文章目录
-
- 9.1
- 9.4
- 9.5
- 9.9
- 9.12
- 9.13
9.1
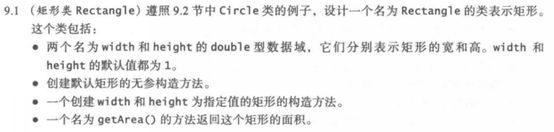

package wwr;
class Rectangle {
double width;
double height;
Rectangle() {
width = 1;
height = 1;
}
Rectangle(double newWidth, double newHeight) {
width = newWidth;
height = newHeight;
}
double getArea() {
return width * height;
}
double getPerimeter() {
return 2 * (width + height);
}
}
public class Demo {
public static void main(String[] args) {
Rectangle rectangle1 = new Rectangle(4, 40);
System.out.println("The width of rectangle1 is " + rectangle1.width);
System.out.println("The height of rectangle1 is " + rectangle1.height);
System.out.println("The area of rectangle1 is " + rectangle1.getArea());
System.out.println("The perimeterof rectangle1 is " + rectangle1.getPerimeter());
Rectangle rectangle2 = new Rectangle(3.5, 35.9);
System.out.println("The width of rectangle2 is " + rectangle2.width);
System.out.println("The height of rectangle2 is " + rectangle2.height);
System.out.println("The area of rectangle2 is " + rectangle2.getArea());
System.out.println("The perimeterof rectangle2 is " + rectangle2.getPerimeter());
}
}
9.4

package wwr;
import java.util.Random;
public class Demo {
public static void main(String[] args) {
Random random = new java.util.Random(1000);
for(int i = 0; i < 50; i++) {
System.out.print(random.nextInt(100) + " ");
}
}
}
9.5
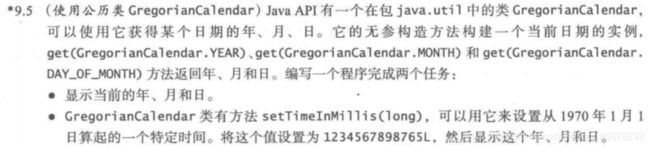
package wwr;
import java.util.GregorianCalendar;
public class Demo {
public static void main(String[] args) {
GregorianCalendar calendar = new GregorianCalendar();
System.out.print(calendar.get(GregorianCalendar.YEAR) +
" " + (calendar.get(GregorianCalendar.MONTH) + 1) +
" " + calendar.get(GregorianCalendar.DAY_OF_MONTH));
System.out.println();
calendar.setTimeInMillis(1234567898765L);
System.out.print(calendar.get(GregorianCalendar.YEAR) +
" " + (calendar.get(GregorianCalendar.MONTH) + 1) +
" " + calendar.get(GregorianCalendar.DAY_OF_MONTH));
}
}
9.9
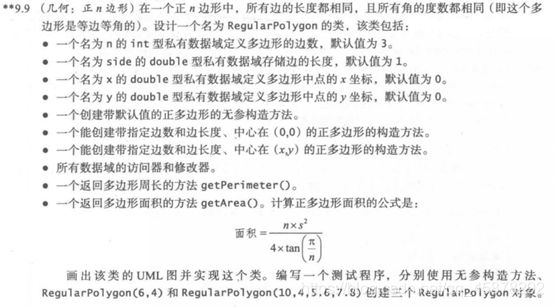

package wwr;
class RegularPolygon {
private int n;
private double side;
private double x;
private double y;
RegularPolygon() {
n = 3;
side = 1;
x = 0;
y = 0;
}
RegularPolygon(int newN, double newSide) {
n = newN;
side = newSide;
x = 0;
y = 0;
}
RegularPolygon(int newN, double newSide, double newX, double newY) {
n = newN;
side = newSide;
x = newX;
y = newY;
}
int getN() {
return n;
}
double getSide() {
return side;
}
double getX() {
return x;
}
double getY() {
return y;
}
void setN(int newN) {
n = newN;
}
void setSide(double newSide) {
side = newSide;
}
void setX(double newX) {
x = newX;
}
void setY(double newY) {
y = newY;
}
double getPerimeter() {
return side * n;
}
double getArea() {
return (n * side * side) / (4 * Math.tan(Math.PI / n));
}
}
public class Demo {
public static void main(String[] args) {
RegularPolygon rp1 = new RegularPolygon();
RegularPolygon rp2 = new RegularPolygon(6, 4);
RegularPolygon rp3 = new RegularPolygon(10, 4, 5.6, 7.8);
System.out.println("rp1's perimeter is " +
rp1.getPerimeter() + ", rp1's area is " + rp1.getArea());
System.out.println("rp2's perimeter is " +
rp2.getPerimeter() + ", rp2's area is " + rp2.getArea());
System.out.println("rp3's perimeter is " +
rp3.getPerimeter() + ", rp3's area is " + rp3.getArea());
}
}
9.12

package wwr;
import java.util.Scanner;
class LinearEquation {
private double a;
private double b;
private double c;
private double d;
private double e;
private double f;
LinearEquation(double a, double b, double c, double d, double e, double f) {
this.a = a;
this.b = b;
this.c = c;
this.d = d;
this.e = e;
this.f = f;
}
double getA() {
return a;
}
double getB() {
return b;
}
double getC() {
return c;
}
double getD() {
return d;
}
double getE() {
return e;
}
double getF() {
return f;
}
double getX() {
return (e * d - b * f) / (a * d - b * c) ;
}
double getY() {
return (a * f - e * c) / (a * d - b * c);
}
}
public class Demo {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the two endpoints of the first line segment (x1, y1, x2, y2): ");
double x1 = input.nextInt();
double y1 = input.nextInt();
double x2 = input.nextInt();
double y2 = input.nextInt();
double a = (y1 - y2) / (x2 - x1);
double b = 1;
double e = y1 - ((x1 * (y2 - y1)) / (x2 - x1));
System.out.print("Enter the two endpoints of the second line segment (x3, y3, x4, y4): ");
double x3 = input.nextInt();
double y3 = input.nextInt();
double x4 = input.nextInt();
double y4 = input.nextInt();
double c = (y3 - y4) / (x4 - x3);
double d = 1;
double f = y3 - ((x3 * (y4 - y3)) / (x4 - x3));
if(a * d - b * c == 0)
System.out.println("The equation has no solution");
else {
LinearEquation le = new LinearEquation(a, b, c, d, e, f);
double tepX = le.getX();
double tepY = le.getY();
System.out.print("x is " + tepX + " and y is " + tepY);
}
}
}
9.13
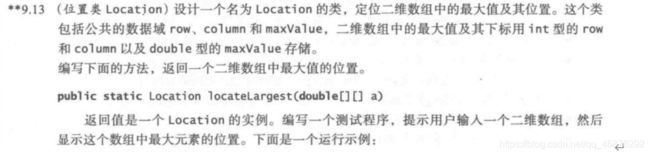
package wwr;
import java.util.Scanner;
class Location {
public int row;
public int column;
public double maxValue;
public static Location locateLargest(double[][] a) {
Location lo = new Location();
lo.maxValue = a[0][0];
for(int i = 0; i < a.length; i++)
for(int j = 0; j < a[0].length; j++ )
if(a[i][j] > lo.maxValue) {
lo.maxValue = a[i][j];
lo.row = i;
lo.column = j;
}
return lo;
}
}
public class Demo {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter the number of rows and columns in the array: ");
int Row = input.nextInt();
int Column = input.nextInt();
System.out.println("Enter the array: ");
double array[][] = new double[Row + 2][Column + 2];
for(int i = 0; i < Row; i++)
for(int j = 0; j < Column; j++)
array[i][j] = input.nextDouble();
Location LO = new Location();
LO = LO.locateLargest(array);
System.out.print("The location of the largest element is " + LO.maxValue
+ " at (" + LO.row + ", " + LO.column + ")");
}
}