.makeText(getApplicationContext(), "下载完成", Toast.LENGTH_LONG).show();
Toast.makeText(getApplicationContext(), strs[lastSTR-1].toString(), Toast.LENGTH_LONG).show();
break;
default:
break;
}
}
}
};
private void DownFile(String urlString)
{
String LastName="";
try {
URL url=new URL(urlString);
String[] MFileName=urlString.split("/");
LastName=MFileName[MFileName.length-1];
System.out.println("***********************"+LastName);
connection=url.openConnection();
if (connection.getReadTimeout()==5) {
Log.i("---------->", "当前网络有问题");
}
inputStream=connection.getInputStream();
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
String savePAth= Environment.getExternalStorageDirectory()+"/kgmusic/download";
File file1=new File(savePAth);
if (!file1.exists()) {
file1.mkdir();
}
String savePathString=Environment.getExternalStorageDirectory()+"/kgmusic/download/"+LastName;
File file =new File(savePathString);
if (!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
Message message=new Message();
try {
outputStream=new FileOutputStream(file);
byte [] buffer=new byte[1024*4];
FileLength=connection.getContentLength();
message.what=0;
handler2.sendMessage(message);
while (DownedFileLength<FileLength) {
outputStream.write(buffer);
DownedFileLength+=inputStream.read(buffer);
Log.i("-------->", DownedFileLength+"");
Message message1=new Message();
message1.what=1;
handler2.sendMessage(message1);
}
Message message2=new Message();
message2.what=2;
handler2.sendMessage(message2);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TabHost tab = (TabHost) findViewById(android.R.id.tabhost);
tab.setup();
tab.addTab(tab.newTabSpec("tab1").setIndicator("本地音乐" , null).setContent(R.id.tab1));
tab.addTab(tab.newTabSpec("tab2").setIndicator("网络音乐" , null).setContent(R.id.tab2));
initView();
Button btn = findViewById(R.id.button);
edt=(EditText)findViewById(R.id.editText);
ls= (ListView) findViewById(R.id.ListName);
progressBar=(ProgressBar) findViewById(R.id.progress);
textView=(TextView) findViewById(R.id.text);
button=(Button) findViewById(R.id.button);
ls.setOnItemClickListener(new AdapterView.OnItemClickListener() {
private String MusicPath="";
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
System.out.println(id);
System.out.println("********************************************");
Toast.makeText(MainActivity.this,strArr[position],Toast.LENGTH_SHORT).show();
MusicPath=strArr[position];
DownedFileLength=0;
Thread thread=new Thread(){
public void run(){
try {
DownFile(MusicPath);
} catch (Exception e) {
}
}
};
thread.start();
}
});
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
NeedFindWords=edt.getText().toString();
startNetThread();
}
});
mediaPlayer=new MediaPlayer();
mDatas=new ArrayList<>();
adapter=new LocalMusicAdater(this,mDatas);
musicRv.setAdapter(adapter);
LinearLayoutManager layoutManager=new LinearLayoutManager(this,LinearLayoutManager.VERTICAL,false);
musicRv.setLayoutManager(layoutManager);
loadLocalMusicData();
setEventListener();
}
private void setEventListener() {
adapter.setOnItemClickListener(new LocalMusicAdater.OnItemClickListener() {
@Override
public void OnItemClick(View view, int position) {
currnetPalyPosition=position;
LocalMusicBean musicBean=mDatas.get(position);
playMusicInMusicBean(musicBean);
}
});
}
public void playMusicInMusicBean(LocalMusicBean musicBean) {
singerTv.setText(musicBean.getSinger());
songTv.setText(musicBean.getSong());
stopMusic();
mediaPlayer.reset();
try {
mediaPlayer.setDataSource(musicBean.getPath());
palyMusic();
} catch (IOException e) {
e.printStackTrace();
}
}
private void palyMusic() {
if(mediaPlayer!=null&&!mediaPlayer.isPlaying()){
if(currentPausePositionInSong==0){
try {
mediaPlayer.prepare();
mediaPlayer.start();
} catch (IOException e) {
e.printStackTrace();
}
}else {
mediaPlayer.seekTo(currentPausePositionInSong);
mediaPlayer.start();
}
playIv.setImageResource(R.mipmap.icon_pause);
}
}
private void pauseMusic() {
if(mediaPlayer!=null&&mediaPlayer.isPlaying()){
currentPausePositionInSong=mediaPlayer.getCurrentPosition();
mediaPlayer.pause();
playIv.setImageResource(R.mipmap.icon_play);
}
}
private void stopMusic() {
if(mediaPlayer!=null){
currentPausePositionInSong=0;
mediaPlayer.pause();
mediaPlayer.seekTo(0);
mediaPlayer.stop();
playIv.setImageResource(R.mipmap.icon_play);
}
}
@Override
protected void onDestroy() {
super.onDestroy();
stopMusic();
}
private void loadLocalMusicData() {
ContentResolver resolver=getContentResolver();
Uri uri= MediaStore.Audio.Media.EXTERNAL_CONTENT_URI;
Cursor cursor=resolver.query(uri,null,null,null,null);
int id=0;
while(cursor.moveToNext()){
String song = cursor.getString(cursor.getColumnIndex(MediaStore.Audio.Media.TITLE));
String singer=cursor.getString(cursor.getColumnIndex(MediaStore.Audio.Media.ARTIST));
String album=cursor.getString(cursor.getColumnIndex(MediaStore.Audio.Media.ALBUM));
id++;
String sid=String.valueOf(id);
String path=cursor.getString(cursor.getColumnIndex(MediaStore.Audio.Media.DATA));
long duration=cursor.getLong(cursor.getColumnIndex(MediaStore.Audio.Media.DURATION));
SimpleDateFormat sdf=new SimpleDateFormat("mm:ss");
String time = sdf.format(new Date(duration));
LocalMusicBean bean=new LocalMusicBean(sid,song,singer,album,time,path);
mDatas.add(bean);
}
adapter.notifyDataSetChanged();
}
private void initView() {
nextIv=findViewById(R.id.local_music_bottom_iv_next);
playIv=findViewById(R.id.local_music_bottom_iv_play);
lastIv=findViewById(R.id.local_music_bottom_iv_last);
singerTv=findViewById(R.id.local_music_bottom_tv_singer);
songTv=findViewById(R.id.local_music_bottom_tv_song);
musicRv=findViewById(R.id.local_music_rv);
nextIv.setOnClickListener(this);
lastIv.setOnClickListener(this);
playIv.setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()){
case R.id.local_music_bottom_iv_last:
if(currnetPalyPosition==0){
Toast.makeText(this,"已经是第一首歌曲。",Toast.LENGTH_SHORT).show();
}
currnetPalyPosition=currnetPalyPosition-1;
LocalMusicBean lastBean=mDatas.get(currnetPalyPosition);
playMusicInMusicBean(lastBean);
break;
case R.id.local_music_bottom_iv_next:
if(currnetPalyPosition==mDatas.size()-1){
Toast.makeText(this,"next Music...",Toast.LENGTH_SHORT).show();
}
currnetPalyPosition=currnetPalyPosition+1;
LocalMusicBean nextBean=mDatas.get(currnetPalyPosition);
playMusicInMusicBean(nextBean);
break;
case R.id.local_music_bottom_iv_play:
if(currnetPalyPosition==-1){
Toast.makeText(this, "last Music...", Toast.LENGTH_SHORT).show();
return;
}
if(mediaPlayer.isPlaying()){
pauseMusic();
}else {
palyMusic();
}
break;
}
}
}
package com.example.mytabmp3;
public class LocalMusicBean {
private String id;
private String song;
private String singer;
private String album;
private String duration;
private String path;
public LocalMusicBean() {
}
public LocalMusicBean(String id, String song, String singer, String album, String duration, String path) {
this.id = id;
this.song = song;
this.singer = singer;
this.album = album;
this.duration = duration;
this.path = path;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getSong() {
return song;
}
public void setSong(String song) {
this.song = song;
}
public String getSinger() {
return singer;
}
public void setSinger(String singer) {
this.singer = singer;
}
public String getAlbum() {
return album;
}
public void setAlbum(String album) {
this.album = album;
}
public String getDuration() {
return duration;
}
public void setDuration(String duration) {
this.duration = duration;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
}
package com.example.mytabmp3;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.util.List;
class LocalMusicAdater extends RecyclerView.Adapter<LocalMusicAdater.LocalMusicViewHolder>{
Context context;
List<LocalMusicBean> mDatas;
OnItemClickListener onItemClickListener;
public void setOnItemClickListener(OnItemClickListener onItemClickListener){
this.onItemClickListener=onItemClickListener;
}
public interface OnItemClickListener{
public void OnItemClick(View view, int position);
}
public LocalMusicAdater(Context context, List<LocalMusicBean> mDatas) {
this.context = context;
this.mDatas = mDatas;
}
@NonNull
@Override
public LocalMusicViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view= LayoutInflater.from(context).inflate(R.layout.item_local_music,parent,false);
LocalMusicViewHolder holder=new LocalMusicViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(@NonNull LocalMusicViewHolder holder, final int position) {
LocalMusicBean musicBean=mDatas.get(position);
holder.idTv.setText(musicBean.getId());
holder.songTv.setText(musicBean.getSong());
holder.singerTv.setText(musicBean.getSinger());
holder.albumTv.setText(musicBean.getAlbum());
holder.timeTv.setText(musicBean.getDuration());
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
onItemClickListener.OnItemClick(view,position);
}
});
}
@Override
public int getItemCount() {
return mDatas.size();
}
class LocalMusicViewHolder extends RecyclerView.ViewHolder{
TextView idTv,songTv,singerTv,albumTv,timeTv;
public LocalMusicViewHolder(@NonNull View itemView) {
super(itemView);
idTv=itemView.findViewById(R.id.item_local_music_num);
songTv=itemView.findViewById(R.id.item_local_music_song);
singerTv=itemView.findViewById(R.id.item_local_music_singer);
albumTv=itemView.findViewById(R.id.item_local_music_album);
timeTv=itemView.findViewById(R.id.item_local_music_durtion);
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.mytabmp3">
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:usesCleartextTraffic="true"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TabHost
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/tabhost"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- TabWidget组件id值不可变-->
<TabWidget
android:id="@android:id/tabs"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</TabWidget>
<!-- FrameLayout布局,id值不可变-->
<FrameLayout
android:id="@android:id/tabcontent"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_above="@android:id/tabs">
<!-- 第一个tab的布局 -->
<RelativeLayout
android:id="@+id/tab1"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="70dp"
android:layout_alignParentBottom="true"
android:id="@+id/local_music_bottomlayout">
<ImageView
android:layout_width="match_parent"
android:layout_height="0.5dp"
android:background="#9933FA"/>
<ImageView
android:layout_width="60dp"
android:layout_height="60dp"
android:src="@mipmap/icon_song"
android:layout_centerVertical="true"
android:layout_marginLeft="10dp"
android:id="@+id/local_music_bottom_iv_icon"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/local_music_bottom_tv_song"
android:text=""
android:layout_toRightOf="@+id/local_music_bottom_iv_icon"
android:layout_marginTop="10dp"
android:layout_marginLeft="10dp"
android:textSize="16sp"
android:textStyle="bold"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/local_music_bottom_tv_singer"
android:text=""
android:textSize="12sp"
android:layout_below="@+id/local_music_bottom_tv_song"
android:layout_alignLeft="@+id/local_music_bottom_tv_song"
android:layout_marginTop="10dp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/local_music_bottom_iv_next"
android:layout_centerVertical="true"
android:src="@mipmap/icon_next"
android:layout_alignParentRight="true"
android:layout_marginRight="10dp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/local_music_bottom_iv_play"
android:layout_centerVertical="true"
android:src="@mipmap/icon_play"
android:layout_toLeftOf="@+id/local_music_bottom_iv_next"
android:layout_marginRight="20dp"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/local_music_bottom_iv_last"
android:layout_centerVertical="true"
android:src="@mipmap/icon_last"
android:layout_toLeftOf="@+id/local_music_bottom_iv_play"
android:layout_marginRight="20dp"/>
</RelativeLayout>
<androidx.recyclerview.widget.RecyclerView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/local_music_rv"
android:layout_above="@+id/local_music_bottomlayout">
</androidx.recyclerview.widget.RecyclerView>
</RelativeLayout>
<!-- 第二个tab的布局 -->
<RelativeLayout
android:id="@+id/tab2"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<EditText
android:id="@+id/editText"
android:layout_width="301dp"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPersonName"
android:text="请输入歌曲名称"
tools:layout_editor_absoluteX="10dp"
tools:layout_editor_absoluteY="11dp" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:text="搜索歌曲"
tools:layout_editor_absoluteX="264dp"
tools:layout_editor_absoluteY="8dp" />
<ProgressBar
android:id="@+id/progress"
android:layout_below="@+id/button"
style="?android:attr/progressBarStyleHorizontal"
android:layout_width="match_parent"
android:layout_height="25dp"
/>
<TextView
android:id="@+id/text"
android:layout_below="@+id/progress"
android:layout_width="match_parent"
android:layout_height="20dp"
android:text="TextView" />
<ListView
android:id="@+id/ListName"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/text"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_marginTop="7dp"
android:layout_marginEnd="3dp"
android:layout_marginRight="3dp"
android:text="Hello World!"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="98dp" >
</ListView>
</RelativeLayout>
</FrameLayout>
</LinearLayout>
</TabHost>
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_marginRight="10dp"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"
app:contentPadding="10dp"
app:cardCornerRadius="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/item_local_music_num"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:layout_centerVertical="true"
android:textSize="24sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/item_local_music_song"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:layout_toRightOf="@id/item_local_music_num"
android:text=""
android:textSize="18sp"
android:textStyle="bold"/>
<TextView
android:id="@+id/item_local_music_singer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text=""
android:layout_below="@+id/item_local_music_song"
android:layout_alignLeft="@+id/item_local_music_song"
android:layout_marginTop="10dp"
android:textSize="14sp"
android:textStyle="bold"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/item_local_music_album"
android:text=""
android:layout_toRightOf="@+id/item_local_music_singer"
android:layout_alignTop="@+id/item_local_music_singer"
android:textSize="14sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/item_local_music_durtion"
android:layout_below="@+id/item_local_music_singer"
android:layout_alignParentRight="true"
android:text="3:52"/>
</RelativeLayout>
</androidx.cardview.widget.CardView>
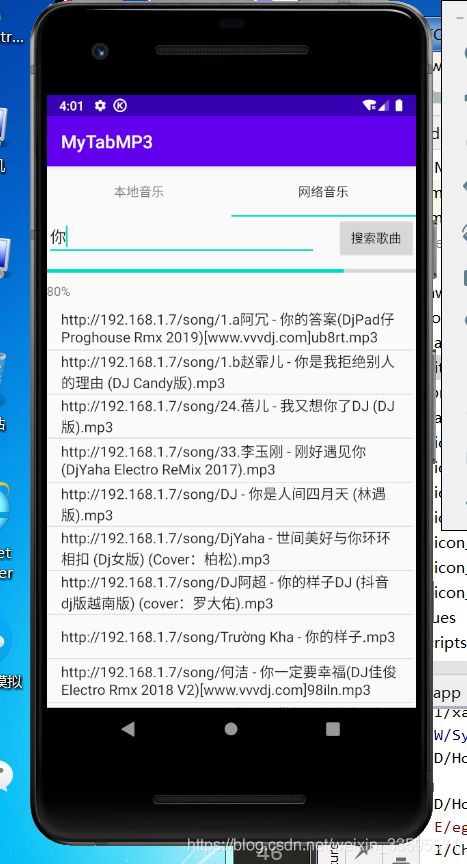