系统共有五大功能:增加医生;删除医生;增加患者;删除患者;查询患者信息。
医生的属性有姓名,性别,科室;患者的属性有姓名,性别,科室,医生。
系统可以通过控制台进行人机交互,选择功能,并管理医生和患者的信息。
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map.Entry;
import java.util.Scanner;
public class Hospital {
public static void main(String[] args) {
LinkedHashMap mapDoctors = new LinkedHashMap();
LinkedHashMap mapPatients = new LinkedHashMap();
initDoctorsAndPatients(mapDoctors, mapPatients);
System.out.println("医院信息系统上线");
System.out.println("=========医生信息========");
printDoctor(mapDoctors);
System.out.println("=========患者信息========");
printPatient(mapPatients);
playSystem(mapDoctors, mapPatients);
}
// 初始信息
public static void initDoctorsAndPatients(LinkedHashMap mapDoctors,
LinkedHashMap mapPatients) {
mapDoctors.put("李敏", new Doctor("李敏", "女", "心脏科"));
mapDoctors.put("王磊", new Doctor("王磊", "男", "皮肤科"));
mapPatients.put("王华", new Patient("王华", "男", "心脏科", new Doctor("李敏", "女", "心脏科")));
mapPatients.put("陆天赐", new Patient("陆天赐", "男", "外科", new Doctor("王怡", "女", "外科")));
}
// 功能设计
public static void playSystem(LinkedHashMap mapDoctors,
LinkedHashMap mapPatients) {
Scanner input = new Scanner(System.in);
Boolean isContinue = true;
while (isContinue) {
System.out.print("功能:1-增加医生 2-删除医生 3-增加患者 4-删除患者 5-查询 6-退出系统" + "\n输入想要使用的功能对应的数字: ");
int num = input.nextInt();
if (num > 6 || num < 1) {
// System.out.print("输入错误!\n请输入1-6之间的数字: ");
// num=input.nextInt();
System.out.println("输入错误!请输入1-6之间的数字 !");
continue;
}
switch (num) {
case 1:
addDoctor(mapDoctors);
System.out.println("=========医生信息========");
printDoctor(mapDoctors);
break;
case 2:
deleteDoctor(mapDoctors);
System.out.println("=========医生信息========");
printDoctor(mapDoctors);
break;
case 3:
addPatient(mapPatients);
System.out.println("=========患者信息========");
printPatient(mapPatients);
break;
case 4:
deletePatient(mapPatients);
System.out.println("=========患者信息========");
printPatient(mapPatients);
break;
case 5:
search(mapPatients);
break;
case 6:
isContinue = false;
quit();
}
}
}
// 功能1-增加医生
public static void addDoctor(LinkedHashMap mapDoctors) {
Scanner input = new Scanner(System.in);
System.out.print("录入医生的个数:");
int number = input.nextInt();
for (int i = 0; i < number; i++) {
System.out.print("录入医生的姓名:");
String n = input.next();
System.out.print("录入医生的性别:");
String s = input.next();
System.out.print("录入医生的科室:");
String r = input.next();
mapDoctors.put(n, new Doctor(n, s, r));
}
}
// 功能2-删除医生
public static void deleteDoctor(LinkedHashMap mapDoctors) {
Scanner input = new Scanner(System.in);
System.out.print("删除医生的个数:");
int number = input.nextInt();
for (int i = 0; i < number; i++) {
System.out.print("删除医生:");
String n = input.next();
mapDoctors.remove(n);
}
}
// 功能3-增加患者
public static void addPatient(LinkedHashMap mapPatients) {
Scanner input = new Scanner(System.in);
System.out.print("录入患者的个数:");
int number = input.nextInt();
for (int i = 0; i < number; i++) {
System.out.print("录入患者的姓名:");
String ns = input.next();
System.out.print("录入患者的性别:");
String ss = input.next();
System.out.print("录入患者的科室:");
String rs = input.next();
System.out.print("录入患者医生的姓名:");
String n = input.next();
System.out.print("录入患者医生的性别:");
String s = input.next();
System.out.print("录入患者医生的科室:");
String r = input.next();
mapPatients.put(ns, new Patient(ns, ss, rs, new Doctor(n, s, r)));
}
}
// 功能4-删除患者
public static void deletePatient(LinkedHashMap mapPatients) {
Scanner input = new Scanner(System.in);
System.out.print("删除患者的个数:");
int number = input.nextInt();
for (int i = 0; i < number; i++) {
System.out.print("删除患者:");
String n = input.next();
mapPatients.remove(n);
}
}
// 功能5-查询
public static void search(LinkedHashMap mapPatients) {
Scanner input = new Scanner(System.in);
System.out.print("查询的患者姓名:");
String name = input.next();
if (mapPatients.get(name) == null) {
System.out.print("系统中没有此患者!\n");
return;
}
System.out.println("患者姓名:" + mapPatients.get(name).name + " 性别:" + mapPatients.get(name).sex + " 科室:"
+ mapPatients.get(name).room + "\n主治医生:" + mapPatients.get(name).doctor.name + " 性别:"
+ mapPatients.get(name).doctor.sex + " 科室:" + mapPatients.get(name).doctor.room);
}
// 功能6-退出系统
public static void quit() {
System.out.println("系统关闭");
System.exit(0);
}
// 打印医生
public static void printDoctor(LinkedHashMap mapDoctors) {
for (Entry entry : mapDoctors.entrySet()) {
System.out.println("医生姓名:" + entry.getKey() + "\n个人信息:" + "姓名:" + entry.getValue().name + " 性别:"
+ entry.getValue().sex + " 科室:" + entry.getValue().room);
}
}
// 打印患者
public static void printPatient(LinkedHashMap mapPatients) {
for (Entry entry : mapPatients.entrySet()) {
System.out.println("患者姓名:" + entry.getKey() + "\n个人信息:" + " 姓名:" + entry.getValue().name + " 性别:"
+ entry.getValue().sex + " 科室:" + entry.getValue().room + "\n主治医生:" + " 姓名:"
+ entry.getValue().doctor.name + " 性别:" + entry.getValue().doctor.sex + " 科室:"
+ entry.getValue().doctor.room);
}
}
}
public class Doctor {
String name;
String sex;
String room;
public Doctor(String name, String sex, String room) {
this.name = name;
this.sex = sex;
this.room = room;
}
}
public class Patient {
String name;
String sex;
String room;
Doctor doctor;
public Patient(String name, String sex, String room, Doctor doctor) {
this.name = name;
this.sex = sex;
this.room = room;
this.doctor = doctor;
}
}
系统运行结果之一
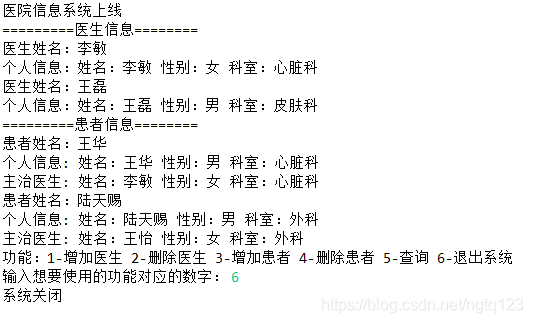