一、首先创建一个web项目,导入SpringMVC所需要的jar包
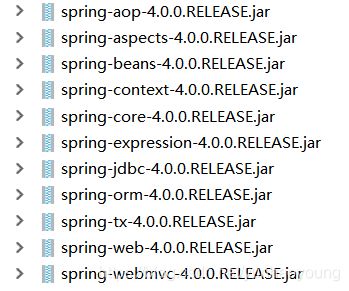
- AOP所需jar包

- Spring日志jar包

- JSTL所需jar包


二、配置web.xml
1. 配置前端处理器
SpringMVC
org.springframework.web.servlet.DispatcherServlet
contextConfigLocation
classpath*:springmvc.xml
SpringMVC
/
2. 配置Spring配置文件
contextConfigLocation
classpath*:beans.xml
三、创建SpringMVC的配置文件,命名为上面param-value标签所配置的名字springmvc.xml
1. 加入命名空间
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
beans>
2. 配置视图解析器
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/jsp/">property>
<property name="suffix" value=".jsp">property>
bean>
3. 配置静态资源所需要的默认处理器DefaultServletHttpRequestHandler
<mvc:default-servlet-handler>mvc:default-servlet-handler>
4. 当我们使用了这个配置,其他的RequeMapping请求会失效,需要加上如下配置
<mvc:annotation-driven>mvc:annotation-driven>
5. 配置包扫描
<context:component-scan base-package="com.github">context:component-scan>
四、创建Spring配置文件,beans.xml
- Spring的配置文件用于整合第三方框架,例如Mybatis
1. 加入命名空间
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
beans>
2. 配置包扫描
<context:component-scan base-package="com.github">context:component-scan>
- 注意:当我们在Spring的配置文件和SpringMVC配置文件中同时配置包扫描的时候,会将bean创建俩次
3.Spring 的 IOC 容器不应该扫描 SpringMVC 中的 bean, 对应的SpringMVC 的 IOC 容器不应该扫描 Spring 中的 bean,如何解决
<context:component-scan base-package="com.github">
<context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller">context:exclude-filter>
<context:exclude-filter type="annotation" expression="org.springframework.web.bind.annotation.ControllerAdvice">context:exclude-filter>
context:component-scan>
<context:component-scan base-package="com.github">
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller">context:include-filter>
<context:include-filter type="annotation" expression="org.springframework.web.bind.annotation.ControllerAdvice">context:include-filter>
context:component-scan>
五、创建PO类
public class Employee {
private Integer id;
private String lastName;
private String email;
private Integer gender;
private Department department;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", lastName='" + lastName + '\'' +
", email='" + email + '\'' +
", gender=" + gender +
", department=" + department +
'}';
}
public Employee(Integer id, String lastName, String email, Integer gender,
Department department) {
super();
this.id = id;
this.lastName = lastName;
this.email = email;
this.gender = gender;
this.department = department;
}
public Employee() {
}
}
public class Department {
private Integer id;
private String departmentName;
public Department() {
}
public Department(int i, String string) {
this.id = i;
this.departmentName = string;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getDepartmentName() {
return departmentName;
}
public void setDepartmentName(String departmentName) {
this.departmentName = departmentName;
}
@Override
public String toString() {
return "Department [id=" + id + ", departmentName=" + departmentName
+ "]";
}
}
六、创建Dao
- 这里我们模拟一个数据库,没有真正存入到数据库,我们也可以导入Mybatis的jar包,持久化到数据库
- EmployeeDao
@Repository
public class EmployeeDao {
private static Map<Integer, Employee> employees = null;
@Autowired
private DepartmentDao departmentDao;
static{
employees = new HashMap<Integer, Employee>();
employees.put(1001, new Employee(1001, "E-AA", "[email protected]", 1, new Department(101, "D-AA")));
employees.put(1002, new Employee(1002, "E-BB", "[email protected]", 1, new Department(102, "D-BB")));
employees.put(1003, new Employee(1003, "E-CC", "[email protected]", 0, new Department(103, "D-CC")));
employees.put(1004, new Employee(1004, "E-DD", "[email protected]", 0, new Department(104, "D-DD")));
employees.put(1005, new Employee(1005, "E-EE", "[email protected]", 1, new Department(105, "D-EE")));
}
private static Integer initId = 1006;
public void save(Employee employee){
if(employee.getId() == null){
employee.setId(initId++);
}
employee.setDepartment(departmentDao.getDepartment(employee.getDepartment().getId()));
employees.put(employee.getId(), employee);
}
public Collection<Employee> getAll(){
return employees.values();
}
public Employee get(Integer id){
return employees.get(id);
}
public void delete(Integer id){
employees.remove(id);
}
}
@Repository
public class DepartmentDao {
private static Map<Integer, Department> departments = null;
static{
departments = new HashMap<Integer, Department>();
departments.put(101, new Department(101, "D-AA"));
departments.put(102, new Department(102, "D-BB"));
departments.put(103, new Department(103, "D-CC"));
departments.put(104, new Department(104, "D-DD"));
departments.put(105, new Department(105, "D-EE"));
}
public Collection<Department> getDepartments(){
return departments.values();
}
public Department getDepartment(Integer id){
return departments.get(id);
}
}
七、创建Controller
- 我们只做CRUD,比较简单,没有业务,这里就不写Service了
@Controller
public class EmployeeController {
@Autowired
DepartmentDao departmentDao;
@Autowired
EmployeeDao employeeDao;
@RequestMapping(value="/emps", method=RequestMethod.GET)
public String showlist(Map<String, Object> map){
map.put("employees", employeeDao.getAll());
return "show";
}
@RequestMapping(value = "/emp/{id}", method=RequestMethod.DELETE)
public String emp(@PathVariable("id") Integer id){
employeeDao.delete(id);
return "redirect:/emps";
}
@RequestMapping(value="/emp", method=RequestMethod.GET)
public String input(Map<String, Object> map){
map.put("departments", departmentDao.getDepartments());
map.put("employee", new Employee());
return "input";
}
@RequestMapping(value="/emp/{id}", method=RequestMethod.GET)
public String input(@PathVariable("id") Integer id,Map<String,Object> map){
map.put("employee", employeeDao.get(id));
map.put("departments", departmentDao.getDepartments());
return "input";
}
@RequestMapping(value = "/emp", method = RequestMethod.POST)
public String sava(Employee employee){
employeeDao.save(employee);
return "redirect:/emps";
}
@ModelAttribute
public void getEmployee(@RequestParam(value="id",required=false) Integer id,
Map<String, Object> map){
if(id != null){
map.put("employee", employeeDao.get(id));
}
}
@RequestMapping(value = "/emp", method = RequestMethod.PUT)
public String update(Employee employee){
employeeDao.save(employee);
return "redirect:/emps";
}
}
八、jsp代码
<%@ page contentType="text/html;charset=UTF-8" language="java" pageEncoding="UTF-8"%>
<html>
<head>
<title>title>
head>
<body>
<a href="/emps">列表展示a>
body>
html>
<%@ page contentType="text/html;charset=UTF-8" language="java" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Titletitle>
<script type="text/javascript" src="scripts/jquery-1.9.1.min.js">script>
<script type="text/javascript">
$(function(){
$(".delete").click(function(){
var href = $(this).attr("href");
$("form").attr("action", href).submit();
return false;
});
})
script>
head>
<body>
<form action="" method="POST">
<input type="hidden" name="_method" value="DELETE"/>
form>
<c:if test="${empty requestScope.employees }">
没有任何员工信息.
c:if>
<c:if test="${!empty requestScope.employees }">
<table border="1" cellpadding="10" cellspacing="0">
<tr>
<th>IDth>
<th>LastNameth>
<th>Emailth>
<th>Genderth>
<th>Departmentth>
<th>Editth>
<th>Deleteth>
tr>
<c:forEach items="${requestScope.employees }" var="emp">
<tr>
<td>${emp.id }td>
<td>${emp.lastName }td>
<td>${emp.email }td>
<td>${emp.gender == 0 ? 'Female' : 'Male' }td>
<td>${emp.department.departmentName }td>
<td><a href="emp/${emp.id}">修改a>td>
<td><a class="delete" href="emp/${emp.id}">删除a>td>
tr>
c:forEach>
table>
c:if>
<br><br>
<a href="emp">添加员工a>
body>
html>
<%@ page contentType="text/html;charset=UTF-8" language="java" pageEncoding="UTF-8" %>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Titletitle>
head>
<body>
<form:form action="${pageContext.request.contextPath }/emp" method="POST"
modelAttribute="employee">
<c:if test="${employee.id == null }">
LastName: <form:input path="lastName"/>
c:if>
<c:if test="${employee.id != null }">
<form:hidden path="id"/>
<input type="hidden" name="_method" value="PUT"/>
c:if>
<br>
Email: <form:input path="email"/>
<br>
<%
Map<String, String> genders = new HashMap();
genders.put("1", "Male");
genders.put("0", "Female");
request.setAttribute("genders", genders);
%>
Gender:
<br>
<form:radiobuttons path="gender" items="${genders }" delimiter="
"/>
<br>
Department: <form:select path="department.id"
items="${departments }" itemLabel="departmentName" itemValue="id">form:select>
<br>
<input type="submit" value="Submit"/>
form:form>
body>
html>
九、分析
1. SpringMVC请求不支持PUT、DELETE,如何解决
<filter>
<filter-name>HiddenHttpMethodFilterfilter-name>
<filter-class>org.springframework.web.filter.HiddenHttpMethodFilterfilter-class>
filter>
<filter-mapping>
<filter-name>HiddenHttpMethodFilterfilter-name>
<url-pattern>/*url-pattern>
filter-mapping>
- 然后为修改、删除绑定一个input的隐藏域,name为"_method"
1.1 修改
<input type="hidden" name="_method" value="PUT"/>
1.2 删除
- 这里用到了jQuery,需要导入jQuery的包

- 创建一个表单
<form action="" method="POST">
<input type="hidden" name="_method" value="DELETE"/>
form>
<script type="text/javascript" src="scripts/jquery-1.9.1.min.js"></script>
<script type="text/javascript">
$(function(){
$(".delete").click(function(){
var href = $(this).attr("href");
$("form").attr("action", href).submit();
return false;
});
})
</script>
2. 当我们添加用户时,会出现中文乱码问题,如何解决
<filter>
<filter-name>characterEncodingFilterfilter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilterfilter-class>
<init-param>
<param-name>encodingparam-name>
<param-value>UTF-8param-value>
init-param>
<init-param>
<param-name>forceEncodingparam-name>
<param-value>trueparam-value>
init-param>
filter>
<filter-mapping>
<filter-name>characterEncodingFilterfilter-name>
<url-pattern>/*url-pattern>
filter-mapping>
- 注意:这个Filter需要配置到最前面,否则有可能失效,因为Filter有先后